
Linear Regression in Machine Learning with R: Step-by-Step Guide
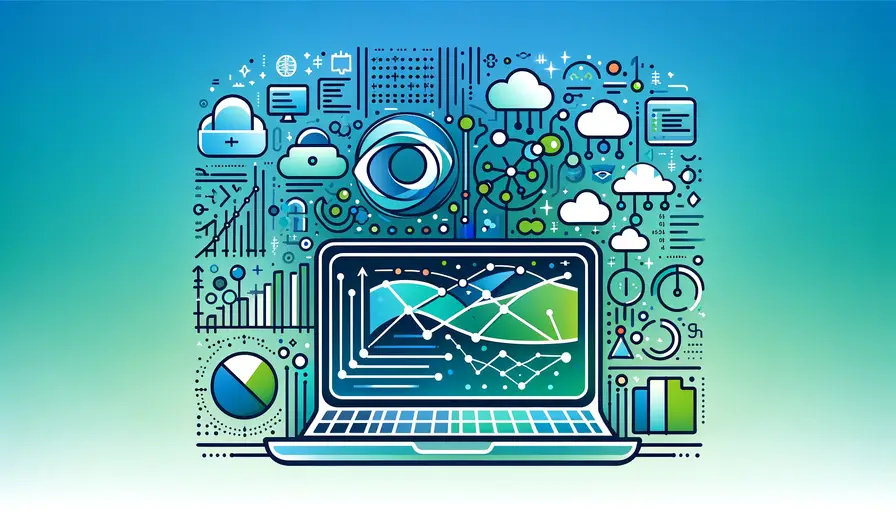
- Understand the Basics of Linear Regression
- Install R and Relevant Packages for Linear Regression
- Import the Dataset into R
- Explore and Preprocess the Dataset
- Split the Dataset into Training and Testing Sets
- Create a Linear Regression Model
- Train the Model Using the Training Set
- Evaluate the Model's Performance on the Testing Set
- Make Predictions Using the Trained Model
- Interpret the Coefficients and Statistical Measures of the Model
- Fine-Tune the Model by Adding or Removing Features
- Use Cross-Validation to Assess the Model's Generalization Ability
- Apply Linear Regression to Real-World Datasets for Prediction or Analysis
Understand the Basics of Linear Regression
How Does Linear Regression Work?
Linear regression is a fundamental statistical method used to model the relationship between a dependent variable and one or more independent variables. The primary goal of linear regression is to fit a linear equation to the observed data. The simplest form, simple linear regression, involves a single independent variable and fits the equation:
$$y = \beta_0 + \beta_1x + \epsilon$$
Here, \(y\) is the dependent variable, \(x\) is the independent variable, \(\beta_0\) is the intercept, \(\beta_1\) is the slope, and \(\epsilon\) is the error term. The slope \(\beta_1\) represents the change in the dependent variable for a one-unit change in the independent variable.
Linear regression assumes a linear relationship between the dependent and independent variables. This assumption simplifies the process of modeling and interpretation, making linear regression a powerful tool for prediction and analysis.
Machine Learning Algorithms for Simultaneously Handling Two DatasetsSteps to Perform Linear Regression with R
Performing linear regression with R involves several key steps, from data preparation to model evaluation. First, you need to import the dataset into R and conduct exploratory data analysis (EDA) to understand its structure and characteristics. This step includes visualizing the data and identifying any potential issues, such as missing values or outliers.
Next, preprocess the dataset by handling missing values, encoding categorical variables, and normalizing numerical features if necessary. After preprocessing, split the dataset into training and testing sets to ensure that the model is evaluated on unseen data.
Train the linear regression model using the training set and evaluate its performance on the testing set using various metrics. Finally, interpret the model coefficients and statistical measures to understand the relationships between the variables and fine-tune the model by adding or removing features.
Install R and Relevant Packages for Linear Regression
Install R
Installing R is the first step to getting started with linear regression in R. R is a free software environment for statistical computing and graphics. To install R, visit the Comprehensive R Archive Network (CRAN) website and download the version appropriate for your operating system (Windows, macOS, or Linux).
The Potential of Decision Trees in Non-Linear Machine LearningOnce downloaded, run the installer and follow the on-screen instructions to complete the installation. After installation, you can launch R from your operating system’s application menu.
Install RStudio (Optional)
Installing RStudio is optional but recommended for an enhanced user experience. RStudio is an integrated development environment (IDE) for R that provides a user-friendly interface and additional features such as syntax highlighting, code completion, and an interactive console.
To install RStudio, visit the RStudio website and download the installer for your operating system. Run the installer and follow the instructions to complete the installation. Once installed, you can launch RStudio, which will automatically detect your R installation.
Install the Necessary Packages
Installing necessary packages is crucial for performing linear regression in R. The primary package used for linear regression is stats
, which is included by default with R. However, additional packages such as tidyverse
for data manipulation and visualization, and caret
for model training and evaluation, can be beneficial.
To install these packages, use the following commands in R or RStudio:
install.packages("tidyverse")
install.packages("caret")
Load the installed packages into your R session using the library()
function:
library(tidyverse)
library(caret)
These packages provide a comprehensive set of tools for data manipulation, visualization, and machine learning in R.
Import the Dataset into R
Exploratory Data Analysis (EDA)
Exploratory Data Analysis (EDA) involves examining the dataset to understand its structure, summarize its main characteristics, and visualize its distribution. EDA helps identify patterns, detect anomalies, and determine the relationships between variables.
Optimal Frequency for Regression Testing: How Often is Ideal?Start by loading the dataset into R using the read.csv()
function:
data <- read.csv("path/to/your/dataset.csv")
Next, use functions like summary()
, str()
, and head()
to get an overview of the data:
summary(data)
str(data)
head(data)
Visualize the data using ggplot2
from the tidyverse
package:
ggplot(data, aes(x = independent_variable, y = dependent_variable)) +
geom_point() +
geom_smooth(method = "lm", se = FALSE) +
theme_minimal()
This plot provides insights into the linear relationship between the variables and helps in identifying any outliers or unusual patterns.
Securing and Ensuring Reliability of Your Machine Learning PipelineExplore and Preprocess the Dataset
Exploring and preprocessing the dataset involves cleaning the data and preparing it for modeling. This step includes handling missing values, encoding categorical variables, and normalizing numerical features. Ensuring data quality is crucial for building an accurate and reliable model.
Begin by identifying and handling missing values. Use functions like is.na()
to detect missing values and impute them using appropriate methods such as mean, median, or mode imputation:
data <- data %>%
mutate(across(everything(), ~ifelse(is.na(.), mean(., na.rm = TRUE), .)))
Encode categorical variables using functions like factor()
or model.matrix()
to convert them into numerical format:
data$category <- as.factor(data$category)
Normalize numerical features if necessary to ensure they are on a similar scale:
Top SVM Models for Machine Learning: An Explorationdata <- data %>%
mutate(across(where(is.numeric), scale))
These preprocessing steps help improve the model’s performance and ensure that the data is suitable for linear regression.
Split the Dataset into Training and Testing Sets
Splitting the dataset into training and testing sets is essential for evaluating the model’s performance on unseen data. This step ensures that the model generalizes well and avoids overfitting. Typically, the dataset is split into 70-80% for training and 20-30% for testing.
Use the createDataPartition()
function from the caret
package to split the data:
set.seed(123)
trainIndex <- createDataPartition(data$dependent_variable, p = .8,
list = FALSE,
times = 1)
dataTrain <- data[trainIndex,]
dataTest <- data[-trainIndex,]
This code splits the dataset into training and testing sets based on the dependent variable’s distribution, ensuring a representative sample in both sets.
Create a Linear Regression Model
Import the Necessary Libraries
Importing necessary libraries is the first step in creating a linear regression model in R. Load the required libraries for data manipulation, visualization, and modeling using the library()
function:
library(tidyverse)
library(caret)
These libraries provide the tools needed to preprocess the data, train the model, and evaluate its performance.
Load the Dataset
Loading the dataset into R is essential for building the linear regression model. Use the read.csv()
function to import the dataset from a CSV file:
data <- read.csv("path/to/your/dataset.csv")
Examine the dataset using functions like summary()
, str()
, and head()
to understand its structure and contents.
Explore the Dataset
Exploring the dataset involves visualizing the data and summarizing its main characteristics. Use functions from the ggplot2
package to create scatter plots and histograms that help identify patterns and relationships:
ggplot(data, aes(x = independent_variable, y = dependent_variable)) +
geom_point() +
geom_smooth(method = "lm", se = FALSE) +
theme_minimal()
This plot provides insights into the linear relationship between the variables.
Prepare the Dataset
Preparing the dataset includes handling missing values, encoding categorical variables, and normalizing numerical features. These preprocessing steps ensure the data is clean and suitable for modeling:
data <- data %>%
mutate(across(everything(), ~ifelse(is.na(.), mean(., na.rm = TRUE), .))) %>%
mutate(across(where(is.factor), as.numeric)) %>%
mutate(across(where(is.numeric), scale))
This code handles missing values, encodes categorical variables, and normalizes numerical features.
Split the Dataset
Splitting the dataset into training and testing sets is crucial for evaluating the model’s performance on unseen data. Use the createDataPartition()
function from the caret
package:
set.seed(123)
trainIndex <- createDataPartition(data$dependent_variable, p = .8,
list = FALSE,
times = 1)
dataTrain <- data[trainIndex,]
dataTest <- data[-trainIndex,]
This code splits the dataset into training and testing sets, ensuring a representative sample in both sets.
Train the Linear Regression Model
Training the linear regression model involves fitting the model to the training data using the lm()
function:
model <- lm(dependent_variable ~ independent_variable, data = dataTrain)
summary(model)
This code fits a linear regression model to the training data and provides a summary of the model, including the coefficients and statistical measures.
Evaluate the Model
Evaluating the model involves assessing its performance on the testing set using various metrics such as Mean Absolute Error (MAE), Mean Squared Error (MSE), Root Mean Squared Error (RMSE), and R-Squared (R²) Score:
predictions <- predict(model, newdata = dataTest)
mae <- mean(abs(predictions - dataTest$dependent_variable))
mse <- mean((predictions - dataTest$
dependent_variable)^2)
rmse <- sqrt(mse)
r_squared <- summary(model)$r.squared
cat("MAE:", mae, "\n")
cat("MSE:", mse, "\n")
cat("RMSE:", rmse, "\n")
cat("R-Squared:", r_squared, "\n")
This code calculates and prints the evaluation metrics for the model.
Train the Model Using the Training Set
Training the model using the training set involves fitting the linear regression model to the prepared data. This step ensures that the model learns the relationships between the independent and dependent variables, enabling it to make accurate predictions on new data.
Use the lm()
function to train the model:
model <- lm(dependent_variable ~ independent_variable, data = dataTrain)
Review the model summary to understand the coefficients and statistical measures, which provide insights into the model's performance.
Evaluate the Model's Performance on the Testing Set
Mean Absolute Error (MAE)
Mean Absolute Error (MAE) is a metric used to evaluate the model's performance by calculating the average absolute difference between the predicted and actual values. A lower MAE indicates better model accuracy:
mae <- mean(abs(predictions - dataTest$dependent_variable))
cat("MAE:", mae, "\n")
This code calculates the MAE for the model's predictions on the testing set.
Mean Squared Error (MSE)
Mean Squared Error (MSE) measures the average squared difference between the predicted and actual values. A lower MSE indicates better model performance:
mse <- mean((predictions - dataTest$dependent_variable)^2)
cat("MSE:", mse, "\n")
This code calculates the MSE for the model's predictions on the testing set.
Root Mean Squared Error (RMSE)
Root Mean Squared Error (RMSE) is the square root of the MSE and provides an estimate of the model's prediction error in the same units as the dependent variable:
rmse <- sqrt(mse)
cat("RMSE:", rmse, "\n")
This code calculates the RMSE for the model's predictions on the testing set.
R-Squared (R²) Score
R-Squared (R²) Score measures the proportion of variance in the dependent variable that is predictable from the independent variables. A higher R² score indicates better model fit:
r_squared <- summary(model)$r.squared
cat("R-Squared:", r_squared, "\n")
This code calculates the R² score for the model, providing insights into its explanatory power.
Make Predictions Using the Trained Model
Making predictions using the trained model involves applying the model to new or unseen data. This step demonstrates the model's ability to generalize and provide accurate predictions based on the relationships it has learned.
Use the predict()
function to make predictions on the testing set or new data:
new_predictions <- predict(model, newdata = new_data)
Evaluate the predictions to ensure they align with expected outcomes and assess the model's performance.
Interpret the Coefficients and Statistical Measures of the Model
Coefficients
Interpreting the coefficients of the linear regression model provides insights into the relationships between the independent and dependent variables. The coefficients represent the change in the dependent variable for a one-unit change in the independent variable.
For example, in a simple linear regression model:
summary(model)$coefficients
Review the coefficients to understand the magnitude and direction of the relationships.
Statistical Measures
Statistical measures such as p-values and confidence intervals provide additional insights into the significance and reliability of the model coefficients. These measures help determine whether the observed relationships are statistically significant.
For example, reviewing the p-values and confidence intervals:
summary(model)$coefficients
confint(model)
These measures help assess the strength and reliability of the model's predictions.
Fine-Tune the Model by Adding or Removing Features
Fine-tuning the model involves adding or removing features to improve its performance. This process includes experimenting with different combinations of independent variables to identify the best model for prediction and analysis.
Use stepwise regression or manual selection to add or remove features:
model <- lm(dependent_variable ~ independent_variable1 + independent_variable2, data = dataTrain)
Evaluate the model's performance with different feature sets to identify the optimal configuration.
Use Cross-Validation to Assess the Model's Generalization Ability
Cross-validation is a technique used to assess the model's ability to generalize to new data. It involves dividing the dataset into multiple folds and training the model on each fold while using the remaining data for validation.
Use the trainControl()
and train()
functions from the caret
package to perform cross-validation:
train_control <- trainControl(method = "cv", number = 10)
cv_model <- train(dependent_variable ~ independent_variable, data = dataTrain, method = "lm", trControl = train_control)
print(cv_model)
Evaluate the cross-validation results to ensure the model generalizes well and avoids overfitting.
Apply Linear Regression to Real-World Datasets for Prediction or Analysis
Why Use Linear Regression?
Linear regression is widely used for prediction and analysis due to its simplicity, interpretability, and effectiveness. It helps identify relationships between variables and predict future outcomes based on historical data. Its applications range from finance and economics to healthcare and social sciences.
Step-by-Step Guide to Linear Regression in R
Applying linear regression to real-world datasets involves following a systematic approach. Start with data collection and preprocessing, followed by model training and evaluation. Fine-tune the model based on performance metrics and use cross-validation to ensure generalization.
For a detailed step-by-step guide, follow the sections outlined in this article, from understanding the basics of linear regression to applying the model to real-world datasets.
By following this guide, you can effectively perform linear regression in R, leveraging its powerful tools and packages to build accurate and reliable models for prediction and analysis.
If you want to read more articles similar to Linear Regression in Machine Learning with R: Step-by-Step Guide, you can visit the Algorithms category.
You Must Read