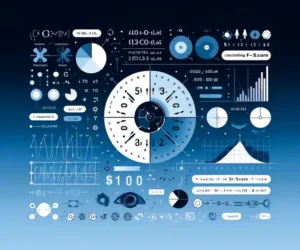
Time Series Forecasting With R
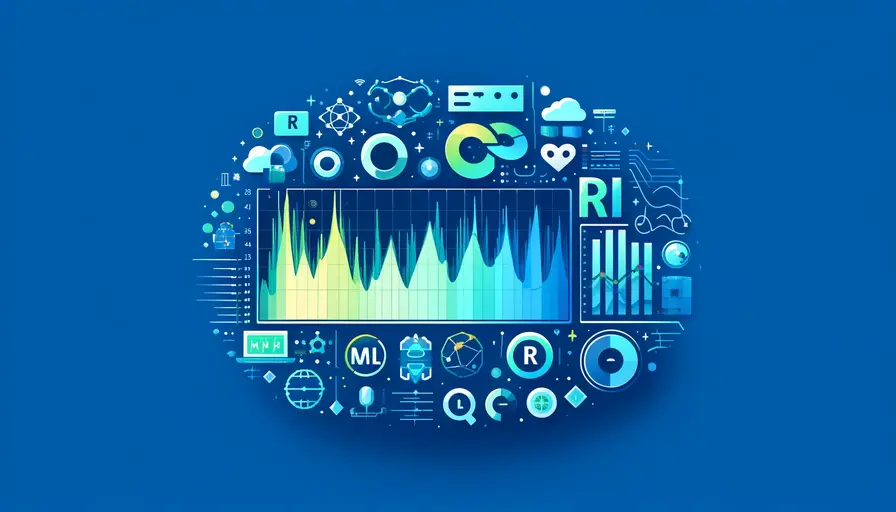
Time series forecasting is an essential technique in many fields such as finance, economics, environmental science, and supply chain management. Using R for time series forecasting leverages its powerful packages and extensive statistical capabilities. This guide outlines the process of building and optimizing machine learning models for time series forecasting in R.
- Machine Learning Model for Time Series Forecasting
- Example Using AirPassengers Dataset
- Why Use R for Time Series Forecasting?
- Preprocess and Clean the Time Series Data
- Use Techniques Like Feature Engineering
- Evaluate the Performance of the Machine Learning Model
- Fine-tune the ML Model to Improve Its Forecasting Accuracy
- Ensemble Methods
- Deep Learning
Machine Learning Model for Time Series Forecasting
Building a machine learning model for time series forecasting involves several key steps, from data preprocessing to model evaluation and prediction.
Load and Preprocess the Data
Load and preprocess the data by importing it into R and performing necessary cleaning operations. Data loading can be done using functions like read.csv()
or read.table()
, while preprocessing might involve handling missing values, outliers, and ensuring data is in a suitable format for analysis.
Split the Data Into Training and Testing Sets
Split the data into training and testing sets to evaluate the model's performance. This is typically done using functions like sample.split()
from the caTools
package, ensuring that the model is trained on a subset of the data and tested on unseen data to assess its predictive capabilities.
Explore the Data
Explore the data to understand its characteristics and underlying patterns. This step involves visualizing the time series, identifying trends and seasonality, and performing summary statistics. Tools like ggplot2
and tseries
packages in R can help with data exploration.
Select an Appropriate Machine Learning Algorithm
Select an appropriate machine learning algorithm based on the data characteristics and forecasting requirements. Common algorithms for time series forecasting include ARIMA, Prophet, and various machine learning models such as Random Forest, Gradient Boosting Machines (GBM), and Neural Networks.
Train the Machine Learning Model
Train the machine learning model using the training dataset. This involves fitting the model to the data and adjusting its parameters to minimize the forecasting error. Functions like train()
from the caret
package can be used for this purpose.
Evaluate the Model
Evaluate the model to ensure it performs well on the testing dataset. This step involves calculating performance metrics such as Mean Squared Error (MSE) and Mean Absolute Error (MAE). Good performance on the testing set indicates that the model can generalize well to unseen data.
Strategies for Zero-Inflated Data in Machine Learning AlgorithmsMake Predictions
Make predictions using the trained model on new or unseen data. This step involves generating forecasts and assessing their accuracy compared to actual outcomes. Functions like predict()
can be used to make future predictions based on the trained model.
Fine-tune and Optimize the Model
Fine-tune and optimize the model by adjusting hyperparameters and exploring different feature engineering techniques. This iterative process aims to improve the model's accuracy and robustness.
Example Using AirPassengers Dataset
Here's an example of time series forecasting in R using the forecast
package and the ARIMA model. We'll use a sample dataset from the forecast
package itself.
Install and Load Required Packages
First, you'll need to install and load the necessary packages.
Exploring Gradient Descent in Linear Regression# Install packages if not already installed
install.packages("forecast")
install.packages("ggplot2")
# Load libraries
library(forecast)
library(ggplot2)
Load and Visualize the Data
We'll use the AirPassengers
dataset, which contains monthly totals of international airline passengers from 1949 to 1960.
# Load the AirPassengers dataset
data("AirPassengers")
ts_data <- AirPassengers
# Plot the time series data
autoplot(ts_data) +
ggtitle("Monthly Air Passengers") +
xlab("Year") +
ylab("Number of Passengers")
Decompose the Time Series
Decompose the time series to understand its components: trend, seasonality, and residuals.
# Decompose the time series
decomposed <- decompose(ts_data)
# Plot decomposed components
autoplot(decomposed) +
ggtitle("Decomposition of Air Passengers Time Series")
Fit an ARIMA Model
Fit an ARIMA model to the time series data.
# Fit an ARIMA model
fit <- auto.arima(ts_data)
# Display model summary
summary(fit)
# Plot the residuals of the fitted model
checkresiduals(fit)
Forecast the Future
Use the fitted ARIMA model to forecast future values.
Feature Selection Methods in scikit-learn: A Comprehensive Overview# Forecast the next 24 months
forecasted <- forecast(fit, h = 24)
# Plot the forecast
autoplot(forecasted) +
ggtitle("Forecasted Monthly Air Passengers") +
xlab("Year") +
ylab("Number of Passengers")
Evaluate the Model
Evaluate the accuracy of the forecast using appropriate metrics.
# Calculate accuracy of the forecast
accuracy(forecasted)
You can enhance this code by trying different time series models, adjusting the model parameters, and exploring other forecasting techniques such as ETS, TBATS, or neural network models.
Why Use R for Time Series Forecasting?
Using R for time series forecasting provides access to a wide range of specialized packages and tools designed for statistical analysis and model building.
The Forecast Package
The Forecast package is a powerful tool in R for time series analysis and forecasting. It provides functions for modeling and forecasting using methods like ARIMA, Exponential Smoothing, and more. The forecast
package simplifies the process of fitting models, evaluating their performance, and generating forecasts.
The Prophet Package
The Prophet package is developed by Facebook for handling complex time series data with strong seasonal effects and missing data. It is particularly useful for business and economic forecasting. Prophet provides an intuitive interface and robust handling of various time series components, making it a popular choice for practitioners.
Getting Started With Time Series Forecasting in R
Getting started with time series forecasting in R involves installing the necessary packages and familiarizing yourself with their functionalities. The forecast
and prophet
packages are essential tools that offer comprehensive support for various forecasting techniques.
Preprocess and Clean the Time Series Data
Preprocessing and cleaning the time series data is crucial for ensuring the accuracy and reliability of the forecasting model.
Removing Outliers and Missing Values
Removing outliers and missing values helps in maintaining the integrity of the dataset. Techniques like interpolation, forward filling, or using specific functions like na.interp()
from the forecast
package can be employed to handle these issues.
Handling Seasonality and Trend
Handling seasonality and trend involves decomposing the time series into its components and adjusting the data accordingly. Functions like stl()
in R can be used to separate the seasonal and trend components from the time series data.
Feature Engineering
Feature engineering involves creating new features from the existing data to improve model performance. This could include generating lagged variables, rolling statistics, and other derived metrics that capture the underlying patterns in the data.
Normalization and Scaling
Normalization and scaling ensure that the data is within a consistent range, which can help improve the performance of machine learning models. Functions like scale()
can be used to standardize the data.
Train-test Splitting
Train-test splitting is essential for evaluating the model's performance. The data should be split into a training set to fit the model and a testing set to validate it. This split helps in assessing how well the model generalizes to new data.
Use Techniques Like Feature Engineering
Using techniques like feature engineering can significantly enhance the performance of time series forecasting models by capturing additional patterns and relationships in the data.
Lagged Variables
Lagged variables are previous values in the time series used as predictors for future values. Creating lagged variables helps in capturing temporal dependencies within the data.
Rolling Statistics
Rolling statistics such as moving averages and rolling standard deviations smooth out short-term fluctuations and highlight longer-term trends. These statistics can be used as features in the forecasting model.
Seasonal Decomposition
Seasonal decomposition involves breaking down the time series into seasonal, trend, and residual components. This decomposition helps in understanding the underlying structure of the data and improving model accuracy.
Fourier Transformations
Fourier transformations can capture cyclical patterns in the time series data. Applying Fourier transformations helps in identifying and modeling periodic components in the data.
Evaluate the Performance of the Machine Learning Model
Evaluating the performance of the machine learning model involves using appropriate metrics to assess its accuracy and reliability.
Mean Squared Error (MSE)
Mean Squared Error (MSE) measures the average squared difference between the predicted and actual values. It penalizes larger errors more than smaller ones, providing a comprehensive measure of model performance.
Mean Absolute Error (MAE)
Mean Absolute Error (MAE) calculates the average absolute difference between the predicted and actual values. It provides an intuitive measure of model accuracy that is less sensitive to outliers than MSE.
Fine-tune the ML Model to Improve Its Forecasting Accuracy
Fine-tuning the ML model involves optimizing hyperparameters, enhancing features, and validating the model through cross-validation techniques to achieve better forecasting accuracy.
Hyperparameter Tuning
Hyperparameter tuning adjusts the model's parameters to find the optimal settings that minimize the error. Techniques like grid search and random search can be used for this purpose.
Feature Engineering
Feature engineering can be revisited to create additional features or modify existing ones based on insights gained from initial model training and evaluation.
Cross-validation
Cross-validation involves dividing the data into multiple subsets and training the model on different combinations of these subsets to ensure it performs well across various data segments. This technique helps in assessing the model's generalizability.
Ensemble Methods
Ensemble methods combine predictions from multiple models to improve forecasting accuracy and robustness. Techniques like bagging, boosting, and stacking leverage the strengths of different models to produce more reliable forecasts.
Deep Learning
Deep learning models such as Long Short-Term Memory (LSTM) networks and Convolutional Neural Networks (CNNs) are powerful tools for time series forecasting. These models can capture complex patterns and dependencies in the data, providing highly accurate forecasts.
Time series forecasting with R involves a systematic approach from data preprocessing to model evaluation and optimization. By leveraging the powerful packages and tools available in R, practitioners can build accurate and reliable forecasting models tailored to their specific needs.
If you want to read more articles similar to Time Series Forecasting With R, you can visit the Algorithms category.
You Must Read