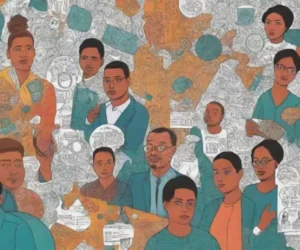
Machine Learning Algorithms and Neural Networks

Machine learning and neural networks have revolutionized how we analyze data, make predictions, and create intelligent systems. Each has its strengths and specific use cases, but their combined power can produce even more robust solutions. This article explores how machine learning algorithms and neural networks work together, their types, applications, and the structure of neural networks.
Machine Learning Algorithms and Neural Networks Working Together
Machine learning algorithms and neural networks often work together to create sophisticated models that leverage the strengths of each approach. Machine learning algorithms excel at handling structured data and can be easily interpreted, making them ideal for tasks like classification, regression, and clustering. On the other hand, neural networks are particularly powerful for unstructured data, such as images, audio, and text, where they can automatically learn and extract features.
Combining these technologies allows for hybrid models that take advantage of the interpretability and simplicity of traditional machine learning while incorporating the deep learning capabilities of neural networks. For example, a neural network might be used to extract features from raw image data, which are then fed into a machine learning algorithm for classification. This combination can result in models that are both accurate and interpretable.
Ensemble methods are another way machine learning algorithms and neural networks can work together. By using multiple models and combining their predictions, ensemble methods can improve accuracy and robustness. Techniques such as stacking involve training different models and using a meta-model to aggregate their predictions, leveraging the strengths of each individual model.
Decoding the AI vs ML Chronological Puzzlefrom sklearn.ensemble import StackingClassifier
from sklearn.linear_model import LogisticRegression
from sklearn.svm import SVC
from sklearn.ensemble import RandomForestClassifier
# Define base learners
base_learners = [
('rf', RandomForestClassifier(n_estimators=100)),
('svm', SVC(probability=True))
]
# Define stacking classifier
stacking_clf = StackingClassifier(estimators=base_learners, final_estimator=LogisticRegression())
# Train the stacking classifier
stacking_clf.fit(X_train, y_train)
# Evaluate the model
accuracy = stacking_clf.score(X_test, y_test)
print(f"Stacking model accuracy: {accuracy}")
Machine Learning Algorithms Are Used to Make Predictions Based on Data
Machine learning algorithms are designed to make predictions based on data by learning patterns and relationships within the dataset. These algorithms can be broadly classified into supervised, unsupervised, and reinforcement learning categories. Supervised learning involves training a model on labeled data, where the outcome is known, to make predictions on new, unseen data. Examples include linear regression, decision trees, and support vector machines.
Unsupervised learning algorithms, such as clustering and dimensionality reduction techniques, are used when the data does not have labeled outcomes. These algorithms identify hidden patterns and structures within the data. Clustering algorithms like K-means and hierarchical clustering group similar data points together, while dimensionality reduction techniques like PCA (Principal Component Analysis) reduce the number of features in a dataset, preserving essential information.
Reinforcement learning involves training an agent to make decisions by interacting with an environment and receiving rewards or penalties. The goal is to learn a policy that maximizes cumulative rewards over time. This approach is widely used in robotics, game playing, and autonomous systems.
Integrating machine learning algorithms with neural networks can enhance predictive capabilities. For instance, feature extraction using neural networks can be followed by traditional machine learning techniques for classification or regression, combining the strengths of both approaches to achieve superior performance.
Is Machine Learning Non-parametric: Exploring Model FlexibilityTypes of Neural Networks
There are several types of neural networks, each designed to address specific problems. Convolutional Neural Networks (CNNs) are primarily used for image and video processing. They leverage convolutional layers to detect spatial hierarchies in data, making them highly effective for tasks like image classification and object detection.
Recurrent Neural Networks (RNNs) are suitable for sequential data, such as time series, speech, and text. They maintain a memory of previous inputs, allowing them to capture temporal dependencies. Variants like Long Short-Term Memory (LSTM) and Gated Recurrent Unit (GRU) address issues like the vanishing gradient problem, improving performance on longer sequences.
Generative Adversarial Networks (GANs) consist of two neural networks, a generator and a discriminator, that compete against each other. The generator creates synthetic data, while the discriminator evaluates its authenticity. This setup enables GANs to generate realistic images, videos, and other data types, making them useful for data augmentation and unsupervised learning.
Autoencoders are another type of neural network used for unsupervised learning. They compress input data into a latent space representation and then reconstruct the output from this representation. Autoencoders are useful for tasks such as noise reduction, anomaly detection, and data compression.
Comparing Machine Learning Techniques: Understanding Differencesfrom tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# Build a CNN model
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(64, 64, 3)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(64, activation='relu'),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Model summary
model.summary()
Applications of Neural Networks
Neural networks have a wide range of applications across various industries. In computer vision, CNNs are used for image classification, object detection, and facial recognition. These applications are crucial for fields such as healthcare (medical imaging), automotive (autonomous driving), and security (surveillance systems).
Natural language processing (NLP) benefits significantly from neural networks, particularly RNNs and transformers. Applications include machine translation, sentiment analysis, and speech recognition. These technologies enable virtual assistants, chatbots, and automated customer service systems to understand and respond to human language effectively.
In finance, neural networks are used for algorithmic trading, fraud detection, and credit scoring. Their ability to process large volumes of data and identify patterns helps financial institutions make data-driven decisions, mitigate risks, and improve customer service.
Healthcare applications of neural networks include diagnosing diseases from medical images, predicting patient outcomes, and personalizing treatment plans. By analyzing complex datasets such as genomic data and electronic health records, neural networks can provide valuable insights that enhance patient care and medical research.
Analysis of Popular Off-the-Shelf Machine Learning ModelsNeural Networks Consist of Interconnected Nodes Called Neurons
Neural networks are composed of interconnected nodes, or neurons, that mimic the functioning of the human brain. These neurons are organized into layers, including an input layer, one or more hidden layers, and an output layer. Each neuron receives inputs, processes them through a weighted sum, applies an activation function, and passes the output to the next layer.
The architecture of a neural network determines its ability to learn and generalize from data. In feedforward neural networks, the connections between neurons do not form cycles, making them straightforward to implement and train. In contrast, recurrent neural networks (RNNs) have connections that form cycles, allowing them to maintain memory of previous inputs and capture temporal dependencies.
Training a neural network involves adjusting the weights of the connections between neurons to minimize the error between predicted and actual outputs. This process, known as backpropagation, uses gradient descent to update the weights iteratively. The learning rate, a hyperparameter that controls the size of weight updates, plays a crucial role in the convergence of the training process.
Activation functions are mathematical functions applied to the output of each neuron. They introduce non-linearity into the model, enabling it to learn complex patterns. Common activation functions include ReLU (Rectified Linear Unit), sigmoid, and tanh. The choice of activation function can significantly impact the performance of the neural network.
Building Machine Learning AIimport tensorflow as tf
from tensorflow.keras import layers, models
# Build a simple neural network model
model = models.Sequential()
model.add(layers.Dense(64, activation='relu', input_shape=(10,)))
model.add(layers.Dense(64, activation='relu'))
model.add(layers.Dense(1, activation='linear'))
# Compile the model
model.compile(optimizer='adam', loss='mse')
# Print the model summary
model.summary()
Machine learning algorithms and neural networks offer complementary strengths that can be harnessed to build powerful models. By understanding their unique capabilities and applications, practitioners can leverage the best of both worlds to solve complex problems and drive innovation across various fields.
If you want to read more articles similar to Machine Learning Algorithms and Neural Networks, you can visit the Artificial Intelligence category.
You Must Read