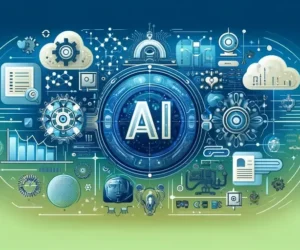
Deep Learning Methods for App Enhancement
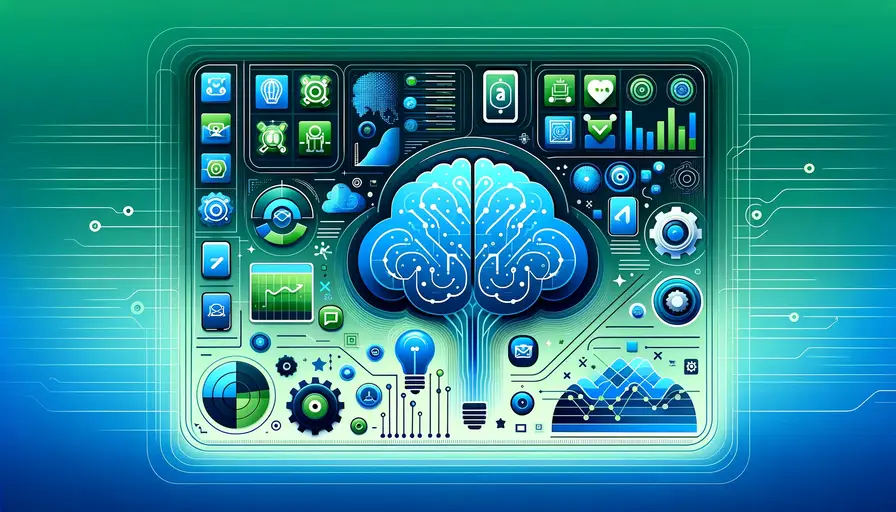
- Enhance Image Recognition with CNNs
- Improve NLP and Speech with RNNs
- Enhance Graphics with GANs
- Optimize Performance with Deep Reinforcement Learning
- Personalized Recommendations with Deep Learning
- Automatic Feature Extraction and Classification
- Real-Time Object Detection and Tracking
- Sentiment Analysis and Emotion Recognition
- Enhancing UI Design with Deep Learning
- Predictive Analytics and Forecasting
Enhance Image Recognition with CNNs
Benefits of CNNs in Apps
Convolutional Neural Networks (CNNs) are powerful tools for enhancing image recognition and processing within applications. CNNs are particularly well-suited for tasks involving image data due to their ability to automatically learn spatial hierarchies from raw pixel data. This capability is crucial for apps that rely on accurate image recognition, such as photo editing software, augmented reality applications, and visual search engines.
Implementing CNNs in your app can significantly improve the accuracy and efficiency of image processing tasks. CNNs can handle various image-related tasks such as object detection, facial recognition, and image segmentation, making them versatile tools for app enhancement. By leveraging CNNs, developers can create more intuitive and responsive user experiences, as the app can quickly and accurately interpret visual information.
Here’s an example of using CNNs for image classification with TensorFlow:
import tensorflow as tf
from tensorflow.keras import datasets, layers, models
# Load and preprocess data
(train_images, train_labels), (test_images, test_labels) = datasets.cifar10.load_data()
train_images, test_images = train_images / 255.0, test_images / 255.0
# Build the CNN model
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(10)
])
# Compile and train the model
model.compile(optimizer='adam', loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True), metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=10, validation_data=(test_images, test_labels))
This code demonstrates how to build and train a CNN for image classification using TensorFlow.
Machine Learning Boosts Nanophotonic Waveguide AnalysesImprove NLP and Speech with RNNs
Enhancing NLP
Recurrent Neural Networks (RNNs) are designed to handle sequential data, making them ideal for natural language processing (NLP) tasks. RNNs can capture temporal dependencies and context in text, allowing them to understand and generate human language more effectively. For apps that require robust NLP capabilities, such as chatbots, language translation tools, and text summarizers, RNNs provide a significant advantage.
By implementing RNNs, developers can enhance the app’s ability to understand user inputs, generate coherent responses, and process complex language structures. This leads to a more natural and engaging user experience, as the app can interact with users in a more meaningful and context-aware manner.
Improving Speech Recognition
Speech recognition is another area where RNNs excel. RNNs can process audio sequences and recognize spoken words and phrases with high accuracy. This capability is essential for apps that offer voice-activated features, virtual assistants, or speech-to-text functionalities. By integrating RNNs for speech recognition, developers can create apps that respond accurately to voice commands, improving user convenience and accessibility.
Here’s an example of using RNNs for text generation with PyTorch:
Explore the Top Machine Learning Projects for Innovative Solutionsimport torch
import torch.nn as nn
import torch.optim as optim
# Define the RNN model
class RNN(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(RNN, self).__init__()
self.hidden_size = hidden_size
self.i2h = nn.Linear(input_size + hidden_size, hidden_size)
self.i2o = nn.Linear(input_size + hidden_size, output_size)
self.softmax = nn.LogSoftmax(dim=1)
def forward(self, input, hidden):
combined = torch.cat((input, hidden), 1)
hidden = self.i2h(combined)
output = self.i2o(combined)
output = self.softmax(output)
return output, hidden
def initHidden(self):
return torch.zeros(1, self.hidden_size)
# Sample input and model initialization
n_letters = 57 # Number of letters in the input
n_hidden = 128 # Number of hidden units
n_categories = 18 # Number of output categories
rnn = RNN(n_letters, n_hidden, n_categories)
This code demonstrates how to build a simple RNN for text generation using PyTorch.
Enhance Graphics with GANs
Introduction to GANs
Generative Adversarial Networks (GANs) are a class of deep learning models that consist of two neural networks: a generator and a discriminator. The generator creates realistic data samples, while the discriminator evaluates their authenticity. GANs are particularly effective in generating high-quality graphics and visuals, making them valuable for enhancing app aesthetics and user engagement.
Enhancing App Graphics
Using GANs in your app can significantly enhance the quality of graphics and visuals. GANs can generate realistic images, animations, and textures, which can be used to create more immersive and visually appealing user interfaces. This is particularly useful for apps in gaming, virtual reality, and digital art, where high-quality graphics are crucial.
Here’s an example of using GANs for image generation with TensorFlow:
AI Translation Technology with Deep Learningimport tensorflow as tf
from tensorflow.keras import layers
# Define the generator model
def make_generator_model():
model = tf.keras.Sequential()
model.add(layers.Dense(7*7*256, use_bias=False, input_shape=(100,)))
model.add(layers.BatchNormalization())
model.add(layers.LeakyReLU())
model.add(layers.Reshape((7, 7, 256)))
model.add(layers.Conv2DTranspose(128, (5, 5), strides=(1, 1), padding='same', use_bias=False))
model.add(layers.BatchNormalization())
model.add(layers.LeakyReLU())
model.add(layers.Conv2DTranspose(64, (5, 5), strides=(2, 2), padding='same', use_bias=False))
model.add(layers.BatchNormalization())
model.add(layers.LeakyReLU())
model.add(layers.Conv2DTranspose(1, (5, 5), strides=(2, 2), padding='same', use_bias=False, activation='tanh'))
return model
# Create the generator
generator = make_generator_model()
This code demonstrates how to build a simple generator model for image generation using TensorFlow.
Optimize Performance with Deep Reinforcement Learning
Deep Reinforcement Learning
Deep reinforcement learning (DRL) combines reinforcement learning with deep learning to create algorithms that learn optimal behaviors through trial and error. DRL is particularly useful for optimizing app performance and user experience based on real-time feedback. By continuously learning from user interactions, DRL algorithms can adjust the app’s features and functionalities to better meet user needs.
Implementing DRL
Implementing DRL in your app involves setting up an environment where the app can learn from user interactions. The DRL algorithm receives feedback in the form of rewards or penalties based on the actions it takes. Over time, the algorithm learns to maximize rewards, leading to improved app performance and user satisfaction.
Here’s an example of implementing a simple DRL agent using OpenAI Gym and TensorFlow:
Benefits of Unsupervised Machine Learningimport gym
import numpy as np
import tensorflow as tf
from tensorflow.keras import layers
# Define the DRL agent
class DQNAgent:
def __init__(self, state_size, action_size):
self.state_size = state_size
self.action_size = action_size
self.model = self._build_model()
def _build_model(self):
model = tf.keras.Sequential()
model.add(layers.Dense(24, input_dim=self.state_size, activation='relu'))
model.add(layers.Dense(24, activation='relu'))
model.add(layers.Dense(self.action_size, activation='linear'))
model.compile(loss='mse', optimizer=tf.keras.optimizers.Adam(lr=0.001))
return model
# Create the environment
env = gym.make('CartPole-v1')
state_size = env.observation_space.shape[0]
action_size = env.action_space.n
# Initialize the agent
agent = DQNAgent(state_size, action_size)
This code demonstrates how to set up a simple DRL agent for the CartPole environment using TensorFlow.
Personalized Recommendations with Deep Learning
Benefits of Personalization
Personalized recommendation systems use deep learning techniques to analyze user behavior and preferences, providing tailored content and suggestions. These systems are essential for enhancing user engagement and satisfaction in apps that offer a wide range of content, such as streaming services, e-commerce platforms, and social media.
Content Curation
Content curation involves selecting and organizing content to match user preferences. Deep learning models can analyze vast amounts of data to identify patterns and trends, allowing the app to deliver personalized recommendations. This improves the user experience by ensuring that users receive relevant and interesting content, increasing their engagement and retention.
Here’s an example of implementing a simple recommendation system using collaborative filtering with TensorFlow:
Guide to Machine Learning Models for Missing Dataimport tensorflow as tf
from tensorflow.keras.layers import Embedding, Input, Dot, Flatten
from tensorflow.keras.models import Model
# Define the recommendation model
user_input = Input(shape=(1,), dtype='int32')
item_input = Input(shape=(1,), dtype='int32')
user_embedding = Embedding(input_dim=100, output_dim=50)(user_input)
item_embedding = Embedding(input_dim=100, output_dim=50)(item_input
)
user_vector = Flatten()(user_embedding)
item_vector = Flatten()(item_embedding)
dot_product = Dot(axes=1)([user_vector, item_vector])
model = Model(inputs=[user_input, item_input], outputs=dot_product)
# Compile the model
model.compile(optimizer='adam', loss='mse')
This code demonstrates how to build a simple collaborative filtering recommendation system using TensorFlow.
Automatic Feature Extraction and Classification
Feature Extraction
Automatic feature extraction is a process where deep learning models automatically identify and extract relevant features from raw data. This capability is crucial for handling unstructured data, such as images, text, and audio. By automating feature extraction, deep learning models can streamline the data preprocessing pipeline, making it more efficient and less reliant on manual intervention.
Classification
Classification involves categorizing data into predefined classes based on the extracted features. Deep learning models excel at classification tasks due to their ability to learn complex patterns and representations. Implementing deep learning for classification can enhance the accuracy and efficiency of your app’s data processing capabilities.
Here’s an example of using a pre-trained CNN for feature extraction and classification with Keras:
Analyzing Accuracy of Loan Approval Prediction with Machine Learningimport tensorflow as tf
from tensorflow.keras.applications import VGG16
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Dense, Flatten
# Load the pre-trained VGG16 model
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# Add custom classification layers
x = base_model.output
x = Flatten()(x)
x = Dense(256, activation='relu')(x)
predictions = Dense(10, activation='softmax')(x)
# Create the new model
model = Model(inputs=base_model.input, outputs=predictions)
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
This code demonstrates how to use a pre-trained CNN for feature extraction and classification with Keras.
Real-Time Object Detection and Tracking
Real-Time Detection
Real-time object detection involves identifying and localizing objects within an image or video stream as it is being captured. This capability is essential for applications such as autonomous driving, surveillance, and augmented reality. Deep learning models, such as YOLO (You Only Look Once) and SSD (Single Shot MultiBox Detector), are commonly used for real-time object detection due to their speed and accuracy.
Object Tracking
Object tracking extends object detection by following the identified objects across multiple frames in a video. This is crucial for applications that require continuous monitoring and interaction with moving objects. Implementing deep learning models for object detection and tracking can enhance your app’s ability to analyze and interact with dynamic environments.
Here’s an example of using a pre-trained YOLO model for real-time object detection with OpenCV:
import cv2
import numpy as np
# Load YOLO model and weights
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# Load image
img = cv2.imread("image.jpg")
height, width, channels = img.shape
# Prepare image for YOLO
blob = cv2.dnn.blobFromImage(img, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
net.setInput(blob)
outs = net.forward(output_layers)
# Analyze detections
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
# Get bounding box coordinates
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
x = int(center_x - w / 2)
y = int(center_y - h / 2)
# Draw bounding box
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(img, str(class_id), (x, y + 30), cv2.FONT_HERSHEY_PLAIN, 1, (0, 255, 0), 2)
# Display image
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
This code demonstrates how to use a YOLO model for real-time object detection with OpenCV.
Sentiment Analysis and Emotion Recognition
Sentiment Analysis
Sentiment analysis uses deep learning to determine the sentiment or emotional tone of text data, such as user reviews, social media posts, and feedback. This analysis helps in understanding user opinions and improving the app’s user experience by addressing concerns and enhancing positive aspects.
Emotion Recognition
Emotion recognition extends sentiment analysis by identifying specific emotions expressed in text or speech. This capability is valuable for apps that aim to provide personalized experiences, such as virtual assistants and customer support systems. Implementing deep learning models for sentiment analysis and emotion recognition can enhance your app’s ability to understand and respond to user emotions effectively.
Here’s an example of using a pre-trained model for sentiment analysis with Hugging Face’s Transformers library:
from transformers import pipeline
# Load pre-trained sentiment analysis model
sentiment_analyzer = pipeline('sentiment-analysis')
# Analyze sentiment of text
result = sentiment_analyzer("I love using this app! It's fantastic.")
print(result)
This code demonstrates how to use a pre-trained model for sentiment analysis with Hugging Face’s Transformers library.
Enhancing UI Design with Deep Learning
Automating UI Design
Automating UI design with deep learning involves using models to generate and optimize user interface layouts. This approach can streamline the design process, ensuring that the UI is intuitive, aesthetically pleasing, and user-friendly. By leveraging deep learning, developers can create dynamic and adaptive UIs that respond to user interactions and preferences.
Enhancing User Interaction
Enhancing user interaction through deep learning involves analyzing user behavior and feedback to improve the app’s usability and engagement. Deep learning models can identify patterns in how users interact with the app, providing insights that inform design improvements and feature enhancements. This leads to a more personalized and satisfying user experience.
Here’s an example of using a deep learning model for UI layout generation with TensorFlow:
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense
from tensorflow.keras.models import Model
# Define the UI layout generation model
input_layer = Input(shape=(10,))
x = Dense(64, activation='relu')(input_layer)
x = Dense(64, activation='relu')(x)
output_layer = Dense(4, activation='sigmoid')(x)
# Create the model
model = Model(inputs=input_layer, outputs=output_layer)
# Compile the model
model.compile(optimizer='adam', loss='mse')
# Summary of the model
model.summary()
This code demonstrates how to build a simple deep learning model for UI layout generation with TensorFlow.
Predictive Analytics and Forecasting
Predictive Analytics
Predictive analytics uses deep learning models to analyze historical data and make predictions about future events. This capability is essential for apps that provide insights and forecasts, such as financial planning tools, demand forecasting systems, and health monitoring applications. By implementing predictive analytics, developers can offer users valuable foresight and decision-making support.
Forecasting
Forecasting involves predicting future trends and patterns based on historical data. Deep learning models, such as Long Short-Term Memory (LSTM) networks, are particularly effective for time series forecasting due to their ability to capture temporal dependencies. Implementing forecasting models can enhance your app’s ability to provide accurate and timely predictions.
Here’s an example of using an LSTM network for time series forecasting with Keras:
import numpy as np
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
# Generate sample time series data
data = np.sin(np.linspace(0, 100, 1000))
X = np.array([data[i:i+10] for i in range(len(data)-10)])
y = data[10:]
# Reshape data for LSTM
X = X.reshape((X.shape[0], X.shape[1], 1))
# Define the LSTM model
model = Sequential()
model.add(LSTM(50, activation='relu', input_shape=(10, 1)))
model.add(Dense(1))
# Compile and train the model
model.compile(optimizer='adam', loss='mse')
model.fit(X, y, epochs=100, verbose=1)
This code demonstrates how to build and train an LSTM network for time series forecasting with Keras.
Deep learning methods offer a wide range of capabilities for enhancing apps across various domains. From improving image and speech recognition to optimizing performance and personalizing user experiences, deep learning provides powerful tools for developers to create more intelligent and responsive applications. By leveraging these techniques, you can maximize the potential of your app and deliver exceptional user experiences.
If you want to read more articles similar to Deep Learning Methods for App Enhancement, you can visit the Applications category.
You Must Read