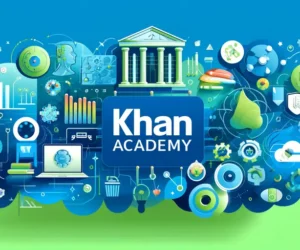
Leading AI and Machine Learning Frameworks

The field of AI and machine learning (ML) is rapidly evolving, supported by powerful frameworks that make developing, training, and deploying models more accessible and efficient. This guide explores some of the leading AI and ML frameworks, highlighting their key features and use cases.
TensorFlow
TensorFlow is an open-source machine learning framework developed by Google. It is widely used for both research and production purposes due to its comprehensive and flexible ecosystem. TensorFlow supports a range of applications from image and speech recognition to language processing and predictive analytics.
One of the primary strengths of TensorFlow is its scalability. It can run on a single CPU or GPU as well as on large-scale distributed systems. TensorFlow provides tools for building and deploying ML models across various platforms, including mobile and embedded devices. Additionally, TensorFlow’s high-level APIs, such as Keras, make it user-friendly for beginners while still being powerful for advanced users.
import tensorflow as tf
# Define a simple sequential model
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(128, activation='relu', input_shape=(784,)),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Print model summary
model.summary()
PyTorch
PyTorch, developed by Facebook’s AI Research lab, is another leading framework that has gained significant popularity, particularly in the research community. PyTorch is known for its dynamic computational graph, which allows for more flexibility and ease of use compared to static graph frameworks.
Choosing the Best Cloud Machine Learning Platform for Your NeedsKey Features of PyTorch:
Key features of PyTorch include its intuitive interface, dynamic computation graph, and strong support for GPU acceleration. PyTorch's native support for Python makes it easier for developers to integrate into their existing Python codebases. Additionally, its extensive library of pre-trained models and utilities accelerates development and experimentation.
PyTorch is widely used in academia and industry for various applications, including computer vision, natural language processing, and reinforcement learning. Its seamless integration with Python allows for easy debugging and rapid prototyping, making it a favorite among researchers and developers alike.
import torch
import torch.nn as nn
import torch.optim as optim
# Define a simple neural network
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(784, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Instantiate the model, define loss and optimizer
model = Net()
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
Scikit-learn
Scikit-learn is a versatile machine learning library built on top of SciPy and NumPy. It is designed for classical ML algorithms and is widely used for tasks such as classification, regression, clustering, and dimensionality reduction.
Key Features:
Key features of Scikit-learn include its simplicity and ease of use, comprehensive documentation, and a wide range of well-implemented algorithms. It provides tools for data preprocessing, model selection, and evaluation, making it a one-stop solution for most ML workflows.
Best Deep Learning Software for NVIDIA GPUs: A Complete GuideScikit-learn's modular design allows users to easily combine different algorithms and utilities to build custom workflows. It is particularly popular in academia and industry for educational purposes and quick prototyping of ML models.
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load dataset
iris = datasets.load_iris()
X, y = iris.data, iris.target
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train a RandomForestClassifier
clf = RandomForestClassifier(n_estimators=100)
clf.fit(X_train, y_train)
# Make predictions and evaluate
y_pred = clf.predict(X_test)
print(f'Accuracy: {accuracy_score(y_test, y_pred)}')
Caffe
Caffe (Convolutional Architecture for Fast Feature Embedding) is a deep learning framework developed by the Berkeley Vision and Learning Center (BVLC). It is known for its speed and efficiency, particularly in image classification and convolutional neural networks (CNNs).
Caffe's main advantages are its performance and scalability. It can process over 60 million images per day on a single GPU. Caffe is used extensively in academic research projects and industrial applications, particularly those involving image and video processing.
However, Caffe's static graph architecture can be less flexible compared to dynamic graph frameworks like PyTorch. It is often favored for production deployment where performance and speed are critical.
Quantum Machine Learning Tools for Advanced Data AnalysisThe Microsoft Cognitive Toolkit (CNTK)
The Microsoft Cognitive Toolkit (CNTK) is an open-source deep learning framework developed by Microsoft. It is designed for performance and scalability, supporting both traditional ML and deep learning.
Key Features of the Microsoft Cognitive Toolkit:
Key features of CNTK include its efficient implementation of training algorithms, support for multi-GPU and distributed training, and a flexible architecture that allows users to combine different models and algorithms easily.
CNTK excels in handling large datasets and complex models, making it suitable for enterprise-level applications. It is often used for speech recognition, image recognition, and natural language processing tasks.
Keras
Keras is a high-level neural networks API, written in Python and capable of running on top of TensorFlow, CNTK, or Theano. It is designed to enable fast experimentation and easy-to-use deep learning models.
Is CML the Ultimate Solution for Machine Learning Pipelines?Key Features of Keras:
Key features of Keras include its user-friendly API, modularity, and extensibility. Keras simplifies the process of building and training deep learning models, making it accessible to beginners and experienced practitioners alike.
Keras is particularly known for its simplicity and ease of use, enabling quick prototyping and experimentation. It abstracts the complexities of backend engines, allowing users to focus on building and refining their models.
from keras.models import Sequential
from keras.layers import Dense
# Define a simple neural network
model = Sequential()
model.add(Dense(128, activation='relu', input_shape=(784,)))
model.add(Dense(10, activation='softmax'))
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Print model summary
model.summary()
MXNet
MXNet is a deep learning framework developed by Apache, known for its efficiency and scalability. It supports both symbolic and imperative programming, providing flexibility for model development and deployment.
MXNet is designed to scale across multiple GPUs and machines, making it suitable for large-scale deep learning projects. It supports a wide range of programming languages, including Python, Scala, and Julia, catering to a diverse set of developers and researchers.
Comparing On-Premise vs Cloud for ML Model DeploymentThe framework's flexibility and performance make it a popular choice for both academic research and industrial applications. MXNet is often used in areas such as computer vision, natural language processing, and reinforcement learning.
Theano
Theano is one of the oldest deep learning frameworks, developed by the Montreal Institute for Learning Algorithms (MILA). Although now deprecated, Theano played a crucial role in the development of other frameworks like Keras and TensorFlow.
Key Features of Theano:
Key features of Theano include its ability to define, optimize, and evaluate mathematical expressions involving multi-dimensional arrays efficiently. It was designed to handle the heavy lifting of numerical computations, allowing researchers to focus on building models.
Use Cases:
Use cases of Theano included research and development in deep learning, particularly for tasks requiring extensive mathematical computations. Despite its deprecation, Theano's legacy lives on through frameworks that built upon its innovations.
Machine Learning Studio vs. Service: Which is Better?Torch
Torch is an open-source machine learning library that offers a wide range of algorithms for deep learning. It provides a flexible and efficient platform for building complex models and is particularly popular in the research community.
Torch's main strengths are its flexibility and speed, thanks to its underlying LuaJIT engine. It supports dynamic graph construction, which allows for more flexible and interactive model development. Torch has been used extensively in projects involving computer vision, natural language processing, and reinforcement learning.
Despite being largely supplanted by PyTorch, Torch remains an important part of the deep learning landscape, particularly in legacy projects and research.
IBM Watson
IBM Watson is a powerful AI platform that offers a suite of tools and services for building and deploying machine learning models. Watson leverages advanced AI technologies to provide solutions for various industries, including healthcare, finance, and customer service.
Key Features of IBM Watson:
Key features of IBM Watson include its natural language processing capabilities, machine learning tools, and pre-trained models that can be easily integrated into applications. Watson provides APIs for text analysis, speech recognition, and visual recognition, among other services.
IBM Watson is known for its ability to handle unstructured data, making it ideal for applications that require advanced text and speech analytics. Its robust infrastructure and integration capabilities make it a popular choice for enterprise-level AI solutions.
The choice of a machine learning framework depends on various factors such as the specific application, required scalability, ease of use, and community support. Each framework has its strengths and unique features that cater to different needs and preferences. By understanding these frameworks, practitioners can select the best tools to enhance their AI and ML projects.
If you want to read more articles similar to Leading AI and Machine Learning Frameworks, you can visit the Tools category.
You Must Read