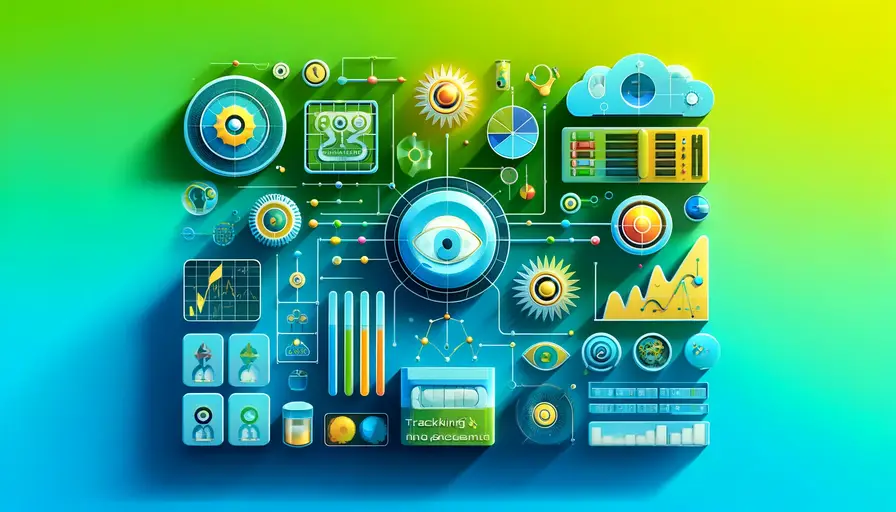
Saving and Loading Machine Learning Models in R

Model Persistence in R
Machine learning models require significant effort and computational resources to train. Once trained, it's crucial to save these models so they can be reused without retraining. This process is known as model persistence. In R, there are various methods to save and load models, ensuring efficiency and reproducibility in machine learning workflows.
Importance of Saving Machine Learning Models
Saving machine learning models is essential for multiple reasons. It allows for the reuse of models in different projects or phases of a project without the need to retrain them. This saves time and computational resources.
Applications of Model Persistence
Persisting models is useful in deploying machine learning models in production, conducting comparative studies, and ensuring reproducibility in research. It also aids in sharing models with others or across different environments.
Example: Model Persistence in R
Here’s an example of saving and loading a machine learning model in R:

# Load necessary library
library(randomForest)
# Load dataset
data(iris)
model <- randomForest(Species ~ ., data=iris)
# Save the model
saveRDS(model, file="random_forest_model.rds")
# Load the model
loaded_model <- readRDS("random_forest_model.rds")
# Predict using the loaded model
predictions <- predict(loaded_model, iris)
print(predictions)
Using Base R Functions for Model Persistence
R provides several base functions for saving and loading objects, including machine learning models. These functions are straightforward and efficient.
Saving Models with saveRDS()
The saveRDS()
function in R is used to save a single R object to a file. It is a preferred method for saving machine learning models due to its simplicity and effectiveness.
How to Use saveRDS()
Using saveRDS()
involves specifying the object to save and the file path where it should be stored. The function serializes the object, making it ready for storage.
Example: Saving a Model with saveRDS()
Here’s an example of saving a decision tree model using saveRDS()
:

# Load necessary library
library(rpart)
# Load dataset
data(iris)
model <- rpart(Species ~ ., data=iris)
# Save the model
saveRDS(model, file="decision_tree_model.rds")
Loading Models with readRDS()
The readRDS()
function is used to load a serialized R object from a file. It restores the object to its original form, allowing it to be used as if it were never saved.
How to Use readRDS()
Using readRDS()
involves specifying the file path of the saved object. The function deserializes the object, making it ready for use.
Example: Loading a Model with readRDS()
Here’s an example of loading a previously saved decision tree model using readRDS()
:
# Load the model
loaded_model <- readRDS("decision_tree_model.rds")
# Predict using the loaded model
predictions <- predict(loaded_model, iris, type="class")
print(predictions)
Using External Packages for Model Persistence
Several R packages provide additional functionalities for saving and loading machine learning models. These packages offer more flexibility and can handle complex workflows.

The caret Package
The caret
package is widely used for building and evaluating machine learning models. It also provides functions for saving and loading models.
Saving Models with caret
The save()
function in caret
allows saving multiple objects, including models and data, into a single file. This is useful for comprehensive project storage.
Example: Saving a Model with caret
Here’s an example of saving a model using the caret
package:
# Load necessary library
library(caret)
# Load dataset
data(iris)
model <- train(Species ~ ., data=iris, method="rf")
# Save the model
save(model, file="caret_model.RData")
Loading Models with caret
The load()
function in caret
loads objects from a file into the R environment. It restores all saved objects, making them ready for use.

Example: Loading a Model with caret
Here’s an example of loading a model using the caret
package:
# Load the model
load("caret_model.RData")
# Predict using the loaded model
predictions <- predict(model, iris)
print(predictions)
Using the mlr3 Package
The mlr3
package is another powerful package for machine learning in R. It offers advanced functionalities for model training, evaluation, and persistence.
Saving Models with mlr3
The mlr3
package uses the saveRDS()
and readRDS()
functions for model persistence. It also provides additional tools for managing machine learning workflows.
Example: Saving a Model with mlr3
Here’s an example of saving a model using the mlr3
package:

# Load necessary library
library(mlr3)
# Load dataset
task <- TaskClassif$new(id="iris", backend=iris, target="Species")
learner <- lrn("classif.rpart")
# Train model
learner$train(task)
# Save the model
saveRDS(learner, file="mlr3_model.rds")
Loading Models with mlr3
Models saved with mlr3
can be loaded using readRDS()
, similar to base R functions. This ensures compatibility and ease of use.
Example: Loading a Model with mlr3
Here’s an example of loading a model using the mlr3
package:
# Load the model
loaded_model <- readRDS("mlr3_model.rds")
# Predict using the loaded model
predictions <- loaded_model$predict(task)
print(predictions)
Advanced Techniques for Model Persistence
Advanced techniques for model persistence involve handling complex workflows and ensuring models can be deployed across different environments seamlessly.
Saving and Loading Multiple Models
In many projects, multiple models are used. Saving and loading these models efficiently requires careful management of model objects and file paths.

Example: Saving Multiple Models
Here’s an example of saving multiple models using save()
:
# Load necessary library
library(caret)
# Load dataset
data(iris)
model1 <- train(Species ~ ., data=iris, method="rf")
model2 <- train(Species ~ ., data=iris, method="rpart")
# Save the models
save(model1, model2, file="multiple_models.RData")
Loading Multiple Models
Loading multiple models involves using the load()
function to restore all saved objects simultaneously, ensuring they are ready for use.
Example: Loading Multiple Models
Here’s an example of loading multiple models:
# Load the models
load("multiple_models.RData")
# Predict using the loaded models
predictions1 <- predict(model1, iris)
predictions2 <- predict(model2, iris)
print(predictions1)
print(predictions2)
Version Control for Models
Version control is essential for tracking changes in models and ensuring reproducibility. This can be achieved using tools like git
and model versioning techniques.
Example: Using git for Model Versioning
Here’s an example of using git
to version control machine learning models:
# Initialize a git repository
git init
# Add model files
git add random_forest_model.rds decision_tree_model.rds
# Commit the changes
git commit -m "Initial commit with saved models"
Best Practices for Model Persistence
Adopting best practices for model persistence ensures efficiency, reproducibility, and seamless integration into production workflows.
Regularly Save Models During Training
Regularly saving models during training helps in recovering from unexpected interruptions and tracking model progress. This practice is especially useful in long training sessions.
Example: Saving Models at Checkpoints
Here’s an example of saving models at checkpoints:
# Load necessary library
library(randomForest)
# Load dataset
data(iris)
model <- randomForest(Species ~ ., data=iris)
# Save the model at a checkpoint
saveRDS(model, file="random_forest_checkpoint.rds")
Documenting Model Metadata
Documenting model metadata, such as training parameters and data preprocessing steps, ensures that models can be accurately reproduced and understood.
Example: Saving Model Metadata
Here’s an example of saving model metadata:
# Load necessary library
library(randomForest)
# Load dataset
data(iris)
model <- randomForest(Species ~ ., data=iris)
# Save the model and metadata
metadata <- list(model_type="randomForest", data_used="iris", date=Sys.Date())
saveRDS(list(model=model, metadata=metadata), file="model_with_metadata.rds")
Ensuring Compatibility Across Environments
Ensuring that saved models can be loaded and used across different environments involves considering dependencies and software versions.
Example: Checking Package Versions
Here’s an example of checking and recording package versions:
# Check package versions
package_versions <- sessionInfo()$otherPkgs
# Save package versions
saveRDS(package_versions, file="package_versions.rds")
Case Studies and Real-World Applications
Exploring case studies and real-world applications demonstrates the practical importance and implementation of model persistence in various domains.
Case Study: Healthcare Predictive Models
In healthcare, predictive models are used to forecast patient outcomes and disease progression. Saving and loading these models ensures they can be reused for patient monitoring and new patient data.
Example: Healthcare Predictive Model
Here’s an example of saving and loading a healthcare predictive model:
# Load necessary library
library(caret)
# Load dataset
data(iris)
model <- train(Species ~ ., data=iris, method="rf")
# Save the model
saveRDS(model, file="healthcare_model.rds")
# Load the model
loaded_model <- readRDS("healthcare_model.rds")
# Predict using the loaded model
predictions <- predict(loaded_model, iris)
print(predictions)
Case Study: Financial Risk Models
In finance, risk models are used to predict market risks and investment outcomes. Persisting these models allows for continuous monitoring and adjustment based on new data.
Example: Financial Risk Model
Here’s an example of saving and loading a financial risk model:
# Load necessary library
library(randomForest)
# Load dataset
data(iris)
model <- randomForest(Species ~ ., data=iris)
# Save the model
saveRDS(model, file="financial_risk_model.rds")
# Load the model
loaded_model <- readRDS("financial_risk_model.rds")
# Predict using the loaded model
predictions <- predict(loaded_model, iris)
print(predictions)
Case Study: Retail Demand Forecasting
In retail, demand forecasting models predict product demand based on historical sales data. Saving these models ensures they can be updated with new data and reused for future forecasts.
Example: Retail Demand Forecasting Model
Here’s an example of saving and loading a retail demand forecasting model:
# Load necessary library
library(caret)
# Load dataset
data(iris)
model <- train(Species ~ ., data=iris, method="rf")
# Save the model
saveRDS(model, file="demand_forecasting_model.rds")
# Load the model
loaded_model <- readRDS("demand_forecasting_model.rds")
# Predict using the loaded model
predictions <- predict(loaded_model, iris)
print(predictions)
Saving and loading machine learning models in R is a critical practice that ensures efficiency, reproducibility, and seamless integration into production workflows. By understanding and implementing the techniques and best practices discussed in this guide, data scientists and analysts can effectively manage their models and drive impactful insights across various domains. Whether using base R functions or leveraging powerful packages like caret
and mlr3
, the ability to persist models is a foundational skill in the machine learning toolkit.
If you want to read more articles similar to Saving and Loading Machine Learning Models in R, you can visit the Tools category.
You Must Read