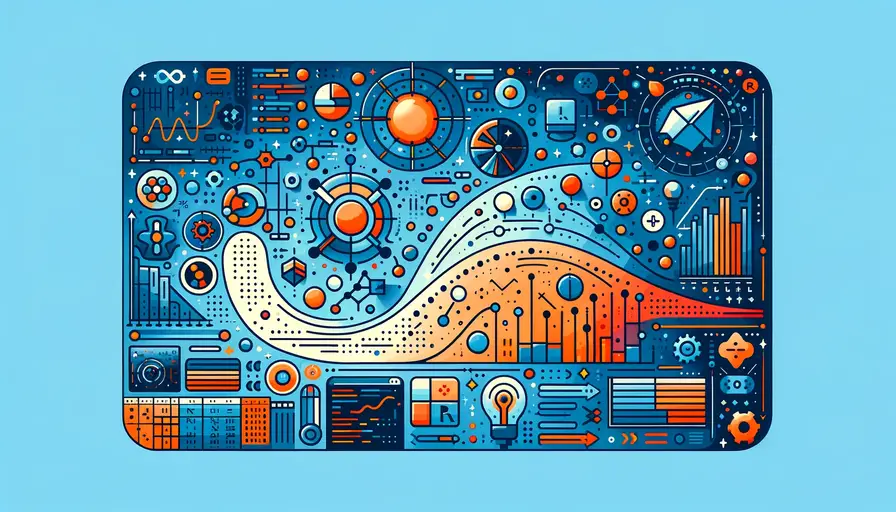
Innovative Project Ideas for Data Mining and Machine Learning
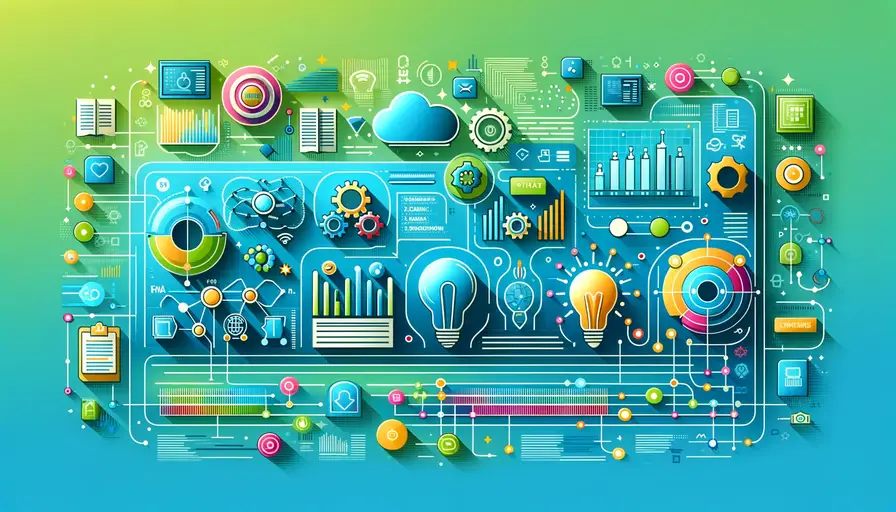
Transforming Healthcare with Data Mining and Machine Learning
Predictive Analytics for Patient Care
Predictive analytics in healthcare has the potential to revolutionize patient care by enabling early diagnosis and personalized treatment plans. By analyzing historical patient data, machine learning models can predict disease onset, progression, and response to treatments. This allows healthcare providers to intervene early and tailor treatments to individual patients, improving outcomes and reducing costs.
One innovative project idea is developing a predictive model to forecast hospital readmissions. High readmission rates are a significant issue for hospitals, often indicating suboptimal patient care and resulting in financial penalties. By leveraging data from electronic health records (EHRs), such as patient demographics, medical history, and previous hospitalizations, a machine learning model can identify patients at high risk of readmission. This enables healthcare providers to allocate resources more effectively and implement preventive measures.
Another project could involve predicting adverse drug reactions (ADRs). ADRs are a major concern in healthcare, leading to patient harm and increased healthcare costs. By mining data from clinical trials, patient records, and pharmacovigilance databases, a machine learning model can identify patterns and risk factors associated with ADRs. This can help in developing safer drug prescriptions and monitoring systems, ensuring patient safety.
Example: Predicting Hospital Readmissions Using Random Forest in Python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load dataset
data = pd.read_csv('hospital_readmissions.csv')
X = data.drop('readmission', axis=1)
y = data['readmission']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Random Forest classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model performance
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
In this example, a Random Forest classifier is used to predict hospital readmissions based on patient data. The model can identify high-risk patients, allowing healthcare providers to take preventive actions.

Enhancing Medical Imaging
Medical imaging is another area where data mining and machine learning can make a significant impact. Traditional methods of analyzing medical images, such as X-rays, MRIs, and CT scans, rely heavily on the expertise of radiologists. Machine learning models, particularly deep learning models like convolutional neural networks (CNNs), can assist in analyzing these images more accurately and efficiently.
A project idea in this domain is developing a deep learning model for early detection of cancer from medical images. By training a CNN on a large dataset of labeled medical images, the model can learn to recognize patterns indicative of cancerous tissues. This can help radiologists in identifying potential cases of cancer at an early stage, when treatment is more likely to be successful.
Another innovative project could involve creating a machine learning model to detect diabetic retinopathy, a leading cause of blindness. Using retinal images, a deep learning model can identify early signs of this condition, enabling timely intervention and preventing vision loss. Such models can be integrated into screening programs, especially in remote areas where access to specialized healthcare is limited.
Example: Detecting Diabetic Retinopathy Using CNN in Python
import tensorflow as tf
from tensorflow.keras import layers, models
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# Load dataset
train_datagen = ImageDataGenerator(rescale=1./255, validation_split=0.2)
train_generator = train_datagen.flow_from_directory(
'retinal_images/train',
target_size=(150, 150),
batch_size=32,
class_mode='binary',
subset='training'
)
validation_generator = train_datagen.flow_from_directory(
'retinal_images/train',
target_size=(150, 150),
batch_size=32,
class_mode='binary',
subset='validation'
)
# Build CNN model
model = models.Sequential()
model.add(layers.Conv2D(32, (3, 3), activation='relu', input_shape=(150, 150, 3)))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Conv2D(64, (3, 3), activation='relu'))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Conv2D(128, (3, 3), activation='relu'))
model.add(layers.MaxPooling2D((2, 2)))
model.add(layers.Flatten())
model.add(layers.Dense(512, activation='relu'))
model.add(layers.Dense(1, activation='sigmoid'))
# Compile model
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# Train model
model.fit(train_generator, epochs=10, validation_data=validation_generator)
In this example, a CNN is used to detect diabetic retinopathy from retinal images. The model can assist in early diagnosis, helping to prevent vision loss in diabetic patients.

Optimizing Resource Allocation
Efficient resource allocation is crucial in healthcare to ensure that patients receive timely and appropriate care. Data mining and machine learning can play a significant role in optimizing the allocation of resources such as hospital beds, medical staff, and equipment. By analyzing historical data and predicting future demand, healthcare providers can make informed decisions about resource distribution.
One project idea is developing a predictive model to forecast emergency department (ED) visits. By analyzing data such as historical visit records, weather conditions, and public health trends, a machine learning model can predict the number of patients likely to visit the ED on a given day. This allows hospitals to allocate staff and resources more effectively, reducing wait times and improving patient care.
Another innovative project could involve creating a model to optimize the scheduling of surgeries. By predicting the duration and complexity of different types of surgeries, the model can help in scheduling operations more efficiently, minimizing downtime and ensuring that operating rooms are utilized optimally. This can lead to better patient outcomes and increased efficiency in surgical departments.
Example: Predicting ED Visits Using Linear Regression in Python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Load dataset
data = pd.read_csv('ed_visits.csv')
X = data.drop('visits', axis=1)
y = data['visits']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Linear Regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model performance
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
In this example, a Linear Regression model is used to predict the number of ED visits based on various factors. The model helps in optimizing resource allocation in emergency departments.

Transforming Finance with Data Mining and Machine Learning
Fraud Detection and Prevention
Fraud detection is a critical application of data mining and machine learning in the finance industry. Fraudulent activities can lead to significant financial losses and damage to reputation. Machine learning models can analyze transaction data to identify suspicious patterns and detect fraud in real-time, enabling financial institutions to take preventive measures.
One innovative project idea is developing a machine learning model for credit card fraud detection. By analyzing transaction data, such as the amount, location, and time of transactions, the model can identify unusual patterns indicative of fraudulent activity. Techniques such as anomaly detection and supervised learning can be used to build robust fraud detection systems.
Another project could involve detecting fraudulent insurance claims. By mining data from past claims, a machine learning model can identify common characteristics of fraudulent claims, such as inflated amounts or inconsistent information. This can help insurance companies in investigating suspicious claims more efficiently, reducing losses and maintaining the integrity of the insurance system.
Example: Credit Card Fraud Detection Using Random Forest in Python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score, classification_report
# Load dataset
data = pd.read_csv('credit_card_fraud.csv')
X = data.drop('fraud', axis=1)
y = data['fraud']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Random Forest classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model performance
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
print(classification_report(y_test, y_pred))
In this example, a Random Forest classifier is used to detect credit card fraud based on transaction data. The model helps in identifying fraudulent transactions, reducing financial losses.

Algorithmic Trading
Algorithmic trading involves using machine learning models to make trading decisions based on market data. These models can analyze historical price data, news, and other factors to predict future price movements and execute trades automatically. Algorithmic trading can lead to higher returns and reduced risks by making data-driven decisions.
One project idea is developing a machine learning model for stock price prediction. By analyzing historical stock prices and market indicators, the model can predict future price movements and generate trading signals. Techniques such as time series analysis, LSTM networks, and reinforcement learning can be used to build effective trading models.
Another innovative project could involve creating a model for sentiment-based trading. By analyzing news articles, social media posts, and other sources of information, the model can gauge market sentiment and predict its impact on stock prices. This approach combines natural language processing (NLP) with machine learning to make informed trading decisions based on market sentiment.
Example: Stock Price Prediction Using LSTM in Python
import numpy as np
import pandas as pd
from sklearn.preprocessing import MinMaxScaler
from keras.models import Sequential
from keras.layers import LSTM, Dense
# Load dataset
data = pd.read_csv('stock_prices.csv')
scaler = MinMaxScaler(feature_range=(0, 1))
scaled_data = scaler.fit_transform(data['Close'].values.reshape(-1, 1))
# Create sequences for training
def create_sequences(data, seq_length):
X, y = [], []
for i in range(len(data) - seq_length):
X.append(data[i:i + seq_length])
y.append(data[i + seq_length])
return np.array(X), np.array(y)
seq_length = 60
X, y = create_sequences(scaled_data, seq_length)
X_train, y_train = X[:-100], y[:-100]
X_test, y_test = X[-100:], y[-100:]
# Build and train LSTM model
model = Sequential()
model.add(LSTM(50, return_sequences=True, input_shape=(seq_length, 1)))
model.add(LSTM(50))
model.add(Dense(1))
model.compile(optimizer='adam', loss='mean_squared_error')
model.fit(X_train, y_train, epochs=20, batch_size=32, validation_data=(X_test, y_test))
# Make predictions
predictions = model.predict(X_test)
predictions = scaler.inverse_transform(predictions)
# Evaluate model performance
actual = scaler.inverse_transform(y_test.reshape(-1, 1))
mse = np.mean((predictions - actual) ** 2)
print(f'Mean Squared Error: {mse}')
In this example, an LSTM model is built to predict stock prices based on historical data, illustrating how machine learning can be used for algorithmic trading.

Credit Scoring
Credit scoring is another crucial application of data mining and machine learning in the finance industry. Accurate credit scoring models help financial institutions assess the creditworthiness of individuals and businesses, reducing the risk of default and improving lending decisions. Machine learning models can analyze a wide range of data, such as credit history, income, and transaction patterns, to generate reliable credit scores.
One project idea is developing a machine learning model for personal credit scoring. By analyzing data from credit reports, bank statements, and other sources, the model can predict an individual's likelihood of defaulting on a loan. Techniques such as logistic regression, decision trees, and gradient boosting can be used to build effective credit scoring models.
Another innovative project could involve creating a model for small business credit scoring. By analyzing financial statements, business plans, and market conditions, the model can assess the creditworthiness of small businesses and predict their ability to repay loans. This can help financial institutions in making informed lending decisions and supporting the growth of small businesses.
Example: Personal Credit Scoring Using Logistic Regression in Python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, roc_auc_score
# Load dataset
data = pd.read_csv('credit_scoring.csv')
X = data.drop('default', axis=1)
y = data['default']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Logistic Regression model
model = LogisticRegression(max_iter=1000)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model performance
accuracy = accuracy_score(y_test, y_pred)
roc_auc = roc_auc_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
print(f'ROC AUC Score: {roc_auc}')
In this example, a Logistic Regression model is used for personal credit scoring, demonstrating how machine learning can help in assessing creditworthiness and reducing the risk of default.

Transforming Retail with Data Mining and Machine Learning
Personalized Recommendations
Personalized recommendations are a powerful application of data mining and machine learning in the retail industry. By analyzing customer data, such as purchase history, browsing behavior, and preferences, machine learning models can generate personalized recommendations that enhance the shopping experience and increase sales.
One project idea is developing a recommendation system for an e-commerce platform. By leveraging collaborative filtering and content-based filtering techniques, the model can recommend products that are likely to interest individual customers. This can lead to higher customer satisfaction and increased revenue for the platform.
Another innovative project could involve creating a recommendation system for a physical retail store. By analyzing data from loyalty programs, in-store sensors, and customer interactions, the model can recommend products and promotions tailored to individual customers. This can enhance the in-store shopping experience and drive customer loyalty.
Example: Product Recommendation Using Collaborative Filtering in Python
import pandas as pd
from sklearn.metrics.pairwise import cosine_similarity
from scipy.sparse import csr_matrix
# Load dataset
data = pd.read_csv('ratings.csv')
user_item_matrix = data.pivot(index='user_id', columns='item_id', values='rating').fillna(0)
user_item_matrix_sparse = csr_matrix(user_item_matrix.values)
# Compute cosine similarity between users
user_similarity = cosine_similarity(user_item_matrix_sparse)
# Function to recommend items for a given user
def recommend_items(user_id, user_item_matrix, user_similarity, top_n=5):
user_index = user_item_matrix.index.get_loc(user_id)
similarity_scores = user_similarity[user_index]
user_ratings = user_item_matrix.iloc[user_index]
unrated_items = user_ratings[user_ratings == 0].index
scores = user_item_matrix[unrated_items].dot(similarity_scores)
recommended_items = scores.nlargest(top_n).index
return recommended_items
# Example recommendation
recommended_items = recommend_items(1, user_item_matrix, user_similarity)
print(f'Recommended items for user 1: {recommended_items}')
In this example, a recommendation system using collaborative filtering is built to suggest products for users based on their similarity to other users, demonstrating the application of machine learning in personalized recommendations.
Inventory Management
Efficient inventory management is crucial for retail businesses to ensure that products are available when customers need them while minimizing excess stock. Machine learning models can analyze historical sales data, seasonal trends, and other factors to optimize inventory levels and improve supply chain efficiency.
One project idea is developing a predictive model for demand forecasting. By analyzing past sales data, promotions, and external factors such as weather and holidays, the model can predict future demand for different products. This helps retailers in making informed decisions about inventory levels, reducing stockouts and overstock situations.
Another innovative project could involve creating a model for optimizing reorder points. By predicting the optimal time to reorder products based on sales patterns and lead times, the model can help retailers maintain adequate stock levels without tying up too much capital in inventory. This can lead to cost savings and improved customer satisfaction.
Example: Demand Forecasting Using Time Series Analysis in Python
import pandas as pd
from statsmodels.tsa.holtwinters import ExponentialSmoothing
# Load dataset
data = pd.read_csv('sales_data.csv', index_col='date', parse_dates=True)
train_data = data[:-30]
test_data = data[-30:]
# Train Exponential Smoothing model
model = ExponentialSmoothing(train_data['sales'], trend='add', seasonal='add', seasonal_periods=12)
fit = model.fit()
# Make predictions
predictions = fit.forecast(steps=30)
# Evaluate model performance
mse = ((predictions - test_data['sales']) ** 2).mean()
print(f'Mean Squared Error: {mse}')
In this example, an Exponential Smoothing model is used for demand forecasting based on historical sales data, illustrating how machine learning can optimize inventory management.
Customer Segmentation
Customer segmentation is a key strategy for retailers to understand their customers better and tailor their marketing efforts. By dividing customers into distinct groups based on their behavior, preferences, and demographics, retailers can develop targeted marketing campaigns that resonate with each segment.
One project idea is developing a machine learning model for customer segmentation using clustering techniques. By analyzing data such as purchase history, browsing behavior, and demographic information, the model can identify distinct customer segments. Techniques such as k-means clustering and hierarchical clustering can be used to group customers with similar characteristics.
Another innovative project could involve creating a model for real-time customer segmentation. By analyzing data from customer interactions, such as website visits and social media activity, the model can dynamically update customer segments. This enables retailers to deliver personalized marketing messages in real-time, enhancing customer engagement and driving sales.
Example: Customer Segmentation Using K-Means Clustering in Python
import pandas as pd
from sklearn.cluster import KMeans
from sklearn.preprocessing import StandardScaler
# Load dataset
data = pd.read_csv('customer_data.csv')
X = data[['age', 'annual_income', 'spending_score']]
# Standardize features
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# Train K-Means clustering model
kmeans = KMeans(n_clusters=5, random_state=42)
kmeans.fit(X_scaled)
# Assign cluster labels to customers
data['cluster'] = kmeans.labels_
# Display cluster centers
print(f'Cluster Centers: {kmeans.cluster_centers_}')
In this example, a K-Means Clustering model is used for customer segmentation based on demographic and behavioral data, demonstrating how machine learning can enhance targeted marketing strategies.
Data mining and machine learning offer numerous innovative project ideas across various industries, including healthcare, finance, and retail. By leveraging these technologies, businesses can improve decision-making, optimize operations, and deliver personalized experiences to their customers. From predictive analytics and fraud detection to personalized recommendations and inventory management, the applications of data mining and machine learning are vast and transformative. By exploring these project ideas, you can harness the power of data to drive innovation and achieve better outcomes in your organization.
If you want to read more articles similar to Innovative Project Ideas for Data Mining and Machine Learning, you can visit the Applications category.
You Must Read