
Exploring Machine Learning Models for POC: A Comprehensive Guide
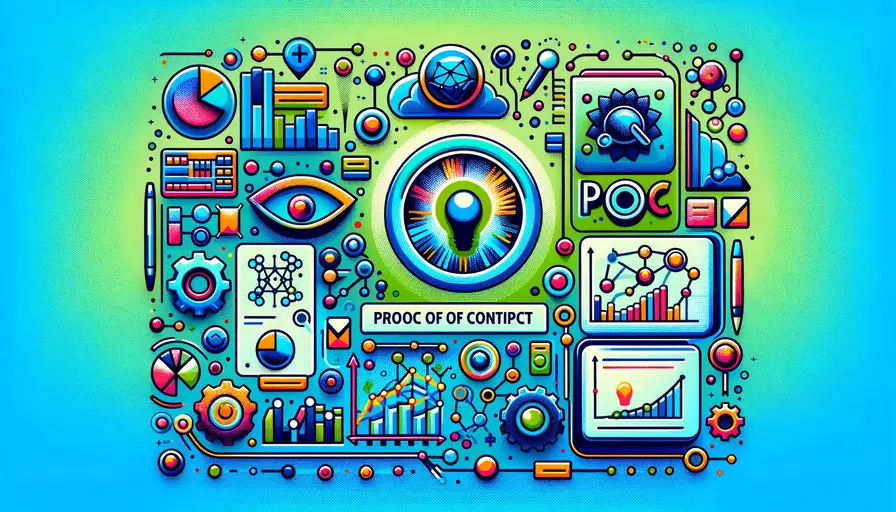
Machine learning has become an integral part of modern technology, transforming industries by enabling systems to learn from data and make informed decisions. This guide explores various machine learning models for proof of concept (POC) projects, offering a comprehensive overview of their applications, implementation, and illustrative code examples. By the end, you'll be well-equipped to apply these models to your own projects, ensuring they are robust and effective.
Binary Classification Models
Logistic Regression for Binary Classification
Logistic regression is a fundamental statistical technique used for binary classification tasks. It is especially useful for scenarios where the outcome is binary, such as predicting whether an email is spam or not. This method models the probability of a binary outcome based on one or more predictor variables.
In the context of machine learning, logistic regression is valued for its simplicity and interpretability. By using a logistic function, it can model the relationship between the predictor variables and the probability of the binary outcome, making it a go-to method for many classification problems.
Here’s how you can implement logistic regression using the Scikit-learn library in Python:
Guide to Named Entity Recognition in Machine Learningfrom sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Example dataset
X = [[0], [1], [2], [3]]
y = [0, 0, 1, 1]
# Splitting data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.25, random_state=0)
# Training the model
model = LogisticRegression()
model.fit(X_train, y_train)
# Making predictions
y_pred = model.predict(X_test)
print(f'Accuracy: {accuracy_score(y_test, y_pred):.2f}')
Decision Trees for Classification
Decision trees are a versatile and powerful method for both classification and regression tasks. They work by recursively splitting the dataset into subsets based on the value of a selected feature. This results in a tree-like model of decisions, which can be easily visualized and interpreted.
One of the main advantages of decision trees is their ability to handle both numerical and categorical data. Additionally, they require little data preprocessing and can capture complex relationships in the
data. However, they can also be prone to overfitting, especially with noisy data, so it's important to tune them carefully.
Here is an example of implementing a decision tree classifier using Scikit-learn:
Can Machine Learning Solve Complex Puzzles?from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import classification_report
# Loading the dataset
iris = load_iris()
X, y = iris.data, iris.target
# Splitting the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Training the model
clf = DecisionTreeClassifier()
clf.fit(X_train, y_train)
# Making predictions
y_pred = clf.predict(X_test)
print(classification_report(y_test, y_pred))
Support Vector Machines for Classification
Support vector machines (SVMs) are a powerful set of supervised learning algorithms used for classification and regression. They are particularly effective in high-dimensional spaces and can be used for both linear and non-linear classifications. The core idea is to find a hyperplane that best separates the classes in the feature space.
SVMs are known for their robustness and ability to handle both linear and non-linear data using kernel functions. They are especially useful when the number of features exceeds the number of samples.
Below is an example of how to implement an SVM classifier using Scikit-learn:
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# Loading the dataset
digits = datasets.load_digits()
X, y = digits.data, digits.target
# Splitting the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Training the model
svc = SVC(kernel='linear')
svc.fit(X_train, y_train)
# Making predictions
y_pred = svc.predict(X_test)
print(f'Accuracy: {accuracy_score(y_test, y_pred):.2f}')
Regression Models
Linear Regression for Predictive Analysis
Linear regression is a foundational statistical method used to model the relationship between a dependent variable and one or more independent variables. It's commonly applied in predictive analysis to forecast future outcomes based on historical data.
Enhancing Investment Decisions with Machine Learning TechniquesLinear regression models assume a linear relationship between the input variables and the single output variable. This simplicity makes them easy to interpret and implement, though they may not capture complex relationships in the data.
Here is how to implement a linear regression model using Scikit-learn:
import numpy as np
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Example dataset
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1.5, 3.0, 4.5, 6.0, 7.5])
# Training the model
model = LinearRegression()
model.fit(X, y)
# Making predictions
y_pred = model.predict(X)
print(f'Mean Squared Error: {mean_squared_error(y, y_pred):.2f}')
Ridge Regression for Regularization
Ridge regression, also known as Tikhonov regularization, is a technique used to analyze multiple regression data that suffer from multicollinearity. When multicollinearity occurs, least squares estimates are unbiased, but their variances are large, leading to overfitting. Ridge regression adds a degree of bias to the regression estimates, which reduces the standard errors.
This method introduces a penalty term to the cost function used in linear regression, which shrinks the coefficients and thus prevents overfitting. Ridge regression is especially useful when the number of predictor variables exceeds the number of observations.
Hybrid Machine Learning Models for Crop Yield PredictionHere is an example of implementing ridge regression using Scikit-learn:
from sklearn.linear_model import Ridge
# Example dataset
X = np.array([[1, 1], [1, 2], [2, 2], [2, 3]])
y = np.dot(X, np.array([1, 2])) + 3
# Training the model
ridge = Ridge(alpha=1.0)
ridge.fit(X, y)
# Making predictions
y_pred = ridge.predict(X)
print(f'Coefficients: {ridge.coef_}')
print(f'Intercept: {ridge.intercept_}')
Lasso Regression for Feature Selection
Lasso regression, or Least Absolute Shrinkage and Selection Operator, is a type of linear regression that uses shrinkage. Shrinkage is where data values are shrunk towards a central point, like the mean. The lasso procedure encourages simple, sparse models (i.e., models with fewer parameters).
Lasso regression adds a penalty equal to the absolute value of the magnitude of the coefficients. This type of regularization can lead to some coefficients being exactly zero, which helps in feature selection by excluding irrelevant features.
Here’s how to implement lasso regression using Scikit-learn:
Improving Radiology Diagnoses: Machine Learning for Accuracyfrom sklearn.linear_model import Lasso
# Example dataset
X = np.array([[0, 0], [1, 1], [2, 2]])
y = np.array([0, 1, 2])
# Training the model
lasso = Lasso(alpha=0.1)
lasso.fit(X, y)
# Making predictions
y_pred = lasso.predict(X)
print(f'Coefficients: {lasso.coef_}')
print(f'Intercept: {lasso.intercept_}')
Clustering Models
K-Means Clustering for Data Segmentation
K-means clustering is a method of vector quantization, originally from signal processing, that is popular for cluster analysis in data mining. K-means clustering aims to partition n observations into k clusters in which each observation belongs to the cluster with the nearest mean, serving as a prototype of the cluster.
This technique is widely used for market segmentation, image compression, and as a preprocessing step for other algorithms. It is effective for large datasets and is simple to implement, though the choice of k (the number of clusters) can significantly affect the results.
Here’s how to implement K-means clustering using Scikit-learn:
from sklearn.cluster import KMeans
import numpy as np
# Example dataset
X = np.array([[1, 2], [1, 4], [1, 0],
[4, 2], [4, 4], [4, 0]])
# Training the model
kmeans = KMeans(n_clusters=2, random_state=0).fit(X)
# Making predictions
print(kmeans.labels_)
print(kmeans.cluster_centers_)
Hierarchical Clustering for Hierarchical Data
Hierarchical clustering is a method of cluster analysis which seeks to build a hierarchy of clusters. Strategies for hierarchical clustering generally fall into two types: Agglomerative and Divisive. Agglomerative clustering is a "bottom-up" approach: each observation starts in its own cluster, and pairs of clusters are merged as one moves up the hierarchy.
Using Machine Learning to Detect and Predict DDoS AttacksThis technique is useful for data that can be represented in a tree-like structure, such as phylogenetic trees in biology. It can also help in understanding the data structure and finding meaningful groupings in the data.
Here’s how to implement hierarchical clustering using SciPy:
from scipy.cluster.hierarchy import dendrogram, linkage
import matplotlib.pyplot as plt
import numpy as np
# Example dataset
X = np.array([[1, 2], [1, 4], [1, 0],
[4, 2], [4, 4], [4, 0]])
# Performing hierarchical clustering
Z = linkage(X, 'ward')
# Plotting the dendrogram
dendrogram(Z)
plt.show()
DBSCAN for Density-Based Clustering
Density-Based Spatial Clustering of Applications with Noise (DBSCAN) is a density-based clustering algorithm that is designed to discover clusters of varying shapes and sizes in large datasets containing noise and outliers. Unlike K-means, DBSCAN does not require specifying the number of clusters in advance, making it more flexible for exploratory data analysis.
DBSCAN identifies clusters based on the density of points. Points in dense regions are grouped together to form clusters, while points in sparse regions are considered outliers. This makes DBSCAN particularly effective for datasets with noise and non-linearly separable clusters.
Here’s how to implement DBSCAN using Scikit-learn:
from sklearn.cluster import DBSCAN
# Example dataset
X = np.array([[1, 2], [2, 2], [2, 3],
[8, 7], [8, 8], [25, 80]])
# Training the model
dbscan = DBSCAN(eps=3, min_samples=2).fit(X)
# Making predictions
print(dbscan.labels_)
Dimensionality Reduction Models
Principal Component Analysis for Feature Reduction
Principal Component Analysis (PCA) is a technique used to emphasize variation and bring out strong patterns in a dataset. It's often used to make data easy to explore and visualize. PCA reduces the dimensionality of the data while retaining most of the variance, which is useful for visualizing high-dimensional data and improving the efficiency of machine learning algorithms.
PCA works by identifying the directions (principal components) along which the variance in the data is maximized. By projecting the data onto these principal components, PCA can reduce the number of dimensions while preserving as much information as possible.
Here’s how to implement PCA using Scikit-learn:
from sklearn.decomposition import PCA
import numpy as np
# Example dataset
X = np.array([[2.5, 2.4], [0.5, 0.7], [2.2, 2.9],
[1.9, 2.2], [3.1, 3.0], [2.3, 2.7]])
# Applying PCA
pca = PCA(n_components=1)
X_reduced = pca.fit_transform(X)
print(X_reduced)
t-SNE for Data Visualization
t-Distributed Stochastic Neighbor Embedding (t-SNE) is a machine learning algorithm for visualization. It is a nonlinear dimensionality reduction technique well-suited for embedding high-dimensional data into a space of two or three dimensions, which can then be visualized in a scatter plot.
t-SNE is particularly effective for visualizing clusters or groups of data points in high-dimensional data. It reduces the dimensionality by modeling each high-dimensional object by a two- or three-dimensional point, capturing the structure of the data in a way that is visually meaningful.
Here’s how to implement t-SNE using Scikit-learn:
from sklearn.manifold import TSNE
import matplotlib.pyplot as plt
# Example dataset
X = np.array([[0, 0], [1, 1], [2, 2], [3, 3],
[8, 8], [9, 9], [10, 10]])
# Applying t-SNE
tsne = TSNE(n_components=2, random_state=0)
X_embedded = tsne.fit_transform(X)
# Plotting the result
plt.scatter(X_embedded[:, 0], X_embedded[:, 1])
plt.show()
Autoencoders for Nonlinear Dimensionality Reduction
Autoencoders are a type of artificial neural network used to learn efficient codings of unlabeled data. The aim of an autoencoder is to learn a representation (encoding) for a set of data, typically for dimensionality reduction, by training the network to ignore noise.
Autoencoders consist of two parts: an encoder that compresses the input into a latent-space representation, and a decoder that reconstructs the input from this representation. They are particularly powerful for nonlinear dimensionality reduction and can be used for tasks such as anomaly detection and denoising.
Here’s how to implement an autoencoder using Keras:
from keras.layers import Input, Dense
from keras.models import Model
import numpy as np
# Example dataset
X = np.array([[0., 0.], [0., 1.], [1., 0.], [1., 1.]])
# Defining the autoencoder
input_layer = Input(shape=(2,))
encoded = Dense(1, activation='relu')(input_layer)
decoded = Dense(2, activation='sigmoid')(encoded)
autoencoder = Model(input_layer, decoded)
autoencoder.compile(optimizer='adam', loss='binary_crossentropy')
# Training the autoencoder
autoencoder.fit(X, X, epochs=50, batch_size=1, shuffle=True)
# Encoding the data
encoder = Model(input_layer, encoded)
X_encoded = encoder.predict(X)
print(X_encoded)
Exploring these machine learning models provides a deep understanding of various techniques and their applications. From classification to clustering, and from regression to dimensionality reduction, each model offers unique capabilities for analyzing and interpreting data. By leveraging tools like Scikit-learn, Keras, and SciPy, you can implement these models effectively in your POC projects, driving innovation and delivering insightful results.
If you want to read more articles similar to Exploring Machine Learning Models for POC: A Comprehensive Guide, you can visit the Applications category.
You Must Read