
Machine Learning's Impact on Language Understanding
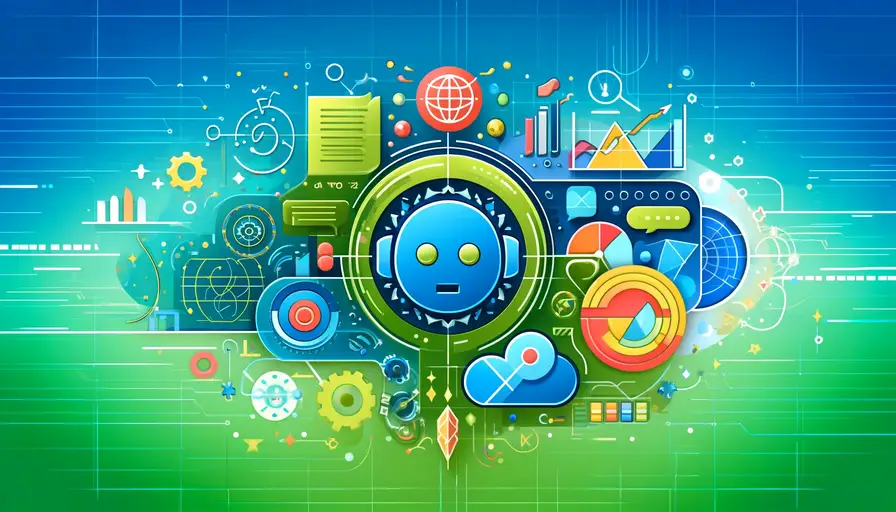
Content
Transforming Natural Language Processing with Machine Learning
Revolutionizing Text Analysis
Machine learning has fundamentally transformed natural language processing (NLP), enabling more sophisticated text analysis than ever before. Traditional text analysis methods relied heavily on manual rules and heuristics, which were often limited in their ability to handle the nuances and complexities of human language. Machine learning models, however, can learn from large datasets, capturing intricate patterns and subtleties in text data.
One of the key advancements in NLP through machine learning is sentiment analysis. This technique determines the emotional tone behind a body of text, such as customer reviews or social media posts. By leveraging models like BERT (Bidirectional Encoder Representations from Transformers) and GPT (Generative Pre-trained Transformer), sentiment analysis can accurately gauge public opinion and provide valuable insights into consumer behavior. These models can understand context and sentiment in a way that was previously impossible with rule-based systems.
Moreover, topic modeling has benefited immensely from machine learning. Algorithms like Latent Dirichlet Allocation (LDA) and Non-negative Matrix Factorization (NMF) can automatically identify topics within large text corpora, enabling researchers and businesses to uncover hidden themes and trends. This capability is particularly useful for analyzing unstructured data, such as news articles, academic papers, and social media conversations, where manual categorization would be impractical.
Enhancing Machine Translation
Machine translation is another area where machine learning has made significant strides. Early translation systems relied on extensive bilingual dictionaries and rule-based grammar, which often resulted in stilted and inaccurate translations. The advent of neural machine translation (NMT) models, such as Google's Neural Machine Translation (GNMT) and OpenAI's GPT, has dramatically improved the fluency and accuracy of translations.
AI and Machine Learning in Unity for Enhanced Game DevelopmentNMT models work by encoding sentences into high-dimensional vectors that capture their meaning, then decoding these vectors into the target language. This approach allows the model to consider the context of entire sentences rather than translating word-by-word, leading to more natural and coherent translations. For instance, Google's GNMT system translates entire sentences at once, providing translations that better preserve the meaning and nuances of the original text.
Another breakthrough in machine translation is the concept of zero-shot translation, where models can translate between language pairs they have never seen during training. This capability is achieved by training on multilingual datasets, allowing the model to learn general representations of language that can be applied across different languages. Zero-shot translation opens up possibilities for translating less common languages and dialects, making information more accessible to a global audience.
Example: Implementing Sentiment Analysis with BERT in Python
import numpy as np
import pandas as pd
from transformers import BertTokenizer, BertForSequenceClassification
from transformers import Trainer, TrainingArguments
# Load dataset
data = pd.read_csv('sentiment_data.csv')
train_data = data.sample(frac=0.8, random_state=42)
test_data = data.drop(train_data.index)
# Tokenize data
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
train_encodings = tokenizer(train_data['text'].tolist(), truncation=True, padding=True)
test_encodings = tokenizer(test_data['text'].tolist(), truncation=True, padding=True)
# Create dataset class
class SentimentDataset(torch.utils.data.Dataset):
def __init__(self, encodings, labels):
self.encodings = encodings
self.labels = labels
def __getitem__(self, idx):
item = {key: torch.tensor(val[idx]) for key, val in self.encodings.items()}
item['labels'] = torch.tensor(self.labels[idx])
return item
def __len__(self):
return len(self.labels)
train_dataset = SentimentDataset(train_encodings, train_data['label'].tolist())
test_dataset = SentimentDataset(test_encodings, test_data['label'].tolist())
# Initialize model
model = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=16,
per_device_eval_batch_size=16,
warmup_steps=500,
weight_decay=0.01,
logging_dir='./logs',
logging_steps=10,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=test_dataset
)
# Train model
trainer.train()
In this example, a BERT model is used to perform sentiment analysis on a dataset of text samples. The model is fine-tuned on the specific task of classifying sentiment, showcasing the application of advanced NLP techniques in understanding language.
Advancements in Dialogue Systems and Conversational AI
Building Intelligent Chatbots
Machine learning has revolutionized the development of chatbots, making them more intelligent and capable of engaging in natural conversations. Traditional rule-based chatbots were limited by their predefined scripts and often failed to handle unexpected user inputs. In contrast, modern chatbots powered by machine learning can understand and generate human-like responses, providing more fluid and meaningful interactions.
Optimizing Databricks ML: Identifying Key Power ScenariosOne of the key advancements in this area is the use of transformer-based models, such as GPT-3 and DialoGPT. These models are trained on vast amounts of conversational data and can generate coherent and contextually relevant responses. By understanding the context and nuances of a conversation, transformer-based chatbots can provide more accurate and helpful responses, improving user satisfaction.
Additionally, reinforcement learning techniques have been applied to train chatbots to optimize their responses based on user feedback. This approach allows chatbots to learn from interactions and continuously improve their performance. For example, a customer service chatbot can be trained to handle a wide range of queries by rewarding successful resolutions and penalizing unhelpful responses, leading to more effective and efficient customer support.
Improving Voice Assistants
Voice assistants like Amazon's Alexa, Google Assistant, and Apple's Siri have become integral parts of our daily lives, thanks to advancements in machine learning. These assistants rely on sophisticated speech recognition and natural language understanding (NLU) models to interpret and respond to voice commands accurately. Machine learning has enabled these systems to handle a wide range of tasks, from setting reminders and playing music to controlling smart home devices.
One significant improvement in voice assistants is the ability to handle contextual conversations. Early voice assistants could only respond to isolated commands, but modern systems can maintain context across multiple interactions. This capability allows users to have more natural and intuitive conversations with their voice assistants. For instance, a user can ask about the weather, then follow up with a related question without having to repeat the context.
Python Model for Detecting Fake News: Step-by-Step GuideAnother area of advancement is multi-turn dialogue management, where voice assistants can handle complex interactions involving multiple exchanges. This capability is particularly useful for tasks that require gathering information over several turns, such as booking a flight or scheduling an appointment. By understanding the user's intent and maintaining context, voice assistants can provide a seamless and efficient experience.
Example: Building a Simple Chatbot with DialoGPT in Python
from transformers import AutoModelForCausalLM, AutoTokenizer
import torch
# Load pre-trained DialoGPT model and tokenizer
tokenizer = AutoTokenizer.from_pretrained("microsoft/DialoGPT-medium")
model = AutoModelForCausalLM.from_pretrained("microsoft/DialoGPT-medium")
# Chat function
def chat_with_bot(prompt):
# Encode input and generate response
input_ids = tokenizer.encode(prompt + tokenizer.eos_token, return_tensors="pt")
chat_history_ids = model.generate(input_ids, max_length=1000, pad_token_id=tokenizer.eos_token_id)
# Decode and return response
response = tokenizer.decode(chat_history_ids[:, input_ids.shape[-1]:][0], skip_special_tokens=True)
return response
# Example conversation
print(chat_with_bot("Hello, how are you?"))
In this example, a simple chatbot is created using the DialoGPT model from Hugging Face. The chatbot can engage in basic conversations by generating responses based on user input, demonstrating the application of machine learning in dialogue systems.
Addressing Challenges in Language Understanding
Handling Ambiguity and Context
One of the primary challenges in language understanding is handling ambiguity and context. Human language is inherently ambiguous, with words and phrases often having multiple meanings depending on the context. Machine learning models must be able to disambiguate these meanings to understand and generate accurate responses.
Contextual embeddings, such as those provided by models like BERT and GPT, have significantly improved the ability to handle ambiguity. These embeddings capture the context in which a word appears, allowing the model to disambiguate its meaning based on surrounding words. For example, the word "bank" can mean a financial institution or the side of a river, but a contextual embedding can determine the correct meaning based on the context in which it appears.
Streamlining Integration of ML Models: Easy Implementation with APIsAdditionally, attention mechanisms in transformer models help capture long-range dependencies in text, allowing the model to understand context over longer passages. This capability is crucial for tasks that require understanding complex sentences and paragraphs, such as reading comprehension and text summarization. By attending to relevant parts of the text, models can generate more accurate and coherent responses.
Ensuring Fairness and Reducing Bias
Ensuring fairness and reducing bias in language understanding models is a critical challenge. Machine learning models can inadvertently learn and perpetuate biases present in the training data, leading to unfair and discriminatory outcomes. Addressing these biases is essential to ensure that language models are fair and equitable.
Researchers have developed various techniques to mitigate bias in language models. One approach is data augmentation, where training data is enriched with diverse examples to reduce the model's reliance on biased patterns. Another technique is bias detection and correction, where models are evaluated for biased behavior, and corrective measures are applied to reduce the impact of bias.
Moreover, incorporating fairness constraints during training can help ensure that models treat different groups fairly. These constraints can be designed to promote equal performance across different demographic groups, ensuring that the model does not disproportionately favor or disadvantage any particular group. By actively addressing bias, researchers can develop more fair and inclusive language models.
Enhancing Credit Rating Accuracy through Machine LearningExample: Detecting Bias in Language Models Using Python
from transformers import pipeline
# Load sentiment analysis pipeline
sentiment_pipeline = pipeline("sentiment-analysis")
# Sample sentences to evaluate for bias
sentences = [
"The doctor is a man.",
"The doctor is a woman.",
"The nurse is a man.",
"The nurse is a woman."
]
# Analyze sentiment
results = sentiment_pipeline(sentences)
for sentence, result in zip(sentences, results):
print(f"Sentence: '{sentence}' - Sentiment: {result['label']}, Score: {result['score']:.2f}")
In this example, the Hugging Face sentiment analysis pipeline is used to evaluate potential bias in language models. By comparing the sentiment scores of sentences with different gender references, researchers can detect and address biases in the model's behavior.
Future Directions in Language Understanding
Multilingual Models and Cross-Lingual Transfer
The future of language understanding lies in developing multilingual models that can handle multiple languages with equal proficiency. Current models often require separate training for each language, which is resource-intensive and limits their applicability. Multilingual models, such as mBERT and XLM-R, are trained on diverse datasets across multiple languages, enabling cross-lingual transfer and improving performance on low-resource languages.
These models leverage cross-lingual transfer learning, where knowledge gained from high-resource languages is transferred to low-resource languages. This approach allows models to learn universal language representations that can be applied across different languages. As a result, multilingual models can achieve high performance even on languages with limited training data, making language technology more accessible and inclusive.
Furthermore, advancements in unsupervised learning techniques are pushing the boundaries of multilingual models. Unsupervised methods can leverage large corpora of text without requiring labeled data, allowing models to learn language representations from raw text. This capability is particularly valuable for languages with scarce annotated data, enabling the development of powerful language models for a wide range of languages.
Are Machine Learning Applications Too Complex for Cloud Deployment?Integration with Other AI Technologies
Integrating language understanding models with other AI technologies holds immense potential for creating more intelligent and versatile systems. For instance, combining NLP with computer vision can lead to applications such as image captioning, where models generate descriptive text based on visual inputs. This integration enhances the model's ability to understand and describe complex scenes, enabling applications in accessibility, content creation, and surveillance.
Another promising direction is the integration of NLP with knowledge graphs. Knowledge graphs represent information as a network of entities and relationships, providing a structured way to store and retrieve knowledge. By incorporating knowledge graphs into language models, systems can leverage structured information to improve their understanding and generation of text. This integration enables more accurate question answering, information retrieval, and decision support systems.
Moreover, the fusion of NLP with reinforcement learning can lead to more adaptive and interactive systems. Reinforcement learning allows models to learn from interactions and optimize their behavior based on feedback. By combining this capability with language understanding, systems can engage in more natural and goal-oriented conversations, improving user experience and effectiveness.
Example: Multimodal Image Captioning Using Python
import torch
from transformers import VisionEncoderDecoderModel, ViTFeatureExtractor, AutoTokenizer
# Load pre-trained model and feature extractor
model = VisionEncoderDecoderModel.from_pretrained("nlpconnect/vit-gpt2-image-captioning")
feature_extractor = ViTFeatureExtractor.from_pretrained("nlpconnect/vit-gpt2-image-captioning")
tokenizer = AutoTokenizer.from_pretrained("nlpconnect/vit-gpt2-image-captioning")
# Load image and preprocess
image = Image.open("example_image.jpg")
pixel_values = feature_extractor(images=image, return_tensors="pt").pixel_values
# Generate caption
outputs = model.generate(pixel_values)
caption = tokenizer.decode(outputs[0], skip_special_tokens=True)
print(f"Caption: {caption}")
In this example, a multimodal model combines vision and language capabilities to generate captions for images. The model leverages pre-trained components to understand visual inputs and produce descriptive text, demonstrating the integration of NLP and computer vision.
Machine learning has profoundly impacted language understanding, transforming text analysis, machine translation, and dialogue systems. Despite challenges such as handling ambiguity and ensuring fairness, advancements in multilingual models, integration with other AI technologies, and future research directions hold great promise. By continuing to innovate and address these challenges, machine learning will further enhance our ability to understand and interact with human language, driving progress across various domains.
If you want to read more articles similar to Machine Learning's Impact on Language Understanding, you can visit the Applications category.
You Must Read