
Leveraging Machine Learning: Unlocking the Benefits for Developers
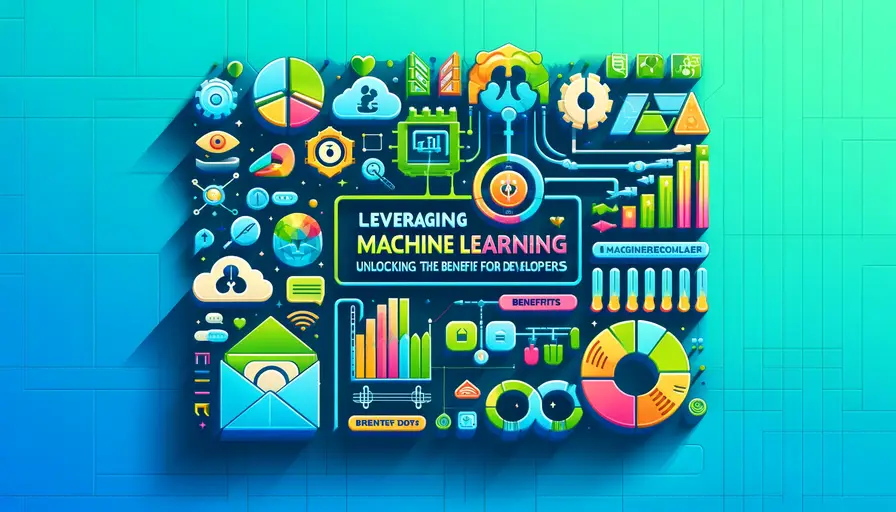
Machine learning (ML) is transforming how developers approach problem-solving, enabling them to create smarter applications and more efficient systems. By integrating ML into their workflows, developers can unlock new capabilities, optimize processes, and deliver more intelligent user experiences. This article explores the myriad benefits of leveraging machine learning for developers, detailing key concepts, practical implementations, and the tools necessary to succeed in this dynamic field.
Enhancing Development Efficiency with Machine Learning
Automating Repetitive Tasks
Automating repetitive tasks is one of the primary advantages of incorporating machine learning into development workflows. ML algorithms can handle mundane tasks, freeing developers to focus on more complex and creative aspects of their projects. For example, automated code reviews can identify potential bugs and suggest improvements, ensuring higher code quality and reducing the time spent on manual reviews.
By using tools like DeepCode and Codacy, developers can leverage machine learning to automatically analyze codebases, detect issues, and recommend fixes. These tools use ML models trained on vast amounts of code to identify patterns and common errors, helping developers maintain clean and efficient code.
Moreover, machine learning can automate testing processes. Tools like Testim use ML to create, execute, and maintain tests. They adapt to changes in the codebase, ensuring that test cases remain relevant and effective. This reduces the burden of manual testing and enhances the overall development process.
Top Techniques for Machine Learning-based Size RecognitionHere’s an example of using a simple script to automate code analysis with Python:
import pylint.lint
# Define the code to be analyzed
code = """
def add(a, b):
return a + b
print(add(2, 3))
"""
# Save the code to a temporary file
with open('temp_code.py', 'w') as file:
file.write(code)
# Run pylint on the temporary file
pylint_opts = ['temp_code.py']
pylint.lint.Run(pylint_opts)
Improving Code Quality
Improving code quality is another significant benefit of leveraging machine learning in development. ML models can analyze code to ensure adherence to best practices, detect security vulnerabilities, and optimize performance. This proactive approach helps maintain high standards of code quality and reduces the likelihood of introducing bugs.
Tools like SonarQube and Semgrep use machine learning to provide real-time feedback on code quality. These tools scan codebases for issues related to syntax, logic, and security. They also offer actionable insights and recommendations for improvement, enabling developers to address potential problems early in the development cycle.
Machine learning models can also assist in refactoring code by suggesting more efficient algorithms and data structures. This can lead to better performance and maintainability, ensuring that applications run smoothly and are easier to update. By incorporating ML into their development workflows, developers can produce high-quality code that meets industry standards and performs optimally.
Exploring AI Robots Utilizing Deep Learning TechnologyHere’s an example of using a code quality tool with Python:
import subprocess
# Define the code to be analyzed
code = """
def multiply(a, b):
return a * b
print(multiply(2, 3))
"""
# Save the code to a temporary file
with open('temp_code.py', 'w') as file:
file.write(code)
# Run SonarQube analysis on the temporary file
subprocess.run(['sonar-scanner', '-Dsonar.projectKey=my_project', '-Dsonar.sources=.'])
Enhancing Debugging and Error Handling
Enhancing debugging and error handling through machine learning involves using predictive models to identify and resolve issues before they escalate. ML models can analyze logs, trace errors, and predict potential failures based on historical data. This predictive capability helps developers address problems proactively, reducing downtime and improving system reliability.
By integrating tools like Sentry and New Relic, developers can leverage machine learning to monitor applications in real-time, detect anomalies, and automatically resolve issues. These tools use ML algorithms to identify patterns in error logs and performance metrics, providing insights into the root causes of issues.
Additionally, machine learning can assist in debugging by suggesting potential fixes based on similar past issues. This accelerates the debugging process and helps developers quickly implement effective solutions. By incorporating ML into their error handling workflows, developers can enhance the resilience and stability of their applications.
Healthcare: AI Innovators Enhancing Patient OutcomesHere’s an example of using Sentry for error monitoring in a Python application:
import sentry_sdk
# Initialize Sentry SDK
sentry_sdk.init("https://examplePublicKey@o0.ingest.sentry.io/0")
def divide(a, b):
try:
return a / b
except ZeroDivisionError as e:
# Capture the exception with Sentry
sentry_sdk.capture_exception(e)
return "Error: Division by zero"
print(divide(10, 2))
print(divide(10, 0))
Empowering Intelligent Application Development
Creating Personalized User Experiences
Creating personalized user experiences is a powerful application of machine learning in development. By analyzing user behavior and preferences, ML models can deliver customized content, recommendations, and interactions that enhance user engagement and satisfaction.
Recommendation systems, such as those used by Netflix and Amazon, leverage machine learning to suggest relevant content and products to users. These systems analyze past user behavior, preferences, and similar users' actions to provide tailored recommendations that improve the user experience.
Natural language processing (NLP) models can also personalize user interactions by enabling chatbots and virtual assistants to understand and respond to user queries contextually. Tools like Dialogflow and IBM Watson offer developers the ability to create intelligent conversational agents that enhance customer support and engagement.
Affordable Machine Learning: Boosting Business EffectivenessHere’s an example of creating a simple recommendation system using collaborative filtering with scikit-learn:
import numpy as np
from sklearn.metrics.pairwise import cosine_similarity
# Sample user-item interaction matrix
user_item_matrix = np.array([
[5, 3, 0, 1],
[4, 0, 0, 1],
[1, 1, 0, 5],
[1, 0, 0, 4],
[0, 1, 5, 4],
])
# Compute cosine similarity between users
user_similarity = cosine_similarity(user_item_matrix)
# Predict ratings for a specific user
user_index = 0
predicted_ratings = user_similarity[user_index].dot(user_item_matrix) / np.sum(user_similarity[user_index])
print(f'Predicted ratings for user {user_index}: {predicted_ratings}')
Enhancing Search and Recommendation Systems
Enhancing search and recommendation systems is another critical application of machine learning for developers. ML models improve search relevance and recommendation accuracy by analyzing large datasets and understanding user intent. This leads to more effective search results and recommendations, enhancing user satisfaction.
Search engines like Google use machine learning algorithms to rank search results based on relevance and user behavior. These algorithms consider various factors, including query context, user history, and content quality, to deliver the most pertinent results. Developers can implement similar techniques in their applications to improve search functionality.
Recommendation systems can be further enhanced by incorporating collaborative filtering, content-based filtering, and hybrid approaches. Collaborative filtering leverages user behavior patterns, while content-based filtering analyzes item attributes. Hybrid approaches combine both methods to provide more accurate and diverse recommendations.
Improving Anomaly Detection in Manufacturing with ML and Control ChartsHere’s an example of implementing a content-based recommendation system using scikit-learn:
import pandas as pd
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import linear_kernel
# Sample data
data = pd.DataFrame({
'title': ['Python Tutorial', 'Java Programming', 'Learn Data Science', 'Machine Learning Basics'],
'description': [
'This tutorial covers Python programming.',
'Java programming for beginners.',
'Learn data science concepts and techniques.',
'Basics of machine learning for beginners.'
]
})
# Vectorize the descriptions
tfidf = TfidfVectorizer(stop_words='english')
tfidf_matrix = tfidf.fit_transform(data['description'])
# Compute cosine similarity between descriptions
cosine_sim = linear_kernel(tfidf_matrix, tfidf_matrix)
# Function to get recommendations
def get_recommendations(title, cosine_sim=cosine_sim):
idx = data.index[data['title'] == title].tolist()[0]
sim_scores = list(enumerate(cosine_sim[idx]))
sim_scores = sorted(sim_scores, key=lambda x: x[1], reverse=True)
sim_scores = sim_scores[1:3] # Get the top 2 similar items
item_indices = [i[0] for i in sim_scores]
return data['title'].iloc[item_indices]
# Get recommendations for a specific title
recommendations = get_recommendations('Python Tutorial')
print('Recommendations:')
print(recommendations)
Enhancing Predictive Analytics
Enhancing predictive analytics involves using machine learning models to analyze historical data and predict future outcomes. This capability is invaluable for applications in finance, healthcare, marketing, and more. Predictive analytics helps developers create smarter applications that can anticipate user needs and make informed decisions.
Machine learning models such as linear regression, decision trees, and neural networks can predict various metrics, from stock prices to patient outcomes. Tools like TensorFlow and [PyTorch](https
://pytorch.org) provide powerful libraries for building and deploying predictive models.
Enhancing Gaming Experiences with Neural Networks and Machine LearningIn marketing, predictive analytics can identify potential customers, forecast sales, and optimize marketing campaigns. In healthcare, it can predict disease progression and patient readmissions. By integrating predictive analytics into their applications, developers can provide valuable insights and enhance decision-making processes.
Here’s an example of using linear regression for predictive analytics with scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Sample data
data = pd.DataFrame({
'feature1': [1, 2, 3, 4, 5],
'feature2': [5, 6, 7, 8, 9],
'target': [10, 12, 15, 20, 25]
})
# Define features and target variable
X = data[['feature1', 'feature2']]
y = data['target']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a linear regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Predict on the test data
y_pred = model.predict(X_test)
# Evaluate the model
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
Optimizing Development Processes with Machine Learning
Streamlining Project Management
Streamlining project management with machine learning involves using predictive models to enhance planning, resource allocation, and risk management. ML algorithms can analyze historical project data to forecast timelines, identify potential bottlenecks, and suggest optimal resource allocation.
Tools like Jira and Asana integrate machine learning to provide insights into project progress and team performance. These platforms use ML to predict project completion dates, recommend task assignments, and identify at-risk projects. This helps project managers make informed decisions and keep projects on track.
Machine learning can also assist in prioritizing tasks based on their impact and urgency. By analyzing project dependencies and resource availability, ML models can suggest the most efficient task sequences. This ensures that critical tasks are addressed promptly, improving overall project efficiency.
Here’s an example of using machine learning to predict project completion times with scikit-learn:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_absolute_error
# Sample project data
data = pd.DataFrame({
'task_duration': [5, 10, 7, 8, 12],
'task_complexity': [1, 2, 1, 3, 2],
'resource_availability': [10, 8, 12, 7, 9],
'completion_time': [15, 30, 20, 25, 35]
})
# Define features and target variable
X = data[['task_duration', 'task_complexity', 'resource_availability']]
y = data['completion_time']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a linear regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Predict on the test data
y_pred = model.predict(X_test)
# Evaluate the model
mae = mean_absolute_error(y_test, y_pred)
print(f'Mean Absolute Error: {mae}')
Enhancing DevOps with Machine Learning
Enhancing DevOps with machine learning involves using predictive analytics to optimize deployment processes, monitor system performance, and automate routine tasks. ML models can predict system failures, optimize resource utilization, and ensure continuous integration and delivery (CI/CD) pipelines run smoothly.
Tools like Ansible and Puppet use machine learning to automate configuration management and deployment. These platforms can predict potential deployment issues and suggest optimizations, ensuring seamless and efficient rollouts. This reduces downtime and enhances system reliability.
Machine learning can also monitor system performance and detect anomalies in real-time. By analyzing logs and performance metrics, ML models can identify unusual patterns and trigger alerts before issues escalate. This proactive approach helps DevOps teams maintain system stability and respond quickly to potential problems.
Here’s an example of using machine learning to predict system failures with scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Sample system performance data
data = pd.DataFrame({
'cpu_usage': [70, 80, 90, 60, 50],
'memory_usage': [60, 70, 80, 50, 40],
'disk_io': [100, 200, 150, 80, 70],
'failure': [1, 1, 1, 0, 0]
})
# Define features and target variable
X = data[['cpu_usage', 'memory_usage', 'disk_io']]
y = data['failure']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a random forest classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Predict on the test data
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Optimizing Resource Management
Optimizing resource management with machine learning involves analyzing usage patterns and predicting future needs to allocate resources efficiently. ML models can forecast demand, optimize inventory, and ensure that resources are available when needed, reducing waste and improving operational efficiency.
In cloud computing, machine learning can predict resource utilization and automatically scale infrastructure to meet demand. Tools like AWS Auto Scaling and Google Cloud AutoML use ML to optimize resource allocation, ensuring cost-effectiveness and performance.
In manufacturing and logistics, ML models can predict inventory needs and optimize supply chains. By analyzing historical data and market trends, these models can forecast demand and adjust inventory levels accordingly. This helps businesses maintain optimal stock levels, reduce costs, and meet customer demand efficiently.
Here’s an example of using machine learning to predict inventory needs with scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_absolute_error
# Sample inventory data
data = pd.DataFrame({
'past_sales': [100, 150, 200, 250, 300],
'seasonality_index': [1.0, 1.2, 1.1, 0.9, 1.3],
'marketing_spend': [1000, 1200, 1100, 900, 1300],
'inventory_needed': [120, 180, 220, 230, 350]
})
# Define features and target variable
X = data[['past_sales', 'seasonality_index', 'marketing_spend']]
y = data['inventory_needed']
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a linear regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Predict on the test data
y_pred = model.predict(X_test)
# Evaluate the model
mae = mean_absolute_error(y_test, y_pred)
print(f'Mean Absolute Error: {mae}')
Future Trends in Machine Learning for Developers
Increasing Use of Automated Machine Learning (AutoML)
Increasing use of automated machine learning (AutoML) is a growing trend that simplifies the process of building and deploying ML models. AutoML platforms automate tasks such as data preprocessing, feature selection, model selection, and hyperparameter tuning, making it easier for developers to implement machine learning without deep expertise.
Platforms like Google AutoML and H2O.ai provide tools that allow developers to build high-quality models quickly. These platforms use advanced algorithms to explore different model architectures and configurations, selecting the best performing models for the given data.
AutoML democratizes machine learning by lowering the barrier to entry and enabling more developers to leverage ML in their projects. It accelerates the development process and ensures that models are optimized for performance, making machine learning more accessible and efficient.
Here’s an example of using H2O AutoML in Python:
import h2o
from h2o.automl import H2OAutoML
# Initialize H2O cluster
h2o.init()
# Load sample data
data = h2o.import_file('https://raw.githubusercontent.com/h2oai/h2o-3/master/smalldata/iris/iris_wheader.csv')
# Define features and target
X = data.columns[:-1]
y = data.columns[-1]
# Train an AutoML model
aml = H2OAutoML(max_runtime_secs=60)
aml.train(x=X, y=y, training_frame=data)
# View the AutoML leaderboard
lb = aml.leaderboard
print(lb)
Advancements in Explainable AI
Advancements in explainable AI (XAI) are critical as machine learning models become more complex and widely used. XAI aims to make ML models transparent and understandable, ensuring that their decisions can be explained and trusted. This is particularly important in fields like healthcare, finance, and legal systems, where decisions have significant consequences.
Tools like SHAP and LIME provide methods to interpret model predictions and understand feature importance. These tools help developers and stakeholders gain insights into how models make decisions, fostering trust and accountability.
As XAI techniques continue to evolve, they will enable more transparent and interpretable machine learning applications. This will be essential for regulatory compliance, ethical AI development, and building trust with users.
Here’s an example of using SHAP to explain a model’s predictions in Python:
import shap
import xgboost
import pandas as pd
# Load sample data
data = pd.read_csv('https://raw.githubusercontent.com/ageron/handson-ml/master/datasets/housing/housing.csv')
data = data.dropna()
# Define features and target
X = data.drop('median_house_value', axis=1)
y = data['median_house_value']
# Train an XGBoost model
model = xgboost.XGBRegressor()
model.fit(X, y)
# Create a SHAP explainer
explainer = shap.Explainer(model)
shap_values = explainer(X)
# Plot SHAP values
shap.summary_plot(shap_values, X)
Integration of Machine Learning with Edge Computing
Integration of machine learning with edge computing is an emerging trend that brings ML capabilities closer to the source of data generation. Edge computing allows data to be processed locally on devices like smartphones, IoT devices, and edge servers, reducing latency and bandwidth usage.
Machine learning models deployed on edge devices can provide real-time insights and decisions, enabling applications such as autonomous vehicles, smart homes, and industrial automation. Platforms like TensorFlow Lite and AWS Greengrass support the deployment of ML models on edge devices.
Integrating ML with edge computing enhances the performance and responsiveness of applications. It also improves data privacy and security by processing data locally rather than transmitting it to centralized servers. As edge computing technologies advance, they will enable more sophisticated and efficient machine learning applications.
Here’s an example of deploying a TensorFlow Lite model on an edge device:
import tensorflow as tf
# Load a pre-trained model
model = tf.keras.applications.MobileNetV2(weights='imagenet', input_shape=(224, 224, 3))
# Convert the model to TensorFlow Lite format
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
# Save the TensorFlow Lite model
with open('model.tflite', 'wb') as file:
file.write(tflite_model)
Machine learning offers tremendous benefits for developers, from automating repetitive tasks and improving code quality to creating personalized user experiences and enhancing predictive analytics. By leveraging advanced tools and techniques, developers can unlock the full potential of machine learning and create smarter, more efficient applications. With ongoing advancements in AutoML, explainable AI, and edge computing, the future of machine learning in development looks incredibly promising. Using resources like Google and Kaggle, developers can continue to explore innovative ways to integrate machine learning into their projects and drive technological progress.
If you want to read more articles similar to Leveraging Machine Learning: Unlocking the Benefits for Developers, you can visit the Applications category.
You Must Read