
Understanding Long Short Term Memory (LSTM) in Machine Learning
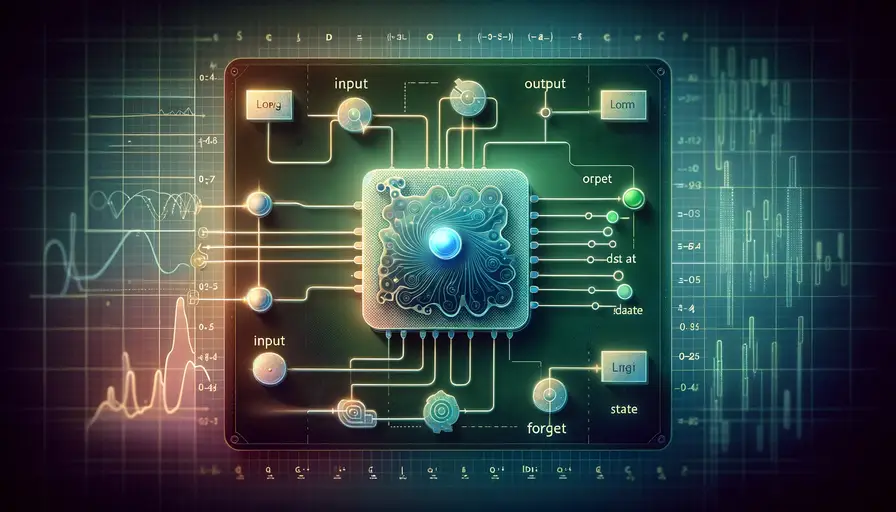
Long Short Term Memory (LSTM) networks are a powerful type of recurrent neural network (RNN) capable of learning long-term dependencies, particularly in sequence prediction problems. They were introduced by Hochreiter and Schmidhuber in 1997 and have since been improved and widely adopted in various applications. This article delves into the principles of LSTM networks, their architecture, and their diverse applications in machine learning.
The Basics of LSTM Networks
What Are LSTM Networks?
LSTM networks are a special kind of RNN designed to avoid the long-term dependency problem. Standard RNNs struggle with retaining information over long sequences, which can lead to the vanishing gradient problem during training. LSTMs address this issue with a unique structure that allows them to maintain a cell state that can carry information across many time steps.
The key to LSTMs is their ability to control the flow of information through three gates: the input gate, the forget gate, and the output gate. These gates regulate the addition of new information, the removal of old information, and the output of information from the cell state, respectively. This gating mechanism enables LSTMs to remember and utilize information over long sequences effectively.
LSTMs are particularly suited for tasks where the context and sequence of data are important. This includes applications like speech recognition, language modeling, and time series forecasting, where maintaining the order and context of information is crucial.
The Architecture of LSTM Networks
The architecture of an LSTM network involves a series of repeating modules, each containing four interacting layers: the cell state, the forget gate, the input gate, and the output gate. These components work together to manage the cell state and control the information flow through the network.
- Cell State: The cell state is a horizontal line running through the LSTM, serving as a highway for information. It can carry information across many time steps, and its content is adjusted by the gates.
- Forget Gate: This gate decides what information to discard from the cell state. It takes the previous hidden state and the current input and passes them through a sigmoid function to produce a value between 0 and 1.
- Input Gate: This gate decides what new information to add to the cell state. It consists of two parts: a sigmoid layer that determines which values to update and a tanh layer that creates new candidate values.
- Output Gate: This gate determines what information to output based on the cell state. It uses the cell state and the current input to produce the new hidden state.
Here’s an example of defining an LSTM model using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
# Building an LSTM model
model = Sequential([
LSTM(50, return_sequences=True, input_shape=(10, 1)),
LSTM(50, return_sequences=False),
Dense(1)
])
# Compiling the model
model.compile(optimizer='adam', loss='mse')
# Display the model summary
model.summary()
Advantages of LSTM Networks
LSTM networks offer several advantages over traditional RNNs, particularly in handling long-term dependencies and mitigating the vanishing gradient problem. These advantages make them highly effective for various sequence prediction tasks.
Firstly, LSTM networks can remember important information over long sequences, thanks to their gating mechanisms. This capability is crucial for tasks where the context and order of information are important, such as language modeling and speech recognition.
Secondly, LSTM networks are more robust to the vanishing gradient problem. The gates in LSTMs help regulate the flow of gradients, preventing them from becoming too small during backpropagation. This allows LSTMs to learn long-term dependencies more effectively than standard RNNs.
Lastly, LSTM networks are versatile and can be used in various applications. They have been successfully applied in fields such as natural language processing, time series analysis, and anomaly detection, demonstrating their broad applicability and effectiveness.
Applications of LSTM Networks
Natural Language Processing
In the field of natural language processing (NLP), LSTM networks have proven to be highly effective for tasks such as language modeling, machine translation, and text generation. Their ability to understand and generate sequences of words while maintaining the context makes them ideal for these applications.
For instance, LSTMs are used in language models to predict the next word in a sentence. By capturing the dependencies between words and phrases, LSTMs can generate coherent and contextually relevant text. This capability is utilized in applications like chatbots and text auto-completion.
In machine translation, LSTMs can be used to translate sentences from one language to another. By processing the input sentence word by word and maintaining the context, LSTMs can generate accurate translations. This is the principle behind models like Google's Neural Machine Translation (GNMT).
Here’s an example of implementing a simple LSTM for text generation using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
from tensorflow.keras.preprocessing.text import Tokenizer
from tensorflow.keras.preprocessing.sequence import pad_sequences
import numpy as np
# Sample text data
text = "Hello world, this is a sample text for LSTM networks in NLP."
# Tokenizing the text
tokenizer = Tokenizer()
tokenizer.fit_on_texts([text])
sequences = tokenizer.texts_to_sequences([text])[0]
# Preparing the input and output sequences
X = []
y = []
seq_length = 3
for i in range(len(sequences) - seq_length):
X.append(sequences[i:i+seq_length])
y.append(sequences[i+seq_length])
X = np.array(X)
y = np.array(y)
# Reshaping the input to be compatible with LSTM
X = X.reshape((X.shape[0], X.shape[1], 1))
# Building the LSTM model
model = Sequential([
LSTM(50, input_shape=(seq_length, 1)),
Dense(len(tokenizer.word_index) + 1, activation='softmax')
])
# Compiling the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy')
# Training the model
model.fit(X, y, epochs=100)
Time Series Forecasting
Time series forecasting is another domain where LSTM networks excel. They are used to predict future values based on historical data, making them invaluable in finance, weather prediction, and demand forecasting. The ability of LSTMs to capture temporal dependencies and trends makes them particularly suited for these tasks.
In finance, LSTM networks are used to predict stock prices, exchange rates, and other financial indicators. By analyzing past trends and patterns, LSTMs can provide accurate forecasts that help investors make informed decisions. Similarly, in weather forecasting, LSTMs are used to predict temperature, rainfall, and other weather-related variables.
Demand forecasting is another application where LSTMs are used to predict future demand for products and services. Retailers and manufacturers use these forecasts to manage inventory, optimize supply chains, and plan production schedules.
Here’s an example of implementing an LSTM for time series forecasting using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
import numpy as np
# Generating sample time series data
data = np.sin(np.arange(0, 100, 0.1))
X = []
y = []
seq_length = 10
for i in range(len(data) - seq_length):
X.append(data[i:i+seq_length])
y.append(data[i+seq_length])
X = np.array(X)
y = np.array(y)
# Reshaping the input to be compatible with LSTM
X = X.reshape((X.shape[0], X.shape[1], 1))
# Building the LSTM model
model = Sequential([
LSTM(50, input_shape=(seq_length, 1)),
Dense(1)
])
# Compiling the model
model.compile(optimizer='adam', loss='mse')
# Training the model
model.fit(X, y, epochs=20, batch_size=32)
# Making predictions
predictions = model.predict(X)
print(predictions)
Speech Recognition
Speech recognition is a field where LSTM networks have made significant advancements. The ability to process sequential data and maintain context over long periods makes LSTMs ideal for recognizing spoken language. Applications of LSTM networks in speech recognition include voice assistants, transcription services, and language translation.
Voice assistants like Google Assistant, Amazon Alexa, and Apple Siri use LSTM networks to understand and respond to spoken commands. These systems process audio input, convert it into text, and generate appropriate responses, all while maintaining context and understanding the intent behind the spoken words.
In transcription services, LSTM networks are used to convert spoken language into written text. This is useful in various settings, including medical transcription, legal documentation, and media subtitling. The ability to accurately recognize and transcribe speech is critical for these applications.
Here’s an example of implementing a simple LSTM for speech recognition using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense, TimeDistributed, Activation
import numpy as np
# Generating sample audio data (sine wave)
fs = 8000 # Sampling frequency
t = np.arange(0, 1.0,
1.0/fs)
audio = np.sin(2 * np.pi * 440 * t) # Sine wave with frequency of 440 Hz
# Preparing the input and output sequences
seq_length = 100
X = []
y = []
for i in range(0, len(audio) - seq_length, seq_length):
X.append(audio[i:i+seq_length])
y.append(audio[i+seq_length])
X = np.array(X)
y = np.array(y)
# Reshaping the input to be compatible with LSTM
X = X.reshape((X.shape[0], X.shape[1], 1))
# Building the LSTM model
model = Sequential([
LSTM(50, return_sequences=True, input_shape=(seq_length, 1)),
LSTM(50, return_sequences=False),
Dense(1),
Activation('linear')
])
# Compiling the model
model.compile(optimizer='adam', loss='mse')
# Training the model
model.fit(X, y, epochs=10, batch_size=32)
# Making predictions
predictions = model.predict(X)
print(predictions)
Advanced Techniques in LSTM Networks
Bidirectional LSTM Networks
Bidirectional LSTM (BiLSTM) networks are an extension of standard LSTMs that improve performance by processing input data in both forward and backward directions. This allows the network to capture information from both past and future contexts, making it particularly effective for tasks where context from both directions is important.
In BiLSTM networks, two separate LSTMs are used: one for the forward pass and one for the backward pass. The outputs of these two LSTMs are then combined to produce the final output. This bidirectional approach enhances the network's ability to understand the sequence and context of data.
Applications of BiLSTM networks include language modeling, speech recognition, and named entity recognition. By leveraging information from both directions, BiLSTMs can achieve higher accuracy and better performance compared to unidirectional LSTMs.
Here’s an example of implementing a BiLSTM network using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Bidirectional, LSTM, Dense
# Building a BiLSTM model
model = Sequential([
Bidirectional(LSTM(50, return_sequences=True), input_shape=(10, 1)),
Bidirectional(LSTM(50, return_sequences=False)),
Dense(1)
])
# Compiling the model
model.compile(optimizer='adam', loss='mse')
# Display the model summary
model.summary()
Attention Mechanisms in LSTM Networks
Attention mechanisms are techniques that allow LSTM networks to focus on specific parts of the input sequence when making predictions. This helps the network to selectively attend to relevant information, improving performance on tasks such as machine translation and text summarization.
Incorporating attention mechanisms into LSTM networks involves adding an additional layer that calculates attention weights for each time step. These weights determine the importance of each time step's information in making the final prediction. The weighted sum of the inputs is then used to produce the output.
Attention mechanisms have been instrumental in advancing NLP tasks. Models like Transformer and BERT utilize attention mechanisms to achieve state-of-the-art performance in various NLP benchmarks.
Here’s an example of implementing an attention mechanism in an LSTM network using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, LSTM, Dense, Attention
# Defining the input
inputs = Input(shape=(10, 1))
lstm_out, forward_h, forward_c, backward_h, backward_c = Bidirectional(
LSTM(50, return_sequences=True, return_state=True))(inputs)
state_h = tf.keras.layers.Concatenate()([forward_h, backward_h])
state_c = tf.keras.layers.Concatenate()([forward_c, backward_c])
# Attention layer
attention = Attention()([lstm_out, lstm_out])
attention = Dense(1, activation='tanh')(attention)
# Final output layer
output = Dense(1)(attention)
# Building the model
model = Model(inputs=inputs, outputs=output)
# Compiling the model
model.compile(optimizer='adam', loss='mse')
# Display the model summary
model.summary()
Combining LSTM Networks with CNNs
Combining LSTM networks with Convolutional Neural Networks (CNNs) leverages the strengths of both architectures, making it possible to handle spatial and temporal dependencies in data effectively. This combination is particularly useful in applications like video analysis, where both spatial and temporal information are important.
In this hybrid approach, CNNs are used to extract spatial features from the input data, such as frames in a video. The extracted features are then passed to an LSTM network, which captures the temporal dependencies and sequences in the data. This combination allows the model to process complex data with spatial and temporal patterns.
Applications of combined CNN and LSTM networks include action recognition in videos, video captioning, and gesture recognition. By integrating the capabilities of CNNs and LSTMs, these hybrid models can achieve high accuracy and performance.
Here’s an example of combining CNN and LSTM networks using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, LSTM, Dense, TimeDistributed
# Building a combined CNN-LSTM model
model = Sequential([
TimeDistributed(Conv2D(32, (3, 3), activation='relu'), input_shape=(10, 64, 64, 3)),
TimeDistributed(MaxPooling2D((2, 2))),
TimeDistributed(Flatten()),
LSTM(50),
Dense(1, activation='sigmoid')
])
# Compiling the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Display the model summary
model.summary()
Best Practices for Implementing LSTM Networks
Preparing Data for LSTM Networks
Effective implementation of LSTM networks begins with proper data preparation. This involves cleaning the data, handling missing values, and transforming variables to ensure they are suitable for modeling. Proper data preparation is crucial for the accuracy and reliability of LSTM models.
Key steps in data preparation include identifying and treating outliers, normalizing continuous variables, and encoding categorical variables. Feature engineering, such as creating interaction terms or polynomial features, can also enhance the model's performance by capturing complex relationships in the data.
Here’s an example of data preparation using Pandas:
import pandas as pd
import numpy as np
# Generating sample data
data = pd.DataFrame({
'feature1': np.random.randn(100),
'feature2': np.random.randn(100),
'target': np.random.randint(0, 2, 100)
})
# Handling missing values
data = data.dropna()
# Normalizing continuous variables
data['feature1'] = (data['feature1'] - data['feature1'].mean()) / data['feature1'].std()
data['feature2'] = (data['feature2'] - data['feature2'].mean()) / data['feature2'].std()
# Encoding categorical variables (if any)
# data['category'] = pd.get_dummies(data['category'], drop_first=True)
print(data.head())
Tuning Hyperparameters
Tuning hyperparameters is crucial for optimizing the performance of LSTM networks. Key hyperparameters include the number of layers, the number of units in each layer, the learning rate, and the batch size. Tuning these parameters involves experimenting with different values and evaluating the model's performance.
Grid search and random search are common techniques for hyperparameter tuning. Grid search exhaustively evaluates all combinations of hyperparameters, while random search randomly samples from the hyperparameter space. Advanced methods like Bayesian optimization use probabilistic models to guide the search for optimal hyperparameters.
Here’s an example of hyperparameter tuning using Keras Tuner:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
from keras_tuner import RandomSearch
def build_model(hp):
model = Sequential()
model.add(LSTM(units=hp.Int('units', min_value=32, max_value=512, step=32), input_shape=(10, 1)))
model.add(Dense(1))
model.compile(optimizer='adam', loss='mse')
return model
tuner = RandomSearch(
build_model,
objective='val_loss',
max_trials=10,
executions_per_trial=3,
directory='my_dir',
project_name='helloworld'
)
# Data preparation and model fitting steps would go here...
# tuner.search(X_train, y_train, epochs=10, validation_data=(X_val, y_val))
Evaluating and Validating Models
Evaluating and validating LSTM models is crucial for ensuring their accuracy and reliability. Cross-validation techniques, such as k-fold cross-validation, help in assessing the model's robustness and generalizability. It is important to use appropriate evaluation metrics, such as accuracy, precision, recall, and F1 score, depending on the specific task.
Regularly updating the model with new data ensures that it remains accurate and relevant. As new data becomes available, retraining the model helps in capturing any changes in the underlying distribution and improving predictive performance.
Monitoring the model's performance over time and incorporating feedback from users and stakeholders can also help in identifying areas for improvement and ensuring the model's continued effectiveness.
Here’s an example of model evaluation using Scikit-learn:
from sklearn.metrics import mean_squared_error, r2_score
# Generating sample predictions (actual predictions would come from the model)
y_true = np.random.rand(100)
y_pred = np.random.rand(100)
# Calculating evaluation metrics
mse = mean_squared_error(y_true, y_pred)
r2 = r2_score(y_true, y_pred)
print(f'Mean Squared Error: {mse}')
print(f'R-squared: {r2}')
Long Short Term Memory (LSTM) networks are a powerful tool in the machine learning arsenal, capable of handling long-term dependencies and sequential data effectively. By understanding their architecture, applications, and advanced techniques, researchers and practitioners can leverage LSTMs to tackle complex problems in natural language processing, time series forecasting, speech recognition, and beyond. Using tools like TensorFlow, Keras Tuner, and Pandas, implementing and optimizing LSTM networks becomes a manageable and impactful task.
If you want to read more articles similar to Understanding Long Short Term Memory (LSTM) in Machine Learning, you can visit the Artificial Intelligence category.
You Must Read