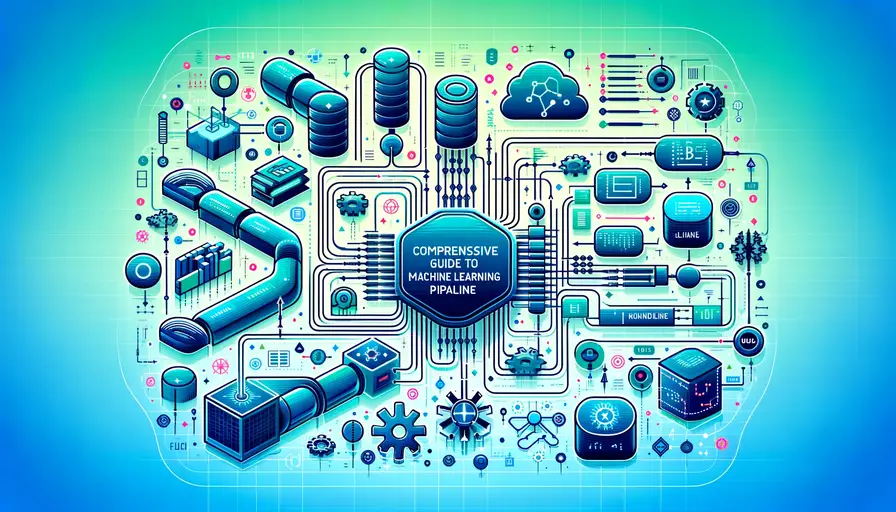
What is Long Short-Term Memory?
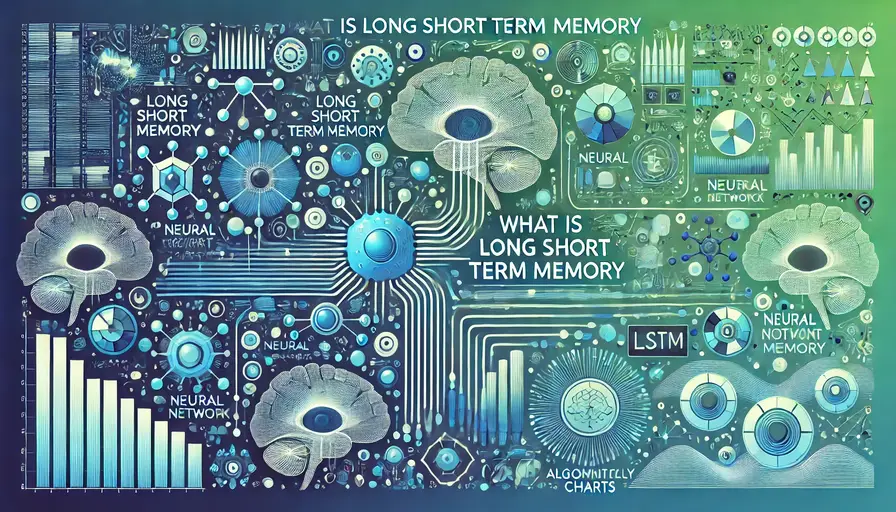
Long Short-Term Memory (LSTM) is a type of recurrent neural network (RNN) architecture that is well-suited for processing and predicting data with sequential dependencies. Unlike traditional RNNs, LSTMs are capable of learning long-term dependencies, making them highly effective for tasks such as language modeling, speech recognition, and time series forecasting.
Fundamentals of Long Short-Term Memory
Origins and Development
Long Short-Term Memory was introduced by Sepp Hochreiter and Jürgen Schmidhuber in 1997 to address the limitations of traditional RNNs, particularly the problem of vanishing gradients. The vanishing gradient problem makes it difficult for RNNs to learn long-term dependencies because gradients used for training diminish exponentially over time. LSTMs mitigate this issue by introducing a memory cell and gate mechanisms that regulate the flow of information.
Architecture and Components
The core of the LSTM architecture consists of memory cells and three primary gates: the input gate, the forget gate, and the output gate. These gates control the information that is added to or removed from the cell state. The input gate determines how much of the new input should be added to the cell state, the forget gate decides what portion of the existing cell state should be discarded, and the output gate regulates the output based on the cell state.
Mathematical Representation
The operations within an LSTM cell can be represented mathematically using the following equations:

Forget gate:
$$ f_t = \sigma(W_f \cdot [h_{t-1}, x_t] + b_f) $$
Input gate:
$$ i_t = \sigma(W_i \cdot [h_{t-1}, x_t] + b_i) $$
$$ \tilde{C}_t = \tanh(W_C \cdot [h_{t-1}, x_t] + b_C)$$

Cell state update:
$$ C_t = f_t * C_{t-1} + i_t * \tilde{C}_t $$
Output gate:
$$ o_t = \sigma(W_o \cdot [h_{t-1}, x_t] + b_o) $$
$$ h_t = o_t * \tanh(C_t) $$

Here, \(\sigma\) represents the sigmoid function, \(\tanh\) is the hyperbolic tangent function, and \(W\) and \(b\) are weights and biases, respectively.
Applications of Long Short-Term Memory
Natural Language Processing
LSTMs have revolutionized the field of natural language processing (NLP). They are extensively used in tasks such as language modeling, machine translation, and text generation. By capturing long-term dependencies in text, LSTMs can generate coherent and contextually relevant sentences. For instance, Google Translate uses LSTM networks to improve translation quality by considering the context of entire sentences rather than just individual words.
Time Series Forecasting
In time series forecasting, LSTMs excel at predicting future values based on historical data. Their ability to retain information over long sequences makes them ideal for applications such as stock price prediction, weather forecasting, and demand planning. By learning from patterns in past data, LSTMs can make accurate forecasts even in the presence of complex temporal dependencies.
Speech Recognition
LSTMs are pivotal in modern speech recognition systems. They are used to transcribe spoken language into text by processing audio signals over time. Amazon Alexa and Google Assistant leverage LSTM networks to understand and respond to voice commands. The ability of LSTMs to handle varying lengths of input sequences and maintain context makes them indispensable for achieving high accuracy in speech recognition tasks.

Implementing Long Short-Term Memory
Basic Implementation
Implementing an LSTM network in Python using popular libraries like TensorFlow or PyTorch is straightforward. Below is a basic example using TensorFlow to create an LSTM model for time series forecasting:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
# Sample data
x_train = [[[1], [2], [3]], [[2], [3], [4]], [[3], [4], [5]]]
y_train = [4, 5, 6]
# Define the LSTM model
model = Sequential()
model.add(LSTM(50, activation='relu', input_shape=(3, 1)))
model.add(Dense(1))
model.compile(optimizer='adam', loss='mse')
# Train the model
model.fit(x_train, y_train, epochs=200, verbose=0)
# Make predictions
x_input = [[4], [5], [6]]
x_input = np.array(x_input).reshape((1, 3, 1))
yhat = model.predict(x_input, verbose=0)
print(yhat)
Advanced Techniques
Advanced techniques can enhance the performance of LSTMs. Bidirectional LSTMs process data in both forward and backward directions, capturing dependencies from both past and future contexts. Attention mechanisms allow the network to focus on relevant parts of the input sequence, improving performance on tasks like machine translation. Combining LSTMs with Convolutional Neural Networks (CNNs) can further improve results in applications such as video analysis.
Hyperparameter Tuning
Optimizing LSTM networks involves tuning hyperparameters such as the number of layers, the number of units per layer, and the learning rate. Techniques like Grid Search and Bayesian Optimization can automate the hyperparameter tuning process. Using platforms like Kaggle for competitions and experimentation can provide practical insights into effective hyperparameter settings.
Comparison with Other RNN Variants
Gated Recurrent Units
Gated Recurrent Units (GRUs) are a variant of LSTMs with a simpler architecture. GRUs have two gates (reset and update) instead of three, which reduces computational complexity. Although GRUs often perform similarly to LSTMs, they are more efficient and easier to implement. The choice between LSTMs and GRUs depends on the specific application and dataset.

Vanilla RNNs
Vanilla RNNs are the simplest form of recurrent neural networks, with a single layer that loops over the sequence data. While they can handle short-term dependencies, they struggle with long-term dependencies due to the vanishing gradient problem. LSTMs address this limitation with their memory cells and gating mechanisms, making them more suitable for tasks requiring long-term memory.
Temporal Convolutional Networks
Temporal Convolutional Networks (TCNs) offer an alternative to RNNs by using convolutional layers to process sequential data. TCNs can capture long-term dependencies with a simpler training process and better parallelization. However, LSTMs remain popular due to their established effectiveness and wide adoption in various applications.
Challenges and Solutions in Long Short-Term Memory
Training Complexity
Training LSTMs can be computationally intensive due to their complex architecture. Parallel processing and hardware accelerators like GPUs and TPUs can significantly speed up training. Techniques such as gradient clipping help mitigate exploding gradients, ensuring stable training.
Overfitting
Overfitting is a common issue in LSTM networks, especially with small datasets. Regularization techniques such as dropout and L2 regularization help prevent overfitting by reducing model complexity. Cross-validation is another essential technique to ensure the model generalizes well to unseen data.

Sequence Lengths
Handling varying sequence lengths can be challenging in LSTM networks. Padding sequences to a fixed length and using masking layers in frameworks like TensorFlow help manage this issue. Truncated Backpropagation Through Time (TBPTT) is another technique to efficiently train LSTMs on long sequences by segmenting them into smaller chunks.
Real-World Examples
Language Modeling
LSTMs are widely used in language modeling tasks to predict the next word in a sequence, enabling applications like text completion and auto-correction. OpenAI's GPT and Google's BERT are examples of advanced language models leveraging LSTM architectures to understand and generate human language with high accuracy.
Financial Forecasting
In financial forecasting, LSTMs are used to predict stock prices, trading volumes, and other market indicators. Their ability to capture temporal dependencies makes them ideal for modeling complex financial time series. Companies like Bloomberg and Goldman Sachs use LSTM-based models to gain insights and make informed trading decisions.
Healthcare Analytics
LSTMs are transforming healthcare analytics by enabling predictive modeling for patient outcomes, disease progression, and treatment efficacy. They are used in applications like electronic health records (EHRs) to predict patient readmissions and genomics to analyze DNA sequences. Organizations like Mayo Clinic leverage LSTM models to enhance patient care and research.
Practical Considerations
Model Interpretation
Interpreting LSTM models can be challenging due to their complex architecture. Attention mechanisms provide insights into which parts of the input sequence the model focuses on, aiding interpretation. Model explainability tools like SHAP and LIME help demystify LSTM predictions, making them more transparent and trustworthy.
Resource Management
LSTMs require significant computational resources for training and deployment. Efficient resource management involves optimizing batch sizes, leveraging cloud services like AWS and Google Cloud, and using hardware accelerators. Model quantization and pruning are techniques to reduce model size and inference time without sacrificing accuracy.
Ethical Considerations
The deployment of LSTMs in sensitive applications like healthcare and finance raises ethical concerns regarding bias and fairness. Ensuring diverse and representative training data, implementing bias detection algorithms, and adhering to ethical guidelines are crucial for responsible AI development. Organizations like AI Now Institute advocate for ethical AI practices and provide resources to address these challenges.
Future Directions
Integration with Reinforcement Learning
Integrating LSTMs with reinforcement learning (RL) enables the development of intelligent agents capable of learning from sequential data and making decisions in dynamic environments. This integration enhances applications like autonomous driving, robotics, and game playing. DeepMind's AlphaStar is an example of an RL agent using LSTM networks to master complex strategy games.
Quantum Long Short-Term Memory
Quantum Long Short-Term Memory (QLSTM) is an emerging field that explores the use of quantum computing principles in LSTM architectures. QLSTM leverages quantum mechanics to enhance computational efficiency and solve problems intractable for classical LSTMs. Research in this area is nascent but holds promise for breakthroughs in quantum machine learning.
Automated Machine Learning
Automated Machine Learning (AutoML) aims to automate the design and tuning of LSTM networks, making advanced AI accessible to non-experts. Platforms like Google AutoML and H2O.ai provide tools for building and deploying LSTM models with minimal human intervention. The future of AutoML involves continuous advancements in automation, efficiency, and user-friendliness.
Long Short-Term Memory networks have revolutionized the field of deep learning with their ability to model sequential data effectively. By understanding their fundamentals, exploring advanced techniques, and addressing practical considerations, practitioners can harness the full potential of LSTMs for a wide range of applications. As research and technology advance, LSTMs will continue to play a pivotal role in the evolution of artificial intelligence and machine learning.
If you want to read more articles similar to What is Long Short-Term Memory?, you can visit the Algorithms category.
You Must Read