
SVM Regression in Machine Learning: Understanding the Basics
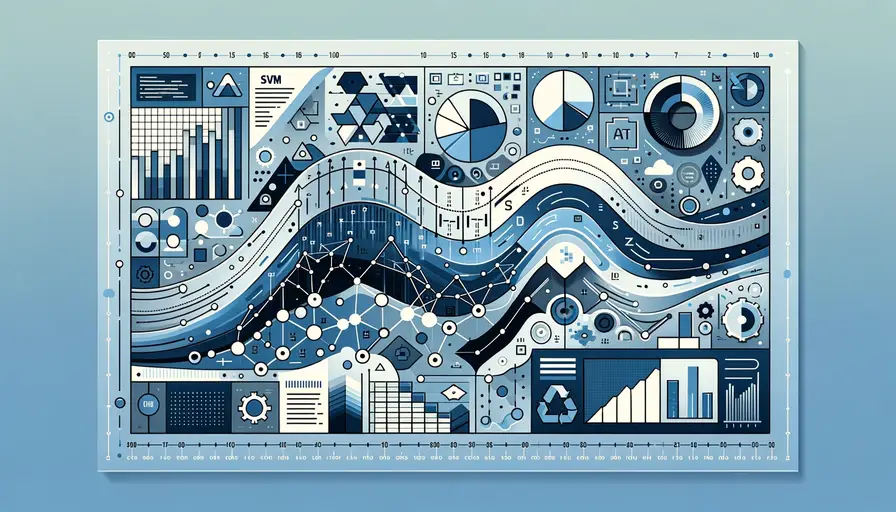
Support Vector Machines (SVM) are a set of supervised learning methods used for classification, regression, and outliers detection. While SVM is predominantly known for its application in classification tasks, it is also a powerful tool for regression. This article delves into the basics of SVM regression, highlighting its importance, explaining key concepts, and providing practical examples using scikit-learn.
Fundamentals of SVM Regression
Concept of Support Vector Regression (SVR)
Support Vector Regression (SVR) is an extension of SVM for regression tasks. Unlike classification, where the objective is to find a hyperplane that best separates the classes, regression aims to find a function that approximates the relationship between input variables and the continuous output variable. SVR uses the same principles as SVM but modifies them to predict continuous values rather than classes.
SVR tries to fit the best line within a threshold value \(\epsilon\), where the goal is to ensure that the error of prediction is within this threshold. The objective function of SVR not only tries to minimize the error but also ensures that the flatness of the regression line is maximized.
Here is a simple example of how SVR works:
Machine Learning Models that Require Feature Scalingfrom sklearn.svm import SVR
import numpy as np
import matplotlib.pyplot as plt
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1.2, 1.9, 3.0, 4.1, 5.1])
# Fit the model
model = SVR(kernel='linear')
model.fit(X, y)
# Predictions
X_test = np.linspace(0, 6, 100).reshape(-1, 1)
y_pred = model.predict(X_test)
# Plot the results
plt.scatter(X, y, color='red', label='Data Points')
plt.plot(X_test, y_pred, color='blue', label='SVR Fit')
plt.xlabel('X')
plt.ylabel('y')
plt.title('SVR Example')
plt.legend()
plt.show()
This code demonstrates the basics of fitting an SVR model to a simple dataset and visualizing the fitted regression line.
Importance of Kernel Functions
Kernel functions are a crucial component of SVR as they enable the model to fit complex data. The kernel trick allows SVR to operate in a high-dimensional space without explicitly computing the coordinates of the data in that space, making the computation efficient. Common kernels include linear, polynomial, and radial basis function (RBF).
- Linear Kernel: Suitable for linearly separable data.
- Polynomial Kernel: Suitable for polynomial relationships between features.
- RBF Kernel: Suitable for non-linear relationships and is the most commonly used kernel in SVR.
Here is an example of using different kernels in SVR:
from sklearn.svm import SVR
import numpy as np
import matplotlib.pyplot as plt
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1.2, 1.9, 3.0, 4.1, 5.1])
# Fit models with different kernels
kernels = ['linear', 'poly', 'rbf']
predictions = {}
for kernel in kernels:
model = SVR(kernel=kernel, degree=3 if kernel == 'poly' else None)
model.fit(X, y)
predictions[kernel] = model.predict(X)
# Plot the results
plt.scatter(X, y, color='red', label='Data Points')
for kernel, y_pred in predictions.items():
plt.plot(X, y_pred, label=f'SVR {kernel} Kernel')
plt.xlabel('X')
plt.ylabel('y')
plt.title('SVR with Different Kernels')
plt.legend()
plt.show()
This code shows how to fit SVR models with different kernels and visualize their predictions.
Anomaly Detection with Logistic Regression in MLParameters of SVR
SVR involves several parameters that need tuning to optimize model performance. Key parameters include:
- C (Regularization Parameter): Controls the trade-off between achieving a low error on the training data and minimizing the model complexity.
- epsilon (ε): Defines the margin of tolerance where no penalty is given to errors.
- Kernel Parameters: Specific to the chosen kernel, such as the degree for polynomial kernels or gamma for RBF kernels.
Here is an example of tuning SVR parameters:
from sklearn.model_selection import GridSearchCV
from sklearn.svm import SVR
import numpy as np
import pandas as pd
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1.2, 1.9, 3.0, 4.1, 5.1])
# Parameter grid
param_grid = {
'C': [0.1, 1, 10],
'epsilon': [0.1, 0.2, 0.5],
'kernel': ['linear', 'poly', 'rbf'],
'degree': [2, 3] if 'poly' in ['poly'] else [None],
'gamma': ['scale', 'auto'] if 'rbf' in ['rbf'] else [None]
}
# Fit model using GridSearchCV
svr = SVR()
grid_search = GridSearchCV(estimator=svr, param_grid=param_grid, cv=3)
grid_search.fit(X, y)
# Best parameters
print(f"Best parameters: {grid_search.best_params_}")
This code demonstrates how to use GridSearchCV to tune the parameters of an SVR model.
Implementing SVR in Scikit-learn
Preparing the Data
Before implementing SVR, it's essential to prepare the data properly. This includes handling missing values, encoding categorical features, and scaling numerical features. Proper data preparation ensures that the model can learn effectively from the data.
Unleashing Machine Learning: Mastering Validation TechniquesHere is an example of preparing data for SVR:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Load dataset
data = pd.read_csv('your_dataset.csv')
X = data.drop('target', axis=1)
y = data['target']
# Handle missing values
X.fillna(X.mean(), inplace=True)
# Encode categorical features
X = pd.get_dummies(X, drop_first=True)
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Scale numerical features
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
This code shows how to prepare data by handling missing values, encoding categorical features, and scaling numerical features.
Training the SVR Model
Once the data is prepared, the next step is to train the SVR model. This involves fitting the model to the training data and evaluating its performance on the testing data.
Here is an example of training an SVR model:
The Role of Weights in Machine Learning: Purpose and Applicationfrom sklearn.svm import SVR
from sklearn.metrics import mean_squared_error, r2_score
# Train the SVR model
model = SVR(kernel='rbf', C=1.0, epsilon=0.1)
model.fit(X_train_scaled, y_train)
# Make predictions
y_pred_train = model.predict(X_train_scaled)
y_pred_test = model.predict(X_test_scaled)
# Evaluate the model
mse_train = mean_squared_error(y_train, y_pred_train)
r2_train = r2_score(y_train, y_pred_train)
mse_test = mean_squared_error(y_test, y_pred_test)
r2_test = r2_score(y_test, y_pred_test)
print(f"Training MSE: {mse_train}, R2: {r2_train}")
print(f"Testing MSE: {mse_test}, R2: {r2_test}")
This code demonstrates how to train an SVR model and evaluate its performance using metrics such as mean squared error and R-squared.
Cross-Validation
Cross-validation is a crucial technique for assessing the generalizability of the model. It involves partitioning the data into multiple folds and training the model on each fold, ensuring that the model's performance is not overly dependent on any single subset of the data.
Here is an example of implementing cross-validation for SVR:
from sklearn.model_selection import cross_val_score
import numpy as np
# Cross-validation scores
cv_scores = cross_val_score(model, X_train_scaled, y_train, cv=5, scoring='neg_mean_squared_error')
cv_scores_mean = np.mean(cv_scores)
cv_scores_std = np.std(cv_scores)
print(f"Cross-Validation MSE: Mean={-cv_scores_mean}, Std={cv_scores_std}")
This code shows how to perform cross-validation for an SVR model, providing insights into the model's stability and reliability.
Popular R Packages for Machine Learning Variable SelectionAdvanced Topics in SVR
Handling Non-Linearity with Kernels
Non-linear relationships between features and the target variable can be effectively handled using kernel functions in SVR. The choice of kernel significantly impacts the model's ability to capture complex patterns in the data.
Here is an example of using a non-linear kernel (RBF) in SVR:
from sklearn.svm import SVR
from sklearn.metrics import mean_squared_error, r2_score
# Train the SVR model with RBF kernel
model_rbf = SVR(kernel='rbf', C=1.0, epsilon=0.1, gamma='scale')
model_rbf.fit(X_train_scaled, y_train)
# Make predictions
y_pred_test_rbf = model_rbf.predict(X_test_scaled)
# Evaluate the model
mse_test_rbf = mean_squared_error(y_test, y_pred_test_rbf)
r2_test_rbf = r2_score(y_test, y_pred_test_rbf)
print(f"Testing MSE (RBF Kernel): {mse_test_rbf}, R2: {r2_test_rbf}")
This code demonstrates how to use an RBF kernel in SVR to handle non-linear relationships.
Feature Importance and Selection
Understanding which features contribute most to the model's predictions can provide valuable insights and improve model performance. Feature selection techniques can be used to identify and retain the most important features, reducing overfitting and improving interpretability.
Comparing Machine Learning Models in R: A Guide to Choose the BestHere is an example of feature selection using Recursive Feature Elimination (RFE) with SVR:
from sklearn.feature_selection import RFE
# Perform RFE with SVR
model = SVR(kernel='linear')
selector = RFE(model, n_features_to_select=5, step=1)
selector.fit(X_train_scaled, y_train)
# Selected features
selected_features = X_train.columns[selector.support_]
print(f"Selected Features: {selected_features}")
# Train and evaluate the model with selected features
X_train_selected = selector.transform(X_train_scaled)
X_test_selected = selector.transform(X_test_scaled)
model.fit(X_train_selected, y_train)
y_pred_test_selected = model.predict(X_test_selected)
# Evaluate the model
mse_test_selected = mean_squared_error(y_test, y_pred_test_selected)
r2_test_selected = r2_score(y_test, y_pred_test_selected)
print(f"Testing MSE (Selected Features): {mse_test_selected}, R2: {r2_test_selected}")
This code demonstrates how to perform feature selection using RFE with SVR and evaluate the model with the selected features.
Model Deployment and Scalability
Deploying an SVR model involves integrating it into a production environment where it can make real-time predictions on new data. Flask is a lightweight web framework that can be used to deploy machine learning models as web services.
Here is an example of deploying an SVR model with Flask:
from flask import Flask, request, jsonify
import joblib
import numpy as np
app = Flask(__name__)
# Load the trained model
model = joblib.load('svr_model.pkl')
@app.route('/')
def home():
return "Welcome to the SVR Model Deployment!"
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json()
features = np.array(data['features']).reshape(1, -1)
prediction = model.predict(features)
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(debug=True)
This code sets up a Flask application that loads a pre-trained SVR model and provides an endpoint for making predictions.
By understanding the basics of SVR, implementing it using scikit-learn, and exploring advanced topics such as kernel functions, feature selection, and model deployment, you can leverage the power of SVM regression to solve complex regression tasks effectively. Whether you are handling linear or non-linear data, SVR offers a robust and flexible approach to modeling continuous outcomes in machine learning.
If you want to read more articles similar to SVM Regression in Machine Learning: Understanding the Basics, you can visit the Algorithms category.
You Must Read