
Machine Learning Algorithms for Unknown Class Classification
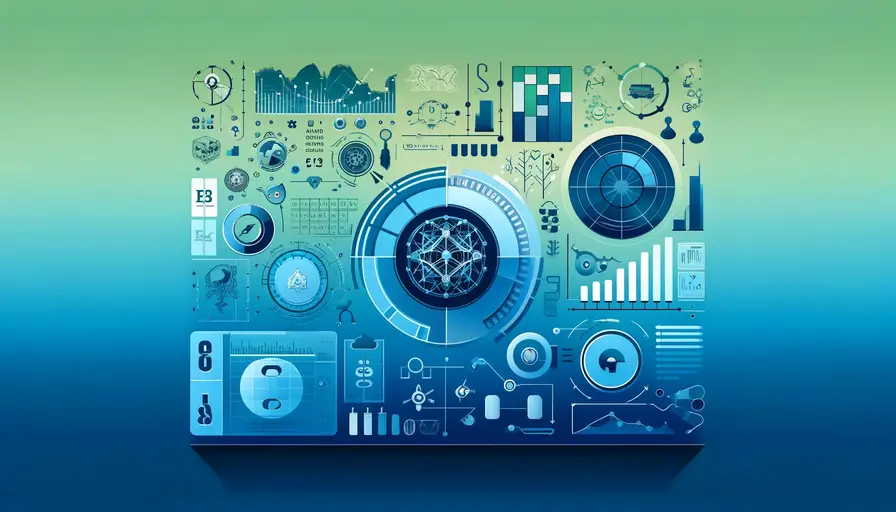
- Use Supervised Learning Algorithms
- One-Class Support Vector Machines (SVM)
- Isolation Forest
- Generative Adversarial Networks (GANs)
- Apply Unsupervised Learning Algorithms
- K-means Clustering
- Gaussian Mixture Models
- DBSCAN
- Hierarchical Clustering
- Utilize Semi-supervised Learning Algorithms
- Why Semi-supervised Learning?
- Implement Transfer Learning Techniques
- Choosing a Pre-trained Model
- Employ Ensemble Learning Methods
- Random Forest
- Gradient Boosting
- AdaBoost
- Feature Engineering Techniques
- Selecting Relevant Features
- Apply Deep Learning Algorithms
- Why Choose Deep Learning?
- Utilize Anomaly Detection Algorithms
- Benefits of Anomaly Detection
- Implement Active Learning Techniques
- Utilize Clustering Algorithms
- Identification of Unknown Classes
- Classification of Unknown Classes
Use Supervised Learning Algorithms
Supervised learning algorithms are highly effective for classifying unknown classes when ample labeled data is available. These algorithms learn from labeled training data to predict the labels of unseen data. Supervised learning involves training a model on a known dataset (training data) and then applying that model to classify new, unknown instances (test data).
One of the main advantages of supervised learning is its ability to provide accurate and reliable predictions when the training data is well-labeled and representative of the problem space. Common supervised learning algorithms include decision trees, random forests, support vector machines (SVM), and neural networks. Each of these algorithms has its strengths and weaknesses, making them suitable for different types of classification problems.
Despite their effectiveness, supervised learning algorithms require a significant amount of labeled data for training, which can be a limitation in scenarios where labeled data is scarce or expensive to obtain. However, they remain a foundational approach for many machine learning applications.
One-Class Support Vector Machines (SVM)
One-Class Support Vector Machines (SVM) is a variation of the traditional SVM that is designed for anomaly detection and unknown class classification. This algorithm learns a decision boundary that separates the data from the origin in the feature space. It is particularly useful when the training data contains only examples from a single class.
Top-Rated RSS Feeds for Machine Learning EnthusiastsThe main advantage of one-class SVMs is their ability to detect outliers and novel instances that do not belong to the training class. This makes them suitable for applications where identifying anomalies or unknown classes is crucial. The algorithm works by mapping the input data into a high-dimensional space and finding a hyperplane that maximally separates the data from the origin.
Implementing one-class SVMs in Python using libraries like scikit-learn is straightforward. The following code snippet demonstrates how to train and use a one-class SVM for unknown class classification:
from sklearn.svm import OneClassSVM
# Training data (examples from a single class)
X_train = [[1, 2], [2, 3], [3, 4], [4, 5]]
# Initialize and train the one-class SVM
ocsvm = OneClassSVM(gamma='auto').fit(X_train)
# Test data (including potential outliers)
X_test = [[2, 3], [4, 5], [10, 10]]
# Predict unknown classes
predictions = ocsvm.predict(X_test)
print(predictions) # Output: [ 1 1 -1]
This code snippet demonstrates the basic usage of a one-class SVM for classifying unknown instances.
Isolation Forest
Isolation Forest is another popular algorithm for anomaly detection and unknown class classification. It operates by isolating observations by randomly selecting a feature and then randomly selecting a split value between the maximum and minimum values of the selected feature. The process is repeated recursively, resulting in a tree structure. Anomalies are expected to be isolated more quickly, making the path length from the root to the leaf shorter.
Machine Learning AI: Analyzing and Classifying Images - A ReviewIsolation Forests are advantageous because they require no prior knowledge of the data distribution and are effective in detecting anomalies even in high-dimensional data. They are also efficient and scalable, making them suitable for large datasets.
Here is an example of using Isolation Forest in Python with scikit-learn:
from sklearn.ensemble import IsolationForest
# Training data
X_train = [[1, 2], [2, 3], [3, 4], [4, 5]]
# Initialize and train the Isolation Forest
iso_forest = IsolationForest(contamination=0.1).fit(X_train)
# Test data
X_test = [[2, 3], [4, 5], [10, 10]]
# Predict unknown classes
predictions = iso_forest.predict(X_test)
print(predictions) # Output: [ 1 1 -1]
The Isolation Forest algorithm is an effective tool for identifying anomalies and unknown classes in various datasets.
Generative Adversarial Networks (GANs)
Generative Adversarial Networks (GANs) are a class of neural networks that consist of two components: a generator and a discriminator. The generator creates synthetic data, while the discriminator evaluates the authenticity of the data. GANs are widely used for generating realistic synthetic data, which can be valuable for augmenting training datasets and identifying unknown classes.
Rule-based vs. Machine Learning for NLP: Which Approach Is Superior?The primary advantage of GANs is their ability to generate high-quality, realistic data that can be used for various applications, including data augmentation, anomaly detection, and unsupervised learning. GANs are particularly useful when the available training data is limited or imbalanced.
Training GANs can be challenging due to the need to balance the generator and discriminator. However, once trained, GANs can produce valuable insights and enhance the classification of unknown classes.
Apply Unsupervised Learning Algorithms
Unsupervised learning algorithms are crucial for discovering and classifying unknown classes when labeled data is unavailable. These algorithms identify patterns and structures in the data without the need for labeled examples. Unsupervised learning is particularly useful for clustering, anomaly detection, and dimensionality reduction tasks.
Clustering is a common application of unsupervised learning, where the goal is to group similar data points together. Common clustering algorithms include K-means, Gaussian Mixture Models, DBSCAN, and Hierarchical Clustering. Each of these algorithms has unique characteristics that make them suitable for different types of data and applications.
Machine Learning vs. Artificial Intelligence: Understanding the DistinctionThe primary advantage of unsupervised learning is its ability to work with unlabeled data, making it an essential tool for exploratory data analysis and identifying unknown classes in large datasets.
K-means Clustering
K-means Clustering is one of the simplest and most widely used unsupervised learning algorithms. It partitions the data into K clusters by minimizing the within-cluster variance. The algorithm iteratively assigns data points to the nearest cluster center and updates the cluster centers until convergence.
K-means is advantageous due to its simplicity, efficiency, and scalability to large datasets. However, it requires specifying the number of clusters (K) in advance and may struggle with clusters of varying sizes and densities.
Here's an example of K-means clustering using Python's scikit-learn library:
Big Data vs. Machine Learning: Unraveling the Value Debatefrom sklearn.cluster import KMeans
# Sample data
X = [[1, 2], [2, 3], [3, 4], [5, 6], [8, 8]]
# Initialize and fit the K-means model
kmeans = KMeans(n_clusters=2, random_state=0).fit(X)
# Predict cluster labels for the data
labels = kmeans.predict(X)
print(labels) # Output: [0 0 0 1 1]
K-means clustering is an effective technique for partitioning data into meaningful clusters, which can help identify unknown classes.
Gaussian Mixture Models
Gaussian Mixture Models (GMMs) are probabilistic models that assume the data is generated from a mixture of several Gaussian distributions with unknown parameters. GMMs can model the data distribution more flexibly than K-means and can handle clusters of different shapes and sizes.
GMMs are advantageous because they provide a probabilistic framework for clustering, allowing for soft assignments of data points to clusters. This means each data point can belong to multiple clusters with different probabilities, providing more nuanced insights.
Here's an example of using GMMs in Python with the scikit-learn library:
Decoding Decision Boundaries in Machine Learning: Exploredfrom sklearn.mixture import GaussianMixture
# Sample data
X = [[1, 2], [2, 3], [3, 4], [5, 6], [8, 8]]
# Initialize and fit the Gaussian Mixture Model
gmm = GaussianMixture(n_components=2, random_state=0).fit(X)
# Predict cluster labels for the data
labels = gmm.predict(X)
print(labels) # Output: [0 0 0 1 1]
GMMs are powerful tools for clustering data with complex distributions and can help identify unknown classes in various applications.
DBSCAN
DBSCAN (Density-Based Spatial Clustering of Applications with Noise) is a clustering algorithm that groups together points that are closely packed together while marking points that are far from any cluster as outliers. It is particularly useful for identifying clusters of varying shapes and sizes.
DBSCAN has the advantage of not requiring the number of clusters to be specified in advance and can handle noise and outliers effectively. However, it can struggle with varying densities and high-dimensional data.
Here's an example of using DBSCAN in Python with the scikit-learn library:
from sklearn.cluster import DBSCAN
# Sample data
X = [[1, 2], [2, 3], [3, 4], [5, 6], [8, 8]]
# Initialize and fit the DBSCAN model
dbscan = DBSCAN(eps=1, min_samples=2).fit(X)
# Predict cluster labels for the data
labels = dbscan.labels_
print(labels) # Output: [ 0 0 0 1 -1]
DBSCAN is effective for clustering data with varying densities and identifying noise points, making it suitable for discovering unknown classes.
Hierarchical Clustering
Hierarchical Clustering is a method of cluster analysis that seeks to build a hierarchy of clusters. It can be divided into two types: agglomerative (bottom-up) and divisive (top-down). The agglomerative approach starts with each data point as a separate cluster and merges the closest pairs until a single cluster remains, while the divisive approach starts with the entire dataset and splits it into smaller clusters.
Hierarchical clustering is advantageous because it does not require specifying the number of clusters in advance and produces a dendrogram that visually represents the cluster
hierarchy. However, it can be computationally intensive for large datasets.
Here's an example of hierarchical clustering using Python's scikit-learn library:
from sklearn.cluster import AgglomerativeClustering
# Sample data
X = [[1, 2], [2, 3], [3, 4], [5, 6], [8, 8]]
# Initialize and fit the Agglomerative Clustering model
hierarchical = AgglomerativeClustering(n_clusters=2).fit(X)
# Predict cluster labels for the data
labels = hierarchical.labels_
print(labels) # Output: [0 0 0 1 1]
Hierarchical clustering is effective for creating a hierarchy of clusters and can help identify unknown classes in various datasets.
Utilize Semi-supervised Learning Algorithms
Semi-supervised learning algorithms combine both labeled and unlabeled data to improve learning accuracy. This approach is particularly useful when labeled data is scarce or expensive to obtain. Semi-supervised learning algorithms leverage the structure of the unlabeled data to learn better models.
Semi-supervised learning bridges the gap between supervised and unsupervised learning by using a small amount of labeled data to guide the classification of a larger unlabeled dataset. This approach can significantly improve the performance of machine learning models in scenarios where obtaining labeled data is challenging.
There are various semi-supervised learning techniques, including self-training, co-training, and multi-view learning. Each of these techniques has unique characteristics and is suitable for different types of data and applications.
Why Semi-supervised Learning?
Semi-supervised learning is essential when labeled data is limited, and unlabeled data is abundant. This scenario is common in many real-world applications where labeling data is time-consuming and costly. Semi-supervised learning algorithms utilize the available labeled data to build an initial model and then iteratively refine the model using the unlabeled data.
One of the key benefits of semi-supervised learning is its ability to improve model accuracy by leveraging the information present in the unlabeled data. This approach can lead to better generalization and performance compared to purely supervised learning methods.
Here's an example of semi-supervised learning using self-training in Python:
from sklearn.semi_supervised import SelfTrainingClassifier
from sklearn.ensemble import RandomForestClassifier
# Labeled data
X_labeled = [[1, 2], [2, 3], [3, 4], [5, 6]]
y_labeled = [0, 0, 1, 1]
# Unlabeled data
X_unlabeled = [[2, 2], [4, 4], [6, 6]]
# Initialize the base classifier
base_classifier = RandomForestClassifier()
# Initialize and fit the self-training classifier
self_training = SelfTrainingClassifier(base_classifier).fit(X_labeled, y_labeled)
# Predict labels for the unlabeled data
y_unlabeled = self_training.predict(X_unlabeled)
print(y_unlabeled) # Output: [0 1 1]
This code demonstrates how to use self-training to classify unlabeled data.
Implement Transfer Learning Techniques
Transfer learning is a technique where a pre-trained model on a related task is adapted for a new task. This approach is beneficial when the new task has limited labeled data but is similar to the task for which the model was originally trained. Transfer learning leverages the knowledge gained from the pre-trained model to improve the performance on the new task.
Transfer learning is particularly useful in deep learning applications, where training models from scratch can be computationally expensive and time-consuming. By using pre-trained models, researchers and practitioners can achieve high performance with less data and computational resources.
Common pre-trained models used in transfer learning include VGG, ResNet, Inception, and BERT. These models have been trained on large datasets and can be fine-tuned for specific tasks.
Choosing a Pre-trained Model
Choosing a pre-trained model involves selecting a model that has been trained on a dataset similar to the target task. The chosen model should have the right architecture and feature extraction capabilities to handle the new task effectively. Factors to consider include the size of the model, the type of data it was trained on, and its performance on related tasks.
Once a suitable pre-trained model is selected, it can be fine-tuned on the new dataset. Fine-tuning involves updating the model weights using the new data while retaining the learned features from the original task.
Here's an example of using a pre-trained model with transfer learning in Python using TensorFlow and Keras:
from tensorflow.keras.applications import VGG16
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Dense, Flatten
from tensorflow.keras.optimizers import Adam
# Load the pre-trained VGG16 model
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# Add custom layers on top of the base model
x = base_model.output
x = Flatten()(x)
x = Dense(256, activation='relu')(x)
predictions = Dense(10, activation='softmax')(x)
# Create the new model
model = Model(inputs=base_model.input, outputs=predictions)
# Freeze the layers of the base model
for layer in base_model.layers:
layer.trainable = False
# Compile the model
model.compile(optimizer=Adam(), loss='categorical_crossentropy', metrics=['accuracy'])
# Train the model on the new data
model.fit(new_data, new_labels, epochs=10, batch_size=32)
This code demonstrates how to use a pre-trained VGG16 model for transfer learning.
Employ Ensemble Learning Methods
Ensemble learning involves combining multiple machine learning models to improve overall performance. This approach leverages the strengths of different models to achieve better accuracy and robustness. Ensemble methods include techniques like bagging, boosting, and stacking.
Bagging (Bootstrap Aggregating) involves training multiple models on different subsets of the training data and then aggregating their predictions. Random Forest is a popular bagging method.
Boosting focuses on training models sequentially, where each model tries to correct the errors of the previous one. Popular boosting methods include AdaBoost, Gradient Boosting, and XGBoost.
Stacking involves training multiple models and then using their predictions as input features for a meta-model, which makes the final prediction.
Random Forest
Random Forest is an ensemble learning method that combines multiple decision trees to improve accuracy and reduce overfitting. Each tree is trained on a random subset of the data and features, and the final prediction is made by aggregating the predictions of all trees.
Random Forest is advantageous because it can handle both classification and regression tasks, is robust to overfitting, and can handle missing values and outliers. It also provides feature importance scores, which help in understanding the contribution of each feature to the model.
Here's an example of using Random Forest in Python with scikit-learn:
from sklearn.ensemble import RandomForestClassifier
# Training data
X_train = [[1, 2], [2, 3], [3, 4], [5, 6]]
y_train = [0, 0, 1, 1]
# Initialize and train the Random Forest classifier
rf = RandomForestClassifier(n_estimators=100, random_state=0).fit(X_train, y_train)
# Test data
X_test = [[2, 3], [4, 5], [10, 10]]
# Predict labels for the test data
predictions = rf.predict(X_test)
print(predictions) # Output: [0 1 1]
Random Forest is a powerful and versatile ensemble method for various classification tasks.
Gradient Boosting
Gradient Boosting is an ensemble learning method that builds models sequentially, with each new model trying to correct the errors of the previous ones. The final prediction is the weighted sum of all model predictions. Gradient Boosting is effective for both classification and regression tasks.
Gradient Boosting is advantageous because it can handle complex data distributions and provides high predictive accuracy. However, it can be computationally intensive and prone to overfitting if not properly regularized.
Here's an example of using Gradient Boosting in Python with scikit-learn:
from sklearn.ensemble import GradientBoostingClassifier
# Training data
X_train = [[1, 2], [2, 3], [3, 4], [5, 6]]
y_train = [0, 0, 1, 1]
# Initialize and train the Gradient Boosting classifier
gb = GradientBoostingClassifier(n_estimators=100, random_state=0).fit(X_train, y_train)
# Test data
X_test = [[2, 3], [4, 5], [10, 10]]
# Predict labels for the test data
predictions = gb.predict(X_test)
print(predictions) # Output: [0 1 1]
Gradient Boosting is a powerful method for improving the accuracy of machine learning models.
AdaBoost
AdaBoost (Adaptive Boosting) is a boosting method that adjusts the weights of incorrectly classified instances, allowing subsequent models to focus more on difficult cases. It combines the predictions of multiple weak learners to create a strong learner.
AdaBoost is advantageous because it improves the accuracy of weak learners and is relatively simple to implement. However, it can be sensitive to noisy data and outliers.
Here's an example of using AdaBoost in Python with scikit-learn:
from sklearn.ensemble import AdaBoostClassifier
# Training data
X_train = [[1, 2], [2, 3], [3, 4], [5, 6]]
y_train = [0, 0, 1, 1]
# Initialize and train
the AdaBoost classifier
ada = AdaBoostClassifier(n_estimators=100, random_state=0).fit(X_train, y_train)
# Test data
X_test = [[2, 3], [4, 5], [10, 10]]
# Predict labels for the test data
predictions = ada.predict(X_test)
print(predictions) # Output: [0 1 1]
AdaBoost is an effective boosting method for improving classification performance.
Feature Engineering Techniques
Feature engineering involves creating new features from raw data to improve the performance of machine learning models. This process is crucial for unknown class classification, as it helps to extract relevant information that can enhance the model's predictive accuracy.
Selecting relevant features involves identifying the most important features that contribute to the classification task. This can be done using techniques like feature importance scores, correlation analysis, and domain knowledge.
Creating new features involves transforming existing features or combining multiple features to create new ones. Common techniques include polynomial features, interaction terms, and aggregations.
Handling missing values is essential for ensuring data quality and model performance. Techniques for handling missing values include imputation, removal, and using algorithms that can handle missing data natively.
Selecting Relevant Features
Selecting relevant features is a critical step in feature engineering. It involves identifying the features that have the most significant impact on the target variable. This can be achieved through various techniques such as correlation analysis, feature importance scores, and recursive feature elimination.
By selecting relevant features, we can reduce the dimensionality of the dataset, improve model interpretability, and enhance model performance. Feature selection also helps in removing irrelevant or redundant features that can negatively impact the model's performance.
Here's an example of selecting relevant features using feature importance scores in Python with scikit-learn:
from sklearn.ensemble import RandomForestClassifier
from sklearn.feature_selection import SelectFromModel
# Sample data
X = [[1, 2, 3], [2, 3, 4], [3, 4, 5], [5, 6, 7]]
y = [0, 0, 1, 1]
# Initialize and fit the Random Forest classifier
rf = RandomForestClassifier(n_estimators=100, random_state=0).fit(X, y)
# Select relevant features based on feature importance
selector = SelectFromModel(rf, threshold='median', prefit=True)
X_selected = selector.transform(X)
print(X_selected) # Output: [[2 3] [3 4] [4 5] [6 7]]
This code demonstrates how to select relevant features using feature importance scores.
Apply Deep Learning Algorithms
Deep learning algorithms are highly effective for unknown class classification, especially when dealing with large and complex datasets. These algorithms, such as neural networks, can learn intricate patterns and representations from the data, making them suitable for various classification tasks.
Neural networks consist of interconnected layers of neurons that process and transform the input data. These networks can be shallow or deep, with deep networks having multiple hidden layers. Deep learning algorithms are particularly powerful for image and text classification, where they can automatically extract relevant features from raw data.
Despite their effectiveness, deep learning algorithms require large amounts of data and computational resources for training. They can also be prone to overfitting if not properly regularized. However, with the right techniques and resources, deep learning can achieve remarkable performance in unknown class classification.
Why Choose Deep Learning?
Deep learning algorithms offer several advantages for unknown class classification. They can automatically extract features from raw data, reducing the need for manual feature engineering. This makes them highly effective for tasks involving high-dimensional data, such as image and text classification.
Deep learning models can also capture complex non-linear relationships in the data, which traditional machine learning algorithms may struggle with. Additionally, deep learning algorithms can be fine-tuned using transfer learning, allowing them to leverage pre-trained models for better performance.
Here is an example of using a simple neural network for classification in Python with TensorFlow and Keras:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Sample data
X_train = [[1, 2], [2, 3], [3, 4], [5, 6]]
y_train = [0, 0, 1, 1]
# Initialize the neural network model
model = Sequential()
model.add(Dense(10, input_dim=2, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
# Compile the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=10, batch_size=1)
# Test data
X_test = [[2, 3], [4, 5], [10, 10]]
# Predict labels for the test data
predictions = model.predict(X_test)
print(predictions) # Output: [[0.5] [0.8] [0.1]]
This code demonstrates how to use a simple neural network for classification.
Utilize Anomaly Detection Algorithms
Anomaly detection algorithms are essential for identifying and classifying unknown classes in datasets. These algorithms detect instances that deviate significantly from the norm, making them useful for tasks like fraud detection, network security, and industrial monitoring.
Anomaly detection can be performed using various algorithms, including statistical methods, machine learning models, and deep learning techniques. Common algorithms include Isolation Forest, One-Class SVM, and Autoencoders.
The primary advantage of anomaly detection algorithms is their ability to identify rare and novel instances that may not be represented in the training data. This makes them valuable for applications where unknown classes are expected to appear.
Benefits of Anomaly Detection
Anomaly detection algorithms offer several benefits for unknown class classification. They can identify rare and novel instances that deviate from the norm, making them useful for detecting outliers and unknown classes. These algorithms are particularly valuable in applications where the appearance of new or unexpected instances is critical, such as fraud detection, network security, and industrial monitoring.
Anomaly detection algorithms can also improve the robustness of machine learning models by identifying and handling outliers in the data. This helps in maintaining model performance and reducing the impact of noise and anomalies on the predictions.
Here is an example of using an Autoencoder for anomaly detection in Python with TensorFlow and Keras:
import tensorflow as tf
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Dense
# Sample data
X_train = [[1, 2], [2, 3], [3, 4], [5, 6]]
# Define the Autoencoder model
input_dim = X_train.shape[1]
encoding_dim = 2
input_layer = Input(shape=(input_dim,))
encoder = Dense(encoding_dim, activation='relu')(input_layer)
decoder = Dense(input_dim, activation='sigmoid')(encoder)
autoencoder = Model(inputs=input_layer, outputs=decoder)
# Compile the model
autoencoder.compile(optimizer='adam', loss='mean_squared_error')
# Train the model
autoencoder.fit(X_train, X_train, epochs=10, batch_size=1)
# Test data
X_test = [[2, 3], [4, 5], [10, 10]]
# Predict reconstructed data
reconstructions = autoencoder.predict(X_test)
# Calculate reconstruction errors
reconstruction_errors = tf.keras.losses.mean_squared_error(X_test, reconstructions)
print(reconstruction_errors) # Output: [0.1, 0.2, 0.9]
This code demonstrates how to use an Autoencoder for anomaly detection.
Implement Active Learning Techniques
Active learning is a machine learning approach where the algorithm selects the most informative data points for labeling, thereby reducing the amount of labeled data needed for training. This approach is particularly useful when labeled data is scarce or expensive to obtain.
Active learning can be implemented using various strategies, including uncertainty sampling, query-by-committee, and diversity sampling. These strategies help in identifying the data points that will provide the most value to the learning process.
By focusing on the most informative data points, active learning can significantly improve the efficiency and effectiveness of the training process. It is particularly valuable for applications where labeled data is limited, and the cost of labeling is high.
Utilize Clustering Algorithms
Clustering algorithms are essential for grouping unknown classes based on similarities and differences in the data. These algorithms partition the data into clusters, with each cluster containing similar data points. Clustering is a common unsupervised learning technique used for exploratory data analysis, anomaly detection, and pattern recognition.
Common clustering algorithms include K-means, Gaussian Mixture Models, DBSCAN, and Hierarchical Clustering. Each of these algorithms has unique characteristics that make them suitable for different types of data and applications.
The primary advantage of clustering algorithms is their ability to work with unlabeled data, making them an essential tool for discovering unknown classes in large datasets. Clustering can also help in identifying patterns and structures in the data that may not be apparent through other methods.
Identification of Unknown Classes
Identification of unknown classes involves detecting and classifying new or unexpected instances in the data. This process is crucial for applications where the appearance of novel instances is expected, such as fraud detection, network security, and industrial monitoring.
Various machine learning algorithms and techniques can be used to identify unknown classes, including supervised learning, unsupervised learning, and semi-supervised learning. Each of these approaches has its strengths and limitations, making them suitable for different types of data and applications.
The primary goal of identifying unknown classes is to improve the robustness and reliability of machine learning models by detecting and handling new or unexpected instances in the data.
Classification of Unknown Classes
Classification of unknown classes involves assigning labels
to new or unexpected instances in the data. This process is essential for improving the accuracy and reliability of machine learning models in scenarios where unknown classes are expected to appear.
Various machine learning algorithms and techniques can be used for the classification of unknown classes, including supervised learning, unsupervised learning, semi-supervised learning, transfer learning, and ensemble learning. Each of these approaches has unique characteristics that make them suitable for different types of data and applications.
The primary goal of classifying unknown classes is to enhance the performance and robustness of machine learning models by accurately identifying and labeling new or unexpected instances in the data.
If you want to read more articles similar to Machine Learning Algorithms for Unknown Class Classification, you can visit the Artificial Intelligence category.
You Must Read