
The Advantages of Spiking Neural Networks for Machine Learning
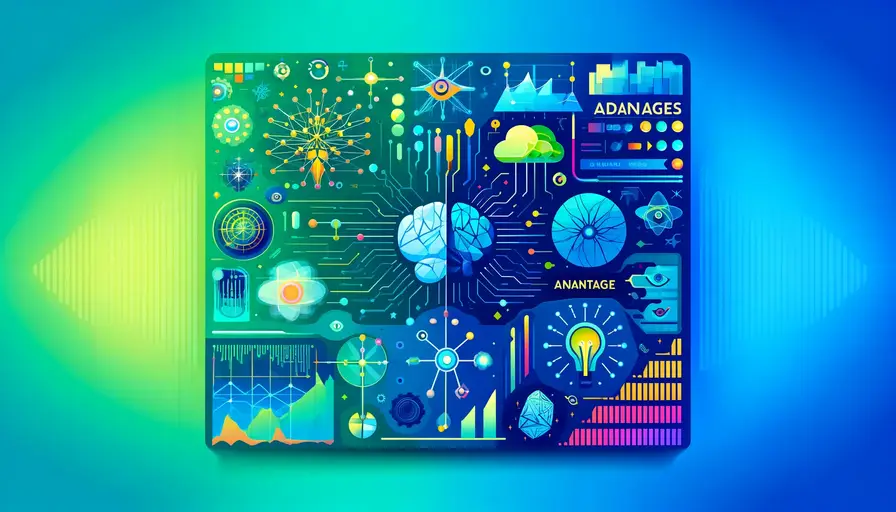
Understanding Spiking Neural Networks
Characteristics of Spiking Neural Networks
Spiking Neural Networks (SNNs) represent a significant shift in the way neural networks are modeled, incorporating elements of biological neurons to create more sophisticated and energy-efficient computational systems. Unlike traditional artificial neural networks (ANNs) that use continuous values for processing information, SNNs use discrete events called spikes to transmit information between neurons. This event-driven approach allows SNNs to more closely mimic the functioning of biological brains, making them a powerful tool for machine learning.
The main characteristic that sets SNNs apart from traditional neural networks is their temporal dynamics. Neurons in SNNs fire spikes only when their membrane potential reaches a certain threshold. This behavior introduces a temporal dimension to the computation, allowing the network to encode information not just in the firing rates but also in the precise timing of spikes. This temporal aspect enables SNNs to process time-dependent data more effectively, making them suitable for applications like speech and sound recognition, robotic control, and real-time signal processing.
Another key feature of SNNs is their sparsity in communication. Since neurons fire only when necessary, the overall number of spikes—and hence the computational and energy cost—can be significantly reduced. This sparsity can lead to more efficient use of resources, particularly in hardware implementations like neuromorphic chips. The event-driven nature of SNNs aligns well with the asynchronous processing capabilities of neuromorphic hardware, further enhancing their efficiency.
Applications of Spiking Neural Networks
Spiking Neural Networks have a wide range of applications due to their unique characteristics. In robotics, SNNs are used to control autonomous systems, leveraging their ability to process sensory information in real time and adapt to changing environments. The temporal coding and efficiency of SNNs enable robots to perform complex tasks like navigation, object recognition, and interaction with humans more effectively.
The Potential of Automated Machine LearningIn the field of neuroscience, SNNs are employed to model and understand the functioning of the human brain. By replicating the spiking behavior of biological neurons, researchers can study neural mechanisms and disorders more accurately. SNNs are also used in brain-computer interfaces (BCIs) to decode neural signals and translate them into commands for external devices, offering new possibilities for individuals with disabilities.
Another promising application of SNNs is in the development of low-power, energy-efficient computing systems. Neuromorphic hardware designed to run SNNs can significantly reduce power consumption compared to traditional digital processors. This capability is particularly valuable for edge computing and IoT devices, where energy efficiency is crucial. Additionally, SNNs are explored in fields like financial modeling, where the ability to process and predict time-series data can provide a competitive edge.
Example: Implementing a Simple Spiking Neuron Model
import numpy as np
import matplotlib.pyplot as plt
# Parameters
T = 1000 # total time in ms
dt = 1 # time step in ms
time = np.arange(0, T, dt)
tau_m = 10 # membrane time constant in ms
V_reset = -65 # reset potential in mV
V_thresh = -50 # threshold potential in mV
R_m = 1 # membrane resistance in ohms
I = 1.5 # input current in nA
# Initialize variables
V = np.zeros(len(time))
V[0] = V_reset
spike_times = []
# Simulate spiking neuron
for t in range(1, len(time)):
dV = (-(V[t-1] - V_reset) + R_m * I) / tau_m * dt
V[t] = V[t-1] + dV
if V[t] >= V_thresh:
V[t] = V_reset
spike_times.append(t * dt)
# Plot membrane potential
plt.figure(figsize=(10, 4))
plt.plot(time, V, label="Membrane potential")
plt.scatter(spike_times, [V_thresh]*len(spike_times), color='red', label="Spikes")
plt.xlabel("Time (ms)")
plt.ylabel("Membrane potential (mV)")
plt.title("Simple Spiking Neuron Model")
plt.legend()
plt.show()
In this example, Python is used to implement a simple spiking neuron model. The model simulates the behavior of a neuron that fires spikes when its membrane potential exceeds a threshold. This simulation illustrates the temporal dynamics of spiking neurons and their ability to encode information in the timing of spikes.
Advantages of Spiking Neural Networks
Energy Efficiency
One of the most significant advantages of Spiking Neural Networks is their energy efficiency. Unlike traditional neural networks that continuously update neuron states, SNNs operate on an event-driven basis. Neurons in SNNs only perform computations when they receive spikes, which drastically reduces the number of operations and consequently the energy consumption. This efficiency makes SNNs particularly well-suited for deployment in low-power environments, such as IoT devices and edge computing systems.
Using NLP and Machine Learning in R for Effective Data AnalysisThe sparse activity in SNNs means that the computational load is distributed over time, reducing the peak power requirements. Neuromorphic hardware, designed to run SNNs, leverages this sparsity to achieve further energy savings. Chips like Intel’s Loihi and IBM’s TrueNorth are examples of such hardware, which mimic the brain’s architecture to achieve high efficiency and performance.
The energy efficiency of SNNs is not only beneficial for portable and battery-operated devices but also for large-scale data centers where power consumption is a critical concern. By adopting SNNs, data centers can reduce their energy footprint, leading to cost savings and a lower environmental impact. This makes SNNs a promising technology for sustainable computing.
Real-Time Processing
Spiking Neural Networks excel in real-time processing due to their inherent temporal dynamics. The ability to encode information in the timing of spikes allows SNNs to process and respond to inputs with minimal latency. This capability is crucial for applications that require immediate reactions, such as autonomous driving, robotic control, and real-time surveillance systems.
In robotics, for example, SNNs enable robots to process sensory inputs and make decisions on the fly. The event-driven nature of SNNs ensures that computations are performed only when necessary, leading to faster response times compared to traditional neural networks. This real-time processing capability enhances the robot’s ability to navigate dynamic environments, avoid obstacles, and interact with objects and humans.
IBM's Machine Learning vs AI: Who Reigns Supreme?In auditory and visual processing, SNNs can efficiently handle continuous streams of data, such as speech or video, and detect patterns in real-time. This makes them suitable for applications like speech recognition, where timely and accurate interpretation of spoken words is essential. Additionally, SNNs are used in real-time anomaly detection systems, where they can quickly identify deviations from normal patterns, providing timely alerts and responses.
Example: Real-Time Signal Processing with SNNs
import numpy as np
# Generate a noisy signal
time = np.linspace(0, 1, 1000)
signal = np.sin(2 * np.pi * 10 * time) + np.random.normal(0, 0.5, time.shape)
# Simple spiking neuron for real-time signal processing
tau_m = 10 # membrane time constant in ms
V_reset = -65 # reset potential in mV
V_thresh = -50 # threshold potential in mV
R_m = 1 # membrane resistance in ohms
# Initialize variables
V = V_reset
spike_times = []
# Process signal
for t in range(len(signal)):
dV = (-(V - V_reset) + R_m * signal[t]) / tau_m
V += dV
if V >= V_thresh:
V = V_reset
spike_times.append(t)
# Plot original signal and spike times
import matplotlib.pyplot as plt
plt.figure(figsize=(10, 4))
plt.plot(time, signal, label="Original signal")
plt.scatter(np.array(spike_times) / 1000, [V_thresh]*len(spike_times), color='red', label="Spikes")
plt.xlabel("Time (s)")
plt.ylabel("Signal amplitude")
plt.title("Real-Time Signal Processing with SNNs")
plt.legend()
plt.show()
In this example, a simple spiking neuron model is used for real-time signal processing. The neuron processes a noisy input signal and fires spikes based on the signal amplitude. The spikes indicate significant changes in the signal, demonstrating the ability of SNNs to process and detect patterns in real-time data.
Robustness and Fault Tolerance
Spiking Neural Networks offer enhanced robustness and fault tolerance compared to traditional neural networks. The event-driven nature and sparse communication of SNNs contribute to their resilience, making them capable of handling noise and partial failures without significant degradation in performance. This robustness is particularly valuable in real-world applications where data is often noisy and systems must operate reliably under various conditions.
In neuromorphic hardware, the decentralized and parallel architecture of SNNs allows the system to continue functioning even if some components fail. This fault tolerance is akin to the resilience observed in biological brains, where the failure of individual neurons does not lead to the collapse of the entire system. As a result, SNNs can maintain their performance and continue processing information even in the presence of hardware faults or communication errors.
The Evolution of Machine Learning: A Brief History and TimelineThe robustness of SNNs also extends to their ability to generalize from limited and noisy data. The temporal coding and adaptive learning mechanisms in SNNs enable them to extract meaningful patterns from noisy inputs, improving their generalization capabilities. This makes SNNs particularly suitable for applications in uncertain and dynamic environments, where the ability to adapt and learn from noisy data is crucial.
Implementing Spiking Neural Networks
Software Tools for SNNs
Several software tools are available for implementing Spiking Neural Networks, providing researchers and developers with the resources to design, simulate, and deploy SNN models. One popular tool is NEST, a highly scalable simulation software designed for large-scale neural network simulations. NEST supports the simulation of diverse neuron and synapse models, making it a versatile tool for exploring the dynamics of SNNs.
Another widely used tool is Brian, a simulator for spiking neural networks that emphasizes simplicity and flexibility. Brian is particularly suitable for small to medium-sized networks and provides a user-friendly interface for defining and running SNN simulations. The tool is designed to be accessible for researchers with varying levels of programming experience, making it a valuable resource for educational purposes.
For those interested in neuromorphic hardware, NESTML provides a modeling language for the NEST simulator, enabling the creation of models that can be deployed on neuromorphic platforms. Additionally, SpiNNaker is a hardware platform specifically designed for real-time simulation of SNNs, offering a unique environment for developing and testing neuromorphic applications.
Machine Learning: A Comprehensive Analysis of Data-driven LearningNeuromorphic Hardware
Neuromorphic hardware is designed to mimic the structure and function of the human brain, providing an efficient platform for running Spiking Neural Networks. These hardware systems leverage the event-driven nature and parallel processing capabilities of SNNs to achieve high performance and low power consumption. Notable examples of neuromorphic hardware include Intel’s Loihi and IBM’s TrueNorth.
Intel’s Loihi chip is designed to support a wide range of SNN models and offers features such as on-chip learning and real-time processing. The chip includes a large number of programmable neurons and synapses, enabling the development of complex and adaptive neural networks. Loihi’s energy-efficient design makes it suitable for applications in robotics, edge computing, and AI research.
IBM’s TrueNorth chip is another prominent example of neuromorphic hardware, designed to emulate the brain’s architecture and function. TrueNorth features a highly parallel and distributed architecture, with a large number of cores that can independently process spikes and update synapses. The chip’s low power consumption and high performance make it an ideal platform for developing and deploying SNN-based applications.
Example: Simulating SNNs with Brian
from brian2 import *
# Parameters
tau = 10*ms
V_reset = -70*mV
V_thresh = -50*mV
R = 10*Mohm
I = 1*nA
# Neuron model
eqs = '''
dv/dt = (-(v - V_reset) + R*I)/tau : volt
'''
# Create neuron group
G = NeuronGroup(1, eqs, threshold='v>V_thresh', reset='v=V_reset', method='exact')
G.v = V_reset
# Monitor neuron state
M = StateMonitor(G, 'v', record=True)
spikemon = SpikeMonitor(G)
# Run simulation
run(100*ms)
# Plot results
figure(figsize=(10, 4))
subplot(121)
plot(M.t/ms, M.v[0]/mV)
xlabel('Time (ms)')
ylabel('Membrane potential (mV)')
subplot(122)
plot(spikemon.t/ms, spikemon.i, 'ob')
xlabel('Time (ms)')
ylabel('Spike')
show()
In this example, Brian is used to simulate a simple spiking neuron model. The neuron fires spikes based on the input current, and the simulation results are plotted to visualize the membrane potential and spike times. This example demonstrates how to use Brian for simulating and analyzing SNNs.
Unveiling the Mechanisms: How Machine Learning Models Learn from DataFuture Directions for Spiking Neural Networks
Advances in Learning Algorithms
One of the key areas of research in Spiking Neural Networks is the development of advanced learning algorithms. Traditional learning algorithms used in ANNs, such as backpropagation, are not directly applicable to SNNs due to the discrete nature of spikes. Therefore, researchers are exploring new learning methods that can effectively train SNNs while leveraging their temporal dynamics and sparsity.
Spike-Timing-Dependent Plasticity (STDP) is a biologically inspired learning rule that adjusts synaptic weights based on the timing of pre- and post-synaptic spikes. STDP has shown promise in training SNNs, enabling them to learn temporal patterns and adapt to changing inputs. Researchers are also investigating hybrid learning approaches that combine STDP with gradient-based methods to improve learning efficiency and accuracy.
Another area of focus is the development of unsupervised learning algorithms for SNNs. These algorithms aim to enable SNNs to learn from unlabelled data by identifying patterns and structures in the input. Unsupervised learning in SNNs can lead to the development of more autonomous and adaptive systems that can learn and evolve without extensive human intervention.
Integration with Traditional Neural Networks
Integrating Spiking Neural Networks with traditional neural networks is a promising direction for creating hybrid models that leverage the strengths of both approaches. SNNs excel in temporal processing and energy efficiency, while traditional ANNs are well-established for tasks requiring high accuracy and large-scale data processing. Combining these networks can lead to models that are both powerful and efficient.
One approach to integration is to use SNNs for the initial stages of processing, where temporal dynamics and sparsity are critical, and then pass the processed information to traditional ANNs for further analysis and decision-making. This hybrid approach can enhance the overall performance and efficiency of the model, making it suitable for a wider range of applications.
Researchers are also exploring ways to convert trained ANNs into SNNs, enabling the use of well-established ANN training methods while benefiting from the efficiency of SNNs. Techniques such as weight normalization and threshold adjustment are used to ensure that the converted SNNs retain the performance of the original ANNs. This integration can pave the way for more widespread adoption of SNNs in practical applications.
Example: Hybrid Model Combining SNN and ANN
import numpy as np
import torch
import torch.nn as nn
# Define a simple ANN model
class ANN(nn.Module):
def __init__(self):
super(ANN, self).__init__()
self.fc1 = nn.Linear(10, 50)
self.fc2 = nn.Linear(50, 10)
self.fc3 = nn.Linear(10, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = torch.sigmoid(self.fc3(x))
return x
# Define a simple SNN model (using a custom spiking layer for demonstration)
class SNN(nn.Module):
def __init__(self):
super(SNN, self).__init__()
self.spike_layer = nn.Linear(10, 50) # Placeholder for actual spiking neuron layer
self.fc = nn.Linear(50, 10)
def forward(self, x):
x = torch.relu(self.spike_layer(x)) # Placeholder for spiking neuron dynamics
x = torch.relu(self.fc(x))
return x
# Combine SNN and ANN in a hybrid model
class HybridModel(nn.Module):
def __init__(self):
super(HybridModel, self).__init__()
self.snn = SNN()
self.ann = ANN()
def forward(self, x):
x = self.snn(x)
x = self.ann(x)
return x
# Example usage
model = HybridModel()
input_data = torch.randn(1, 10)
output = model(input_data)
print(output)
In this example, a hybrid model combining SNN and ANN components is implemented using PyTorch. The SNN processes the initial input, and the ANN performs further analysis and decision-making. This approach demonstrates how to leverage the strengths of both SNNs and ANNs in a single model.
Expanding Applications of SNNs
As research and development in Spiking Neural Networks continue to advance, the range of applications for SNNs is expected to expand. In addition to existing applications in robotics, neuroscience, and low-power computing, SNNs are poised to play a significant role in emerging fields such as neuromorphic engineering, real-time data analytics, and adaptive control systems.
In neuromorphic engineering, SNNs are used to develop hardware systems that mimic the structure and function of the brain. These systems can achieve unprecedented levels of efficiency and performance, making them suitable for a wide range of applications, from autonomous systems to large-scale data processing. The development of new materials and fabrication techniques will further enhance the capabilities of neuromorphic hardware.
Real-time data analytics is another promising area for SNNs. The ability to process and analyze continuous streams of data in real time can provide valuable insights and enable timely decision-making. SNNs can be used in financial markets, environmental monitoring, and industrial automation, where real-time data processing is critical.
Adaptive control systems, such as those used in autonomous vehicles and smart grids, can benefit from the robustness and fault tolerance of SNNs. These systems need to operate reliably in dynamic and uncertain environments, and SNNs can provide the adaptability and resilience required for such tasks. As the technology matures, SNNs are expected to become a key component of advanced control systems.
Spiking Neural Networks offer significant advantages for machine learning, including energy efficiency, real-time processing capabilities, and robustness. By leveraging the unique characteristics of SNNs, researchers and developers can create powerful and efficient computational systems that mimic the functioning of biological brains. The future of SNNs holds great promise, with advances in learning algorithms, integration with traditional neural networks, and expanding applications driving the development of this exciting field.
If you want to read more articles similar to The Advantages of Spiking Neural Networks for Machine Learning, you can visit the Artificial Intelligence category.
You Must Read