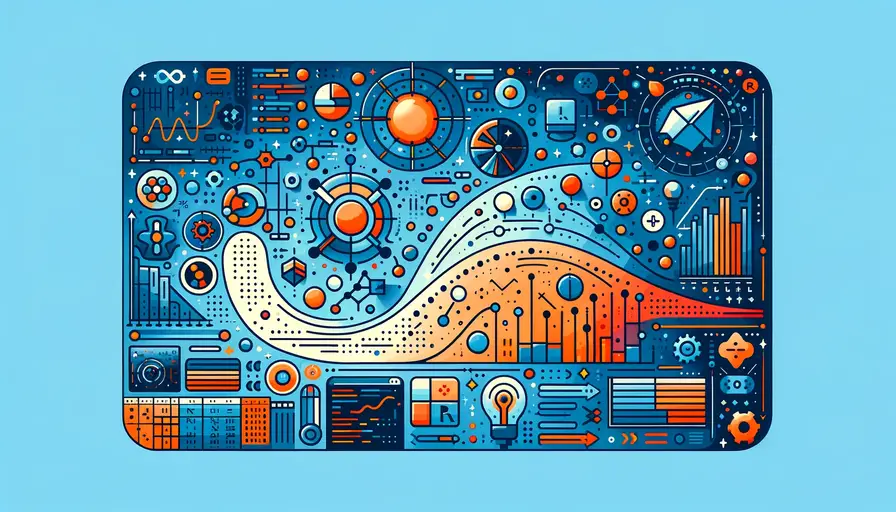
Using Machine Learning to Detect and Predict DDoS Attacks

Importance of Addressing DDoS Attacks
Growing Threat of Cybersecurity Breaches
Distributed Denial of Service (DDoS) attacks have become a prevalent threat in the digital age, disrupting online services by overwhelming networks, servers, and applications with massive amounts of traffic. These attacks can incapacitate websites, online services, and even entire networks, causing significant financial and reputational damage. With the increasing reliance on digital infrastructure, the frequency and sophistication of DDoS attacks have escalated, making robust detection and mitigation strategies critical.
Cybersecurity experts emphasize the need for proactive measures to counteract these threats. Traditional defense mechanisms, such as firewalls and intrusion detection systems, often struggle to cope with the sheer volume and complexity of modern DDoS attacks. This challenge has led to the exploration of advanced technologies like machine learning, which can provide more effective and adaptive solutions for detecting and predicting these attacks.
Machine learning models can analyze large volumes of network traffic data in real-time, identifying patterns and anomalies that indicate potential DDoS attacks. By leveraging historical data and continuously learning from new threats, these models can enhance the ability to detect and mitigate DDoS attacks, reducing the impact on targeted systems and networks.
Financial and Operational Impact
The financial and operational impact of DDoS attacks is substantial. Organizations of all sizes, from small businesses to large enterprises, can suffer significant losses due to service downtime, lost revenue, and the costs associated with mitigating the attacks and restoring services. Additionally, the reputational damage resulting from a successful DDoS attack can lead to a loss of customer trust and long-term business consequences.

For example, high-profile DDoS attacks on major websites and online services have resulted in millions of dollars in losses. E-commerce platforms can lose sales during peak shopping periods, and financial institutions may face disruptions in online banking services, affecting customer transactions and trust. The operational impact extends beyond immediate financial losses, as IT teams must allocate resources to address the attack, diverting attention from other critical tasks.
Machine learning can play a crucial role in minimizing these impacts by providing early detection and automated responses to DDoS attacks. By swiftly identifying malicious traffic patterns and triggering mitigation actions, machine learning models can help maintain service availability and protect organizations from significant financial and operational disruptions.
Regulatory and Compliance Requirements
In addition to the financial and operational implications, organizations must also consider regulatory and compliance requirements related to cybersecurity. Many industries, such as finance, healthcare, and critical infrastructure, are subject to stringent regulations that mandate robust security measures to protect sensitive data and ensure the availability of critical services.
Regulatory bodies often require organizations to implement proactive security measures, including the detection and mitigation of DDoS attacks. Failure to comply with these regulations can result in hefty fines, legal liabilities, and further reputational damage. Machine learning-based DDoS detection systems can help organizations meet these compliance requirements by providing advanced threat detection and response capabilities.

For instance, the General Data Protection Regulation (GDPR) in the European Union mandates that organizations take appropriate measures to protect personal data and ensure the resilience of their systems. Similarly, the Health Insurance Portability and Accountability Act (HIPAA) in the United States requires healthcare providers to implement safeguards to protect patient data. By leveraging machine learning for DDoS detection, organizations can enhance their cybersecurity posture and ensure compliance with relevant regulations.
Machine Learning Techniques for DDoS Detection
Supervised Learning for Anomaly Detection
Supervised learning is a widely used machine learning technique for DDoS detection, where models are trained on labeled datasets containing both normal and malicious traffic patterns. By learning the characteristics of each class, these models can effectively identify anomalies that may indicate a DDoS attack.
One common approach is to use classification algorithms such as decision trees, support vector machines (SVM), and neural networks. These algorithms can distinguish between legitimate and malicious traffic based on various features, such as packet size, traffic volume, and connection duration. The trained models can then be deployed to monitor network traffic in real-time, flagging any suspicious activity for further investigation.
For example, a decision tree classifier can be trained on a dataset of network traffic, with features like packet size and flow duration labeled as either normal or malicious. Once trained, the model can classify incoming traffic based on these features, alerting security teams to potential DDoS attacks.

Example: Using Decision Tree Classifier for DDoS Detection in Python
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
from sklearn.metrics import accuracy_score, classification_report
# Load dataset
data = pd.read_csv('network_traffic.csv')
X = data.drop('label', axis=1)
y = data['label']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Decision Tree classifier
model = DecisionTreeClassifier(random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model performance
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
print(classification_report(y_test, y_pred))
In this example, a Decision Tree classifier is trained to detect DDoS attacks based on network traffic features. The model's performance is evaluated using accuracy and a classification report, demonstrating its effectiveness in identifying malicious traffic.
Unsupervised Learning for Clustering
Unsupervised learning techniques, such as clustering, can also be employed for DDoS detection, especially in scenarios where labeled data is scarce. Clustering algorithms group similar data points together, allowing the identification of patterns and anomalies without prior knowledge of what constitutes normal or malicious traffic.
One popular clustering algorithm is k-means, which partitions the data into k clusters based on the similarity of their features. By analyzing the clusters, security teams can identify unusual traffic patterns that deviate from the norm, potentially indicating a DDoS attack. This approach is particularly useful for detecting novel attack patterns that were not seen during the training phase.
Another effective clustering algorithm is DBSCAN (Density-Based Spatial Clustering of Applications with Noise), which identifies clusters based on the density of data points. DBSCAN is advantageous for DDoS detection because it can discover clusters of arbitrary shapes and handle noise, making it robust against the varied nature of network traffic.

Example: Using K-Means Clustering for Anomaly Detection in Python
import pandas as pd
from sklearn.preprocessing import StandardScaler
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
# Load dataset
data = pd.read_csv('network_traffic.csv')
X = data.drop('label', axis=1)
# Standardize features
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# Train K-Means clustering model
kmeans = KMeans(n_clusters=2, random_state=42)
kmeans.fit(X_scaled)
# Predict cluster labels
data['cluster'] = kmeans.labels_
# Plot clusters
plt.scatter(X_scaled[:, 0], X_scaled[:, 1], c=data['cluster'], cmap='viridis')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.title('K-Means Clustering of Network Traffic')
plt.show()
In this example, K-Means Clustering is used to identify anomalies in network traffic. The clusters are visualized to highlight potential outliers that may indicate DDoS attacks.
Deep Learning for Complex Patterns
Deep learning techniques, particularly convolutional neural networks (CNNs) and recurrent neural networks (RNNs), have shown great promise in detecting complex patterns in network traffic data. These models can capture intricate relationships and temporal dependencies in the data, making them well-suited for identifying sophisticated DDoS attacks.
CNNs can be used to analyze network traffic by treating the data as a multi-dimensional array, similar to how images are processed. By applying convolutional filters, CNNs can detect spatial patterns in the traffic data, such as sudden spikes in packet volume or unusual packet distributions, which may indicate a DDoS attack.
RNNs, particularly long short-term memory (LSTM) networks, are effective for analyzing sequential data and capturing temporal dependencies. LSTM networks can learn from historical traffic patterns and predict future anomalies, providing early warning of potential DDoS attacks. By continuously monitoring network traffic, LSTM models can adapt to evolving attack patterns and improve detection accuracy over time.

Example: Using LSTM for DDoS Detection in Python
import numpy as np
import pandas as pd
from sklearn.preprocessing import MinMaxScaler
from keras.models import Sequential
from keras.layers import LSTM, Dense
# Load dataset
data = pd.read_csv('network_traffic.csv')
scaler = MinMaxScaler(feature_range=(0, 1))
scaled_data = scaler.fit_transform(data.drop('label', axis=1))
# Create sequences for training
def create_sequences(data, seq_length):
X, y = [], []
for i in range(len(data) - seq_length):
X.append(data[i:i + seq_length])
y.append(data[i + seq_length][-1]) # Assuming label is the last column
return np.array(X), np.array(y)
seq_length = 60
X, y = create_sequences(scaled_data, seq_length)
X_train, y_train = X[:-1000], y[:-1000]
X_test, y_test = X[-1000:], y[-1000:]
# Build and train LSTM model
model = Sequential()
model.add(LSTM(50, return_sequences=True, input_shape=(seq_length, X_train.shape[2])))
model.add(LSTM(50))
model.add(Dense(1, activation='sigmoid'))
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
model.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_test, y_test))
# Evaluate model performance
loss, accuracy = model.evaluate(X_test, y_test)
print(f'Loss: {loss}, Accuracy: {accuracy}')
In this example, an LSTM model is used to detect DDoS attacks based on sequential network traffic data, showcasing the application of deep learning for complex pattern recognition.
Implementing Real-Time DDoS Detection Systems
Integrating Machine Learning with Network Security Tools
To implement a robust real-time DDoS detection system, it is essential to integrate machine learning models with existing network security tools. This integration allows for seamless data collection, analysis, and response to potential threats. Security tools such as firewalls, intrusion detection systems (IDS), and security information and event management (SIEM) systems can be augmented with machine learning capabilities to enhance their effectiveness.
For example, a SIEM system can collect and aggregate network traffic data from various sources, including firewalls, IDS, and servers. By integrating machine learning models, the SIEM system can analyze this data in real-time, identifying patterns and anomalies that may indicate a DDoS attack. This enables security teams to respond quickly and effectively to mitigate the impact of the attack.
Furthermore, machine learning models can be deployed at the network edge, where they can analyze traffic at entry points to the network. This approach allows for early detection of DDoS attacks and prevents malicious traffic from reaching critical network infrastructure. Edge computing platforms and network appliances equipped with machine learning capabilities can provide an additional layer of security and resilience.

Example: Integrating Machine Learning with SIEM in Python
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
import json
import requests
# Load dataset
data = pd.read_csv('network_traffic.csv')
X = data.drop('label', axis=1)
y = data['label']
# Train a Random Forest classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X, y)
# Function to send alerts to SIEM system
def send_alert(alert):
url = 'http://siem-system/alert'
headers = {'Content-Type': 'application/json'}
response = requests.post(url, data=json.dumps(alert), headers=headers)
return response.status_code
# Real-time traffic monitoring
def monitor_traffic(new_traffic):
prediction = model.predict([new_traffic])
if prediction[0] == 1: # Assuming 1 indicates a DDoS attack
alert = {'message': 'DDoS attack detected', 'traffic_data': new_traffic}
send_alert(alert)
# Example new traffic data
new_traffic = [0.1, 0.2, 0.3, 0.4, 0.5]
monitor_traffic(new_traffic)
In this example, a Random Forest classifier is trained to detect DDoS attacks, and a function is implemented to send alerts to a SIEM system when an attack is detected, demonstrating the integration of machine learning with network security tools.
Continuous Model Training and Adaptation
To ensure the effectiveness of machine learning-based DDoS detection systems, it is crucial to implement continuous model training and adaptation. As cyber threats evolve, new attack patterns emerge, and the network environment changes, machine learning models must be updated regularly to maintain their accuracy and reliability.
Continuous model training involves collecting new data, retraining models, and deploying updated models in the production environment. This process can be automated using tools such as Kaggle, which provides access to large datasets and machine learning competitions, and Google Cloud AI Platform, which offers scalable machine learning services for training and deploying models.
Moreover, techniques such as online learning and transfer learning can be employed to adapt models to new data more efficiently. Online learning allows models to update incrementally as new data arrives, while transfer learning leverages pre-trained models to quickly adapt to new tasks with limited additional training.
Example: Automating Continuous Model Training in Python
import pandas as pd
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
import joblib
# Function to train and save model
def train_model(data_path):
data = pd.read_csv(data_path)
X = data.drop('label', axis=1)
y = data['label']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LogisticRegression(max_iter=1000)
model.fit(X_train, y_train)
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
joblib.dump(model, 'ddos_model.pkl')
# Function to load and predict with model
def predict(new_data):
model = joblib.load('ddos_model.pkl')
prediction = model.predict([new_data])
return prediction
# Train model with new data
train_model('new_network_traffic.csv')
# Predict with updated model
new_traffic = [0.1, 0.2, 0.3, 0.4, 0.5]
prediction = predict(new_traffic)
print(f'Prediction: {prediction}')
In this example, a Logistic Regression model is trained and saved for future use. The model is updated with new data, ensuring continuous adaptation to evolving threats.
Collaboration and Threat Intelligence Sharing
Collaboration and threat intelligence sharing are essential components of an effective DDoS detection strategy. By sharing information about emerging threats and attack patterns, organizations can enhance their collective ability to detect and respond to DDoS attacks. Threat intelligence platforms and industry consortia, such as the Information Sharing and Analysis Centers (ISACs), facilitate this collaboration.
Machine learning models can benefit from threat intelligence data by incorporating new threat indicators and attack signatures into their training datasets. This enables models to detect the latest attack vectors and tactics used by cybercriminals. Organizations can also share anonymized attack data and model performance metrics to help improve the overall effectiveness of DDoS detection systems.
For instance, threat intelligence feeds can provide real-time updates on known malicious IP addresses, domain names, and attack patterns. By integrating these feeds into machine learning models, organizations can enhance their ability to detect and block malicious traffic before it causes significant harm. Collaborative efforts and information sharing can create a more resilient cybersecurity ecosystem, where organizations work together to combat the growing threat of DDoS attacks.
Using machine learning to detect and predict DDoS attacks offers a powerful and adaptive approach to cybersecurity. By leveraging supervised and unsupervised learning techniques, integrating machine learning with network security tools, implementing continuous model training, and fostering collaboration through threat intelligence sharing, organizations can significantly enhance their ability to protect against DDoS attacks. As the threat landscape continues to evolve, machine learning will play an increasingly vital role in ensuring the security and resilience of digital infrastructure.
If you want to read more articles similar to Using Machine Learning to Detect and Predict DDoS Attacks, you can visit the Applications category.
You Must Read