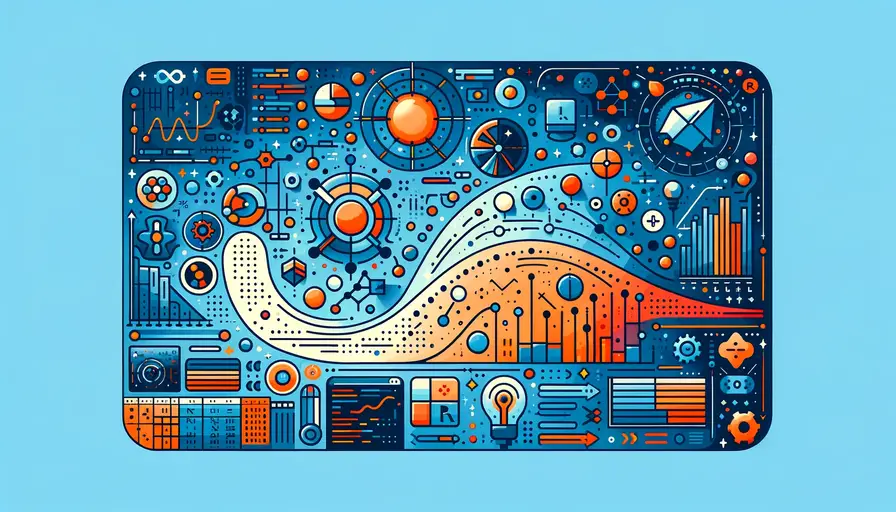
Enhancing JavaScript Chatbot with Machine Learning

The rise of chatbots has transformed customer service, providing instant and efficient interactions with users. Traditional rule-based chatbots, however, often lack the ability to handle complex queries and adapt to new information. Integrating machine learning with JavaScript chatbots enhances their capabilities, making them smarter, more responsive, and more human-like. This article explores how to enhance JavaScript chatbots using machine learning, focusing on key techniques, practical implementations, and the benefits of this integration.
Benefits of Integrating Machine Learning with Chatbots
Improved User Interaction
Machine learning enables chatbots to understand and respond to user queries more accurately. Unlike rule-based bots, which rely on predefined scripts, machine learning algorithms can analyze user input, recognize patterns, and generate appropriate responses. This leads to more natural and engaging conversations, enhancing the overall user experience.
With natural language processing (NLP), chatbots can understand the nuances of human language, including slang, idioms, and context. This allows them to provide more relevant and personalized responses, improving user satisfaction and engagement.
Scalability and Efficiency
Machine learning-powered chatbots can handle a large volume of interactions simultaneously, making them highly scalable. This is particularly beneficial for businesses that experience high traffic, as the chatbot can efficiently manage multiple conversations without compromising response quality.

Automation of repetitive tasks and queries reduces the workload on human agents, allowing them to focus on more complex issues. This increases operational efficiency and reduces costs associated with customer support.
Continuous Learning and Improvement
One of the significant advantages of machine learning is its ability to learn from data. Chatbots integrated with machine learning can continuously improve their performance by analyzing past interactions and updating their responses based on user feedback. This iterative learning process ensures that the chatbot becomes more accurate and effective over time.
Machine learning models can identify gaps in the chatbot's knowledge and suggest improvements, leading to a more robust and comprehensive system. This adaptive learning capability is crucial for maintaining high-quality interactions and staying relevant in a rapidly changing environment.
Key Machine Learning Techniques for Chatbots
Natural Language Processing (NLP)
NLP is a branch of artificial intelligence that focuses on the interaction between computers and human language. It enables chatbots to understand, interpret, and respond to user input in a meaningful way. Key NLP tasks include tokenization, part-of-speech tagging, named entity recognition, and sentiment analysis.

NLP techniques allow chatbots to comprehend the context and intent behind user queries, enabling more accurate and relevant responses. This is essential for creating conversational agents that can engage users effectively and provide valuable assistance.
Example of basic NLP processing using Python and NLTK:
import nltk
from nltk.tokenize import word_tokenize
from nltk.tag import pos_tag
# Sample text
text = "Hello! How can I assist you today?"
# Tokenize the text
tokens = word_tokenize(text)
# Perform part-of-speech tagging
tagged_tokens = pos_tag(tokens)
print("Tokenized Text:", tokens)
print("POS Tagged Text:", tagged_tokens)
Machine Learning Models
Various machine learning models can be employed to enhance chatbot capabilities. Common models include support vector machines (SVM), decision trees, and neural networks. These models can be trained on large datasets to recognize patterns and make predictions based on user input.
Neural networks, particularly recurrent neural networks (RNNs) and transformers, are widely used for building advanced chatbots. These models excel at handling sequential data and capturing long-term dependencies, making them ideal for processing and generating human-like responses.

Sentiment Analysis
Sentiment analysis is a technique used to determine the emotional tone behind a text. It helps chatbots understand the user's mood and tailor responses accordingly. By analyzing the sentiment of user queries, chatbots can provide empathetic and contextually appropriate replies, enhancing the user experience.
Sentiment analysis models classify text as positive, negative, or neutral, enabling the chatbot to adjust its tone and approach based on the user's emotional state. This capability is particularly useful in customer service applications, where understanding and addressing user emotions can lead to better outcomes.
Example of sentiment analysis using Python and TextBlob:
from textblob import TextBlob
# Sample text
text = "I am really happy with the service!"
# Perform sentiment analysis
blob = TextBlob(text)
sentiment = blob.sentiment
print("Sentiment:", sentiment)
Practical Implementation of Machine Learning in JavaScript Chatbots
Integrating NLP Libraries
To enhance a JavaScript chatbot with NLP capabilities, developers can use libraries such as Natural and compromise. These libraries provide tools for text processing, tokenization, and sentiment analysis, enabling the chatbot to understand and respond to user input more effectively.

Example of using Natural for basic text processing in JavaScript:
const natural = require('natural');
const tokenizer = new natural.WordTokenizer();
const text = "Hello! How can I assist you today?";
const tokens = tokenizer.tokenize(text);
console.log("Tokenized Text:", tokens);
Training Machine Learning Models
Training machine learning models for chatbots typically involves collecting and preprocessing data, selecting an appropriate algorithm, and evaluating model performance. In JavaScript, developers can use libraries such as Brain.js and TensorFlow.js to build and train models directly in the browser or on the server.
Example of training a simple neural network using Brain.js:
const brain = require('brain.js');
// Create a neural network
const net = new brain.NeuralNetwork();
// Training data
const trainingData = [
{ input: { hello: 1 }, output: { greeting: 1 } },
{ input: { hi: 1 }, output: { greeting: 1 } },
{ input: { bye: 1 }, output: { farewell: 1 } },
{ input: { goodbye: 1 }, output: { farewell: 1 } }
];
// Train the network
net.train(trainingData);
// Test the network
const output = net.run({ hello: 1 });
console.log("Output:", output);
Implementing Sentiment Analysis
To incorporate sentiment analysis into a JavaScript chatbot, developers can use libraries like Sentiment and Aylien. These tools provide pre-trained models for sentiment analysis, enabling the chatbot to understand and respond to user emotions.

Example of using Sentiment for sentiment analysis in JavaScript:
const Sentiment = require('sentiment');
const sentiment = new Sentiment();
const text = "I am really happy with the service!";
const result = sentiment.analyze(text);
console.log("Sentiment Analysis Result:", result);
Enhancing User Experience with Advanced Features
Context-Aware Responses
One of the key advantages of integrating machine learning with chatbots is the ability to provide context-aware responses. Machine learning models can maintain the context of a conversation, allowing the chatbot to understand and respond to follow-up questions appropriately. This leads to more coherent and meaningful interactions.
Context-aware chatbots can handle multi-turn conversations, where the user's query depends on previous interactions. By maintaining a memory of the conversation, the chatbot can provide accurate and relevant responses, improving the overall user experience.
Personalized Interactions
Personalization is another significant benefit of machine learning-powered chatbots. By analyzing user data and preferences, chatbots can tailor their responses to meet individual needs. This includes recommending products, providing personalized support, and addressing users by their names.

Machine learning models can segment users based on their behavior and preferences, enabling the chatbot to offer personalized content and suggestions. This level of personalization enhances user engagement and fosters a stronger connection between the user and the brand.
Multilingual Support
Machine learning models can also enable chatbots to support multiple languages, expanding their reach and accessibility. NLP techniques, such as language detection and translation, allow chatbots to interact with users in their preferred language.
Multilingual chatbots can automatically detect the language of the user's input and provide responses in the same language. This capability is crucial for businesses operating in diverse markets, as it ensures that users can communicate with the chatbot effectively, regardless of their language.
Example of using Google Translate API for multilingual support in JavaScript:
const { Translate } = require('@google-cloud/translate').v2;
const translate = new Translate();
const text = "Hello, how can I help you?";
const target = 'es';
// Translate text
translate.translate(text, target).then(([translation]) => {
console.log(`Translation: ${translation}`);
}).catch(err => {
console.error('ERROR:', err);
});
Overcoming Challenges in Chatbot Development
Handling Ambiguity and Variability
One of the challenges in chatbot development is handling ambiguous and variable user input. Users may phrase their queries in different ways, use slang, or make typos. Machine learning models need to be robust enough to understand and interpret such input accurately.
To address this challenge, developers can use techniques such as fuzzy matching, spelling correction, and paraphrase detection. These techniques help the chatbot recognize the intended meaning behind user input, even when it is not perfectly phrased.
Ensuring Data Privacy and Security
Data privacy and security are critical considerations in chatbot development. Chatbots often handle sensitive user information, such as personal details and payment information. Ensuring that this data is securely stored and transmitted is essential to maintain user trust.
Developers must implement strong encryption, access controls, and compliance with data protection regulations, such as GDPR and CCPA. Regular security audits and updates are also necessary to protect against potential vulnerabilities.
Maintaining Conversational Flow
Maintaining a natural conversational flow is essential for a positive user experience. Chatbots should be able to handle interruptions, changes in topic, and follow-up questions without losing the context of the conversation.
Machine learning models can help maintain conversational flow by tracking the context and state of the conversation. This enables the chatbot to manage complex interactions and provide coherent and contextually appropriate responses.
Future Trends in Chatbot Technology
Advancements in Deep Learning
Deep learning is driving significant advancements in chatbot technology. Models such as GPT-3 by OpenAI and BERT by Google have set new benchmarks in natural language understanding and generation. These models can produce human-like text, making chatbots more conversational and engaging.
Future developments in deep learning will likely lead to even more sophisticated chatbots, capable of understanding and responding to complex queries with high accuracy. This will further enhance the user experience and expand the potential applications of chatbots.
Integration with Other AI Technologies
The integration of chatbots with other AI technologies, such as computer vision and voice recognition, will open up new possibilities. For example, chatbots could use computer vision to analyze images or videos sent by users and provide relevant assistance.
Voice-enabled chatbots, powered by speech recognition and synthesis technologies, offer a more natural and intuitive way for users to interact. This is particularly useful for applications where typing is impractical, such as in-car assistants or smart home devices.
Expanding Use Cases
As chatbot technology continues to evolve, new use cases will emerge across various industries. In healthcare, chatbots can assist with patient triage, appointment scheduling, and medication reminders. In education, they can provide personalized tutoring and support for students.
The integration of chatbots with e-commerce platforms can enhance the shopping experience by offering personalized recommendations, processing orders, and providing customer support. As businesses recognize the value of chatbots, their adoption will continue to grow, driving innovation and improving customer interactions.
Enhancing JavaScript chatbots with machine learning transforms them into powerful tools capable of understanding and responding to complex user queries. By leveraging NLP, machine learning models, and sentiment analysis, chatbots can provide more accurate, personalized, and context-aware interactions. Overcoming challenges related to ambiguity, data privacy, and conversational flow is essential for developing effective chatbots. Future trends in deep learning and AI integration will further expand the capabilities and applications of chatbots, making them an integral part of customer service and user engagement.
If you want to read more articles similar to Enhancing JavaScript Chatbot with Machine Learning, you can visit the Applications category.
You Must Read