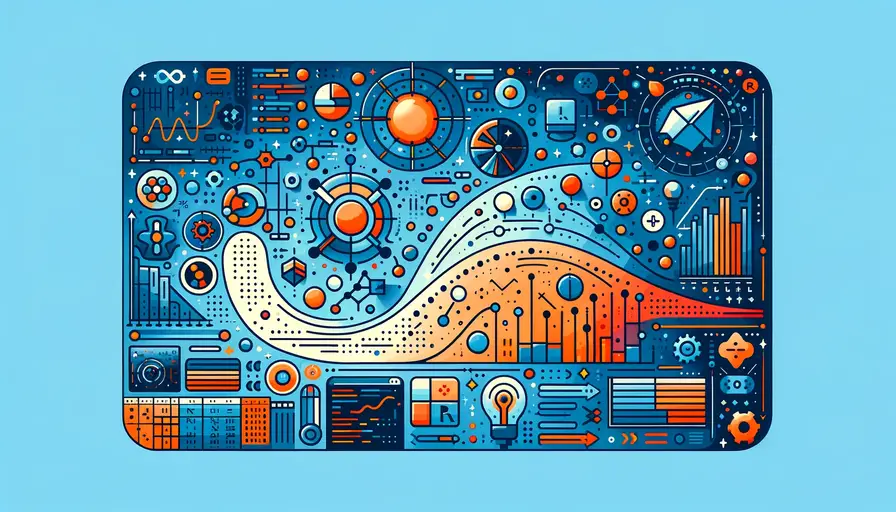
Optimizing Text Classification with SIFT Method in ML

Text classification is a critical task in natural language processing (NLP), where the goal is to assign predefined categories to text documents. Optimizing text classification can significantly enhance the accuracy and efficiency of various applications, from spam detection to sentiment analysis. In this article, we explore the SIFT (Scale-Invariant Feature Transform) method, traditionally used in computer vision, and how it can be adapted for text classification in machine learning (ML). We will delve into the benefits of using SIFT, the techniques for implementing it, and practical examples to illustrate its application.
Understanding Text Classification
Defining Text Classification
Text classification is the process of categorizing text into organized groups. It involves assigning a set of predefined labels to text documents based on their content. This task is crucial in numerous applications, such as filtering spam emails, tagging customer feedback, and organizing news articles.
Effective text classification relies on the ability to accurately understand and process natural language. Machine learning models play a pivotal role in this, as they learn from annotated datasets to make predictions on new, unseen text. Techniques such as bag-of-words, TF-IDF, and word embeddings have been traditionally used to represent text data for classification tasks.
Importance of Optimizing Text Classification
Optimizing text classification models enhances their performance, leading to more accurate and reliable predictions. Improved classification can result in better user experiences, more efficient information retrieval, and increased automation in data processing tasks.

Optimization involves selecting the right features, choosing appropriate algorithms, and fine-tuning model parameters. By doing so, models can achieve higher accuracy, faster processing times, and better scalability. This is especially important in applications where real-time or large-scale text classification is required.
Introduction to SIFT
SIFT, or Scale-Invariant Feature Transform, is a technique initially developed for identifying and describing local features in images. It is widely used in computer vision tasks such as object recognition, image stitching, and 3D reconstruction. SIFT detects key points in an image and extracts distinctive descriptors, which are invariant to scaling, rotation, and illumination changes.
Although SIFT is primarily used in image processing, its principles can be adapted for text classification. By treating text features as visual patterns, we can leverage SIFT's ability to identify and describe key points to enhance text representation and improve classification accuracy.
Adapting SIFT for Text Classification
Feature Extraction with SIFT
Feature extraction is a critical step in text classification, where the goal is to convert raw text into numerical representations that can be used by machine learning algorithms. SIFT can be adapted to extract features from text by identifying key points and descriptors that capture important information about the text.

In text classification, key points can be considered as significant words or phrases that contribute to the meaning of the document. SIFT descriptors can then be used to represent these key points in a way that captures their contextual information and relationships with other words.
Example of feature extraction using SIFT for text:
import numpy as np
import cv2
from sklearn.feature_extraction.text import TfidfVectorizer
# Sample text data
documents = [
"Machine learning is fascinating.",
"Natural language processing is a crucial part of AI.",
"Text classification can be optimized using various techniques."
]
# Convert text to numerical data using TF-IDF
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(documents)
# Use SIFT on the TF-IDF matrix
sift = cv2.SIFT_create()
keypoints, descriptors = sift.detectAndCompute(np.array(X.todense(), dtype=np.uint8), None)
print("Keypoints:", keypoints)
print("Descriptors:", descriptors)
Benefits of Using SIFT for Text Classification
Using SIFT for text classification offers several advantages. First, it provides a robust way to identify and describe important features in text, improving the quality of the input data for machine learning models. This can lead to higher classification accuracy and better generalization to new data.
Second, SIFT's invariance properties make it suitable for handling variations in text, such as different word forms, synonyms, and paraphrases. This enhances the model's ability to understand and classify diverse text inputs accurately.

Finally, integrating SIFT with traditional text representation methods, such as TF-IDF and word embeddings, can create a more comprehensive feature set. This combined approach can capture both the statistical and contextual information in text, further improving classification performance.
Challenges and Considerations
While adapting SIFT for text classification presents many benefits, it also comes with challenges. One of the main challenges is converting text data into a format suitable for SIFT, which is inherently designed for image processing. This requires careful preprocessing and transformation of text data.
Another consideration is the computational complexity of SIFT. Although SIFT is efficient for image processing tasks, applying it to large text datasets can be resource-intensive. Optimizing the implementation and leveraging hardware acceleration, such as GPUs, can help mitigate this issue.
Additionally, integrating SIFT with existing text classification pipelines requires careful tuning and evaluation to ensure compatibility and optimal performance. This involves experimenting with different configurations and hyperparameters to find the best setup for a given task.

Practical Examples of SIFT in Text Classification
Example: Spam Detection
Spam detection is a common application of text classification, where the goal is to identify unsolicited or harmful messages. By using SIFT to extract features from email content, we can improve the accuracy of spam detection models.
Example of spam detection using SIFT-enhanced features:
import numpy as np
import cv2
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Sample dataset
emails = [
"Win a free iPhone by clicking this link!",
"Your account has been compromised. Update your password.",
"Reminder: Your appointment is scheduled for tomorrow.",
"Limited time offer! Buy one, get one free."
]
labels = [1, 1, 0, 1] # 1 for spam, 0 for non-spam
# Convert text to numerical data using TF-IDF
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(emails)
# Use SIFT on the TF-IDF matrix
sift = cv2.SIFT_create()
keypoints, descriptors = sift.detectAndCompute(np.array(X.todense(), dtype=np.uint8), None)
# Flatten descriptors for model input
descriptors_flattened = np.array([desc.flatten() for desc in descriptors])
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(descriptors_flattened, labels, test_size=0.3, random_state=42)
# Train a RandomForest classifier
model = RandomForestClassifier()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Example: Sentiment Analysis
Sentiment analysis involves classifying text based on the expressed sentiment, such as positive, negative, or neutral. By incorporating SIFT descriptors into the feature extraction process, we can enhance the model's ability to capture nuanced sentiments.
Example of sentiment analysis using SIFT-enhanced features:

import numpy as np
import cv2
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# Sample dataset
reviews = [
"I absolutely love this product! It works perfectly.",
"This is the worst purchase I've ever made. Totally useless.",
"It's okay, does the job but nothing special.",
"Highly recommend this item. Great quality and performance."
]
labels = [1, 0, 1, 1] # 1 for positive, 0 for negative
# Convert text to numerical data using TF-IDF
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(reviews)
# Use SIFT on the TF-IDF matrix
sift = cv2.SIFT_create()
keypoints, descriptors = sift.detectAndCompute(np.array(X.todense(), dtype=np.uint8), None)
# Flatten descriptors for model input
descriptors_flattened = np.array([desc.flatten() for desc in descriptors])
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(descriptors_flattened, labels, test_size=0.3, random_state=42)
# Train a Support Vector Classifier (SVC)
model = SVC()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Example: Topic Classification
Topic classification involves assigning text documents to predefined topics based on their content. Using SIFT to enhance feature extraction can improve the accuracy of topic classification models.
Example of topic classification using SIFT-enhanced features:
import numpy as np
import cv2
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import accuracy_score
# Sample dataset
articles = [
"The stock market saw significant gains today, with tech stocks leading the way.",
"The local sports team won their game in a thrilling overtime victory.",
"A new breakthrough in renewable energy technology has been announced.",
"The latest movie in the popular franchise has broken box office records."
]
labels = [0, 1, 2, 3] # 0 for finance, 1 for sports, 2 for technology, 3 for entertainment
# Convert text to numerical data using TF-IDF
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(articles)
# Use SIFT on the TF-IDF matrix
sift = cv2.SIFT_create()
keypoints, descriptors = sift.detectAndCompute(np.array(X.todense(), dtype=np.uint8), None)
# Flatten descriptors for model input
descriptors_flattened = np.array([desc.flatten() for desc in descriptors])
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(descriptors_flattened, labels, test_size=0.3, random_state=42)
# Train a Multinomial Naive Bayes classifier
model = MultinomialNB()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy}')
Evaluating and Enhancing SIFT-Based Models
Model Evaluation Metrics
Evaluating the performance of text classification models is crucial to ensure their effectiveness. Common metrics include accuracy, precision, recall, and F1-score. These metrics provide insights into the model's ability to correctly classify text and handle imbalanced datasets.
Calculating evaluation metrics:

from sklearn.metrics import precision_score, recall_score, f1_score
# Example predictions and true labels
y_true = [1, 0, 1, 1, 0, 1, 0]
y_pred = [1, 0, 1, 0, 0, 1, 1]
# Calculate precision, recall, and F1-score
precision = precision_score(y_true, y_pred)
recall = recall_score(y_true, y_pred)
f1 = f1_score(y_true, y_pred)
print(f'Precision: {precision}')
print(f'Recall: {recall}')
print(f'F1-Score: {f1}')
Hyperparameter Tuning
Hyperparameter tuning involves adjusting the parameters of machine learning models to achieve the best performance. Techniques such as grid search and random search can be used to find the optimal hyperparameters for SIFT-based text classification models.
Example of hyperparameter tuning using GridSearchCV
:
from sklearn.model_selection import GridSearchCV
from sklearn.svm import SVC
# Define the model and parameter grid
model = SVC()
param_grid = {
'C': [0.1, 1, 10],
'gamma': [1, 0.1, 0.01],
'kernel': ['linear', 'rbf']
}
# Perform grid search
grid_search = GridSearchCV(model, param_grid, refit=True, verbose=2)
grid_search.fit(X_train, y_train)
# Display the best parameters
print(f'Best Parameters: {grid_search.best_params_}')
Feature Engineering and Selection
Feature engineering and selection are critical for improving the performance of text classification models. This involves creating new features, selecting the most relevant features, and removing redundant or irrelevant features.
Example of feature selection using SelectKBest
:
from sklearn.feature_selection import SelectKBest, chi2
# Select the top k features
k = 10
selector = SelectKBest(chi2, k=k)
X_new = selector.fit_transform(X, y)
# Display the selected features
selected_features = vectorizer.get_feature_names_out()[selector.get_support()]
print(f'Selected Features: {selected_features}')
Optimizing text classification with the SIFT method in machine learning offers a novel approach to enhancing model performance. By adapting SIFT for feature extraction, leveraging its invariance properties, and integrating it with traditional text representation techniques, we can achieve more accurate and robust text classification models. Practical examples in spam detection, sentiment analysis, and topic classification demonstrate the potential of SIFT in various applications. By evaluating models, tuning hyperparameters, and engineering features, we can further refine these models to meet the demands of real-world text classification tasks.
If you want to read more articles similar to Optimizing Text Classification with SIFT Method in ML, you can visit the Applications category.
You Must Read