
Enhancing Investment Decisions with Machine Learning Techniques
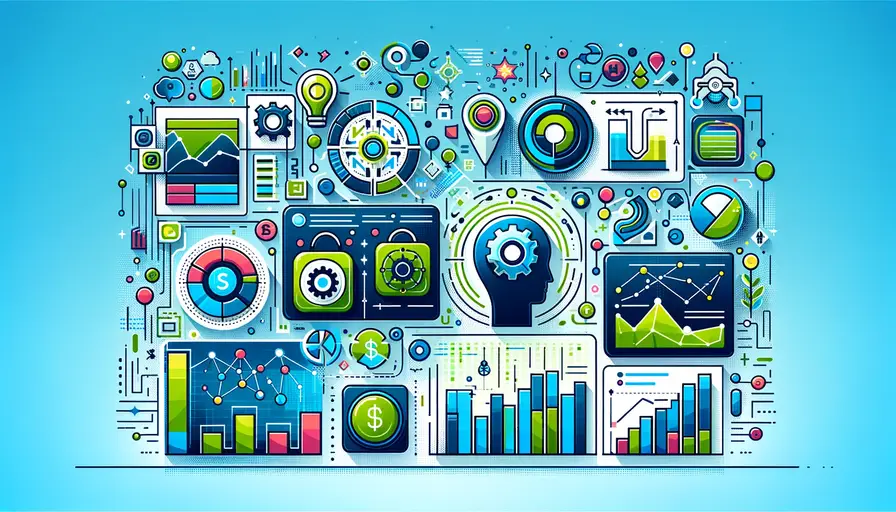
Content
-
Transforming Investment Strategies with Machine Learning
- Leveraging Predictive Analytics for Better Insights
- Example: Stock Price Prediction Using LSTM in Python
- Enhancing Portfolio Management
- Example: Portfolio Optimization Using Reinforcement Learning in Python
- Improving Sentiment Analysis for Market Sentiment
- Example: Market Sentiment Analysis Using BERT in Python
- Real-World Applications of Machine Learning in Investment
Transforming Investment Strategies with Machine Learning
Leveraging Predictive Analytics for Better Insights
Machine learning has revolutionized the field of investment by providing powerful predictive analytics capabilities. Traditional investment strategies often relied on historical data and human intuition, which could lead to suboptimal decisions. Machine learning models, on the other hand, can analyze vast amounts of data to uncover patterns and trends that may not be immediately apparent to human analysts.
Predictive analytics in investment involves using machine learning algorithms to forecast future asset prices, market movements, and economic indicators. Techniques such as time series analysis and regression models can predict stock prices, helping investors make informed decisions. By incorporating variables such as historical prices, trading volume, and macroeconomic indicators, these models provide a comprehensive view of potential market trends.
For example, LSTM (Long Short-Term Memory) networks, a type of recurrent neural network (RNN), are particularly effective for time series forecasting. LSTM models can capture long-term dependencies in sequential data, making them ideal for predicting stock prices over time. By training on historical price data, LSTM models can generate accurate forecasts, aiding investors in making data-driven decisions.
Moreover, machine learning can enhance risk management by predicting potential market downturns and identifying high-risk assets. By analyzing historical market data and identifying patterns associated with past crises, machine learning models can provide early warning signals for future risks. This predictive capability allows investors to adjust their portfolios proactively, mitigating potential losses.
Hybrid Machine Learning Models for Crop Yield PredictionExample: Stock Price Prediction Using LSTM in Python
import numpy as np
import pandas as pd
from sklearn.preprocessing import MinMaxScaler
from keras.models import Sequential
from keras.layers import LSTM, Dense
# Load and preprocess data
data = pd.read_csv('stock_prices.csv')
scaler = MinMaxScaler(feature_range=(0, 1))
scaled_data = scaler.fit_transform(data['Close'].values.reshape(-1, 1))
# Create sequences for training
def create_sequences(data, seq_length):
X, y = [], []
for i in range(len(data) - seq_length):
X.append(data[i:i + seq_length])
y.append(data[i + seq_length])
return np.array(X), np.array(y)
seq_length = 60
X, y = create_sequences(scaled_data, seq_length)
X_train, y_train = X[:-100], y[:-100]
X_test, y_test = X[-100:], y[-100:]
# Build and train LSTM model
model = Sequential()
model.add(LSTM(50, return_sequences=True, input_shape=(seq_length, 1)))
model.add(LSTM(50))
model.add(Dense(1))
model.compile(optimizer='adam', loss='mean_squared_error')
model.fit(X_train, y_train, epochs=20, batch_size=32, validation_data=(X_test, y_test))
# Make predictions
predictions = model.predict(X_test)
predictions = scaler.inverse_transform(predictions)
# Evaluate model performance
actual = scaler.inverse_transform(y_test.reshape(-1, 1))
mse = np.mean((predictions - actual) ** 2)
print(f'Mean Squared Error: {mse}')
In this example, an LSTM model is built using Keras to predict stock prices based on historical data. The model is trained on sequences of past prices and can forecast future prices, demonstrating the application of predictive analytics in investment.
Enhancing Portfolio Management
Machine learning techniques have also transformed portfolio management by optimizing asset allocation and improving diversification strategies. Traditional portfolio management often involves balancing risk and return based on historical performance and analyst recommendations. Machine learning models can enhance this process by identifying optimal asset allocations that maximize returns while minimizing risk.
Reinforcement learning is particularly useful for dynamic portfolio management. In reinforcement learning, an agent learns to make decisions by interacting with the environment and receiving feedback in the form of rewards or penalties. This approach allows the agent to optimize portfolio allocation over time, adapting to changing market conditions. Techniques such as Q-learning and Deep Q-Networks (DQNs) enable the development of intelligent trading agents that can learn and improve their strategies autonomously.
Additionally, machine learning models can identify hidden correlations and dependencies between assets, improving diversification. By analyzing large datasets of asset returns, machine learning algorithms can uncover complex relationships that traditional methods might miss. These insights enable investors to construct diversified portfolios that are better positioned to withstand market volatility.
Improving Radiology Diagnoses: Machine Learning for AccuracyMoreover, factor investing has benefited from machine learning by identifying factors that drive asset returns. Machine learning models can analyze vast amounts of data to discover new factors that influence asset performance. These factors can then be incorporated into investment strategies, enhancing returns and reducing risk. By leveraging machine learning, investors can gain a deeper understanding of the drivers of asset returns and make more informed decisions.
Example: Portfolio Optimization Using Reinforcement Learning in Python
import numpy as np
import pandas as pd
import gym
from gym import spaces
class PortfolioEnv(gym.Env):
def __init__(self, prices, initial_cash=10000):
self.prices = prices
self.initial_cash = initial_cash
self.n_assets = prices.shape[1]
self.action_space = spaces.Box(low=-1, high=1, shape=(self.n_assets,), dtype=np.float32)
self.observation_space = spaces.Box(low=0, high=np.inf, shape=(self.n_assets + 1,), dtype=np.float32)
self.reset()
def reset(self):
self.cash = self.initial_cash
self.holdings = np.zeros(self.n_assets)
self.current_step = 0
return self._get_observation()
def _get_observation(self):
return np.concatenate(([self.cash], self.holdings))
def step(self, action):
action = np.clip(action, -1, 1)
prices = self.prices[self.current_step]
self.holdings += action
self.cash -= np.sum(prices * action)
self.current_step += 1
done = self.current_step >= len(self.prices) - 1
reward = self._get_portfolio_value() - self.initial_cash
return self._get_observation(), reward, done, {}
def _get_portfolio_value(self):
prices = self.prices[self.current_step]
return self.cash + np.sum(prices * self.holdings)
# Load and preprocess data
prices = pd.read_csv('asset_prices.csv').values
env = PortfolioEnv(prices)
# Training using a simple reinforcement learning algorithm (placeholder for actual implementation)
from stable_baselines3 import PPO
model = PPO('MlpPolicy', env, verbose=1)
model.learn(total_timesteps=10000)
# Evaluate the trained model
obs = env.reset()
for _ in range(len(prices) - 1):
action, _ = model.predict(obs)
obs, reward, done, _ = env.step(action)
if done:
break
print(f'Final portfolio value: {env._get_portfolio_value()}')
In this example, a simple reinforcement learning environment is created for portfolio management. The agent learns to allocate assets dynamically to maximize portfolio value, demonstrating the application of reinforcement learning in investment.
Improving Sentiment Analysis for Market Sentiment
Sentiment analysis is a powerful tool for understanding market sentiment and predicting asset price movements. By analyzing text data from news articles, social media posts, and financial reports, machine learning models can gauge the overall sentiment of the market. This information can then be used to make more informed investment decisions.
Natural language processing (NLP) techniques, such as transformer-based models like BERT and GPT-3, have significantly improved sentiment analysis. These models can understand the context and nuances of text, providing more accurate sentiment predictions. For example, a sentiment analysis model can analyze financial news to determine whether the overall sentiment is positive or negative, helping investors predict market movements.
Using Machine Learning to Detect and Predict DDoS AttacksFurthermore, sentiment analysis can be combined with other data sources, such as historical prices and trading volume, to create more robust predictive models. By incorporating sentiment data, machine learning models can capture the psychological factors that influence market behavior. This approach provides a more comprehensive view of the market, enabling better investment decisions.
Sentiment analysis is also valuable for event-driven trading, where models react to specific news events or market developments. By analyzing the sentiment surrounding these events, models can predict their impact on asset prices and adjust trading strategies accordingly. This capability allows investors to capitalize on short-term market opportunities and mitigate risks associated with negative events.
Example: Market Sentiment Analysis Using BERT in Python
import numpy as np
import pandas as pd
from transformers import BertTokenizer, BertForSequenceClassification
from transformers import Trainer, TrainingArguments
# Load dataset
data = pd.read_csv('market_sentiment_data.csv')
train_data = data.sample(frac=0.8, random_state=42)
test_data = data.drop(train_data.index)
# Tokenize data
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
train_encodings = tokenizer(train_data['text'].tolist(), truncation=True, padding=True)
test_encodings = tokenizer(test_data['text'].tolist(), truncation=True, padding=True)
# Create dataset class
class SentimentDataset(torch.utils.data.Dataset):
def __init__(self, encodings, labels):
self.encodings = encodings
self.labels = labels
def __getitem__(self, idx):
item = {key: torch.tensor(val[idx]) for key, val in self.encodings.items()}
item['labels'] = torch.tensor(self.labels[idx])
return item
def __len__(self):
return len(self.labels)
train_dataset = SentimentDataset(train_encodings, train_data['label'].tolist())
test_dataset = SentimentDataset(test_encodings, test_data['label'].tolist())
# Initialize model
model = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
# Training arguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=16,
per_device_eval_batch_size=16,
warmup_steps=500,
weight_decay=0.01,
logging_dir='./logs',
logging_steps=10,
)
# Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=test_dataset
)
# Train model
trainer.train()
In this example, a BERT model is used to perform sentiment analysis on market-related text data. The model can classify the sentiment of news articles and social media posts, providing insights into market sentiment and aiding investment decisions.
Real-World Applications of Machine Learning in Investment
Automated Trading Systems
Automated trading systems, also known as algorithmic trading, have become increasingly popular due to advancements in machine learning. These systems use algorithms to execute trades based on predefined criteria and real-time market data. Machine learning enhances automated trading by enabling more sophisticated strategies that can adapt to changing market conditions.
Top Stocks Benefiting from Deep Learning Neural NetworksOne of the key benefits of automated trading systems is their ability to operate at high speed and frequency, executing trades within milliseconds. This capability is particularly valuable in high-frequency trading (HFT), where small price movements can be exploited for profit. Machine learning models can analyze vast amounts of data and identify trading opportunities faster than human traders, providing a competitive edge in the market.
Moreover, automated trading systems can reduce the emotional bias associated with manual trading. Human traders are often influenced by fear and greed, leading to suboptimal decisions. Machine learning algorithms, on the other hand, follow predefined rules and are not subject to emotional biases. This approach ensures more consistent and disciplined trading, improving overall performance.
Automated trading systems also benefit from backtesting, where models are tested on historical data to evaluate their performance. By simulating trades based on past market conditions, machine learning models can be fine-tuned and optimized before being deployed in live trading. This process helps identify potential issues and ensures that the trading strategy is robust and reliable.
Personalized Financial Advice
Machine learning has the potential to revolutionize personalized financial advice by providing tailored recommendations based on individual investor profiles. Traditional financial advisory services often rely on generic advice that may not account for an individual's specific needs and preferences. Machine learning models, however, can analyze a wide range of factors to provide personalized investment recommendations.
ML for NLP in ElasticsearchPersonalized financial advice involves analyzing an investor's financial goals, risk tolerance, investment horizon, and other personal factors. Machine learning algorithms can process this information and recommend a customized portfolio that aligns with the investor's objectives. This approach ensures that investment strategies are tailored to the unique needs of each investor, improving the likelihood of achieving their financial goals.
Furthermore, machine learning models can continuously monitor an investor's portfolio and provide real-time recommendations. As market conditions change, the models can suggest adjustments to the portfolio to optimize performance. This dynamic approach ensures that investors can respond to market developments quickly and take advantage of new opportunities.
Personalized financial advice also benefits from natural language processing (NLP), which enables conversational interfaces for financial planning. Chatbots and virtual financial advisors can interact with investors, gather information, and provide advice in a user-friendly manner. This capability makes financial planning more accessible and convenient, encouraging more people to engage in proactive financial management.
Example: Personalized Portfolio Recommendation Using Python
import numpy as np
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
# Load and preprocess data
data = pd.read_csv('investor_profiles.csv')
X = data.drop('recommended_portfolio', axis=1)
y = data['recommended_portfolio']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Random Forest classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate model performance
accuracy = np.mean(y_pred == y_test)
print(f'Accuracy: {accuracy}')
# Example recommendation for a new investor
new_investor = pd.DataFrame({
'age': [30],
'income': [70000],
'risk_tolerance': [7],
'investment_horizon': [10]
})
recommended_portfolio = model.predict(new_investor)
print(f'Recommended Portfolio: {recommended_portfolio[0]}')
In this example, a Random Forest classifier is trained to recommend personalized portfolios based on investor profiles. The model can analyze various factors and provide tailored investment recommendations, demonstrating the application of machine learning in personalized financial advice.
Accurate Name Recognition and Classification using Machine LearningRisk Assessment and Management
Effective risk assessment and management are critical components of successful investment strategies. Machine learning models can enhance these processes by providing more accurate and comprehensive risk assessments. By analyzing historical data and identifying patterns associated with risk, machine learning models can predict potential risks and help investors make informed decisions.
One of the key applications of machine learning in risk management is credit risk assessment. Financial institutions use machine learning algorithms to evaluate the creditworthiness of borrowers by analyzing their financial history, transaction patterns, and other relevant factors. These models can predict the likelihood of default and provide a more accurate assessment of credit risk, enabling lenders to make better-informed lending decisions.
Machine learning also plays a crucial role in fraud detection, where models analyze transaction data to identify suspicious activity. By detecting anomalies and patterns indicative of fraud, machine learning models can prevent financial losses and protect both institutions and customers. This capability is particularly valuable in the era of digital transactions, where the volume and complexity of data make manual fraud detection impractical.
Furthermore, machine learning models can assess market risk by analyzing factors such as volatility, trading volume, and economic indicators. These models can predict potential market downturns and help investors manage their portfolios accordingly. By providing early warning signals and actionable insights, machine learning enhances risk management and ensures more resilient investment strategies.
Machine learning techniques have revolutionized investment strategies by enhancing predictive analytics, optimizing portfolio management, improving sentiment analysis, and enabling automated trading systems. Real-world applications in personalized financial advice and risk management demonstrate the transformative potential of machine learning in the investment industry. By leveraging these advanced techniques, investors can make more informed decisions, optimize their portfolios, and achieve better financial outcomes.
If you want to read more articles similar to Enhancing Investment Decisions with Machine Learning Techniques, you can visit the Applications category.
You Must Read