
The Role of Weights in Machine Learning: Purpose and Application
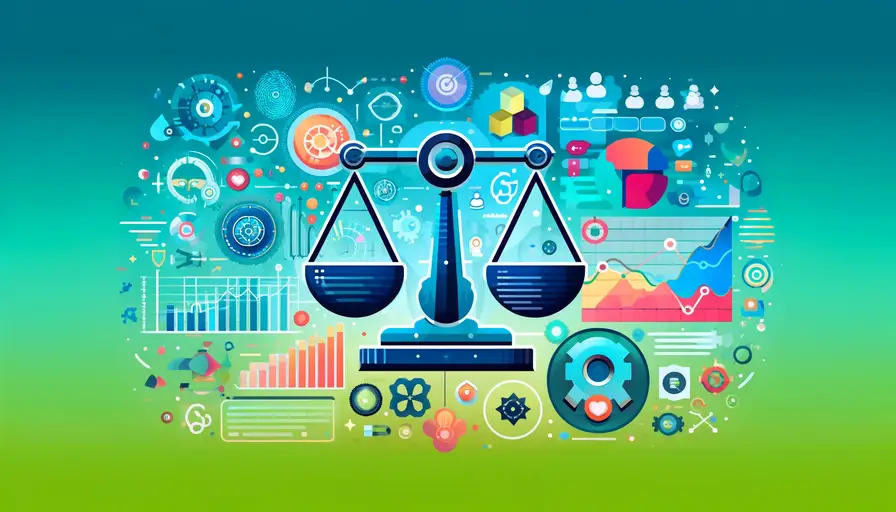
Weights are fundamental components in machine learning models, playing a critical role in how these models learn and make predictions. This article delves into the significance of weights in machine learning, exploring their purpose, application, and impact on model performance. We will examine key concepts, practical examples, and important tools and resources to provide a comprehensive guide on the subject.
Importance of Weights in Machine Learning
Understanding Weights in Neural Networks
Understanding weights in neural networks is essential for comprehending how these models function. In the context of a neural network, weights are parameters that are adjusted during the training process to minimize the error in predictions. Each connection between neurons has an associated weight that determines the influence of one neuron on another.
In a neural network, the input data is multiplied by the weights, and then a bias term is added before applying an activation function. This process is repeated for each layer of the network, transforming the input data as it propagates through the network. The weights are updated using optimization algorithms like gradient descent, which aim to reduce the loss function, a measure of the difference between the predicted and actual outputs.
The significance of weights is that they allow the network to learn complex patterns in the data. By adjusting the weights, the network can capture the underlying relationships between the input features and the target variable, leading to accurate predictions. The process of weight adjustment is what enables the model to learn from data.
Popular R Packages for Machine Learning Variable SelectionWeights in Linear Models
Weights in linear models such as linear regression and logistic regression are simpler but equally important. In linear regression, the model predicts the output as a weighted sum of the input features. The weights in this context represent the coefficients of the linear equation, indicating the strength and direction of the relationship between each input feature and the output.
In logistic regression, weights are used to predict the probability of a binary outcome. The model applies a logistic function to the weighted sum of the inputs, transforming it into a probability. The weights are learned during training and determine the contribution of each input feature to the likelihood of the outcome.
Here’s an example of implementing linear regression with weights using scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
# Sample data
data = {'feature1': [1, 2, 3, 4, 5],
'feature2': [5, 6, 7, 8, 9],
'target': [1, 2, 3, 4, 5]}
df = pd.DataFrame(data)
# Features and target variable
X = df[['feature1', 'feature2']]
y = df['target']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a linear regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Get the weights (coefficients)
weights = model.coef_
print(f'Weights: {weights}')
Impact of Weights on Model Performance
The impact of weights on model performance is profound. Weights determine how the input features influence the model's predictions. Properly tuned weights can lead to accurate and reliable predictions, while poorly tuned weights can result in overfitting or underfitting.
Comparing Machine Learning Models in R: A Guide to Choose the BestOverfitting occurs when the model's weights are too closely aligned with the training data, capturing noise rather than the underlying pattern. This results in a model that performs well on the training data but poorly on new, unseen data. Regularization techniques like L1 and L2 regularization can be applied to penalize large weights, reducing the risk of overfitting.
Underfitting happens when the model's weights do not adequately capture the complexity of the data, leading to poor performance on both training and test data. This can be addressed by using more complex models or adding more features to the input data.
Optimization algorithms play a crucial role in adjusting the weights to improve model performance. Techniques like gradient descent iteratively update the weights to minimize the loss function, guiding the model towards better predictions.
Training Models with Weights
Gradient Descent and Weight Updates
Gradient descent and weight updates are central to training machine learning models. Gradient descent is an optimization algorithm used to minimize the loss function by iteratively updating the weights. The algorithm calculates the gradient of the loss function with respect to each weight and adjusts the weights in the opposite direction of the gradient.
Best Machine Learning Algorithms for Multi-Label ClassificationThe learning rate, a hyperparameter, controls the size of the weight updates. A small learning rate results in slow convergence, while a large learning rate can cause the model to overshoot the optimal solution. Choosing an appropriate learning rate is crucial for efficient training.
There are several variants of gradient descent, including batch gradient descent, stochastic gradient descent (SGD), and mini-batch gradient descent. Batch gradient descent uses the entire training dataset to compute the gradient, while SGD uses a single data point, and mini-batch gradient descent uses a subset of the data. Each variant has its advantages and trade-offs in terms of convergence speed and stability.
Here’s an example of implementing gradient descent for a simple linear regression model:
import numpy as np
# Sample data
X = np.array([1, 2, 3, 4, 5])
y = np.array([1, 2, 3, 4, 5])
# Initialize weights and hyperparameters
weights = np.random.rand(2)
learning_rate = 0.01
epochs = 1000
# Add bias term to the input data
X_b = np.c_[np.ones((X.shape[0], 1)), X]
# Gradient descent
for epoch in range(epochs):
gradients = 2 / X_b.shape[0] * X_b.T.dot(X_b.dot(weights) - y)
weights -= learning_rate * gradients
print(f'Final weights: {weights}')
Regularization Techniques
Regularization techniques are employed to prevent overfitting by adding a penalty to the loss function, discouraging overly complex models with large weights. The two most common regularization techniques are L1 regularization (Lasso) and L2 regularization (Ridge).
Building a Decision Tree Classifier in scikit-learnL1 regularization adds the absolute value of the weights to the loss function, promoting sparsity by driving some weights to zero. This can be useful for feature selection, as it effectively removes irrelevant features from the model.
L2 regularization adds the square of the weights to the loss function, preventing any single weight from becoming too large. This results in a smoother and more generalizable model. Elastic Net combines L1 and L2 regularization, providing a balance between the two.
Here’s an example of implementing L2 regularization using scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import Ridge
# Sample data
data = {'feature1': [1, 2, 3, 4, 5],
'feature2': [5, 6, 7, 8, 9],
'target': [1, 2, 3, 4, 5]}
df = pd.DataFrame(data)
# Features and target variable
X = df[['feature1', 'feature2']]
y = df['target']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Ridge regression model
model = Ridge(alpha=1.0)
model.fit(X_train, y_train)
# Get the weights (coefficients)
weights = model.coef_
print(f'Weights with L2 regularization: {weights}')
Optimizing Weight Initialization
Optimizing weight initialization is crucial for training deep neural networks. Poor weight initialization can lead to slow convergence, vanishing or exploding gradients, and suboptimal solutions. Proper initialization methods help ensure that the model trains efficiently and effectively.
Comparison of Decision Tree and Random Forest for ClassificationCommon initialization techniques include random initialization, Xavier initialization, and He initialization. Random initialization assigns small random values to the weights, ensuring that the network starts with different weights and learns diverse features. However, this method can sometimes lead to vanishing or exploding gradients.
Xavier initialization, also known as Glorot initialization, sets the weights based on the number of input and output neurons. It aims to keep the variance of the activations and gradients consistent across layers, improving convergence. He initialization is similar but uses a different scaling factor, making it suitable for networks with ReLU activation functions.
Here’s an example of implementing He initialization using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Build a simple neural network with He initialization
model = Sequential([
Dense(10, activation='relu', kernel_initializer='he_normal', input_shape=(5,)),
Dense(1, activation='sigmoid')
])
# Compile the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Print the model summary
model.summary()
Practical Applications of Weights in ML Models
Weights in Convolutional Neural Networks (CNNs)
Weights in Convolutional Neural Networks (CNNs) are pivotal for tasks such as image recognition and processing. CNNs use convolutional layers, where weights are applied to small regions of the input image, known as receptive fields. These weights, or filters, detect local patterns such as edges, textures, and shapes.
Choosing the Right Machine Learning Model: A Comprehensive GuideAs the input image passes through multiple convolutional layers, the filters learn to recognize more complex patterns and features. The weights are updated during training using backpropagation, enabling the network to improve its feature detection capabilities.
Pooling layers, which follow the convolutional layers, reduce the spatial dimensions of the data, preserving the most important features while reducing the computational load. Fully connected layers at the end of the network use weights to combine the features extracted by the convolutional layers, producing the final classification or regression output.
Here’s an example of implementing a simple CNN using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# Build a simple CNN
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D(pool_size=(2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Print the model summary
model.summary()
Weights in Recurrent Neural Networks (RNNs)
Weights in Recurrent Neural Networks (RNNs) are essential for tasks involving sequential data, such as time series forecasting, natural language processing, and speech recognition. RNNs use weights to connect the neurons within a layer to the neurons in the next time step, allowing the network to retain information across sequences.
In RNNs, the weights are shared across time steps, enabling the network to process sequences of variable length. The hidden state, which is influenced by the previous inputs and weights, captures the temporal dependencies in the data. This makes RNNs suitable for tasks where the order of the data is important.
However, traditional RNNs can suffer from vanishing or exploding gradients, making it difficult to learn long-term dependencies. Long Short-Term Memory (LSTM) and Gated Recurrent Unit (GRU) networks address this issue by introducing gating mechanisms that regulate the flow of information, allowing the network to capture long-term dependencies more effectively.
Here’s an example of implementing an LSTM using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
# Build a simple LSTM model
model = Sequential([
LSTM(50, activation='relu', input_shape=(100, 1)),
Dense(1)
])
# Compile the model
model.compile(optimizer='adam', loss='mean_squared_error')
# Print the model summary
model.summary()
Weights in Transfer Learning
Weights in transfer learning involve leveraging pre-trained models on new tasks. Transfer learning allows models trained on large datasets to be fine-tuned on smaller, task-specific datasets, reducing the amount of training data and time required to achieve high performance.
In transfer learning, the weights of a pre-trained model are used as the starting point for a new model. The lower layers, which capture generic features, are often frozen, while the higher layers, which capture task-specific features, are fine-tuned on the new data. This approach leverages the knowledge learned from the pre-trained model, improving the performance and efficiency of the new model.
Popular pre-trained models for transfer learning include VGG, ResNet, and Inception for image classification, and BERT and GPT for natural language processing. These models are available through libraries like TensorFlow and PyTorch, making it easy to implement transfer learning in various applications.
Here’s an example of implementing transfer learning using TensorFlow and a pre-trained ResNet model:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Flatten
from tensorflow.keras.applications import ResNet50
# Load the pre-trained ResNet50 model
base_model = ResNet50(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# Freeze the base model
base_model.trainable = False
# Build a new model on top of the base model
model = Sequential([
base_model,
Flatten(),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Print the model summary
model.summary()
Weights play a fundamental role in the functionality and performance of machine learning models. From simple linear models to complex neural networks, weights are the parameters that allow models to learn from data and make predictions. By understanding the importance of weights, how they are optimized, and their application in various ML models, practitioners can develop more effective and efficient models. Utilizing tools like Google, Kaggle, and popular libraries such as TensorFlow and scikit-learn, professionals can enhance their machine learning workflows and achieve better results.
If you want to read more articles similar to The Role of Weights in Machine Learning: Purpose and Application, you can visit the Algorithms category.
You Must Read