
Unveiling the Advanced ML Chatbot: Exploring New Frontiers
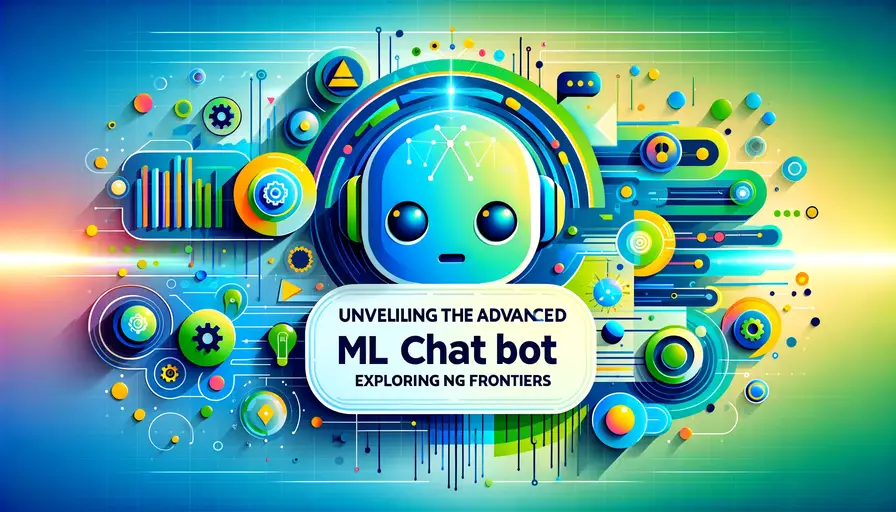
Machine learning (ML) chatbots have become an integral part of modern communication, enhancing customer service, streamlining business operations, and providing personalized user experiences. This article delves into the advancements in ML chatbots, examining their development, applications, and future potential. We will explore key concepts, implementation strategies, and provide detailed code examples to help you create and optimize ML chatbots.
Evolution of ML Chatbots
Early Chatbots and Their Limitations
Early chatbots were primarily rule-based systems that relied on predefined scripts and keyword matching to interact with users. These chatbots followed a rigid set of rules to process user inputs and generate responses, making them relatively simple and easy to implement. However, they had significant limitations.
One major limitation was their inability to understand natural language nuances. Rule-based chatbots could only respond accurately to inputs that closely matched their predefined patterns. This often led to frustration for users when the chatbot failed to understand or respond appropriately to more complex or varied inputs.
Another limitation was the lack of contextual awareness. Early chatbots could not maintain a conversation context, leading to disjointed and unhelpful interactions. This made it difficult for users to have meaningful conversations with the chatbot, as it could not remember previous interactions or understand the context of ongoing dialogues.
Exploring Practical Machine Learning Applications for IoTAdvancements in Machine Learning
Advancements in machine learning have revolutionized chatbot development, enabling the creation of more sophisticated and intelligent systems. ML algorithms, particularly those in natural language processing (NLP) and deep learning, have significantly improved chatbots' ability to understand and generate human-like responses.
Natural language processing techniques allow chatbots to parse and comprehend user inputs more effectively. By leveraging NLP, chatbots can analyze the syntax, semantics, and context of user messages, resulting in more accurate and relevant responses. Techniques such as named entity recognition (NER), sentiment analysis, and part-of-speech tagging play a crucial role in enhancing chatbot interactions.
Deep learning, especially through neural networks, has further propelled chatbot capabilities. Recurrent neural networks (RNNs) and transformers, such as GPT-3 from OpenAI, enable chatbots to generate coherent and contextually appropriate responses. These models can process large volumes of text data, learn from context, and generate human-like text, making interactions with chatbots more natural and engaging.
Modern Chatbot Architectures
Modern chatbot architectures combine multiple machine learning techniques to create highly responsive and context-aware systems. These architectures often involve integrating various components, such as intent recognition, dialogue management, and response generation, to handle complex interactions seamlessly.
Advanced Conversational AI Techniques by ChatGPTIntent recognition is a fundamental aspect of modern chatbots. By identifying the user's intent, chatbots can determine the appropriate action or response. Techniques like support vector machines (SVMs), random forests, and deep learning models are commonly used for intent classification.
Dialogue management systems manage the flow of conversation, maintaining context and handling multi-turn interactions. These systems use state machines, rule-based approaches, or reinforcement learning to manage dialogue states and transitions. Dialogue management ensures that chatbots can handle complex queries and maintain coherent conversations.
Response generation is the final step, where chatbots formulate and deliver responses. This can involve template-based approaches, retrieval-based methods, or generative models. Template-based responses use predefined templates to generate answers, while retrieval-based methods select the most relevant response from a predefined set. Generative models, powered by neural networks, can create unique and contextually appropriate responses on the fly.
Building an ML Chatbot
Setting Up the Development Environment
Setting up the development environment is crucial for building an ML chatbot. You need to install essential tools and libraries that facilitate machine learning, natural language processing, and chatbot development. Python is a popular choice for building ML chatbots due to its extensive libraries and community support.
Automating Software Testing with Machine Learning and NLPStart by installing Python from the official Python website. Once Python is installed, you can set up a virtual environment to manage dependencies for your chatbot project. Virtual environments help isolate your project’s dependencies, ensuring that different projects do not conflict with each other.
Next, install essential libraries like TensorFlow, PyTorch, and NLTK. TensorFlow and PyTorch are popular deep learning frameworks that provide tools for building and training neural networks. NLTK (Natural Language Toolkit) is a comprehensive library for natural language processing in Python, offering tools for text processing, tokenization, and more.
Here’s how you can set up a virtual environment and install the necessary libraries:
# Create a virtual environment
python -m venv chatbot_env
# Activate the virtual environment
# On Windows
chatbot_env\Scripts\activate
# On macOS/Linux
source chatbot_env/bin/activate
# Install necessary libraries
pip install tensorflow torch nltk
Implementing Natural Language Processing
Implementing natural language processing (NLP) is a crucial step in building an ML chatbot. NLP techniques enable the chatbot to understand and process user inputs effectively. This involves text preprocessing, intent recognition, and entity extraction.
Exciting Machine Learning Projects to Spark Your InterestText preprocessing involves cleaning and transforming text data into a format suitable for analysis. This includes tasks like tokenization, stemming, lemmatization, and removing stop words. Tokenization breaks down text into individual words or tokens, while stemming and lemmatization reduce words to their base forms.
Intent recognition is essential for understanding the user's purpose or goal. This can be achieved using classification algorithms, where the chatbot is trained on labeled data to recognize different intents. Libraries like scikit-learn, TensorFlow, and PyTorch provide tools for training and implementing intent classifiers.
Entity extraction involves identifying and extracting specific information from user inputs. Named entity recognition (NER) models can be used to identify entities like names, dates, locations, and more. This information is crucial for providing accurate and relevant responses.
Here’s an example of implementing text preprocessing and intent recognition using NLTK and TensorFlow:
Complete Guide to End-to-End Machine Learning Projectsimport nltk
from nltk.tokenize import word_tokenize
from nltk.stem import WordNetLemmatizer
import tensorflow as tf
from sklearn.preprocessing import LabelEncoder
import numpy as np
# Download NLTK resources
nltk.download('punkt')
nltk.download('wordnet')
# Sample data
texts = ["Hello, how can I help you?", "I want to book a flight", "What's the weather like?"]
intents = ["greeting", "booking", "weather"]
# Text preprocessing
lemmatizer = WordNetLemmatizer()
processed_texts = []
for text in texts:
tokens = word_tokenize(text.lower())
lemmatized_tokens = [lemmatizer.lemmatize(token) for token in tokens]
processed_texts.append(" ".join(lemmatized_tokens))
# Encode labels
label_encoder = LabelEncoder()
encoded_intents = label_encoder.fit_transform(intents)
# Create a simple neural network for intent recognition
model = tf.keras.Sequential([
tf.keras.layers.Input(shape=(None,), dtype="int32"),
tf.keras.layers.Embedding(input_dim=1000, output_dim=64),
tf.keras.layers.GlobalAveragePooling1D(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(len(np.unique(encoded_intents)), activation='softmax')
])
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Convert texts to sequences
tokenizer = tf.keras.preprocessing.text.Tokenizer()
tokenizer.fit_on_texts(processed_texts)
sequences = tokenizer.texts_to_sequences(processed_texts)
padded_sequences = tf.keras.preprocessing.sequence.pad_sequences(sequences, padding='post')
# Train the model
model.fit(padded_sequences, encoded_intents, epochs=10)
# Save the model and tokenizer
model.save('intent_model.h5')
with open('tokenizer.pickle', 'wb') as handle:
pickle.dump(tokenizer, handle, protocol=pickle.HIGHEST_PROTOCOL)
Integrating Dialogue Management
Integrating dialogue management into your ML chatbot is essential for maintaining coherent and context-aware conversations. Dialogue management systems track the state of the conversation, handle user intents, and generate appropriate responses based on the context.
Rule-based dialogue management uses predefined rules to manage dialogue states and transitions. This approach is simple and easy to implement but lacks flexibility for handling complex interactions. Machine learning-based dialogue management uses algorithms to learn and manage dialogue flows, offering greater adaptability and scalability.
Reinforcement learning (RL) is a popular technique for dialogue management. RL algorithms learn optimal dialogue policies by interacting with users and receiving feedback in the form of rewards or penalties. This enables the chatbot to improve its performance over time by learning from successful and unsuccessful interactions.
Here’s an example of integrating dialogue management using a rule-based approach:
Writing Data for Machine Learning Algorithmsclass DialogueManager:
def __init__(self):
self.intents = {
"greeting": self.handle_greeting,
"booking": self.handle_booking,
"weather": self.handle_weather
}
self.state = "initial"
def handle_greeting(self, user_input):
return "Hello! How can I assist you today?"
def handle_booking(self, user_input):
return "Sure, I can help you book a flight. Where would you like to go?"
def handle_weather(self, user_input):
return "The weather is sunny and pleasant. Do you need more details?"
def manage_dialogue(self, intent, user_input):
if intent in self.intents:
response = self.intents[intent](user_input)
return response
else:
return "I'm not sure how to help with that. Can you please rephrase?"
# Example usage
dialogue_manager = DialogueManager()
user_input = "I want to book a flight"
intent = "booking" # This would be predicted by your intent recognition model
response = dialogue_manager.manage_dialogue(intent, user_input)
print
(response)
Enhancing Chatbot Capabilities
Contextual Awareness and Memory
Contextual awareness and memory are critical for creating advanced ML chatbots that can maintain coherent conversations over multiple turns. By keeping track of the conversation context, chatbots can provide more relevant and personalized responses, enhancing the user experience.
Maintaining context involves storing and retrieving information from previous interactions. This can be achieved using dialogue state tracking and memory mechanisms. Dialogue state tracking keeps track of the conversation state, including user intents, entities, and any actions taken by the chatbot.
Memory mechanisms, such as long short-term memory (LSTM) networks and attention mechanisms, enable chatbots to remember and leverage information from past interactions. These techniques help chatbots handle complex queries, follow-up questions, and provide consistent responses.
Here’s an example of integrating context tracking using a simple state management approach:
class ContextManager:
def __init__(self):
self.context = {}
def update_context(self, key, value):
self.context[key] = value
def get_context(self, key):
return self.context.get(key, None)
# Example usage
context_manager = ContextManager()
context_manager.update_context("destination", "Paris")
context_manager.update_context("travel_date", "2022-09-15")
# Retrieve context information
destination = context_manager.get_context("destination")
travel_date = context_manager.get_context("travel_date")
print(f"Destination: {destination}, Travel Date: {travel_date}")
Personalization and User Profiling
Personalization and user profiling are essential for delivering customized experiences to users. By leveraging user data, chatbots can tailor their responses and recommendations to match individual preferences, behaviors, and needs.
User profiling involves collecting and analyzing data about user preferences, behaviors, and demographics. This data can be used to create personalized responses, recommend products or services, and provide a more engaging user experience. Machine learning models can analyze user data to identify patterns and preferences, enabling chatbots to offer relevant suggestions and assistance.
Personalization can be implemented using collaborative filtering, content-based filtering, and hybrid recommendation systems. Collaborative filtering recommends items based on the preferences of similar users, while content-based filtering recommends items based on the user's past interactions and preferences.
Here’s an example of implementing a simple content-based recommendation system:
import pandas as pd
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
# Sample user data
user_data = {
"user_id": [1, 2, 3],
"preferences": ["travel, flights", "weather, news", "movies, entertainment"]
}
df_users = pd.DataFrame(user_data)
# Sample item data
item_data = {
"item_id": [101, 102, 103],
"description": ["Cheap flights to Paris", "Latest weather updates", "Top movies to watch"]
}
df_items = pd.DataFrame(item_data)
# Compute TF-IDF vectors for user preferences and item descriptions
vectorizer = TfidfVectorizer()
tfidf_user = vectorizer.fit_transform(df_users["preferences"])
tfidf_item = vectorizer.transform(df_items["description"])
# Compute cosine similarity between user preferences and item descriptions
similarity_matrix = cosine_similarity(tfidf_user, tfidf_item)
# Recommend items for each user based on similarity
recommendations = {}
for user_idx, user_id in enumerate(df_users["user_id"]):
similar_items = similarity_matrix[user_idx].argsort()[::-1]
recommendations[user_id] = df_items["item_id"].iloc[similar_items].tolist()
print(recommendations)
Handling Multi-Language Support
Handling multi-language support is increasingly important for chatbots in today's globalized world. By supporting multiple languages, chatbots can reach a broader audience and provide more inclusive services. Natural language processing techniques and translation APIs can be used to enable multi-language support in chatbots.
Machine translation services, such as Google Translate API and Microsoft Translator API, can be integrated into chatbots to translate user inputs and generate responses in different languages. This allows chatbots to interact with users in their preferred language, enhancing accessibility and user satisfaction.
Multi-language support can also be achieved by training language-specific models for intent recognition and response generation. This approach ensures that the chatbot can understand and respond accurately in each supported language, providing a seamless user experience.
Here’s an example of integrating Google Translate API for multi-language support:
from googletrans import Translator
# Initialize Google Translate API
translator = Translator()
# Translate user input to English
user_input = "Quiero reservar un vuelo"
translated_input = translator.translate(user_input, src='es', dest='en').text
print(f"Translated Input: {translated_input}")
# Generate response in English
response = "Sure, I can help you book a flight. Where would you like to go?"
# Translate response back to Spanish
translated_response = translator.translate(response, src='en', dest='es').text
print(f"Translated Response: {translated_response}")
Future Trends in ML Chatbots
Integration with Voice Assistants
Integration with voice assistants is a growing trend in the development of ML chatbots. Voice assistants like Amazon Alexa, Google Assistant, and Apple Siri enable users to interact with chatbots using natural language speech, providing a more intuitive and hands-free experience.
Integrating chatbots with voice assistants involves using speech recognition and text-to-speech (TTS) technologies. Speech recognition converts spoken language into text, allowing the chatbot to process and understand user inputs. TTS converts text responses generated by the chatbot into spoken language, enabling seamless voice interactions.
Voice assistants can enhance chatbot capabilities by providing additional functionalities, such as voice commands, hands-free operation, and integration with smart home devices. This integration can improve user convenience, accessibility, and engagement.
Here’s an example of integrating speech recognition and TTS using Python's speech_recognition
and pyttsx3
libraries:
import speech_recognition as sr
import pyttsx3
# Initialize speech recognizer and text-to-speech engine
recognizer = sr.Recognizer()
tts_engine = pyttsx3.init()
# Recognize speech input from the microphone
with sr.Microphone() as source:
print("Listening...")
audio = recognizer.listen(source)
try:
user_input = recognizer.recognize_google(audio)
print(f"User Input: {user_input}")
except sr.UnknownValueError:
print("Sorry, I could not understand the audio.")
except sr.RequestError:
print("Sorry, there was a problem with the speech recognition service.")
# Generate chatbot response
response = "Sure, I can help you book a flight. Where would you like to go?"
# Convert response to speech
tts_engine.say(response)
tts_engine.runAndWait()
Emotion Recognition and Sentiment Analysis
Emotion recognition and sentiment analysis are emerging trends that enable chatbots to understand and respond to users' emotional states. By analyzing the sentiment and emotions expressed in user inputs, chatbots can provide more empathetic and contextually appropriate responses.
Sentiment analysis involves classifying text as positive, negative, or neutral based on the emotional tone. Emotion recognition goes a step further by identifying specific emotions, such as happiness, sadness, anger, or fear. These techniques can enhance user experience by making interactions with the chatbot feel more human and emotionally aware.
Machine learning models, such as recurrent neural networks (RNNs) and transformers, can be trained on labeled datasets to perform sentiment analysis and emotion recognition. Pre-trained models, like BERT and GPT-3, can also be fine-tuned for these tasks, leveraging their ability to understand and generate text.
Here’s an example of performing sentiment analysis using the Hugging Face Transformers library:
from transformers import pipeline
# Initialize sentiment analysis pipeline
sentiment_analyzer = pipeline('sentiment-analysis')
# Analyze sentiment of user input
user_input = "I am really happy with the service!"
sentiment = sentiment_analyzer(user_input)
print(f"Sentiment: {sentiment}")
Autonomous Learning and Adaptation
Autonomous learning and adaptation are future trends that will enable chatbots to improve their performance over time without explicit retraining. By continuously learning from user interactions, feedback, and new data, chatbots can adapt to changing user needs and preferences.
Reinforcement learning (RL) is a key technique for enabling autonomous learning in chatbots. RL algorithms allow chatbots to learn optimal dialogue policies by interacting with users and receiving feedback. This continuous learning process helps chatbots refine their responses and improve user satisfaction.
Transfer learning is another approach that enables chatbots to leverage knowledge gained from one domain to improve performance in another. By transferring learned representations and models, chatbots can quickly adapt to new tasks and domains, enhancing their versatility and effectiveness.
Here’s an example of implementing a simple reinforcement learning agent for dialogue management:
import numpy as np
import random
class RLDialogueAgent:
def __init__(self, actions):
self.actions = actions
self.q_table = {}
self.alpha = 0.1
self.gamma = 0.9
self.epsilon = 0.1
def get_state_action(self, state, action):
return self.q_table.get((state, action), 0.0)
def choose_action(self, state):
if random.uniform(0, 1) < self.epsilon:
return random.choice(self.actions)
else:
q_values = [self.get_state_action(state, action) for action in self.actions]
max_q = max(q_values)
return self.actions[q_values.index(max_q)]
def update_q_table(self, state, action, reward, next_state):
max_next_q = max([self.get_state_action(next_state, next_action) for next_action in self.actions])
current_q = self.q_table.get((state, action), 0.0)
self.q_table[(state, action)] = current_q + self.alpha * (reward + self.gamma * max_next_q - current_q)
# Example usage
actions = ["greeting", "booking", "weather"]
agent = RLDialogueAgent(actions)
# Simulate interaction
state = "initial"
action = agent.choose_action(state)
reward = 1 # Example reward
next_state = "booking"
agent.update_q_table(state, action, reward, next_state)
# Print Q-table
print(agent.q_table)
Machine learning chatbots have evolved significantly, thanks to advancements in NLP, deep learning, and reinforcement learning. By leveraging these technologies, chatbots can provide intelligent, context-aware, and personalized interactions. Future trends like voice integration, emotion recognition, and autonomous learning will further enhance chatbot capabilities, making them indispensable tools for businesses and users alike. Using tools like Google and Kaggle, developers can access resources and datasets to build and optimize advanced ML chatbots, exploring new frontiers in AI-driven communication.
If you want to read more articles similar to Unveiling the Advanced ML Chatbot: Exploring New Frontiers, you can visit the Applications category.
You Must Read