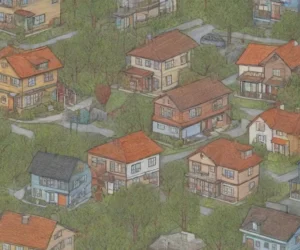
Can Machine Learning Classification Be Validated for Accuracy?
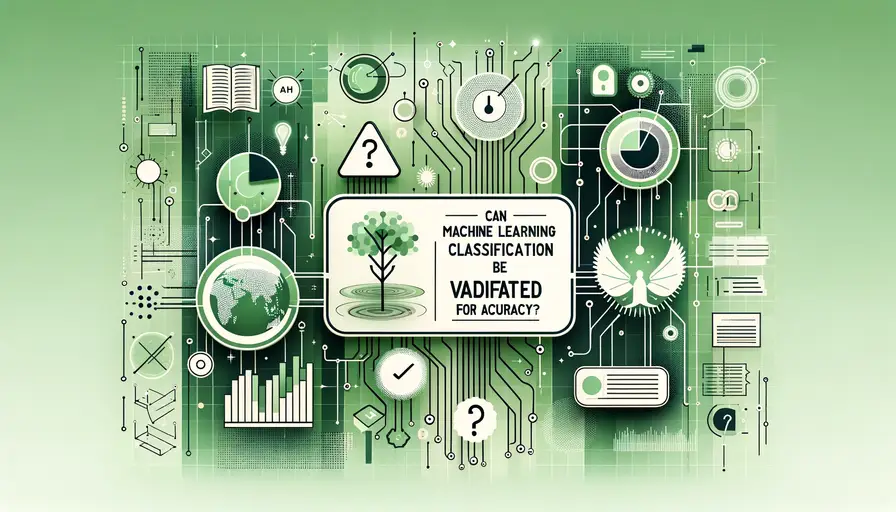
Validating machine learning classification models for accuracy is crucial to ensure that they perform well on unseen data. Various validation techniques and evaluation metrics are used to assess model performance, prevent overfitting, and ensure generalizability. This guide explores the different methods for validating machine learning classification models.
Types of Validation
Validation is the process of evaluating the performance of a machine learning model. There are several types of validation techniques, each with its advantages and limitations. These techniques help in understanding how well a model will generalize to new, unseen data.
The primary goal of validation is to provide an unbiased estimate of model performance. By using validation techniques, we can detect issues like overfitting, where the model performs well on the training data but poorly on the test data. Effective validation ensures that the model will be reliable when deployed in real-world scenarios.
Evaluation Metrics
Evaluation metrics are critical for assessing the accuracy and performance of classification models. Different metrics provide insights into various aspects of model performance, such as precision, recall, and the trade-off between them.
Key Evaluation Scenarios for Machine Learning ModelsCommon evaluation metrics for classification models include accuracy, precision, recall, F1 score, and the area under the ROC curve (AUC-ROC). Each metric has its strengths and weaknesses, and the choice of metric depends on the specific problem and goals of the model.
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
# Example of calculating evaluation metrics
y_true = [0, 1, 1, 0, 1, 0]
y_pred = [0, 1, 0, 0, 1, 1]
accuracy = accuracy_score(y_true, y_pred)
precision = precision_score(y_true, y_pred)
recall = recall_score(y_true, y_pred)
f1 = f1_score(y_true, y_pred)
print(f'Accuracy: {accuracy}, Precision: {precision}, Recall: {recall}, F1 Score: {f1}')
Cross-validation Techniques
Cross-validation is a robust technique for assessing the performance of machine learning models. It involves partitioning the data into multiple subsets and training the model on different combinations of these subsets to ensure reliable performance estimates.
K-Fold Cross-Validation
K-fold cross-validation involves dividing the dataset into k
equally sized folds. The model is trained on k-1
folds and validated on the remaining fold. This process is repeated k
times, with each fold serving as the validation set once. The final performance metric is the average of the individual fold results.
The main advantage of K-fold cross-validation is that it provides a more accurate estimate of model performance compared to a single train-test split. It ensures that each data point is used for both training and validation, reducing the risk of bias in performance estimates.
Evaluating the Accuracy Score of Your Machine Learning ModelStratified Cross-Validation
Stratified cross-validation is a variation of K-fold cross-validation that ensures each fold has a similar distribution of classes as the original dataset. This technique is particularly useful for imbalanced datasets, where certain classes are underrepresented.
By preserving the class distribution, stratified cross-validation provides a more realistic evaluation of model performance, especially for classification problems where maintaining class balance is crucial.
Leave-One-Out Cross-Validation
Leave-one-out cross-validation (LOOCV) is an extreme form of cross-validation where k
equals the number of data points in the dataset. In LOOCV, the model is trained on all data points except one, which is used for validation. This process is repeated for each data point.
LOOCV provides an unbiased estimate of model performance but can be computationally expensive for large datasets. It is most useful for small datasets where other cross-validation techniques may not be feasible.
Determining the Optimal Sample Size for Machine Learning ModelsHoldout Validation
Holdout validation involves splitting the dataset into two parts: a training set and a validation set. The model is trained on the training set and evaluated on the validation set to estimate its performance.
Advantages of Holdout Validation
The main advantage of holdout validation is its simplicity and speed. It is straightforward to implement and computationally efficient, making it suitable for large datasets or preliminary model evaluation.
Limitations of Holdout Validation
However, holdout validation has limitations. It provides a single estimate of model performance, which can be sensitive to the specific split of data. This approach may lead to overfitting or underfitting if the training and validation sets are not representative of the overall data distribution.
Why is Using a Separate Test Set Important?
Using a separate test set is crucial for unbiased model evaluation. The test set should only be used once for final model validation, ensuring that performance estimates are not influenced by the training process.
Are Machine Learning Models Statistically Valid?How to Use a Separate Test Set for Validation
To use a separate test set for validation, split the dataset into three parts: training, validation, and test sets. The model is trained on the training set, hyperparameters are tuned on the validation set, and final performance is assessed on the test set.
from sklearn.model_selection import train_test_split
# Splitting data into training, validation, and test sets
X_train, X_temp, y_train, y_temp = train_test_split(X, y, test_size=0.4, random_state=42)
X_val, X_test, y_val, y_test = train_test_split(X_temp, y_temp, test_size=0.5, random_state=42)
# Train and validate the model
model.fit(X_train, y_train)
val_predictions = model.predict(X_val)
print(f'Validation Accuracy: {accuracy_score(y_val, val_predictions)}')
# Test the model
test_predictions = model.predict(X_test)
print(f'Test Accuracy: {accuracy_score(y_test, test_predictions)}')
Stratified Sampling
Stratified sampling is a technique used to ensure that each class is proportionally represented in the training and validation sets. This approach is particularly useful for imbalanced datasets.
How Does Stratified Sampling Work?
Stratified sampling works by dividing the data into strata based on class labels and then sampling proportionally from each stratum. This ensures that the training and validation sets have a similar distribution of classes, leading to more reliable model performance estimates.
Benefits of Stratified Sampling
The benefits of stratified sampling include improved representation of minority classes, reduced bias in performance estimates, and better generalization of the model. This technique helps in providing a more accurate and realistic evaluation of model performance, especially for classification tasks.
The Purpose of ROC Curve in Machine LearningValidating machine learning classification models is essential for ensuring their accuracy and reliability. Various validation techniques, such as cross-validation, holdout validation, and stratified sampling, provide robust methods for assessing model performance. By using appropriate evaluation metrics and following best practices, practitioners can develop models that generalize well to new data and deliver accurate predictions.
If you want to read more articles similar to Can Machine Learning Classification Be Validated for Accuracy?, you can visit the Performance category.
You Must Read