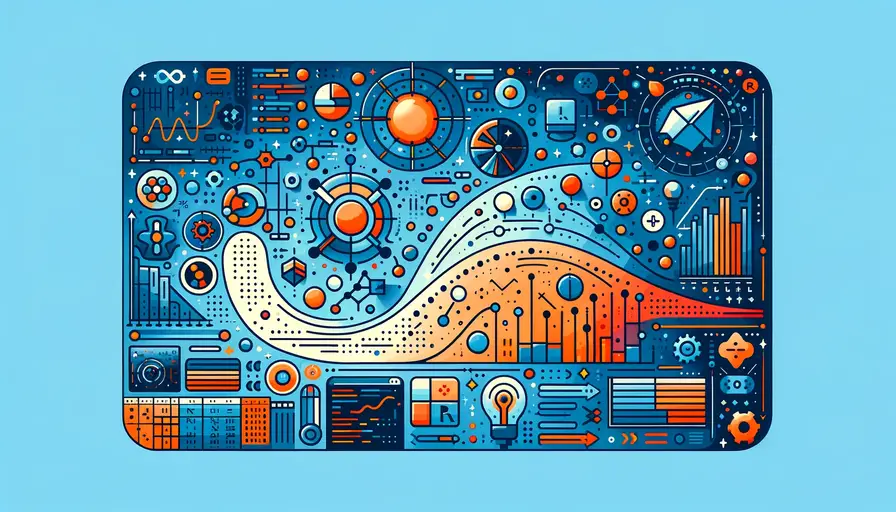
Top Techniques for Machine Learning-based Size Recognition

Machine learning has revolutionized various domains, and size recognition is no exception. Size recognition involves identifying and categorizing objects based on their dimensions, which is essential in fields like manufacturing, retail, and healthcare. This article explores top techniques for machine learning-based size recognition, providing insights into different models, applications, and practical examples. By the end, you'll have a comprehensive understanding of how to implement these techniques effectively.
Understanding Size Recognition in Machine Learning
Importance of Size Recognition
Size recognition plays a crucial role in numerous industries. In manufacturing, it ensures that components meet specified dimensions, reducing defects and improving quality control. In retail, size recognition helps in inventory management by categorizing products accurately. In healthcare, it aids in diagnosing conditions based on the size of anatomical structures.
Accurate size recognition can lead to significant cost savings and efficiency improvements. For instance, automated systems can replace manual inspection processes, reducing human error and increasing throughput. This is particularly important in industries with high-volume production where consistency and precision are critical.
Machine learning techniques enhance size recognition by learning from vast amounts of data, identifying patterns, and making accurate predictions. These techniques can handle complex and varied data, making them suitable for real-world applications where traditional methods may fall short.

Role of Machine Learning Models
Machine learning models are pivotal in size recognition due to their ability to process and analyze large datasets. Convolutional Neural Networks (CNNs) are particularly effective for image-based size recognition tasks. They can capture spatial hierarchies and patterns, enabling accurate identification and categorization of objects based on their dimensions.
Other models, such as Support Vector Machines (SVMs) and Random Forests, can also be used for size recognition, especially when dealing with numerical data. These models can classify objects based on size-related features, providing reliable and efficient solutions.
By leveraging machine learning models, businesses can automate size recognition tasks, improving accuracy and efficiency. These models can be trained on labeled datasets to learn the relationship between input features and object sizes, making them capable of handling various scenarios and data types.
Data Preparation and Feature Engineering
Data preparation is a critical step in machine learning-based size recognition. It involves collecting, cleaning, and organizing data to ensure it is suitable for analysis. High-quality data is essential for training accurate and reliable models.

Feature engineering involves creating new features from existing data to improve model performance. For size recognition, this may include calculating aspect ratios, extracting edge features, or normalizing dimensions. These features help the model understand the underlying patterns and relationships in the data.
Here’s an example of data preparation and feature engineering using Pandas:
import pandas as pd
# Loading the dataset
df = pd.read_csv('size_data.csv')
# Handling missing values
df = df.dropna()
# Feature engineering: calculating aspect ratios
df['aspect_ratio'] = df['width'] / df['height']
# Normalizing dimensions
df['width_normalized'] = (df['width'] - df['width'].mean()) / df['width'].std()
df['height_normalized'] = (df['height'] - df['height'].mean()) / df['height'].std()
# Displaying the processed DataFrame
print(df.head())
Convolutional Neural Networks for Image-Based Size Recognition
Basics of Convolutional Neural Networks
Convolutional Neural Networks (CNNs) are a type of deep learning model designed for image processing tasks. They consist of convolutional layers that apply filters to input images, extracting features such as edges, textures, and shapes. These features are then used to classify or recognize objects in the image.
CNNs are highly effective for size recognition due to their ability to capture spatial hierarchies and patterns. By learning from large datasets of labeled images, CNNs can accurately identify and categorize objects based on their dimensions.

Key components of CNNs include convolutional layers, pooling layers, and fully connected layers. Convolutional layers extract features from the input image, pooling layers reduce dimensionality, and fully connected layers perform classification based on the extracted features.
Implementing CNNs with TensorFlow
TensorFlow is a popular deep learning framework for building and training CNNs. It provides a wide range of tools and libraries for creating complex neural network architectures and performing efficient computations. Using TensorFlow, you can implement a CNN for size recognition with just a few lines of code.
Here’s an example of implementing a CNN using TensorFlow:
import tensorflow as tf
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# Defining the CNN architecture
model = tf.keras.models.Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Conv2D(128, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dense(1, activation='linear')
])
# Compiling the model
model.compile(optimizer='adam', loss='mean_squared_error')
# Displaying the model summary
model.summary()
Training and Evaluating the CNN
Training a CNN involves feeding it labeled data, allowing it to learn the relationship between input images and their corresponding sizes. The model’s performance is evaluated using metrics such as mean squared error (MSE) for regression tasks or accuracy for classification tasks.

The dataset is typically split into training and validation sets to ensure the model generalizes well to unseen data. Data augmentation techniques, such as rotation and flipping, can be applied to increase the diversity of the training data and improve model robustness.
Here’s an example of training and evaluating a CNN using TensorFlow:
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# Creating data generators for training and validation
train_datagen = ImageDataGenerator(rescale=1./255, rotation_range=20, width_shift_range=0.2, height_shift_range=0.2, shear_range=0.2, zoom_range=0.2, horizontal_flip=True, fill_mode='nearest')
val_datagen = ImageDataGenerator(rescale=1./255)
train_generator = train_datagen.flow_from_directory('train_data', target_size=(64, 64), batch_size=32, class_mode='raw')
val_generator = val_datagen.flow_from_directory('val_data', target_size=(64, 64), batch_size=32, class_mode='raw')
# Training the model
history = model.fit(train_generator, epochs=10, validation_data=val_generator)
# Evaluating the model
val_loss = model.evaluate(val_generator)
print(f'Validation Loss: {val_loss}')
Support Vector Machines for Size Classification
Basics of Support Vector Machines
Support Vector Machines (SVMs) are supervised learning models used for classification and regression tasks. They work by finding the optimal hyperplane that separates data points of different classes in the feature space. SVMs are particularly effective for binary classification tasks but can be extended to multi-class problems.
For size recognition, SVMs can classify objects based on size-related features extracted from images or other data sources. The model learns to identify the decision boundaries that separate objects of different sizes, providing accurate and robust classification.

Key parameters of SVMs include the kernel type (e.g., linear, polynomial, radial basis function) and the regularization parameter, which controls the trade-off between maximizing the margin and minimizing classification errors.
Implementing SVMs with Scikit-learn
Scikit-learn is a popular machine learning library in Python that provides simple and efficient tools for data analysis and modeling. It includes implementations of SVMs and other machine learning algorithms, making it easy to apply these techniques to size recognition tasks.
Here’s an example of implementing an SVM using Scikit-learn:
from sklearn.svm import SVC
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Loading the dataset
X = df[['width_normalized', 'height_normalized', 'aspect_ratio']]
y = df['size_category']
# Splitting the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Defining the SVM model
svm_model = SVC(kernel='rbf', C=1.0, gamma='scale')
# Training the SVM model
svm_model.fit(X_train, y_train)
# Making predictions
y_pred = svm_model.predict(X_test)
# Evaluating the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Optimizing SVM Performance
Optimizing SVM performance involves tuning hyperparameters and selecting the appropriate kernel for the problem. Grid search and cross-validation are common techniques for finding the optimal hyperparameters. These methods systematically evaluate different combinations of parameters to identify the best-performing model.

Feature scaling is also important for SVMs, as it ensures that all features contribute equally to the decision boundary. Standardizing or normalizing features can improve the performance and convergence of the SVM model.
Here’s an example of optimizing SVM performance using Scikit-learn:
from sklearn.model_selection import GridSearchCV
from sklearn.preprocessing import StandardScaler
# Standardizing the features
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
# Defining the SVM model and parameter grid
svm_model = SVC()
param_grid = {'C': [0.1, 1, 10], 'gamma': ['scale', 'auto'], 'kernel': ['rbf', 'poly', 'linear']}
# Performing grid search with cross-validation
grid_search = GridSearchCV(svm_model, param_grid, cv=5)
grid_search.fit(X_train_scaled, y_train)
# Evaluating the optimized model
best_model = grid_search.best_estimator()
y_pred_optimized = best_model.predict(X_test_scaled)
optimized_accuracy = accuracy_score(y_test, y_pred_optimized)
print(f'Optimized Accuracy: {optimized_accuracy}')
Random Forests for Size Recognition
Basics of Random Forests
Random Forests are ensemble learning methods that combine multiple decision trees to improve classification and regression performance. Each tree in the forest is trained on a random subset of the data, and the final prediction is made by aggregating the predictions of all trees. This approach reduces overfitting and improves generalization.
For size recognition, Random Forests can classify objects based on a variety of size-related features. The model learns to identify the most important features and their relationships, providing accurate and robust classification.
Random Forests are particularly effective for handling large datasets with many features. They can also handle missing values and categorical variables, making them versatile and widely applicable.
Implementing Random Forests with Scikit-learn
Scikit-learn provides a straightforward implementation of Random Forests, allowing you to easily apply this technique to size recognition tasks. The library includes various hyperparameters for tuning the model, such as the number of trees, maximum depth, and criteria for splitting nodes.
Here’s an example of implementing a Random Forest using Scikit-learn:
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
# Defining the Random Forest model
rf_model = RandomForestClassifier(n_estimators=100, max_depth=None, random_state=42)
# Training the Random Forest model
rf_model.fit(X_train, y_train)
# Making predictions
y_pred_rf = rf_model.predict(X_test)
# Evaluating the model
print(classification_report(y_test, y_pred_rf))
Feature Importance in Random Forests
One of the key advantages of Random Forests is their ability to provide insights into feature importance. The model can rank features based on their contribution to the prediction, helping you understand which features are most relevant for size recognition.
Feature importance can be useful for feature selection, allowing you to focus on the most impactful features and potentially improve model performance. It can also provide valuable insights into the underlying patterns in the data.
Here’s an example of extracting feature importance using Scikit-learn:
# Extracting feature importance
feature_importances = rf_model.feature_importances_
feature_names = X.columns
importance_df = pd.DataFrame({'feature': feature_names, 'importance': feature_importances})
# Sorting and displaying feature importance
importance_df = importance_df.sort_values(by='importance', ascending=False)
print(importance_df)
Applications of Size Recognition
Manufacturing Quality Control
In manufacturing, size recognition is essential for quality control. Automated systems can inspect components for size conformity, ensuring that they meet specified dimensions and tolerances. This reduces defects and improves overall product quality.
Machine learning models can be integrated into manufacturing lines to perform real-time size recognition. By analyzing images or sensor data, these models can detect deviations from the desired size and trigger corrective actions. This automation enhances efficiency and reduces the need for manual inspections.
Implementing size recognition in manufacturing requires high precision and reliability. Machine learning models must be trained on extensive datasets to ensure they can accurately identify size deviations and provide consistent results.
Retail Inventory Management
In retail, accurate size recognition is crucial for inventory management. Products must be categorized correctly based on their dimensions to ensure efficient storage, retrieval, and shipment. Machine learning models can automate this process, reducing errors and improving inventory accuracy.
Retailers can use size recognition to optimize warehouse space, streamline logistics, and enhance customer satisfaction. For example, by accurately recognizing product sizes, retailers can ensure that items are stored in the most appropriate locations and that orders are packed efficiently.
Machine learning models for retail inventory management must handle a wide variety of product sizes and shapes. Training these models requires diverse datasets that capture the different characteristics of products in the inventory.
Healthcare and Medical Imaging
In healthcare, size recognition is used in medical imaging to diagnose and monitor conditions. For example, the size of tumors, organs, or anatomical structures can provide critical information for diagnosis and treatment planning. Machine learning models can analyze medical images to accurately measure and categorize these structures.
Automating size recognition in medical imaging improves the accuracy and speed of diagnosis, enabling timely interventions. Machine learning models can assist radiologists by highlighting areas of concern and providing quantitative measurements.
Implementing size recognition in healthcare requires high accuracy and robustness. Models must be trained on medical image datasets and validated rigorously to ensure they provide reliable results. Collaboration with medical professionals is essential to develop models that meet clinical needs.
Future Directions and Advancements
Integrating Deep Learning Models
The integration of deep learning models, such as CNNs and GANs, with traditional machine learning techniques can enhance size recognition capabilities. These models can capture complex patterns and relationships in data, providing more accurate and robust size recognition solutions.
Combining deep learning with ensemble methods, such as Random Forests, can improve performance by leveraging the strengths of both approaches. This hybrid approach can handle diverse data types and complex scenarios, making it suitable for a wide range of applications.
Future advancements in deep learning, including the development of more efficient architectures and training techniques, will further enhance the capabilities of size recognition models. These advancements will enable more accurate and scalable solutions for various industries.
Leveraging Transfer Learning
Transfer learning is a technique where models trained on one task are adapted for another related task. This approach can significantly reduce training time and improve model performance, especially when labeled data is limited. In size recognition, transfer learning can be used to leverage pre-trained models for tasks such as image classification or object detection.
By fine-tuning pre-trained models on size recognition datasets, you can achieve high accuracy with less data and computational resources. Transfer learning can also help improve model generalization, making it more robust to different data distributions and variations.
Future research in transfer learning will focus on developing techniques that enable seamless adaptation of models across diverse tasks and domains. This will enhance the applicability and effectiveness of size recognition models in real-world scenarios.
Enhancing Real-Time Processing
Real-time processing is essential for many size recognition applications, such as manufacturing quality control and retail inventory management. Advances in hardware, such as GPUs and specialized accelerators, enable faster computations and real-time inference for machine learning models.
Optimizing machine learning models for real-time processing involves reducing their complexity and latency while maintaining accuracy. Techniques such as model pruning, quantization, and efficient neural network architectures can help achieve this balance.
Future developments in hardware and optimization techniques will further enhance the real-time capabilities of size recognition models. These advancements will enable more responsive and scalable solutions for various industries, improving efficiency and effectiveness.
Machine learning-based size recognition offers powerful tools for various applications, from manufacturing quality control to healthcare and retail inventory management. By leveraging techniques such as CNNs, SVMs, and Random Forests, you can develop accurate and robust models for size recognition. The integration of deep learning, transfer learning, and real-time processing will further enhance the capabilities of these models, driving innovation and efficiency across different domains. Using tools like TensorFlow, Scikit-learn, and Pandas, you can implement and optimize machine learning models for size recognition, ensuring reliable and effective solutions.
If you want to read more articles similar to Top Techniques for Machine Learning-based Size Recognition, you can visit the Applications category.
You Must Read