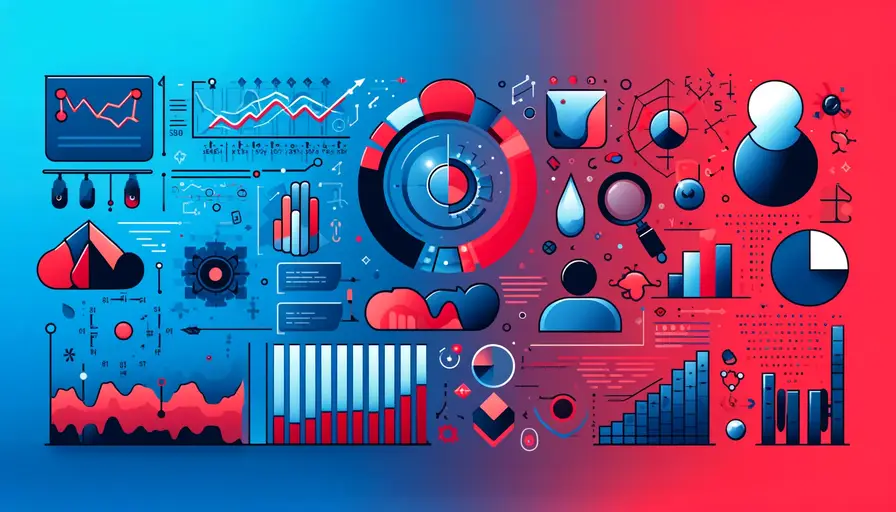
Interpreting Machine Learning Model Results: A Guide

- Understand the Purpose and Goals of the Machine Learning Model
- Examine the Accuracy and Performance Metrics of the Model
- Analyze the Feature Importance and Contribution of Variables in the Model
- Identify Any Biases or Limitations in the Model and Its Results
- Compare the Model Results with Domain Knowledge and Intuition
- Use Visualizations and Charts to Interpret the Model's Predictions
- Validate the Model's Results Through Cross-Validation or Hold-Out Testing
- Seek Feedback and Input from Domain Experts to Gain Additional Insights
- Document and Communicate the Interpretation of the Model Results Clearly
- Continuously Update and Refine the Interpretation as New Data Becomes Available
Understand the Purpose and Goals of the Machine Learning Model
Before diving into the interpretation of a machine learning model's results, it's crucial to clearly understand the purpose and goals of the model. This understanding forms the foundation for any analysis and ensures that the interpretations align with the model's intended use. For example, a model designed to predict customer churn will have different evaluation criteria compared to a model used for image recognition.
By defining the objectives upfront, you can tailor your analysis to focus on the most relevant aspects of the model's performance. This includes identifying key performance metrics, understanding the expected outcomes, and determining the impact of the model's predictions on business decisions. Clear objectives help in assessing whether the model meets the desired requirements and provides actionable insights.
Additionally, understanding the model's goals allows for better communication of results to stakeholders. It ensures that all interpretations and visualizations are aligned with the business context, making it easier for non-technical stakeholders to grasp the significance of the model's outputs.
Examine the Accuracy and Performance Metrics of the Model
Accuracy
Accuracy is one of the most straightforward metrics for evaluating a machine learning model. It measures the proportion of correctly predicted instances out of the total instances. High accuracy indicates that the model is performing well, but it may not always provide a complete picture, especially in cases of imbalanced datasets.

To calculate accuracy, use the following formula:
# Example: Calculating Accuracy
from sklearn.metrics import accuracy_score
y_true = [0, 1, 1, 0, 1, 0]
y_pred = [0, 1, 0, 0, 1, 1]
accuracy = accuracy_score(y_true, y_pred)
print("Accuracy:", accuracy)
Precision and Recall
Precision measures the proportion of true positive predictions out of all positive predictions made by the model. High precision indicates a low false positive rate. Recall (or Sensitivity) measures the proportion of true positive predictions out of all actual positives in the dataset. High recall indicates a low false negative rate.
Both metrics are crucial for understanding the balance between false positives and false negatives. They are particularly important in scenarios where the cost of false positives and false negatives is high, such as in medical diagnosis or fraud detection.
# Example: Calculating Precision and Recall
from sklearn.metrics import precision_score, recall_score
precision = precision_score(y_true, y_pred)
recall = recall_score(y_true, y_pred)
print("Precision:", precision)
print("Recall:", recall)
F1 Score
The F1 Score is the harmonic mean of precision and recall. It provides a single metric that balances both concerns, making it useful when you need a comprehensive evaluation of the model's performance. A high F1 Score indicates that the model has a good balance between precision and recall.

# Example: Calculating F1 Score
from sklearn.metrics import f1_score
f1 = f1_score(y_true, y_pred)
print("F1 Score:", f1)
Confusion Matrix
A Confusion Matrix provides a detailed breakdown of the model's performance by showing the counts of true positives, true negatives, false positives, and false negatives. It helps in identifying specific areas where the model is making errors and provides insights into the types of mistakes the model is making.
# Example: Confusion Matrix
from sklearn.metrics import confusion_matrix
import seaborn as sns
import matplotlib.pyplot as plt
conf_matrix = confusion_matrix(y_true, y_pred)
sns.heatmap(conf_matrix, annot=True, fmt='d', cmap='Blues')
plt.xlabel('Predicted')
plt.ylabel('Actual')
plt.title('Confusion Matrix')
plt.show()
Receiver Operating Characteristic (ROC) Curve
The ROC Curve plots the true positive rate against the false positive rate at various threshold settings. The Area Under the ROC Curve (AUC) provides a single measure of the model's ability to discriminate between positive and negative classes. A higher AUC indicates better performance.
# Example: ROC Curve and AUC
from sklearn.metrics import roc_curve, roc_auc_score
fpr, tpr, _ = roc_curve(y_true, y_pred)
auc = roc_auc_score(y_true, y_pred)
plt.plot(fpr, tpr, label=f'AUC = {auc:.2f}')
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('ROC Curve')
plt.legend()
plt.show()
Analyze the Feature Importance and Contribution of Variables in the Model
Understanding which features contribute the most to the model's predictions is crucial for interpretability. Feature importance can be determined using various techniques, such as feature coefficients in linear models or feature importance scores in tree-based models.
Feature Importance in Tree-Based Models
Tree-based models, like Random Forests and Gradient Boosting, provide built-in methods for evaluating feature importance. These methods indicate how much each feature contributes to the model's decision-making process.

# Example: Feature Importance in Random Forest
from sklearn.ensemble import RandomForestClassifier
model = RandomForestClassifier()
model.fit(X_train, y_train)
importances = model.feature_importances_
# Plot feature importances
plt.barh(range(len(importances)), importances)
plt.yticks(range(len(importances)), feature_names)
plt.xlabel('Importance')
plt.ylabel('Feature')
plt.title('Feature Importance')
plt.show()
SHAP Values
SHAP (SHapley Additive exPlanations) values provide a unified measure of feature importance, showing how each feature contributes to the model's predictions. SHAP values are particularly useful for understanding complex models.
# Example: SHAP Values
import shap
explainer = shap.TreeExplainer(model)
shap_values = explainer.shap_values(X_test)
# Plot SHAP summary plot
shap.summary_plot(shap_values, X_test, feature_names=feature_names)
LIME
LIME (Local Interpretable Model-agnostic Explanations) provides explanations for individual predictions by approximating the model locally with an interpretable model. LIME helps in understanding the model's behavior for specific instances.
# Example: LIME Explanation
import lime
import lime.lime_tabular
explainer = lime.lime_tabular.LimeTabularExplainer(X_train, feature_names=feature_names, class_names=['class0', 'class1'], discretize_continuous=True)
exp = explainer.explain_instance(X_test[0], model.predict_proba, num_features=5)
# Show explanation
exp.show_in_notebook(show_table=True)
Identify Any Biases or Limitations in the Model and Its Results
Data Bias
Bias in the data can lead to biased model predictions. It is essential to examine the training data for any inherent biases, such as underrepresentation of certain groups or overemphasis on specific features. Addressing data bias involves ensuring a representative and balanced dataset.
Feature Importance
Understanding which features the model relies on can help identify potential biases. For example, if the model places too much importance on a particular feature, it could indicate a bias towards that feature. Techniques like SHAP and LIME can help in assessing feature importance.

Model Interpretability
Model interpretability is crucial for identifying biases and understanding model limitations. Interpretable models like linear regression or decision trees provide clear insights into how predictions are made. Complex models may require additional tools like SHAP or LIME for interpretation.
Compare the Model Results with Domain Knowledge and Intuition
Domain Knowledge Integration
Comparing model results with domain knowledge ensures that the model's predictions make sense within the context of the application. Domain experts can provide valuable insights into whether the model is capturing the right patterns and making reasonable predictions.
Intuition Checks
Using intuition checks involves validating the model's predictions with real-world scenarios. For example, if a model predicts high sales for a product in a region where sales have historically been low, it may warrant a closer examination of the model's reasoning.
Feedback from Experts
Gathering feedback from domain experts helps in refining the model and improving its accuracy. Experts can identify any discrepancies between the model's predictions and their expectations, leading to further model improvements.

Use Visualizations and Charts to Interpret the Model's Predictions
Scatter Plots
Scatter plots are useful for visualizing the relationship between two variables and understanding how predictions vary with changes in input features. They help in identifying patterns, outliers, and correlations in the data.
# Example: Scatter Plot
plt.scatter(X_test['feature1'], y_test, label='Actual')
plt.scatter(X_test['feature1'], y_pred, label='Predicted')
plt.xlabel('Feature 1')
plt.ylabel('Target')
plt.title('Scatter Plot of Feature 1 vs Target')
plt.legend()
plt.show()
Line Charts
Line charts are effective for visualizing trends and changes over time. They are particularly useful for time series data and help in understanding how predictions evolve with time.
# Example: Line Chart
plt.plot(time_points, actual_values, label='Actual')
plt.plot(time_points, predicted_values, label='Predicted')
plt.xlabel('Time')
plt.ylabel('Value')
plt.title('Line Chart of Actual vs Predicted Values')
plt.legend()
plt.show()
Bar Charts
Bar charts help in comparing categorical data and understanding the distribution of predictions across different categories. They provide a clear visual representation of how the model performs across various groups.
# Example: Bar Chart
categories = ['cat1', 'cat2', 'cat3']
actual_counts = [50, 30
, 20]
predicted_counts = [45, 35, 20]
x = range(len(categories))
plt.bar(x, actual_counts, width=0.4, label='Actual', align='center')
plt.bar(x, predicted_counts, width=0.4, label='Predicted', align='edge')
plt.xlabel('Categories')
plt.ylabel('Count')
plt.title('Bar Chart of Actual vs Predicted Counts')
plt.legend()
plt.show()
Heatmaps
Heatmaps provide a visual representation of data density and correlations between variables. They are particularly useful for understanding the relationship between multiple features and the target variable.

# Example: Heatmap
import seaborn as sns
corr_matrix = X_test.corr()
sns.heatmap(corr_matrix, annot=True, cmap='coolwarm')
plt.title('Heatmap of Feature Correlations')
plt.show()
Validate the Model's Results Through Cross-Validation or Hold-Out Testing
Cross-Validation
Cross-validation is a technique for assessing how the results of a model will generalize to an independent dataset. It involves partitioning the data into multiple subsets, training the model on some subsets, and validating it on the remaining subsets. This process is repeated several times to ensure a robust evaluation.
# Example: Cross-Validation
from sklearn.model_selection import cross_val_score
model = RandomForestClassifier()
scores = cross_val_score(model, X, y, cv=5)
print("Cross-Validation Scores:", scores)
print("Mean Score:", scores.mean())
Hold-Out Testing
Hold-out testing involves splitting the dataset into a training set and a testing set. The model is trained on the training set and validated on the testing set. This method provides a straightforward evaluation of model performance on unseen data.
# Example: Hold-Out Testing
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
print("Test Accuracy:", accuracy_score(y_test, y_pred))
Seek Feedback and Input from Domain Experts to Gain Additional Insights
Collaboration with Experts
Collaboration with domain experts is crucial for gaining deeper insights into the model's performance and its real-world applicability. Experts can provide valuable feedback on the model's predictions and suggest improvements based on their experience.
Expert Reviews
Expert reviews involve presenting the model's results to domain experts and gathering their feedback. This process helps in identifying any discrepancies and ensures that the model's predictions are aligned with domain knowledge.
Continuous Improvement
Continuous improvement is achieved by incorporating feedback from experts into the model development process. Regular interactions with experts ensure that the model evolves to meet the needs of the application and remains accurate over time.
Document and Communicate the Interpretation of the Model Results Clearly
Clear Explanation of the Model's Purpose
Providing a clear explanation of the model's purpose helps stakeholders understand the context and objectives of the analysis. This includes describing the problem the model addresses and the expected outcomes.
Description of Input Variables
A detailed description of input variables and their significance is essential for interpreting the model's results. This includes explaining how each variable influences the predictions and its relevance to the problem at hand.
Explanation of Performance Metrics
Explaining the performance metrics used to evaluate the model ensures that stakeholders understand the model's effectiveness. This includes discussing accuracy, precision, recall, and other relevant metrics.
Continuously Update and Refine the Interpretation as New Data Becomes Available
Regular Updates
Regularly updating the model and its interpretations ensures that the analysis remains relevant and accurate. This involves incorporating new data, retraining the model, and reassessing its performance.
Refinement of Interpretation
Refining the interpretation based on new data and feedback helps in maintaining the model's accuracy. Continuous improvements and adjustments ensure that the model adapts to changing data patterns.
Ongoing Monitoring
Ongoing monitoring of the model's performance is essential for identifying any issues early. Regular evaluations help in maintaining the model's accuracy and reliability over time.
If you want to read more articles similar to Interpreting Machine Learning Model Results: A Guide, you can visit the Performance category.
You Must Read