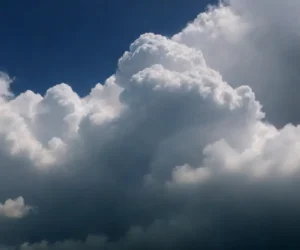
Python-Based Machine Learning: A Student's Guide
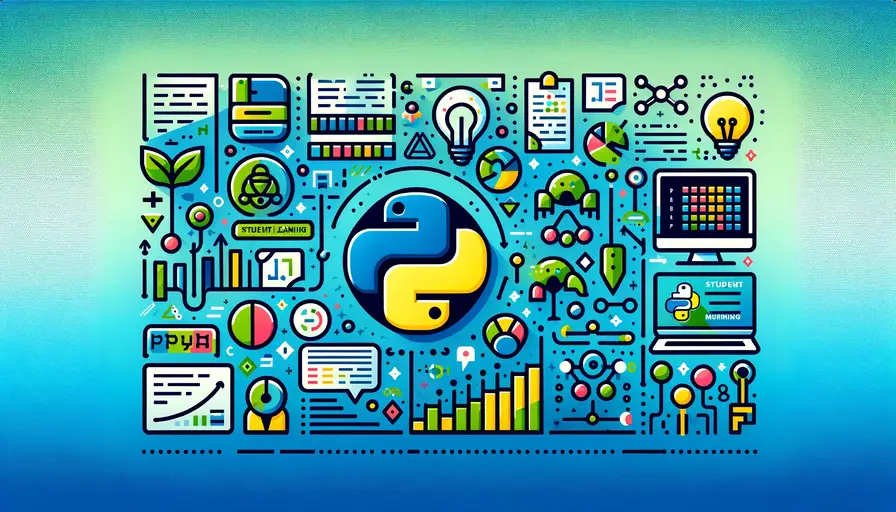
Getting Started with Python for Machine Learning
Setting Up Your Environment
To embark on your machine learning journey with Python, the first task is setting up your environment. Begin by installing Python, preferably version 3.7 or later, from the official Python website. Once Python is installed, you will need an Integrated Development Environment (IDE) to write and run your code. PyCharm, Visual Studio Code, and Jupyter Notebook are popular choices among machine learning practitioners.
Installing essential libraries is the next step. Use pip to install libraries such as NumPy, Pandas, and scikit-learn. These libraries provide powerful tools for data manipulation, analysis, and machine learning. Open your terminal or command prompt and run the following commands:
pip install numpy pandas scikit-learn
Finally, ensure that your environment is correctly set up by creating a simple Python script or Jupyter Notebook that imports these libraries. This setup ensures you are ready to dive into the exciting world of machine learning with Python.
Understanding Basic Python Syntax
Before diving into machine learning, a solid understanding of basic Python syntax is crucial. Python's simplicity and readability make it an ideal language for beginners. Key concepts to master include variables, data types, control structures (such as loops and conditionals), and functions. These fundamentals form the building blocks of more complex machine learning algorithms and data processing tasks.
Essential Skills for Becoming a Machine Learning Data AnalystPython's data structures, such as lists, dictionaries, and sets, are also essential. Lists are ordered collections of items, while dictionaries store data in key-value pairs. Sets are unordered collections of unique items. Understanding how to manipulate these structures is critical for handling data efficiently.
For example, here is a simple Python function that takes a list of numbers and returns their sum:
def sum_numbers(numbers):
total = 0
for number in numbers:
total += number
return total
# Example usage
numbers = [1, 2, 3, 4, 5]
print(sum_numbers(numbers))
This function iterates through the list, adds each number to a running total, and returns the sum. Mastering such basics will prepare you to tackle more complex tasks in machine learning.
Working with Data in Pandas
Pandas is a powerful library for data manipulation and analysis. It provides data structures such as DataFrames, which are essential for handling and analyzing data. Understanding how to load, manipulate, and analyze data using Pandas is a critical skill in machine learning.
Is Polynomial Regression a Machine Learning Algorithm?To get started with Pandas, you need to know how to read data from various sources, such as CSV files. The read_csv
function allows you to load data into a DataFrame. Here’s an example:
import pandas as pd
# Load data from a CSV file
data = pd.read_csv('data.csv')
# Display the first few rows of the DataFrame
print(data.head())
Once the data is loaded, you can perform various operations, such as filtering, grouping, and aggregating. Pandas also provides tools for data cleaning, such as handling missing values and removing duplicates. By mastering these techniques, you can prepare your data for machine learning models.
Building Your First Machine Learning Model
Understanding Supervised Learning
Supervised learning is a fundamental concept in machine learning where the model learns from labeled data. In supervised learning, you have input features (X) and an output label (y). The goal is to learn a mapping from X to y, which can be used to make predictions on new, unseen data. Supervised learning tasks are divided into two main types: regression and classification.
Regression tasks involve predicting a continuous value. For example, predicting the price of a house based on its features is a regression problem. Classification tasks, on the other hand, involve predicting a discrete label. For instance, classifying emails as spam or not spam is a classification problem.
Is a Mathematical Foundation Necessary for Machine Learning?A popular algorithm for supervised learning is the linear regression for regression tasks and logistic regression for classification tasks. Both algorithms are straightforward to implement using libraries like scikit-learn. Understanding these basic algorithms will provide a strong foundation for more advanced models.
Implementing Linear Regression
Linear regression is a simple yet powerful algorithm for predicting a continuous target variable. It assumes a linear relationship between the input features and the target variable. The goal is to find the best-fitting line that minimizes the sum of the squared differences between the predicted and actual values.
Here is an example of how to implement linear regression using scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Load data
data = pd.read_csv('data.csv')
# Define input features (X) and target variable (y)
X = data[['feature1', 'feature2']]
y = data['target']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
mse = mean_squared_error(y_test, y_pred)
print(f"Mean Squared Error: {mse}")
In this example, we load a dataset, split it into training and testing sets, and train a linear regression model. We then make predictions on the test set and evaluate the model's performance using the mean squared error metric.
Python Tutorial: Data Cleaning and Preprocessing for MLImplementing Logistic Regression
Logistic regression is a classification algorithm used to predict binary outcomes. Unlike linear regression, which predicts a continuous value, logistic regression predicts the probability that a given input belongs to a particular class. The algorithm uses the logistic function to model the probability.
Here is an example of how to implement logistic regression using scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Load data
data = pd.read_csv('data.csv')
# Define input features (X) and target variable (y)
X = data[['feature1', 'feature2']]
y = data['target']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
In this example, we load a dataset, split it into training and testing sets, and train a logistic regression model. We then make predictions on the test set and evaluate the model's performance using the accuracy metric.
Exploring Advanced Machine Learning Techniques
Decision Trees and Random Forests
Decision trees are versatile machine learning algorithms that can be used for both regression and classification tasks. They work by recursively splitting the data into subsets based on the value of the input features. Each split is chosen to maximize the separation between classes (for classification) or reduce variance (for regression).
Mastering Machine Learning: How Long Does It Really Take to Learn?Random forests are an ensemble learning method that combines multiple decision trees to improve performance and reduce overfitting. By averaging the predictions of multiple trees, random forests achieve higher accuracy and robustness.
Here is an example of how to implement a random forest classifier using scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load data
data = pd.read_csv('data.csv')
# Define input features (X) and target variable (y)
X = data[['feature1', 'feature2']]
y = data['target']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
In this example, we implement a random forest classifier to predict a target variable. The model is trained on the training set and evaluated on the test set using the accuracy metric.
Support Vector Machines
Support Vector Machines (SVMs) are powerful supervised learning algorithms used for classification and regression tasks. SVMs work by finding the hyperplane that best separates the data into different classes. The hyperplane is chosen to maximize the margin between the classes, providing robust classification boundaries.
Master Machine Learning in Python at JavatpointSVMs can handle both linear and non-linear classification problems. For non-linear classification, SVMs use kernel functions to transform the input features into higher-dimensional spaces where a linear separation is possible.
Here is an example of how to implement an SVM classifier using scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# Load data
data = pd.read_csv('data.csv')
# Define input features (X) and target variable (y)
X = data[['feature1', 'feature2']]
y = data['target']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = SVC(kernel='linear')
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
In this example, we implement an SVM classifier with a linear kernel to predict a target variable. The model is trained on the training set and evaluated on the test set using the accuracy metric.
Neural Networks and Deep Learning
Neural networks are a class of machine learning algorithms inspired by the structure of the human brain. They consist of layers of interconnected neurons that process input data to make predictions. Deep learning is a subset of machine learning that uses neural networks with many layers (deep neural networks) to model complex patterns and relationships in data.
Deep learning has achieved remarkable success in various domains, including image recognition, natural language processing, and speech recognition. Libraries such as TensorFlow and Keras make it easy to build and train neural networks.
Here is an example of how to implement a simple neural network using Keras:
import numpy as np
import pandas as pd
from keras.models import Sequential
from keras.layers import Dense
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.metrics import accuracy_score
# Load data
data = pd.read_csv('data.csv')
# Define input features (X) and target variable (y)
X = data[['feature1', 'feature2']]
y = data['target']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Standardize the input features
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
# Create the neural network model
model = Sequential()
model.add(Dense(32, input_dim=X_train.shape[1], activation='relu'))
model.add(Dense(16, activation='relu'))
model.add(Dense(1, activation='sigmoid'))
# Compile the model
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=50, batch_size=10, verbose=1)
# Make predictions
y_pred = (model.predict(X_test) > 0.5).astype('int32')
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
In this example, we implement a simple neural network to predict a binary target variable. The model consists of two hidden layers with ReLU activation and an output layer with sigmoid activation. The model is trained on the training set and evaluated on the test set using the accuracy metric.
Evaluating and Improving Your Models
Cross-Validation
Cross-validation is a technique used to evaluate the performance of a machine learning model. It involves splitting the data into multiple subsets (folds) and training the model on different combinations of these subsets. This approach provides a more robust estimate of the model's performance by reducing the impact of random variations in the data.
The most common form of cross-validation is k-fold cross-validation, where the data is divided into k folds. The model is trained on k-1 folds and tested on the remaining fold. This process is repeated k times, with each fold serving as the test set once. The results are then averaged to obtain a final performance estimate.
Here is an example of how to perform k-fold cross-validation using scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier
# Load data
data = pd.read_csv('data.csv')
# Define input features (X) and target variable (y)
X = data[['feature1', 'feature2']]
y = data['target']
# Create the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
# Perform k-fold cross-validation
scores = cross_val_score(model, X, y, cv=5)
# Print the cross-validation scores
print(f"Cross-validation scores: {scores}")
print(f"Mean cross-validation score: {scores.mean()}")
In this example, we perform 5-fold cross-validation to evaluate a random forest classifier. The cross-validation scores provide an estimate of the model's performance.
Hyperparameter Tuning
Hyperparameter tuning is the process of optimizing the hyperparameters of a machine learning model to improve its performance. Hyperparameters are settings that control the behavior of the model, such as the learning rate, the number of trees in a random forest, or the number of layers in a neural network.
There are several techniques for hyperparameter tuning, including grid search and random search. Grid search involves exhaustively searching through a predefined set of hyperparameter values, while random search samples hyperparameter values randomly. Both techniques can be implemented using scikit-learn.
Here is an example of how to perform grid search for hyperparameter tuning using scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
# Load data
data = pd.read_csv('data.csv')
# Define input features (X) and target variable (y)
X = data[['feature1', 'feature2']]
y = data['target']
# Create the model
model = RandomForestClassifier(random_state=42)
# Define the hyperparameter grid
param_grid = {
'n_estimators': [50, 100, 150],
'max_depth': [None, 10, 20, 30]
}
# Perform grid search
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X, y)
# Print the best hyperparameters
print(f"Best hyperparameters: {grid_search.best_params_}")
In this example, we perform grid search to optimize the hyperparameters of a random forest classifier. The best hyperparameters are identified based on the cross-validation scores.
Model Evaluation Metrics
Evaluating the performance of a machine learning model requires using appropriate metrics. Different metrics are suitable for different tasks, such as classification or regression. Common evaluation metrics for classification include accuracy, precision, recall, and F1 score. For regression tasks, metrics such as mean squared error (MSE), mean absolute error (MAE), and R-squared are commonly used.
Accuracy measures the proportion of correct predictions, while precision and recall evaluate the model's performance on the positive class. The F1 score is the harmonic mean of precision and recall, providing a balanced measure of both metrics. For regression tasks, MSE measures the average squared difference between the predicted and actual values, while MAE measures the average absolute difference.
Here is an example of how to calculate evaluation metrics using scikit-learn:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
# Load data
data = pd.read_csv('data.csv')
# Define input features (X) and target variable (y)
X = data[['feature1', 'feature2']]
y = data['target']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Calculate evaluation metrics
accuracy = accuracy_score(y_test, y_pred)
precision = precision_score(y_test, y_pred)
recall = recall_score(y_test, y_pred)
f1 = f1_score(y_test, y_pred)
# Print the evaluation metrics
print(f"Accuracy: {accuracy}")
print(f"Precision: {precision}")
print(f"Recall: {recall}")
print(f"F1 Score: {f1}")
In this example, we calculate evaluation metrics for a random forest classifier. These metrics provide a comprehensive assessment of the model's performance.
Machine learning with Python offers endless possibilities for students and enthusiasts. By mastering the basics, exploring advanced techniques, and continually evaluating and improving your models, you can harness the power of machine learning to solve complex problems and create innovative solutions. This guide provides a solid foundation for your journey into the exciting world of machine learning.
If you want to read more articles similar to Python-Based Machine Learning: A Student's Guide, you can visit the Education category.
You Must Read