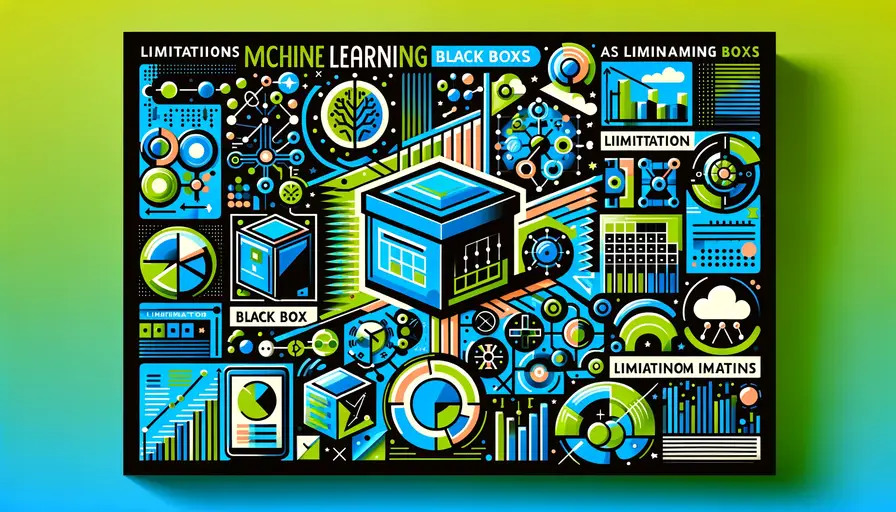
The Impact of Machine Learning on Privacy and Data Security

- Implement Strong Encryption Algorithms to Protect Sensitive Data
- Develop Robust Authentication Systems to Ensure Only Authorized Access to Data
- Train Machine Learning Models with Privacy in Mind
- Regularly Update and Patch Software to Fix Vulnerabilities
- Use Anonymization Techniques to Minimize the Risk of Personally Identifiable Information Being Exposed
- Educate Users and Employees About Best Practices for Protecting Privacy and Data Security
- Collaborate with Regulatory Bodies to Establish and Enforce Data Protection Policies
- Implement Data Minimization Strategies to Collect and Store Only Necessary Information
- Conduct Privacy Impact Assessments to Identify and Mitigate Any Potential Risks to Privacy and Data Security
Implement Strong Encryption Algorithms to Protect Sensitive Data
Implementing strong encryption algorithms is crucial for protecting sensitive data in machine learning applications. Encryption ensures that data is converted into a format that is unreadable to unauthorized users, making it significantly harder for malicious actors to access or tamper with the information. This is particularly important when dealing with personally identifiable information (PII) or financial data, where breaches can lead to severe consequences.
One common approach to encryption is using algorithms such as Advanced Encryption Standard (AES) or RSA encryption. AES is widely adopted due to its efficiency and robustness, offering different key lengths (128, 192, and 256 bits) for varying levels of security. RSA encryption, on the other hand, is often used for secure data transmission over the internet, leveraging public and private keys to encrypt and decrypt data.
For example, encrypting data before training a machine learning model can be done as follows:
# Example: Encrypting Data Using AES
from Crypto.Cipher import AES
import base64
# Function to pad data to be multiples of AES block size
def pad(data):
return data + (AES.block_size - len(data) % AES.block_size) * chr(AES.block_size - len(data) % AES.block_size)
# Encrypt data
def encrypt(plain_text, key):
cipher = AES.new(key.encode('utf-8'), AES.MODE_ECB)
encrypted = cipher.encrypt(pad(plain_text).encode('utf-8'))
return base64.b64encode(encrypted).decode('utf-8')
# Sample usage
key = 'thisisaverysecurekey123' # Must be 16, 24, or 32 bytes long
plain_text = 'Sensitive Data'
encrypted_text = encrypt(plain_text, key)
print("Encrypted Text:", encrypted_text)
Ensuring that both data at rest and data in transit are encrypted is essential for comprehensive data security. This means that not only should the stored data be encrypted, but also the data being transferred between systems should be protected using secure protocols such as HTTPS or TLS.

Robust authentication systems are vital for ensuring that only authorized individuals can access sensitive data within machine learning applications. Implementing multi-factor authentication (MFA) adds an additional layer of security by requiring users to provide multiple forms of verification before accessing data. This can include something the user knows (a password), something the user has (a mobile device), and something the user is (biometric verification).
An effective authentication system not only protects data but also ensures compliance with various data protection regulations. By requiring strong, unique passwords and periodically prompting users to change them, the risk of unauthorized access is significantly reduced. Additionally, using tools like OAuth or SAML can help manage access across different platforms securely.
For example, implementing MFA in a web application might involve integrating with a service like Google Authenticator:
# Example: Implementing Multi-Factor Authentication (MFA)
import pyotp
import qrcode
# Generate a base32 secret for the user
secret = pyotp.random_base32()
totp = pyotp.TOTP(secret)
# Display the QR code to the user
uri = totp.provisioning_uri("user@example.com", issuer_name="Example App")
qrcode.make(uri).show()
# Verify the OTP entered by the user
otp = input("Enter the OTP: ")
if totp.verify(otp):
print("OTP is valid!")
else:
print("Invalid OTP!")
Regularly updating and patching machine learning systems is another critical aspect of maintaining robust security. By keeping systems up to date, organizations can protect against known vulnerabilities and ensure that the latest security features are in place. This proactive approach helps mitigate the risk of data breaches and other security incidents.

Train Machine Learning Models with Privacy in Mind
Training machine learning models with privacy in mind involves implementing techniques and strategies that minimize the exposure of sensitive data. One approach is to use differential privacy, which adds noise to the data in a way that preserves privacy while still allowing the model to learn useful patterns. This technique helps prevent the model from memorizing specific data points, making it harder to reverse-engineer the data from the model's predictions.
Another important technique is federated learning, where the model is trained across multiple decentralized devices or servers holding local data samples, without exchanging the data itself. Instead, the model updates are aggregated centrally, ensuring that the raw data remains on the local devices. This approach significantly reduces the risk of data breaches and enhances privacy.
For example, implementing differential privacy in a machine learning model might look like this:
# Example: Implementing Differential Privacy
import numpy as np
# Function to add differential privacy noise to data
def add_differential_privacy(data, epsilon=1.0):
noise = np.random.laplace(loc=0, scale=1/epsilon, size=data.shape)
return data + noise
# Sample usage
data = np.array([10, 20, 30, 40, 50])
epsilon = 0.5
private_data = add_differential_privacy(data, epsilon)
print("Private Data:", private_data)
Training models with privacy in mind not only protects sensitive data but also builds trust with users and stakeholders. By demonstrating a commitment to data privacy, organizations can foster a positive reputation and comply with regulatory requirements.

Regularly Update and Patch Software to Fix Vulnerabilities
Regularly updating and patching software is essential for maintaining the security of machine learning systems. Software updates often include fixes for known vulnerabilities and security enhancements that protect against new threats. Failing to keep systems updated can leave them exposed to exploits and attacks that could compromise sensitive data.
Implementing an automated update process can help ensure that systems remain up to date without requiring manual intervention. Tools like Ansible or Chef can automate the deployment of updates across multiple servers, reducing the risk of human error and ensuring consistency. Additionally, regularly reviewing and testing updates in a controlled environment before deploying them to production can help identify and resolve potential issues.
For example, an Ansible playbook for updating a server might look like this:
# Example: Ansible Playbook for Updating Server
- name: Update and upgrade server packages
hosts: all
become: yes
tasks:
- name: Update apt cache
apt:
update_cache: yes
- name: Upgrade all packages
apt:
upgrade: dist
Regular patching is not just about applying updates; it also involves monitoring for new vulnerabilities and responding promptly. Organizations should subscribe to security bulletins and alerts from software vendors and security organizations to stay informed about the latest threats and recommended actions.

Use Anonymization Techniques to Minimize the Risk of Personally Identifiable Information Being Exposed
Anonymization techniques play a critical role in protecting personally identifiable information (PII) in machine learning. By transforming data in a way that removes or obscures identifying information, organizations can reduce the risk of data breaches and ensure compliance with data protection regulations.
One common approach is data masking, which involves replacing sensitive information with fictitious data that retains the same format. Another technique is k-anonymity, where data is generalized or suppressed to ensure that individuals cannot be distinguished from at least k other individuals. Differential privacy can also be used to add noise to the data, making it difficult to infer specific information about individuals.
For example, implementing k-anonymity in a dataset might involve the following steps:
# Example: Implementing k-Anonymity
import pandas as pd
from anonymize import Anonymizer
# Sample dataset
data = {
'age': [23, 45, 34, 35, 23],
'gender': ['M', 'F', 'M', 'F', 'M'],
'zipcode': ['12345', '12345', '54321', '54321', '54321']
}
df = pd.DataFrame(data)
# Define anonymizer with k-anonymity parameter
anonymizer = Anonymizer(k=2)
anonymized_df = anonymizer.anonymize(df)
print(anonymized_df)
Anonymization techniques not only protect individual privacy but also enable the sharing and analysis of data without compromising security. By adopting these methods, organizations can leverage data for insights and innovation while maintaining compliance with privacy regulations.

Educate Users and Employees About Best Practices for Protecting Privacy and Data Security
Educating users and employees about best practices for protecting privacy and data security is crucial for creating a security-conscious culture. Regular training sessions and awareness programs can help individuals understand the importance of data security and their role in maintaining it. Topics covered should include strong password management, recognizing phishing attempts, and the proper handling of sensitive information.
Training should be tailored to different roles within the organization, ensuring that everyone from entry-level employees to senior management understands their responsibilities. Interactive sessions, such as workshops and simulations, can make the training more engaging and effective. Additionally, providing ongoing education and updates helps keep everyone informed about the latest threats and best practices.
For example, a phishing awareness simulation might involve sending mock phishing emails to employees to test their ability to recognize and report suspicious messages:
# Example: Phishing Awareness Simulation
import random
# Sample phishing email content
phishing_emails = [
"Your account has been compromised. Click here to reset your password.",
"You have won a prize! Click here to claim it.",
"Urgent: Verify your account information now."
]
# Function to send mock phishing email
def send_phishing_email(email):
print(f"Sending phishing email to {email}")
print(random.choice(ph
ishing_emails))
# Sample usage
employees = ["employee1@example.com", "employee2@example.com", "employee3@example.com"]
for employee in employees:
send_phishing_email(employee)
By educating users and employees, organizations can significantly reduce the risk of human error leading to security incidents. Informed individuals are more likely to follow best practices and recognize potential threats, contributing to a more secure environment.

Collaborate with Regulatory Bodies to Establish and Enforce Data Protection Policies
Collaboration with regulatory bodies is essential for establishing and enforcing data protection policies that comply with legal requirements. Regulatory bodies, such as the General Data Protection Regulation (GDPR) in Europe or the California Consumer Privacy Act (CCPA) in the United States, provide guidelines and standards for protecting personal data. Working closely with these organizations ensures that data protection measures meet or exceed regulatory expectations.
Establishing clear data protection policies involves defining how data is collected, stored, processed, and shared. These policies should be documented and communicated to all employees, ensuring that everyone understands their role in maintaining data security. Regular audits and assessments can help identify any gaps in compliance and provide opportunities for improvement.
For example, a data protection policy might include provisions for data encryption, access controls, and incident response:
# Example: Data Protection Policy
data_protection_policy = {
"data_collection": "Collect only necessary data and obtain consent from individuals.",
"data_storage": "Encrypt all stored data and implement access controls.",
"data_processing": "Ensure data is processed in accordance with privacy regulations.",
"data_sharing": "Share data only with authorized parties and use anonymization techniques.",
"incident_response": "Establish a plan for responding to data breaches and other security incidents."
}
print(data_protection_policy)
Collaboration with regulatory bodies also involves staying updated on changes in legislation and adapting policies accordingly. This proactive approach helps organizations avoid legal issues and maintain a reputation for responsible data management.
Implement Data Minimization Strategies to Collect and Store Only Necessary Information
Data minimization is a principle that involves collecting and storing only the information necessary for a specific purpose. This reduces the risk of data breaches and ensures compliance with privacy regulations. By limiting the amount of data collected, organizations can minimize the potential impact of a breach and reduce the burden of managing and securing large datasets.
Implementing data minimization strategies involves assessing the data needs of each process and eliminating any unnecessary data collection. This can be achieved through techniques such as data anonymization, aggregation, and deletion of redundant data. Regularly reviewing data collection practices helps ensure that only relevant information is retained.
For example, an e-commerce platform might implement data minimization by only collecting the information needed to process orders:
# Example: Data Minimization in E-commerce
order_data = {
"customer_id": 123,
"name": "John Doe",
"address": "123 Main St, Anytown, USA",
"email": "john.doe@example.com",
"phone": "555-1234"
}
# Collect only necessary information
minimized_order_data = {
"customer_id": order_data["customer_id"],
"address": order_data["address"]
}
print(minimized_order_data)
Data minimization not only enhances privacy and security but also improves data quality and efficiency. By focusing on the most relevant information, organizations can streamline their processes and reduce the complexity of data management.
Conduct Privacy Impact Assessments to Identify and Mitigate Any Potential Risks to Privacy and Data Security
Conducting privacy impact assessments (PIAs) is a proactive approach to identifying and mitigating potential risks to privacy and data security. PIAs involve evaluating how personal data is collected, processed, and stored, and assessing the potential impact on individuals' privacy. This process helps organizations identify vulnerabilities and implement measures to protect sensitive information.
A PIA typically includes the following steps: identifying the data involved, assessing the risks, and implementing mitigation strategies. This involves working closely with stakeholders to understand the data flows and potential threats. Regularly conducting PIAs ensures that data protection measures remain effective as new technologies and processes are introduced.
For example, a PIA for a new customer relationship management (CRM) system might involve the following steps:
# Example: Privacy Impact Assessment (PIA) for CRM System
pia_steps = {
"identify_data": "Identify the types of personal data collected and processed by the CRM system.",
"assess_risks": "Evaluate the potential risks to privacy and data security, including unauthorized access and data breaches.",
"mitigation_strategies": "Implement measures to mitigate identified risks, such as encryption and access controls."
}
print(pia_steps)
Conducting PIAs not only helps organizations comply with legal requirements but also builds trust with customers and stakeholders. By demonstrating a commitment to privacy and data security, organizations can enhance their reputation and ensure the responsible handling of personal information.
If you want to read more articles similar to The Impact of Machine Learning on Privacy and Data Security, you can visit the Data Privacy category.
You Must Read