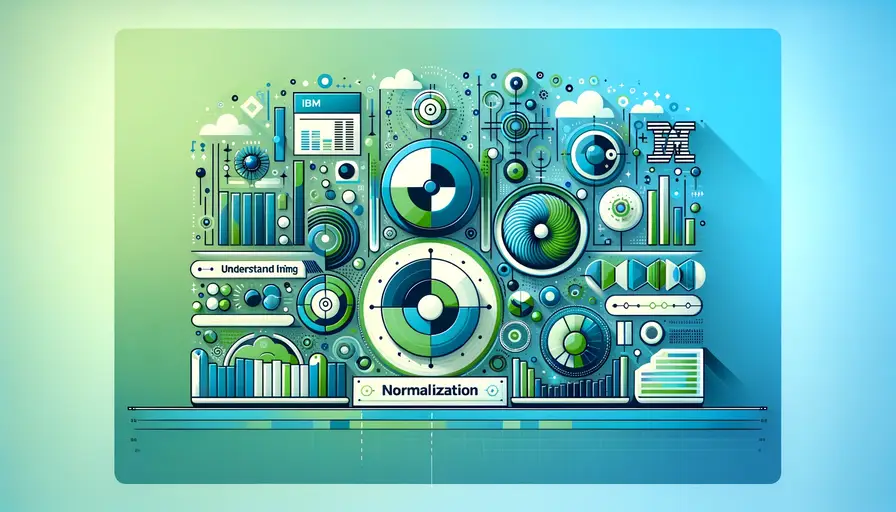
Java Machine Learning Projects: A Comprehensive Guide

Java has long been a popular programming language, known for its versatility, robustness, and extensive libraries. In recent years, Java has also become a powerful tool for developing machine learning (ML) projects. This comprehensive guide explores various Java machine learning projects, highlighting important techniques, applications, and implementation strategies. We will delve into several key areas, providing detailed explanations and code examples to help you get started with ML in Java.
Setting Up Your Java Environment for Machine Learning
Choosing the Right Libraries
Choosing the right libraries is crucial for developing effective machine learning projects in Java. Several libraries offer powerful functionalities for building and deploying ML models. Some of the most popular Java libraries for machine learning include Weka, Deeplearning4j, and Apache Spark MLlib.
Weka is a comprehensive suite of machine learning algorithms for data mining tasks. It provides tools for data preprocessing, classification, regression, clustering, and association rules, along with a user-friendly graphical interface. Weka is widely used for academic and research purposes due to its ease of use and extensive documentation.
Deeplearning4j (DL4J) is a robust, open-source, distributed deep learning library for the JVM. It supports various neural network architectures and provides integrations with popular frameworks like Apache Spark and Hadoop. DL4J is suitable for both research and production environments, offering scalability and performance optimization.

Apache Spark MLlib is a scalable machine learning library built on top of Apache Spark. It provides a rich set of algorithms and tools for classification, regression, clustering, collaborative filtering, and more. MLlib leverages the power of distributed computing, making it ideal for processing large datasets.
Setting Up Your Development Environment
Setting up your development environment is the first step to start working on Java machine learning projects. You need to install Java Development Kit (JDK), an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse, and the required libraries for your project.
First, download and install the latest version of the JDK from the official Oracle website. Ensure that the JDK is properly configured in your system’s environment variables.
Next, choose an IDE like IntelliJ IDEA or Eclipse. Both IDEs offer robust features for Java development, including code completion, debugging, and integrated version control.

Finally, add the necessary libraries to your project. For instance, if you are using Weka, download the Weka library and add it to your project’s build path. If you prefer using Maven or Gradle, you can add the dependencies directly to your pom.xml
or build.gradle
file.
Basic Example: Linear Regression with Weka
To illustrate the setup and usage of a machine learning library in Java, let’s consider a basic example of linear regression using Weka. Linear regression is a simple yet powerful algorithm for predicting a continuous target variable based on one or more predictor variables.
Here’s an example of implementing linear regression with Weka:
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
import weka.classifiers.functions.LinearRegression;
public class LinearRegressionExample {
public static void main(String[] args) {
try {
// Load dataset
DataSource source = new DataSource("data/housing.arff");
Instances dataset = source.getDataSet();
// Set target variable (last attribute)
dataset.setClassIndex(dataset.numAttributes() - 1);
// Build linear regression model
LinearRegression model = new LinearRegression();
model.buildClassifier(dataset);
// Output model
System.out.println(model);
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, we load a dataset in ARFF format, set the target variable, and build a linear regression model using Weka’s LinearRegression class. The model is then printed to the console, showing the coefficients for each predictor variable.

Data Preprocessing and Feature Engineering
Data Cleaning and Transformation
Data cleaning and transformation are essential steps in preparing your data for machine learning. Raw data often contains missing values, outliers, and inconsistencies that need to be addressed before building a model. In Java, you can use libraries like Weka and Apache Commons CSV to clean and transform your data.
Weka provides several filters for data cleaning, including ReplaceMissingValues, RemoveOutliers, and Normalize. These filters can be applied to your dataset to handle missing values, remove outliers, and normalize features.
Here’s an example of using Weka to clean and transform data:
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
import weka.filters.Filter;
import weka.filters.unsupervised.attribute.ReplaceMissingValues;
import weka.filters.unsupervised.instance.RemoveWithValues;
public class DataPreprocessingExample {
public static void main(String[] args) {
try {
// Load dataset
DataSource source = new DataSource("data/housing.arff");
Instances dataset = source.getDataSet();
// Replace missing values
ReplaceMissingValues replaceMissing = new ReplaceMissingValues();
replaceMissing.setInputFormat(dataset);
Instances cleanDataset = Filter.useFilter(dataset, replaceMissing);
// Remove instances with outliers (e.g., remove instances where attribute 2 > 100)
RemoveWithValues removeOutliers = new RemoveWithValues();
removeOutliers.setAttributeIndex("2");
removeOutliers.setNominalIndices("100-");
removeOutliers.setInputFormat(cleanDataset);
Instances finalDataset = Filter.useFilter(cleanDataset, removeOutliers);
// Output cleaned dataset
System.out.println(finalDataset);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Feature Selection and Extraction
Feature selection and extraction are crucial for improving the performance of machine learning models. Feature selection involves identifying the most relevant features for your model, while feature extraction involves creating new features from existing ones.

Weka provides several methods for feature selection, including InformationGainAttributeEval, GainRatioAttributeEval, and PrincipalComponents. These methods can be used to evaluate and select the most important features for your model.
Feature extraction techniques like Principal Component Analysis (PCA) can be used to reduce the dimensionality of your dataset, creating new features that capture the most important information.
Here’s an example of using Weka for feature selection and extraction:
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
import weka.attributeSelection.AttributeSelection;
import weka.attributeSelection.InfoGainAttributeEval;
import weka.attributeSelection.Ranker;
import weka.filters.Filter;
import weka.filters.unsupervised.attribute.PrincipalComponents;
public class FeatureSelectionExample {
public static void main(String[] args) {
try {
// Load dataset
DataSource source = new DataSource("data/housing.arff");
Instances dataset = source.getDataSet();
dataset.setClassIndex(dataset.numAttributes() - 1);
// Feature selection using Information Gain
AttributeSelection selector = new AttributeSelection();
InfoGainAttributeEval evaluator = new InfoGainAttributeEval();
Ranker ranker = new Ranker();
selector.setEvaluator(evaluator);
selector.setSearch(ranker);
selector.SelectAttributes(dataset);
// Output selected features
System.out.println(selector.toResultsString());
// Feature extraction using PCA
PrincipalComponents pca = new PrincipalComponents();
pca.setInputFormat(dataset);
Instances transformedDataset = Filter.useFilter(dataset, pca);
// Output transformed dataset
System.out.println(transformedDataset);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Handling Imbalanced Data
Handling imbalanced data is critical in machine learning projects, especially in classification tasks. Imbalanced data occurs when one class is significantly underrepresented compared to others, leading to biased models that favor the majority class.

Several techniques can be used to address imbalanced data, including resampling methods (oversampling and undersampling) and algorithmic approaches (cost-sensitive learning and ensemble methods). Weka provides tools for handling imbalanced data, such as the SMOTE filter for oversampling and the Resample filter for undersampling.
Here’s an example of using Weka to handle imbalanced data:
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
import weka.filters.Filter;
import weka.filters.supervised.instance.SMOTE;
public class ImbalancedDataExample {
public static void main(String[] args) {
try {
// Load dataset
DataSource source = new DataSource("data/imbalanced.arff");
Instances dataset = source.getDataSet();
dataset.setClassIndex(dataset.numAttributes() - 1);
// Apply SMOTE to oversample the minority class
SMOTE smote = new SMOTE();
smote.setInputFormat(dataset);
Instances balancedDataset = Filter.useFilter(dataset, smote);
// Output balanced dataset
System.out.println(balancedDataset);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Building and Evaluating Machine Learning Models
Classification Algorithms
Classification algorithms are used to predict categorical outcomes based on input features. Java provides several powerful libraries for building classification models, including Weka, Deeplearning4j, and Apache Spark MLlib.
Some popular classification algorithms include decision trees, support vector machines (SVM), and neural networks. These algorithms can be used to solve various classification tasks, such as spam detection, fraud detection, and image recognition.

Here’s an example of
using Weka to build a decision tree classifier:
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
import weka.classifiers.trees.J48;
import weka.classifiers.Evaluation;
public class ClassificationExample {
public static void main(String[] args) {
try {
// Load dataset
DataSource source = new DataSource("data/spam.arff");
Instances dataset = source.getDataSet();
dataset.setClassIndex(dataset.numAttributes() - 1);
// Build decision tree classifier
J48 tree = new J48();
tree.buildClassifier(dataset);
// Evaluate model
Evaluation eval = new Evaluation(dataset);
eval.crossValidateModel(tree, dataset, 10, new Random(1));
// Output evaluation results
System.out.println(eval.toSummaryString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Regression Algorithms
Regression algorithms are used to predict continuous outcomes based on input features. Java libraries like Weka, Deeplearning4j, and Apache Spark MLlib provide various regression algorithms for building predictive models.
Linear regression, polynomial regression, and neural networks are commonly used for regression tasks. These algorithms can be applied to problems such as house price prediction, stock price forecasting, and demand estimation.
Here’s an example of using Weka to build a linear regression model:
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
import weka.classifiers.functions.LinearRegression;
import weka.classifiers.Evaluation;
public class RegressionExample {
public static void main(String[] args) {
try {
// Load dataset
DataSource source = new DataSource("data/housing.arff");
Instances dataset = source.getDataSet();
dataset.setClassIndex(dataset.numAttributes() - 1);
// Build linear regression model
LinearRegression model = new LinearRegression();
model.buildClassifier(dataset);
// Evaluate model
Evaluation eval = new Evaluation(dataset);
eval.crossValidateModel(model, dataset, 10, new Random(1));
// Output evaluation results
System.out.println(eval.toSummaryString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Clustering Algorithms
Clustering algorithms group similar data points together based on their features. These algorithms are useful for tasks such as customer segmentation, anomaly detection, and image segmentation. Java libraries like Weka, Deeplearning4j, and Apache Spark MLlib provide powerful clustering algorithms for various applications.
K-means clustering, hierarchical clustering, and DBSCAN are popular clustering algorithms. K-means clustering is widely used for its simplicity and efficiency, while hierarchical clustering provides a tree-like structure of clusters.
Here’s an example of using Weka to perform K-means clustering:
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
import weka.clusterers.SimpleKMeans;
public class ClusteringExample {
public static void main(String[] args) {
try {
// Load dataset
DataSource source = new DataSource("data/customers.arff");
Instances dataset = source.getDataSet();
// Perform K-means clustering
SimpleKMeans kMeans = new SimpleKMeans();
kMeans.setNumClusters(3);
kMeans.buildClusterer(dataset);
// Output cluster centroids
System.out.println(kMeans);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Advanced Topics in Java Machine Learning
Deep Learning with Deeplearning4j
Deep learning is a subset of machine learning that uses neural networks with multiple layers to learn from large amounts of data. Deeplearning4j (DL4J) is a powerful Java library for building and deploying deep learning models. DL4J supports various neural network architectures, including feedforward networks, convolutional networks (CNNs), and recurrent networks (RNNs).
Deep learning is used in applications such as image recognition, natural language processing, and recommendation systems. DL4J provides tools for training, evaluating, and deploying deep learning models, making it suitable for both research and production environments.
Here’s an example of building a simple feedforward neural network with Deeplearning4j:
import org.deeplearning4j.nn.multilayer.MultiLayerNetwork;
import org.deeplearning4j.nn.conf.NeuralNetConfiguration;
import org.deeplearning4j.nn.conf.layers.DenseLayer;
import org.deeplearning4j.nn.conf.layers.OutputLayer;
import org.deeplearning4j.optimize.listeners.ScoreIterationListener;
import org.nd4j.linalg.activations.Activation;
import org.nd4j.linalg.api.ndarray.INDArray;
import org.nd4j.linalg.dataset.DataSet;
import org.nd4j.linalg.dataset.api.iterator.DataSetIterator;
import org.nd4j.linalg.dataset.api.iterator.impl.ListDataSetIterator;
import org.nd4j.linalg.factory.Nd4j;
import org.nd4j.linalg.learning.config.Adam;
import org.nd4j.linalg.lossfunctions.LossFunctions;
import java.util.Arrays;
import java.util.List;
public class DeepLearningExample {
public static void main(String[] args) {
// Define the network configuration
NeuralNetConfiguration.Builder builder = new NeuralNetConfiguration.Builder();
builder.updater(new Adam(0.001));
NeuralNetConfiguration.ListBuilder listBuilder = builder.list();
listBuilder.layer(0, new DenseLayer.Builder().nIn(2).nOut(3).activation(Activation.RELU).build());
listBuilder.layer(1, new OutputLayer.Builder(LossFunctions.LossFunction.MSE).activation(Activation.IDENTITY)nIn(3).nOut(1).build());
MultiLayerNetwork network = new MultiLayerNetwork(listBuilder.build());
network.init();
// Generate synthetic data
INDArray input = Nd4j.create(new double[][]{{1, 1}, {0, 1}, {1, 0}, {0, 0}});
INDArray output = Nd4j.create(new double[][]{{1}, {0}, {0}, {0}});
DataSet dataSet = new DataSet(input, output);
List<DataSet> dataSetList = Arrays.asList(dataSet);
DataSetIterator iterator = new ListDataSetIterator<>(dataSetList, 1);
// Train the network
network.setListeners(new ScoreIterationListener(10));
for (int i = 0; i < 1000; i++) {
iterator.reset();
network.fit(iterator);
}
// Test the network
INDArray testInput = Nd4j.create(new double[][]{{1, 1}, {0, 1}, {1, 0}, {0, 0}});
INDArray testOutput = network.output(testInput);
System.out.println(testOutput);
}
}
Big Data and Machine Learning with Apache Spark
Big data refers to large and complex datasets that require advanced processing techniques. Apache Spark is a powerful big data processing framework that provides libraries for machine learning through MLlib. Spark MLlib offers scalable and efficient algorithms for classification, regression, clustering, and collaborative filtering.
Integrating machine learning with big data allows for processing and analyzing massive datasets, uncovering valuable insights and making accurate predictions. Spark MLlib leverages distributed computing to handle large-scale data, making it ideal for big data applications.
Here’s an example of using Apache Spark MLlib for classification:
import org.apache.spark.SparkConf;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.mllib.classification.LogisticRegressionModel;
import org.apache.spark.mllib.classification.LogisticRegressionWithLBFGS;
import org.apache.spark.mllib.regression.LabeledPoint;
import org.apache.spark.mllib.util.MLUtils;
public class SparkMLExample {
public static void main(String[] args) {
// Initialize Spark context
SparkConf conf = new SparkConf().setAppName("SparkMLExample").setMaster("local");
JavaSparkContext sc = new JavaSparkContext(conf);
// Load and parse data
String path = "data/sample_libsvm_data.txt";
JavaRDD<LabeledPoint> data = MLUtils.loadLibSVMFile(sc.sc(), path).toJavaRDD();
// Split data into training and test sets
JavaRDD<LabeledPoint> training = data.sample(false, 0.6, 11L);
JavaRDD<LabeledPoint> test = data.subtract(training);
// Train a logistic regression model
LogisticRegressionModel model = new LogisticRegressionWithLBFGS().setNumClasses(2).run(training.rdd());
// Evaluate model on test data
JavaRDD<Double> prediction = model.predict(test.map(LabeledPoint::features));
System.out.println("Predictions: " + prediction.collect());
// Stop Spark context
sc.stop();
}
}
Reinforcement Learning in Java
Reinforcement learning (RL) is a type of machine learning where an agent learns to make decisions by interacting with an environment. RL algorithms are used in applications such as robotics, game playing, and autonomous systems. Java provides several libraries for implementing reinforcement learning, including RL4J (part of Deeplearning4j) and Malmo (a platform for AI experimentation using Minecraft).
Reinforcement learning involves defining states, actions, and rewards, and using algorithms like Q-learning and policy gradients to learn optimal policies. RL4J provides tools for building and training reinforcement learning models, while Malmo offers a rich environment for experimenting with RL algorithms.
Here’s an example of implementing Q-learning with RL4J:
import org.deeplearning4j.rl4j.learning.sync.qlearning.QLearningDiscreteDense;
import org.deeplearning4j.rl4j.mdp.MDP;
import org.deeplearning4j.rl4j.mdp.toy.Maze;
import org.deeplearning4j.rl4j.network.dqn.DQNFactoryStdDense;
import org.deeplearning4j.rl4j.policy.DQNPolicy;
import org.deeplearning4j.rl4j.util.DataManager;
public class ReinforcementLearningExample {
public static void main(String[] args) throws Exception {
// Initialize DataManager
DataManager manager = new DataManager();
// Define MDP (Maze environment)
MDP mdp = new Maze();
// Define Q-learning configuration
QLearningDiscreteDense.Configuration conf = QLearningDiscreteDense.Configuration.builder()
.seed(123)
.maxEpochStep(200)
.maxStep(15000)
.expRepMaxSize(15000)
.batchSize(32)
.targetDqnUpdateFreq(500)
.updateStart(10)
.rewardFactor(1.0)
.gamma(0.99)
.errorClamp(1.0)
.minEpsilon(0.1f)
.epsilonNbStep(1000)
.build();
// Define neural network configuration
DQNFactoryStdDense.Configuration netConf = DQNFactoryStdDense.Configuration.builder()
.l2(0.001)
.updater(new Adam(0.0005))
.numHiddenNodes(64)
.numLayers(3)
.build();
// Create Q-learning agent
QLearningDiscreteDense<Maze.MazeState> dql = new QLearningDiscreteDense<>(mdp, netConf, conf, manager);
// Train agent
dql.train();
// Save trained model
DQNPolicy<Maze.MazeState> policy = dql.getPolicy();
policy.save("maze_policy");
// Close environment
mdp.close();
}
}
Java is a powerful and versatile language for developing machine learning projects. With libraries like Weka, Deeplearning4j, and Apache Spark MLlib, you can build and deploy robust ML models for various applications. By setting up your development environment, preprocessing data, building and evaluating models, and exploring advanced topics like deep learning and reinforcement learning, you can leverage Java's capabilities to create innovative machine learning solutions. Using tools like Google and Kaggle, you can access additional resources and datasets to further enhance your projects.
If you want to read more articles similar to Java Machine Learning Projects: A Comprehensive Guide, you can visit the Artificial Intelligence category.
You Must Read