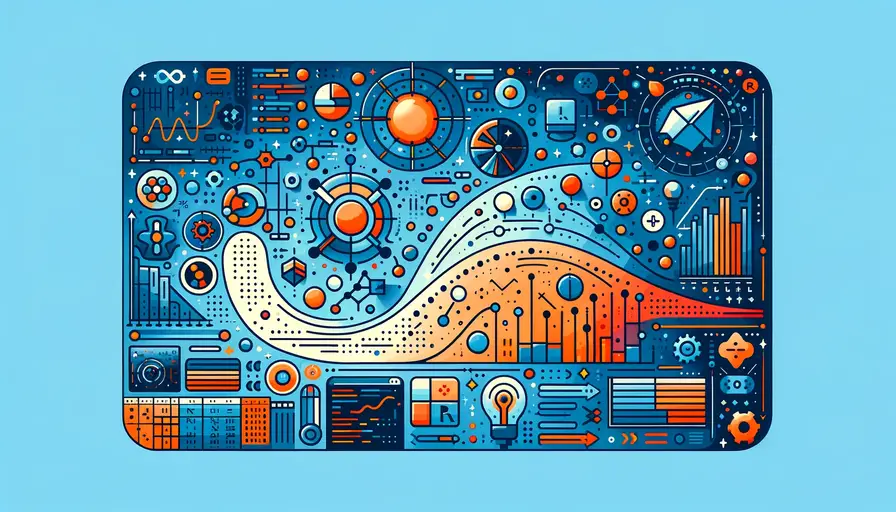
Writing Data for Machine Learning Algorithms

Collecting and Gathering Relevant Data
Collecting and gathering relevant data from various sources is the first and crucial step in creating an effective machine learning model. The quality and diversity of the data significantly impact the model's performance.
Identifying Data Sources
To start, identify diverse data sources relevant to your problem. These sources could include public datasets, company databases, APIs, or even web scraping. Ensuring the data covers various aspects of the problem will help build a robust model.
Gathering Data
Once the sources are identified, the next step is to gather the data. This can involve downloading datasets, querying databases, or writing scripts to extract data from APIs. It is important to ensure that the data collection process is automated and scalable to handle large volumes of data efficiently.
Ensuring Data Quality
During data collection, it is crucial to ensure data quality. This involves checking for completeness, consistency, and accuracy. Data with high quality will lead to more reliable and accurate machine learning models.

Cleaning and Preprocessing the Data
Cleaning and preprocessing the data is essential to remove any inconsistencies or errors. This step ensures that the data is in a suitable format for machine learning algorithms.
Handling Missing Data
Handling missing data is a critical preprocessing task. Missing values can be imputed using techniques such as mean, median, or mode imputation, or more advanced methods like k-nearest neighbors imputation. Alternatively, rows with missing values can be removed if they are few.
Here's an example of handling missing data using Python and pandas:
import pandas as pd
# Sample data with missing values
data = {'feature1': [1, 2, None, 4], 'feature2': [4, None, 3, 1]}
df = pd.DataFrame(data)
# Fill missing values with mean
df.fillna(df.mean(), inplace=True)
print(df)
Handling Outliers
Handling outliers is another important step. Outliers can be detected using statistical methods like z-scores or the IQR method and then either removed or transformed to reduce their impact on the model.

Encoding Categorical Variables
Encoding categorical variables is necessary for converting non-numeric data into a numerical format. Techniques such as one-hot encoding or label encoding can be used to transform categorical features into a format that machine learning algorithms can understand.
Here's an example of encoding categorical variables using Python and pandas:
# Sample categorical data
data = {'color': ['red', 'blue', 'green', 'blue']}
df = pd.DataFrame(data)
# One-hot encoding
df_encoded = pd.get_dummies(df)
print(df_encoded)
Splitting Data into Training and Testing Sets
Splitting the data into training and testing sets is crucial for model evaluation. This helps in assessing the model's performance on unseen data.
Training and Testing Split
A common practice is to split the data into training and testing sets using a ratio such as 80-20 or 70-30. The training set is used to train the model, while the testing set is used to evaluate its performance.

Importance of a Proper Split
Properly splitting the data ensures that the model is evaluated on a separate dataset, which helps in understanding its generalization ability. This step prevents overfitting and ensures that the model performs well on new, unseen data.
Example of Splitting Data
Here's an example of splitting data into training and testing sets using Python and scikit-learn:
from sklearn.model_selection import train_test_split
# Sample data
X = [[1, 2], [3, 4], [5, 6], [7, 8]]
y = [0, 1, 0, 1]
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
print(f'Training Data: {X_train}, {y_train}')
print(f'Testing Data: {X_test}, {y_test}')
Feature Engineering Techniques
Selecting and applying suitable feature engineering techniques enhances the data, making it more suitable for machine learning models.
Handling Missing Data
Revisit handling missing data as part of feature engineering to ensure no important information is lost. Impute missing values intelligently based on data distribution and context.

Encoding Categorical Variables
Encoding categorical variables again comes into play here, ensuring that categorical features are converted into numerical values, which can be processed by the machine learning algorithms.
Scaling and Normalization
Scaling and normalizing the data is essential to bring all features to the same scale. This step helps in improving the convergence speed of gradient-based algorithms and ensures that features contribute equally to the model.
Here's an example of scaling data using Python and scikit-learn:
from sklearn.preprocessing import StandardScaler
# Sample data
data = [[1, 2], [3, 4], [5, 6], [7, 8]]
# Scale data
scaler = StandardScaler()
scaled_data = scaler.fit_transform(data)
print(scaled_data)
Feature Selection
Feature selection involves selecting the most relevant features that contribute to the model's performance. Techniques like recursive feature elimination or feature importance from tree-based models can be used.

Creating New Features
Creating new features from existing data can provide additional insights and improve model performance. This could involve generating interaction features, polynomial features, or domain-specific features.
Choosing and Implementing Algorithms
Choosing and implementing an appropriate machine learning algorithm is crucial for the success of the project. The choice of algorithm depends on the nature of the problem and the characteristics of the data.
Data Preprocessing
Data preprocessing is the first step before applying any machine learning algorithm. This includes cleaning the data, handling missing values, encoding categorical variables, and scaling the data.
Training the Algorithm
Training the algorithm involves fitting the model to the training data. Different algorithms have different requirements for training, so it is essential to understand the specific needs of the chosen algorithm.

Here's an example of training a decision tree classifier using Python and scikit-learn:
from sklearn.tree import DecisionTreeClassifier
# Sample data
X_train = [[1, 2], [3, 4], [5, 6]]
y_train = [0, 1, 0]
# Train decision tree
model = DecisionTreeClassifier()
model.fit(X_train, y_train)
print(model.predict([[2, 3]]))
Evaluating and Fine-Tuning
Evaluating and fine-tuning the model is crucial to ensure optimal performance. This involves testing the model on the testing set and adjusting hyperparameters to improve accuracy and generalization.
Fine-Tuning the Model
Fine-tuning the model by adjusting hyperparameters is essential for achieving the best performance. Different methods can be used to find the optimal set of hyperparameters.
Manual Tuning
Manual tuning involves adjusting hyperparameters based on experience and intuition. While this method can be effective, it is often time-consuming and may not always yield the best results.
Grid Search
Grid search is a systematic approach to hyperparameter tuning. It involves specifying a set of possible values for each hyperparameter and evaluating the model for each combination. This method ensures that the optimal combination is found but can be computationally expensive.
Example of Grid Search
Here's an example of using grid search for hyperparameter tuning using Python and scikit-learn:
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
# Sample data
X_train = [[1, 2], [3, 4], [5, 6]]
y_train = [0, 1, 0]
# Define model and parameters
model = RandomForestClassifier()
param_grid = {'n_estimators': [10, 50, 100], 'max_depth': [None, 10, 20]}
# Grid search
grid_search = GridSearchCV(model, param_grid, cv=3)
grid_search.fit(X_train, y_train)
print(grid_search.best_params_)
Random Search
Random search is an alternative to grid search that evaluates a random subset of the hyperparameter space. This method is often more efficient and can find good hyperparameter combinations with fewer evaluations.
Bayesian Optimization
Bayesian optimization uses probabilistic models to select the most promising hyperparameter values. This method is more efficient than grid search and random search and can find the optimal set of hyperparameters with fewer evaluations.
Validating the Model
Validating the model using the testing data is essential to assess its generalization ability. This step ensures that the model performs well on new, unseen data.
Model Validation
Model validation involves evaluating the model's performance on the testing set. Metrics such as accuracy, precision, recall, and F1-score are commonly used to assess the model's performance.
Generalization Ability
Generalization ability refers to the model's capability to perform well on new, unseen data. Validating the model on the testing set provides an estimate of its generalization performance.
Example of Model Validation
Here's an example of validating a model using Python and scikit-learn:
from sklearn.metrics import accuracy_score
# Sample data
X_test = [[2, 3], [4, 5]]
y_test = [0, 1]
# Model predictions
y_pred = model.predict(X_test)
# Validate model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Making Predictions
Using the trained model to make predictions or classify new data points is the final step in the machine learning pipeline. This involves applying the model to new data and interpreting the results.
Predicting New Data
Predicting new data involves feeding new, unseen data points into the trained model to obtain predictions. This step is crucial for deploying the model in real-world applications.
Interpreting Results
Interpreting the results of the predictions is essential for making informed decisions. This involves understanding the model's output and the confidence of its predictions.
Example of Making Predictions
Here's an example of making predictions using the trained model in Python:
# New data points
new_data = [[6, 7], [8, 9]]
# Make predictions
predictions = model.predict(new_data)
print(f'Predictions: {predictions}')
Monitoring and Updating the Model
Monitoring and updating the model periodically is essential to adapt to changing data patterns. This step ensures that the model remains accurate and relevant over time.
Continuous Monitoring
Continuous monitoring involves tracking the model's performance in real-time and detecting any decline in accuracy or changes in data distribution. This helps identify when the model needs to be retrained or updated.
Updating the Model
Updating the model involves retraining it with new data or adjusting its parameters to improve performance. This step is crucial for maintaining the model's accuracy and relevance as new data becomes available.
Importance of Adaptation
Adapting to changing data patterns is essential for the long-term success of the machine learning model. Regular updates ensure that the model remains effective in dynamic environments.
Writing data for machine learning algorithms involves a comprehensive process of data collection, preprocessing, splitting, feature engineering, model selection, training, validation, and continuous monitoring. By following these steps meticulously, one can build robust and accurate machine learning models that provide valuable insights and predictions for various applications.
If you want to read more articles similar to Writing Data for Machine Learning Algorithms, you can visit the Applications category.
You Must Read