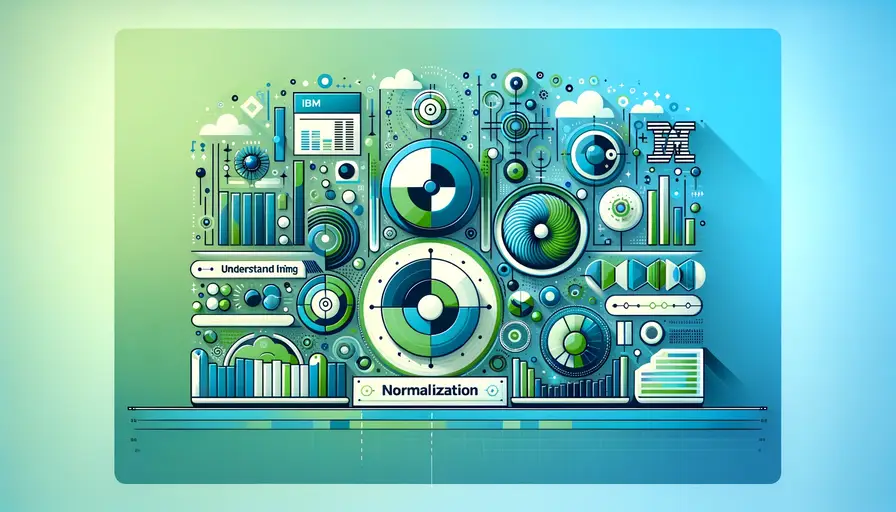
KNN Machine Learning in R: A Syntax Guide
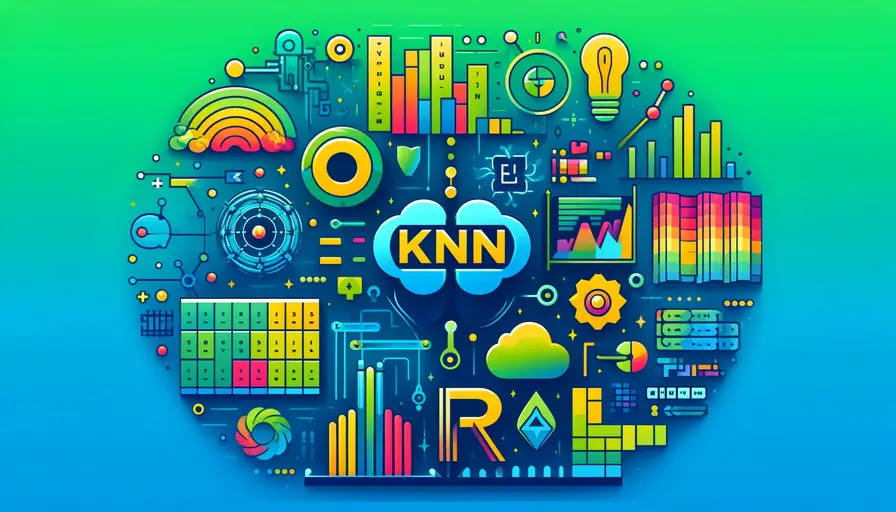
K-Nearest Neighbors (KNN)
K-Nearest Neighbors (KNN) is a simple, yet powerful, machine learning algorithm used for both classification and regression tasks. It works by finding the most similar data points (neighbors) and making predictions based on those points.
What is KNN?
K-Nearest Neighbors (KNN) is a non-parametric, lazy learning algorithm. It makes predictions by identifying the 'k' closest training examples in the feature space. KNN is widely used because of its simplicity and effectiveness.
How Does KNN Work?
KNN works by calculating the distance between the query instance and all the training samples. The most common distance metric used is Euclidean distance. The algorithm then selects the 'k' nearest data points and assigns the class based on the majority vote (for classification) or averages the values (for regression).
Example: Basic KNN in R
Here’s an example of implementing a basic KNN model using the class
package in R:

# Load necessary library
library(class)
# Load dataset
data(iris)
# Prepare training and testing data
set.seed(42)
trainIndex <- sample(1:nrow(iris), 0.7*nrow(iris))
trainData <- iris[trainIndex, ]
testData <- iris[-trainIndex, ]
# Train KNN model
knn_pred <- knn(train = trainData[, -5], test = testData[, -5], cl = trainData[, 5], k = 3)
# Evaluate the model
confusionMatrix <- table(knn_pred, testData$Species)
print(confusionMatrix)
Data Preparation for KNN
Proper data preparation is crucial for the performance of KNN. This involves handling missing values, scaling features, and encoding categorical variables.
Handling Missing Values
Handling missing values is essential to ensure the integrity of the dataset. Missing values can be imputed using various methods such as mean, median, or using more sophisticated algorithms.
Scaling Features
Since KNN relies on distance calculations, scaling features to the same range is crucial. Standardization or normalization techniques are commonly used to achieve this.
Example: Data Preparation in R
Here’s an example of preparing data for a KNN model using the caret
package in R:

# Load necessary library
library(caret)
# Load dataset
data(iris)
# Check for missing values
sum(is.na(iris))
# Scale features
preProcessRangeModel <- preProcess(iris[, -5], method = c("center", "scale"))
iris_scaled <- predict(preProcessRangeModel, iris[, -5])
# Combine scaled features with target variable
iris_prepared <- cbind(iris_scaled, Species = iris$Species)
Choosing the Value of 'k'
The choice of 'k' significantly impacts the performance of the KNN algorithm. The optimal value of 'k' can be determined through cross-validation.
Impact of 'k' on the Model
A small value of 'k' can lead to overfitting, while a large value of 'k' can smooth out the predictions too much, leading to underfitting. Balancing these two is crucial for optimal performance.
Cross-Validation for 'k'
Cross-validation involves splitting the data into training and testing sets multiple times and evaluating the performance for different values of 'k'. This helps in selecting the best 'k'.
Example: Finding Optimal 'k' in R
Here’s an example of using cross-validation to find the optimal value of 'k' in R:

# Load necessary library
library(class)
library(caret)
# Load dataset
data(iris)
# Prepare training and testing data
set.seed(42)
trainIndex <- sample(1:nrow(iris), 0.7*nrow(iris))
trainData <- iris[trainIndex, ]
testData <- iris[-trainIndex, ]
# Cross-validation to find optimal 'k'
accuracy <- sapply(1:10, function(k){
knn_pred <- knn(train = trainData[, -5], test = testData[, -5], cl = trainData[, 5], k = k)
confusionMatrix <- table(knn_pred, testData$Species)
sum(diag(confusionMatrix)) / sum(confusionMatrix)
})
# Optimal 'k'
optimal_k <- which.max(accuracy)
print(optimal_k)
KNN for Classification
KNN is widely used for classification tasks where the goal is to assign labels to new instances based on the majority class of their nearest neighbors.
Application of KNN in Classification
KNN is particularly effective for classification problems with a clear separation between classes. It is used in various domains such as image recognition, spam detection, and medical diagnosis.
Advantages of KNN in Classification
KNN is easy to understand and implement. It does not require any assumptions about the underlying data distribution. It also adapts well to dynamic and noisy data.
Example: KNN Classification in R
Here’s an example of applying KNN for classification in R:

# Load necessary library
library(class)
# Load dataset
data(iris)
# Prepare training and testing data
set.seed(42)
trainIndex <- sample(1:nrow(iris), 0.7*nrow(iris))
trainData <- iris[trainIndex, ]
testData <- iris[-trainIndex, ]
# Train KNN model
knn_pred <- knn(train = trainData[, -5], test = testData[, -5], cl = trainData[, 5], k = optimal_k)
# Evaluate the model
confusionMatrix <- table(knn_pred, testData$Species)
print(confusionMatrix)
KNN for Regression
KNN can also be used for regression tasks, where the goal is to predict a continuous target variable based on the average of the nearest neighbors.
Application of KNN in Regression
KNN regression is used in scenarios where the relationship between features and the target variable is complex and nonlinear. It is applied in areas like price prediction, environmental modeling, and economic forecasting.
Advantages of KNN in Regression
KNN regression is simple and intuitive. It can model complex relationships without the need for a predefined functional form. It is also robust to outliers in the data.
Example: KNN Regression in R
Here’s an example of applying KNN for regression in R:

# Load necessary library
library(FNN)
# Load dataset
data(airquality)
airquality <- na.omit(airquality)
# Prepare training and testing data
set.seed(42)
trainIndex <- sample(1:nrow(airquality), 0.7*nrow(airquality))
trainData <- airquality[trainIndex, ]
testData <- airquality[-trainIndex, ]
# Train KNN regression model
knn_reg <- knn.reg(train = trainData[, -1], test = testData[, -1], y = trainData$Ozone, k = optimal_k)
# Evaluate the model
mse <- mean((testData$Ozone - knn_reg$pred)^2)
print(mse)
Handling Imbalanced Data
Imbalanced data can negatively impact the performance of KNN. Techniques like resampling and using different distance metrics can help address this issue.
Resampling Techniques
Resampling techniques such as oversampling the minority class or undersampling the majority class can help balance the dataset. This improves the performance of KNN by ensuring that all classes are adequately represented.
Distance Metrics for Imbalanced Data
Using different distance metrics such as Manhattan or Minkowski can sometimes yield better results for imbalanced data compared to the traditional Euclidean distance.
Example: Handling Imbalanced Data in R
Here’s an example of handling imbalanced data using resampling in R:

# Load necessary library
library(ROSE)
# Load dataset
data(iris)
# Create an imbalanced dataset
iris_imbalanced <- iris[iris$Species != 'setosa', ]
iris_imbalanced$Species <- factor(iris_imbalanced$Species)
# Oversample the minority class
data_balanced <- ovun.sample(Species ~ ., data = iris_imbalanced, method = "over", N = 100)$data
# Train KNN model
knn_pred <- knn(train = data_balanced[, -5], test = iris_imbalanced[, -5], cl = data_balanced[, 5], k = optimal_k)
# Evaluate the model
confusionMatrix <- table(knn_pred, iris_imbalanced$Species)
print(confusionMatrix)
Advantages and Limitations of KNN
Understanding the advantages and limitations of KNN helps in determining when to use it and when to consider alternative models.
Advantages of KNN
K-Nearest Neighbors offers several advantages:
- Simplicity and ease of implementation.
- No need for training phase, which saves computational resources.
- Works well with small to medium-sized datasets.
Limitations of KNN
Despite its strengths, KNN also has limitations:
- High computational cost for large datasets due to the distance calculations.
- Sensitive to the choice of 'k' and distance metrics.
- Can be negatively impacted by irrelevant or redundant features.
Example: Comparing KNN with Other Models
Here’s an example of comparing KNN with other machine learning models in R:
# Load necessary library
library(caret)
# Load dataset
data(iris)
# Prepare training and testing data
set.seed(42)
trainIndex <- sample(1:nrow(iris), 0.7*nrow(iris))
trainData <- iris[trainIndex, ]
testData <- iris[-trainIndex, ]
# Train KNN model
knn_model <- train(Species ~ ., data = trainData, method = "knn", trControl = trainControl(method = "cv", number = 5))
# Train Random Forest model
rf_model <- train(Species ~ ., data = trainData, method = "rf", trControl = trainControl(method = "cv", number = 5))
# Compare models
resamples <- resamples(list(KNN = knn_model, RF = rf_model))
summary(resamples)
Practical Applications of KNN
KNN is widely used in various practical applications across different domains, from healthcare to finance and marketing.
Healthcare
In healthcare, KNN is used for disease diagnosis, predicting patient outcomes, and identifying risk factors. Its ability to handle complex and nonlinear relationships makes it suitable for medical data.
Finance
In finance, KNN is employed for credit scoring, stock price prediction, and fraud detection. Its simplicity and effectiveness in handling diverse datasets make it a valuable tool in financial analysis.
Marketing
KNN is used in marketing for customer segmentation, recommendation systems, and predicting customer behavior. It helps in understanding customer preferences and improving targeting strategies.
Example: KNN for Customer Segmentation in R
Here’s an example of using KNN for customer segmentation in R:
# Load necessary library
library(class)
# Load dataset
data(mtcars)
# Normalize data
preProcessRangeModel <- preProcess(mtcars, method = c("center", "scale"))
mtcars_scaled <- predict(preProcessRangeModel, mtcars)
# Perform KNN for clustering
set.seed(42)
kmeans_result <- kmeans(mtcars_scaled, centers = 3, nstart = 20)
# Add cluster assignments to data
mtcars$cluster <- kmeans_result$cluster
# Print the cluster centers
print(kmeans_result$centers)
Best Practices for Implementing KNN
Implementing KNN effectively requires following best practices such as proper data preparation, choosing the right distance metric, and tuning hyperparameters.
Data Preparation
Proper data preparation involves handling missing values, scaling features, and encoding categorical variables. This ensures that the KNN model performs well.
Choosing the Right Distance Metric
Choosing the appropriate distance metric (Euclidean, Manhattan, Minkowski) is crucial for capturing the underlying patterns in the data. Experimenting with different metrics helps in selecting the best one for the problem.
Hyperparameter Tuning
Tuning hyperparameters, such as the number of neighbors 'k', is essential for optimizing the model's performance. Techniques like cross-validation are commonly used for this purpose.
Example: Best Practices for KNN in R
Here’s an example of implementing best practices for KNN in R:
# Load necessary library
library(caret)
# Load dataset
data(iris)
# Preprocess data
preProcessRangeModel <- preProcess(iris[, -5], method = c("center", "scale"))
iris_scaled <- predict(preProcessRangeModel, iris[, -5])
# Combine scaled features with target variable
iris_prepared <- cbind(iris_scaled, Species = iris$Species)
# Define the tuning grid
tuneGrid <- expand.grid(k = 1:10)
# Train KNN model with tuning
control <- trainControl(method = "cv", number = 5)
model <- train(Species ~ ., data = iris_prepared, method = "knn", tuneGrid = tuneGrid, trControl = control)
# Print the best parameters and evaluate the model
print(model$bestTune)
print(model)
K-Nearest Neighbors (KNN) is a versatile and powerful algorithm in the machine learning toolkit. By understanding its principles, properly preparing data, and tuning hyperparameters, you can effectively leverage KNN for both classification and regression tasks. Whether using KNN for practical applications in healthcare, finance, or marketing, it remains a valuable method for achieving reliable and accurate results. By following best practices and using R's rich ecosystem of packages, implementing and optimizing KNN becomes a manageable and rewarding endeavor.
If you want to read more articles similar to KNN Machine Learning in R: A Syntax Guide, you can visit the Artificial Intelligence category.
You Must Read