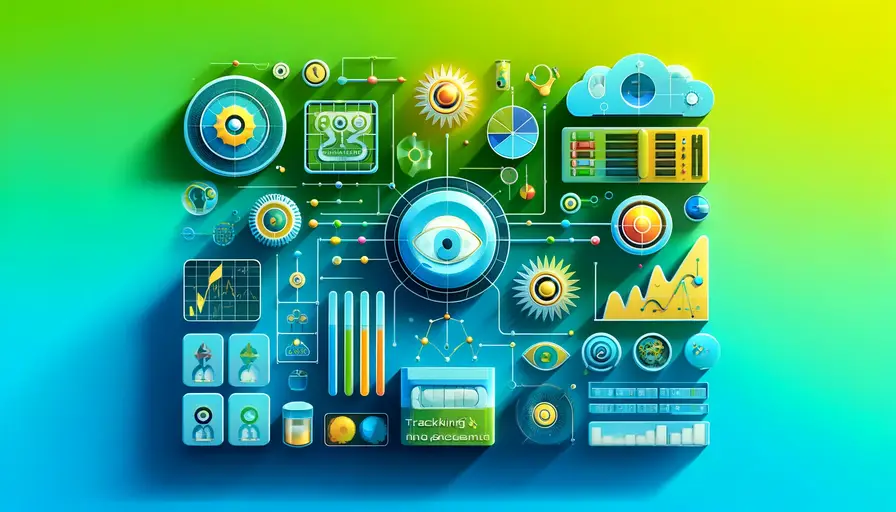
Data Pipeline and ML Implementation Best Practices in Python

In the world of machine learning (ML), having a well-structured data pipeline is crucial for ensuring efficient, reproducible, and scalable model development. A robust data pipeline manages the flow of data from raw input to final output, integrating processes such as data ingestion, preprocessing, feature engineering, model training, and evaluation. This article explores the best practices for implementing data pipelines and machine learning models in Python, emphasizing the importance of each step and providing practical examples.
Designing Efficient Data Pipelines
Importance of Data Pipelines
Data pipelines are essential for automating the data preparation process, ensuring that data flows seamlessly from one stage to another. They help in managing large volumes of data, reducing manual errors, and improving consistency. A well-designed data pipeline also facilitates data versioning and tracking, making it easier to replicate and audit the entire process.
Efficient data pipelines are critical for handling big data and real-time data processing. They enable organizations to process data at scale, ensuring that the data is always up-to-date and available for analysis. This is particularly important for applications such as streaming analytics and real-time ML models, where timely data processing is crucial for making informed decisions.
By automating data processing tasks, data pipelines free up valuable time for data scientists and engineers, allowing them to focus on more strategic activities such as model development and optimization. This improves overall productivity and accelerates the time-to-market for ML solutions.

Components of a Data Pipeline
A typical data pipeline consists of several key components, each responsible for a specific aspect of data processing. These components include data ingestion, data cleaning, data transformation, feature engineering, and data storage. Each component plays a vital role in ensuring that the data is processed efficiently and accurately.
Data ingestion involves collecting data from various sources such as databases, APIs, or flat files. This data is then cleaned and preprocessed to remove any inconsistencies or errors. Data cleaning may involve tasks such as handling missing values, removing duplicates, and correcting data types.
Data transformation involves converting the data into a suitable format for analysis. This may include tasks such as normalizing numerical features, encoding categorical variables, and creating new features through feature engineering. Finally, the processed data is stored in a data warehouse or data lake, where it can be accessed for model training and evaluation.
Here is an example of a simple data pipeline using Pandas and Scikit-learn in Python:

import pandas as pd
from sklearn.preprocessing import StandardScaler, OneHotEncoder
from sklearn.compose import ColumnTransformer
from sklearn.pipeline import Pipeline
# Load data
data = pd.read_csv('data.csv')
# Define numerical and categorical columns
numerical_features = ['age', 'income']
categorical_features = ['gender', 'occupation']
# Define data preprocessing steps
numerical_transformer = Pipeline(steps=[
('scaler', StandardScaler())
])
categorical_transformer = Pipeline(steps=[
('onehot', OneHotEncoder(handle_unknown='ignore'))
])
# Combine preprocessing steps into a single ColumnTransformer
preprocessor = ColumnTransformer(transformers=[
('num', numerical_transformer, numerical_features),
('cat', categorical_transformer, categorical_features)
])
# Fit and transform the data
data_preprocessed = preprocessor.fit_transform(data)
print("Preprocessed Data:\n", data_preprocessed)
This example demonstrates a simple data pipeline that includes data ingestion, cleaning, and transformation using Pandas and Scikit-learn.
Ensuring Scalability and Efficiency
To ensure scalability and efficiency, it is essential to design data pipelines that can handle large volumes of data and scale horizontally. This involves using distributed computing frameworks such as Apache Spark or Dask, which enable parallel processing and efficient data management.
Scalable data pipelines are crucial for organizations dealing with big data and real-time analytics. They ensure that the data processing tasks are distributed across multiple nodes, reducing the time required for data processing and improving overall system performance.
Efficiency can also be improved by optimizing data storage and retrieval mechanisms. This includes using efficient file formats such as Parquet or Avro, which provide better compression and faster read/write speeds compared to traditional formats such as CSV.

Here is an example of using Dask for scalable data processing:
import dask.dataframe as dd
# Load data using Dask
data = dd.read_csv('large_data.csv')
# Define data preprocessing steps
def preprocess(df):
df['income'] = df['income'].fillna(df['income'].mean())
df['age'] = (df['age'] - df['age'].mean()) / df['age'].std()
df = pd.get_dummies(df, columns=['gender', 'occupation'], drop_first=True)
return df
# Apply preprocessing to the Dask DataFrame
data_preprocessed = data.map_partitions(preprocess)
# Compute the result
data_preprocessed = data_preprocessed.compute()
print("Preprocessed Data:\n", data_preprocessed)
This example demonstrates how to use Dask for scalable data processing, ensuring that the data pipeline can handle large volumes of data efficiently.
Implementing Machine Learning Models
Selecting the Right Model
Choosing the right machine learning model is critical for achieving optimal performance. The choice of model depends on various factors such as the nature of the problem, the size and complexity of the dataset, and the computational resources available. Common types of models include linear regression, decision trees, support vector machines (SVM), and neural networks.
Each model has its strengths and weaknesses, and it is essential to evaluate multiple models to identify the best one for a given problem. Linear models, such as linear regression and logistic regression, are simple and interpretable but may not capture complex relationships in the data. Decision trees and ensemble methods, such as random forests and gradient boosting, are powerful and can handle non-linear relationships but may be prone to overfitting.

Neural networks and deep learning models are suitable for complex tasks such as image recognition and natural language processing but require large amounts of data and computational resources. It is essential to consider these trade-offs when selecting a model.
Here is an example of selecting and training a random forest classifier using Scikit-learn:
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load data
data = pd.read_csv('data.csv')
X = data.drop('target', axis=1)
y = data['target']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize and train the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Model Accuracy: {accuracy}")
This example demonstrates how to select and train a random forest classifier, emphasizing the importance of model selection.
Hyperparameter Tuning
Hyperparameter tuning is a critical step in optimizing the performance of machine learning models. Hyperparameters are parameters that are not learned during training but are set before the training process begins. Examples include the learning rate, the number of trees in a random forest, and the number of layers in a neural network.

Tuning hyperparameters involves searching for the optimal combination of hyperparameters that maximize the model's performance. Common techniques for hyperparameter tuning include grid search, random search, and Bayesian optimization.
Grid search involves evaluating the model for every possible combination of hyperparameters in a predefined grid. Random search randomly selects hyperparameter combinations to evaluate, providing a more efficient alternative to grid search. Bayesian optimization uses probabilistic models to guide the search for optimal hyperparameters, offering a more advanced and efficient approach.
Here is an example of hyperparameter tuning using grid search in Scikit-learn:
from sklearn.model_selection import GridSearchCV
# Define the parameter grid
param_grid = {
'n_estimators': [50, 100, 200],
'max_depth': [None, 10, 20, 30],
'min_samples_split': [2, 5, 10],
'min_samples_leaf': [1, 2, 4]
}
# Initialize the grid search
grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=5, scoring='accuracy', n_jobs=-1)
# Fit the grid search to the data
grid_search.fit(X_train, y_train)
# Print the best parameters and score
print(f"Best Parameters: {grid_search.best_params_}")
print(f"Best Score: {grid_search.best_score_}")
This example demonstrates how to perform hyperparameter tuning using grid search, highlighting the importance of optimizing model performance.

Model Evaluation and Validation
Evaluating and validating the performance of machine learning models is crucial for ensuring their reliability and generalizability. Common evaluation metrics include accuracy, precision, recall, F1-score, and ROC-AUC for classification tasks, and mean squared error (MSE), mean absolute error (MAE), and R-squared (R²) for regression tasks.
It is essential to use cross-validation techniques to assess the model's performance on different subsets of the data, ensuring that the model generalizes well to unseen data. K-fold cross-validation is a commonly used technique that involves splitting the data into K folds, training the model on K-1 folds, and evaluating it on the remaining fold. This process is repeated K times, and the results are averaged to obtain a more robust estimate of the model's performance.
Here is an example of model evaluation using cross-validation in Scikit-learn:
from sklearn.model_selection import cross_val_score
# Perform cross-validation
cv_scores = cross_val_score(model, X, y, cv=5, scoring='accuracy')
# Print the cross-validation scores
print(f"Cross-Validation Scores: {cv_scores}")
print(f"Mean Cross-Validation Score: {cv_scores.mean()}")
This example demonstrates how to perform cross-validation to evaluate the model's performance, emphasizing the importance of robust model validation.
Best Practices for ML Implementation
Reproducibility
Reproducibility is a critical aspect of machine learning implementation. It ensures that the results obtained from a model can be consistently reproduced by others, making the model reliable and trustworthy. To achieve reproducibility, it is essential to use version control systems such as Git, document the data preprocessing steps, and set random seeds for all stochastic processes.
Using version control systems allows tracking changes to the code and data, ensuring that the entire workflow is documented and can be replicated. Setting random seeds ensures that the results are consistent across different runs, making it easier to debug and validate the model.
Here is an example of setting random seeds for reproducibility in Python:
import numpy as np
import tensorflow as tf
import random
# Set random seeds for reproducibility
np.random.seed(42)
tf.random.set_seed(42)
random.seed(42)
# Your machine learning code here
This example demonstrates how to set random seeds in Python to ensure reproducibility, highlighting the importance of consistent results.
Automation and Continuous Integration
Automation and continuous integration (CI) are essential for maintaining the efficiency and reliability of machine learning pipelines. Automation involves using tools such as Airflow or Luigi to schedule and manage data processing tasks, ensuring that the data pipeline runs smoothly and without manual intervention.
Continuous integration involves using CI tools such as Jenkins or GitHub Actions to automate the testing and deployment of machine learning models. This ensures that any changes to the code are automatically tested and deployed, reducing the risk of errors and improving overall system reliability.
Here is an example of setting up a simple CI pipeline using GitHub Actions:
name: CI Pipeline
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.8
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: |
pytest
This example demonstrates how to set up a simple CI pipeline using GitHub Actions, ensuring that the code is automatically tested and deployed.
Monitoring and Maintenance
Monitoring and maintenance are crucial for ensuring the long-term reliability and performance of machine learning models. This involves tracking key performance metrics, detecting model drift, and updating the model as necessary. Monitoring tools such as Prometheus and Grafana can be used to track metrics and visualize performance trends.
Model drift occurs when the data distribution changes over time, leading to a decline in model performance. It is essential to regularly evaluate the model on new data and retrain it as necessary to ensure that it remains accurate and reliable.
Here is an example of setting up monitoring for a machine learning model using Prometheus:
from prometheus_client import start_http_server, Summary
import random
import time
# Create a metric to track model inference time
REQUEST_TIME = Summary('model_inference_seconds', 'Time spent processing request')
# Start the Prometheus server
start_http_server(8000)
# Simulate model inference
while True:
start_time = time.time()
# Simulate model inference
time.sleep(random.uniform(0.1, 0.5))
inference_time = time.time() - start_time
REQUEST_TIME.observe(inference_time)
This example demonstrates how to set up monitoring for a machine learning model using Prometheus, ensuring that key performance metrics are tracked and visualized.
By following these best practices for data pipeline design and machine learning implementation, you can ensure that your machine learning projects are efficient, reproducible, and scalable. Whether you're working with big data, real-time analytics, or complex machine learning models, these practices will help you achieve optimal performance and reliability.
If you want to read more articles similar to Data Pipeline and ML Implementation Best Practices in Python, you can visit the Tools category.
You Must Read