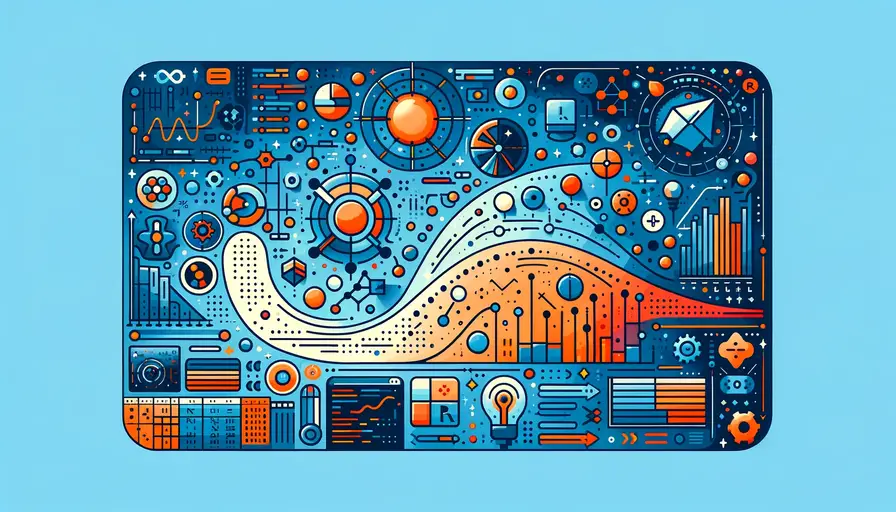
Machine Learning: Enabling Speech to Text Conversion

Machine learning has revolutionized many fields, and one of the most impactful applications is speech-to-text conversion. This technology translates spoken language into written text, enabling various practical applications such as voice-activated assistants, transcription services, and accessibility tools for the hearing impaired. In this article, we will explore the key components and methodologies involved in speech-to-text conversion, delve into the machine learning models that power it, and discuss real-world applications and advancements in this domain.
Fundamentals of Speech-to-Text Conversion
Capturing Audio Input
The process of converting speech to text begins with capturing the audio input. This involves using a microphone or another audio recording device to capture the spoken words. The quality of the audio input significantly affects the accuracy of the subsequent speech-to-text conversion.
Digital audio is represented as a sequence of samples that measure the amplitude of sound waves at discrete intervals. The sampling rate, typically measured in Hertz (Hz), determines how many samples are taken per second. A common sampling rate for speech processing is 16,000 Hz (16 kHz), which provides a good balance between audio quality and computational efficiency.
Preprocessing the audio input is crucial for improving the performance of speech-to-text systems. This step may include noise reduction, normalization, and segmentation of the audio signal. Noise reduction techniques, such as spectral subtraction and Wiener filtering, help to remove background noise, while normalization adjusts the audio levels to a consistent range.

Example of capturing and preprocessing audio using Python's librosa library:
import librosa
import numpy as np
# Load audio file
audio_path = 'path_to_audio_file.wav'
audio, sr = librosa.load(audio_path, sr=16000)
# Normalize audio
audio = librosa.util.normalize(audio)
# Reduce noise (simple example using spectral gating)
def noise_reduction(audio, sr):
noise_reduced_audio = librosa.effects.preemphasis(audio)
return noise_reduced_audio
audio = noise_reduction(audio, sr)
# Display audio properties
print(f'Audio shape: {audio.shape}')
print(f'Sampling rate: {sr}')
Feature Extraction
Once the audio input is captured and preprocessed, the next step is feature extraction. This involves converting the raw audio signal into a set of features that can be used by machine learning models. Common features used in speech recognition include Mel-Frequency Cepstral Coefficients (MFCCs), Mel spectrograms, and Chroma features.
MFCCs are widely used in speech recognition because they effectively capture the characteristics of the human voice. They represent the short-term power spectrum of the audio signal on a non-linear Mel scale of frequency. The process of computing MFCCs involves several steps, including framing, windowing, computing the Fourier transform, and applying the Mel filter bank.
Example of extracting MFCCs using librosa:

# Extract MFCC features
mfccs = librosa.feature.mfcc(audio, sr=sr, n_mfcc=13)
# Display MFCC shape
print(f'MFCCs shape: {mfccs.shape}')
Other useful features include the Mel spectrogram, which provides a time-frequency representation of the audio signal, and Chroma features, which capture the harmonic content of the audio. These features can be combined to provide a rich representation of the audio signal for machine learning models.
Training Speech Recognition Models
Training speech recognition models involves using labeled datasets that contain pairs of audio recordings and their corresponding transcriptions. These datasets are used to train machine learning models to learn the mapping from audio features to text. The models are typically trained using supervised learning techniques.
Deep learning models, particularly recurrent neural networks (RNNs) and convolutional neural networks (CNNs), have achieved state-of-the-art performance in speech recognition. RNNs, such as Long Short-Term Memory (LSTM) networks and Gated Recurrent Units (GRUs), are well-suited for sequential data like speech because they can capture temporal dependencies.
Example of training a simple speech recognition model using TensorFlow/Keras:

import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, LSTM, TimeDistributed, Activation
# Define the model
model = Sequential([
LSTM(128, input_shape=(None, 13), return_sequences=True),
TimeDistributed(Dense(64)),
Activation('relu'),
TimeDistributed(Dense(32)),
Activation('relu'),
TimeDistributed(Dense(1)),
Activation('sigmoid')
])
# Compile the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Display model summary
model.summary()
Advanced Techniques in Speech-to-Text Conversion
Connectionist Temporal Classification (CTC)
One of the challenges in speech-to-text conversion is aligning the variable-length audio input with the variable-length text output. Connectionist Temporal Classification (CTC) is a technique used to address this issue. CTC allows the model to output a probability distribution over all possible alignments between the input and output sequences.
CTC introduces a special "blank" token, which represents no output at a given time step. The model learns to predict the most likely sequence of tokens, including blanks, and the final transcription is obtained by collapsing consecutive repeated tokens and removing blanks.
Example of implementing CTC using TensorFlow/Keras:
from tensorflow.keras.layers import Input, Dense, LSTM
from tensorflow.keras.models import Model
import tensorflow.keras.backend as K
# Define the input
input_data = Input(name='input', shape=(None, 13))
# Define the LSTM layers
lstm_1 = LSTM(128, return_sequences=True, name='lstm_1')(input_data)
lstm_2 = LSTM(128, return_sequences=True, name='lstm_2')(lstm_1)
# Define the dense layer
dense = Dense(32, name='dense')(lstm_2)
# Define the CTC layer
ctc = tf.keras.layers.Lambda(lambda x: x, name='ctc')(dense)
# Define the model
model = Model(inputs=input_data, outputs=ctc)
# Define the CTC loss function
def ctc_loss(y_true, y_pred):
return K.ctc_batch_cost(y_true, y_pred, input_length, label_length)
# Compile the model
model.compile(optimizer='adam', loss=ctc_loss)
# Display model summary
model.summary()
Attention Mechanisms
Attention mechanisms have significantly improved the performance of sequence-to-sequence models, including those used in speech recognition. Attention allows the model to focus on relevant parts of the input sequence when making predictions, enhancing its ability to handle long sequences and complex patterns.

In speech recognition, attention mechanisms can be used to align the audio features with the corresponding text output dynamically. This results in more accurate and robust transcriptions, especially for long and noisy audio inputs.
Example of implementing attention mechanism in a speech recognition model using TensorFlow/Keras:
from tensorflow.keras.layers import Layer, Concatenate, Activation
class Attention(Layer):
def __init__(self, **kwargs):
super(Attention, self).__init__(**kwargs)
def build(self, input_shape):
self.W = self.add_weight(name='attention_weight', shape=(input_shape[-1], 1), initializer='random_normal', trainable=True)
super(Attention, self).build(input_shape)
def call(self, x):
e = K.tanh(K.dot(x, self.W))
alpha = K.softmax(e, axis=1)
context = x * alpha
return K.sum(context, axis=1)
# Define the input
input_data = Input(name='input', shape=(None, 13))
# Define the LSTM layers
lstm_1 = LSTM(128, return_sequences=True, name='lstm_1')(input_data)
lstm_2 = LSTM(128, return_sequences=True, name='lstm_2')(lstm_1)
# Apply attention
attention = Attention()(lstm_2)
# Define the dense layer
dense = Dense(32, name='dense')(attention)
# Define the output layer
output = Dense(1, activation='sigmoid', name='output')(dense)
# Define the model
model = Model(inputs=input_data, outputs=output)
# Compile the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Display model summary
model.summary()
Transfer Learning
Transfer learning involves leveraging pre-trained models to improve the performance of speech-to-text systems. Pre-trained models, such as those trained on large speech datasets, can be fine-tuned on specific tasks or domains, reducing the amount of data and training time required.
Transfer learning is particularly useful for adapting speech recognition models to different languages, accents, or environments. By starting with a model that already has a good understanding of general speech patterns, fine-tuning can quickly adapt it to the target domain.

Example of transfer learning using a pre-trained model from Hugging Face's Transformers library:
from transformers import Wav2Vec2ForCTC, Wav2Vec2Tokenizer
import torch
# Load pre-trained model and tokenizer
tokenizer = Wav2Vec2Tokenizer.from_pretrained('facebook/wav2vec2-base-960h')
model = Wav2Vec2ForCTC.from_pretrained('facebook/wav2vec2-base-960h')
# Load and preprocess audio
audio_input, _ = librosa.load('path_to_audio_file.wav', sr=16000)
input_values = tokenizer(audio_input, return_tensors='pt').input_values
# Perform inference
with torch.no_grad():
logits = model(input_values).logits
# Decode the predicted text
predicted_ids = torch.argmax(logits, dim=-1)
transcription = tokenizer.batch_decode(predicted_ids)
print(f'Transcription: {transcription}')
Applications and Advancements in Speech-to-Text
Voice-Activated Assistants
Voice-activated assistants, such as Google Assistant, Amazon Alexa, and Apple Siri, rely heavily on speech-to-text technology to understand and respond to user commands. These assistants use advanced speech recognition models to process natural language and provide relevant responses or actions.
The integration of speech-to-text with natural language processing (NLP) enables these assistants to handle a wide range of tasks, from setting reminders and playing music to controlling smart home devices and providing weather updates. Continuous advancements in speech recognition and NLP are making these assistants more accurate and capable.
Transcription Services
Automatic transcription services convert spoken language into written text, providing valuable tools for various industries such as journalism, education, and legal. Services like Otter.ai and Rev use sophisticated speech-to-text models to transcribe interviews, lectures, meetings, and more.

These services often incorporate features like speaker identification, timestamping, and punctuation, enhancing the usability and readability of the transcriptions. The accuracy and efficiency of these services have significantly improved with advancements in deep learning and large-scale speech datasets.
Accessibility Tools
Speech-to-text technology plays a crucial role in accessibility, providing tools for individuals with hearing impairments or other disabilities. Real-time captioning systems, such as Google Live Transcribe, enable users to read spoken language in real-time, enhancing communication and inclusion.
In educational settings, speech-to-text tools can transcribe lectures and classroom discussions, making learning materials more accessible to students with disabilities. Similarly, in public settings, live captioning can assist individuals in understanding spoken announcements and presentations.
Future Directions
The future of speech-to-text technology is promising, with ongoing research and development focused on improving accuracy, robustness, and adaptability. Key areas of advancement include multilingual speech recognition, where models can seamlessly switch between languages, and robust speech recognition, where models perform well in noisy or challenging environments.
The integration of speech-to-text with other AI technologies, such as sentiment analysis and emotion recognition, can lead to more intuitive and responsive systems. These advancements will continue to enhance the capabilities and applications of speech-to-text technology, driving innovation across various domains.
In conclusion, machine learning has enabled significant advancements in speech-to-text conversion, transforming how we interact with technology and each other. From capturing and preprocessing audio to training sophisticated models and applying advanced techniques, the journey of converting speech to text involves a combination of science, engineering, and creativity. The applications of this technology are vast and impactful, making it an essential component of modern AI systems.
If you want to read more articles similar to Machine Learning: Enabling Speech to Text Conversion, you can visit the Applications category.
You Must Read