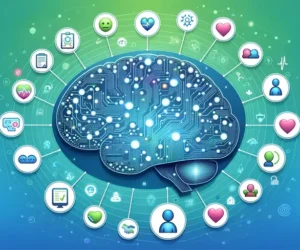
Why Python is the Preferred Language for Machine Learning
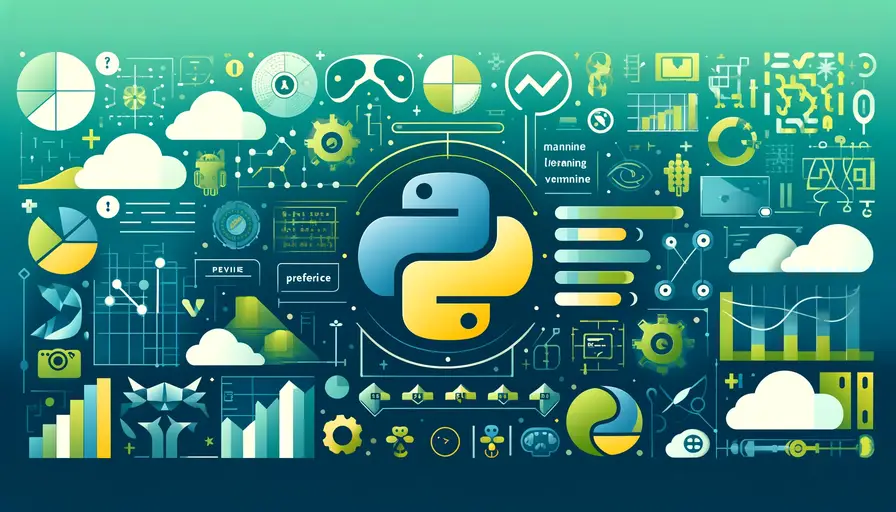
Wide Range of Libraries
Extensive Libraries and Frameworks
Python boasts an extensive range of libraries and frameworks specifically designed for machine learning. Libraries such as Scikit-learn, TensorFlow, and Keras provide robust tools for building and deploying machine learning models. These libraries come with pre-built algorithms and utilities that simplify the development process, making Python a powerful choice for machine learning.
Scikit-learn, for example, offers simple and efficient tools for data mining and data analysis, supporting various machine learning algorithms. TensorFlow, developed by Google, and Keras, an API for neural networks, provide capabilities for deep learning, allowing developers to create complex neural network architectures with ease.
Here’s an example of using Scikit-learn to build a machine learning model:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train the model
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X_train, y_train)
# Predict and evaluate
y_pred = clf.predict(X_test)
print("Accuracy:", accuracy_score(y_test, y_pred))
This code demonstrates how to use Scikit-learn to train and evaluate a machine learning model.
Strategies for Handling Outliers in Machine Learnin RegressionSimple Syntax
Python's Simplicity and Readability
Python's simple and easy-to-understand syntax is one of its greatest strengths. The language is designed to be readable and straightforward, reducing the complexity often associated with programming. This simplicity makes Python accessible to beginners and experienced developers alike, allowing them to focus on problem-solving rather than language intricacies.
The clear and concise syntax of Python enables developers to write less code while accomplishing more, which is particularly beneficial in machine learning. This simplicity also facilitates rapid prototyping and experimentation, essential for testing new ideas and algorithms quickly.
Rapid Prototyping
Python allows for rapid prototyping, a crucial aspect of machine learning development. The ease of writing and testing code means that developers can quickly iterate on their models, experimenting with different approaches and tuning parameters efficiently.
This rapid development cycle is supported by Python’s extensive standard library and third-party packages, which provide ready-to-use solutions for common tasks. As a result, developers can focus on refining their machine learning models without being bogged down by boilerplate code.
Is Machine Learning Difficult to Learn?Here’s an example of Python’s simplicity:
# Calculate the square of numbers in a list
numbers = [1, 2, 3, 4, 5]
squares = [x**2 for x in numbers]
print(squares)
This code snippet demonstrates Python's readability and ease of use.
Active Community
Community Support
Python has a large and active community of developers who contribute to its growth and improvement. This community support means that developers have access to a wealth of resources, including tutorials, forums, and open-source projects. The collaborative nature of the Python community fosters knowledge sharing and problem-solving, making it easier for developers to find solutions and best practices.
The active community also ensures that Python libraries and frameworks are well-maintained and continuously updated. This ongoing development keeps Python at the forefront of technological advancements, ensuring that it remains a relevant and powerful tool for machine learning.
Is Coding Necessary for Machine Learning?Extensive Documentation
Python’s extensive documentation provides detailed guidance on using its libraries and frameworks. This documentation is a valuable resource for developers, offering clear instructions, examples, and best practices for implementing machine learning models.
Official documentation for libraries like TensorFlow and Scikit-learn includes comprehensive guides, tutorials, and API references, helping developers quickly understand and utilize these tools. This wealth of information accelerates learning and development, enabling developers to build and deploy machine learning models more efficiently.
Here’s an example of accessing help in Python:
import numpy as np
# Access help for the numpy array function
help(np.array)
This code snippet shows how to access documentation directly in Python.
Can You Learn Machine Learning Without a Computer Science Background?Versatility
Integration with Other Languages
Python integrates well with other languages and tools, enhancing its versatility. Developers can use Python alongside languages like C++, Java, and R, leveraging the strengths of each language for specific tasks. This interoperability allows for the development of robust and efficient machine learning solutions.
Python’s ability to interface with other languages also facilitates the use of existing codebases and libraries, reducing the need for rewriting code. This seamless integration supports a wide range of applications, from data analysis to web development, making Python a versatile choice for various projects.
Multi-Purpose Use
Python is highly versatile and can be used for various tasks beyond machine learning. It is widely used in web development, data analysis, automation, scientific computing, and more. This versatility makes Python a valuable skill for developers, as it allows them to work on a broad range of projects using a single language.
Python’s extensive library ecosystem supports its use in different domains. Libraries like Django and Flask are popular for web development, while Pandas and NumPy are essential for data analysis. This multi-purpose nature of Python makes it an attractive choice for developers looking to diversify their skill set.
Is Khan Academy a Reliable Resource for Machine Learning Education?Here’s an example of using Python for web development with Flask:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, Flask!"
if __name__ == '__main__':
app.run(debug=True)
This code demonstrates how to create a simple web application using Flask.
Integration
Integration with Tools
Python integrates seamlessly with various tools used in the machine learning workflow. It can work with databases, cloud services, and data processing frameworks like Hadoop and Spark. This flexibility allows developers to build end-to-end machine learning solutions that are scalable and efficient.
The ability to integrate with tools like Jupyter Notebooks enhances the development experience. Jupyter Notebooks provide an interactive environment for writing and executing Python code, making it easy to document and share machine learning experiments. This integration streamlines the development process, enabling faster iteration and collaboration.
Best Programming Language for Machine Learning: R or Python?Language Interoperability
Python’s interoperability with other languages allows developers to combine its ease of use with the performance of languages like C and C++. Through libraries such as Cython and ctypes, Python code can call C/C++ functions directly, improving performance without sacrificing simplicity.
This interoperability is particularly beneficial in machine learning, where computational efficiency is crucial. By leveraging the strengths of multiple languages, developers can create high-performance machine learning models that are both easy to develop and efficient to run.
Here’s an example of integrating Python with C using ctypes:
import ctypes
# Load the shared library
lib = ctypes.CDLL('./mylib.so')
# Call a function from the library
result = lib.my_function(5)
print(result)
This code demonstrates how to call a C function from Python using ctypes.
Documentation
Comprehensive Resources
Python has extensive documentation and resources available for machine learning. These resources include official documentation, tutorials, books, and online courses that cover a wide range of topics, from basic concepts to advanced techniques.
The availability of comprehensive resources makes it easier for developers to learn and master machine learning with Python. These materials provide step-by-step instructions, practical examples, and insights into best practices, helping developers build effective machine learning models.
Learning Support
Python’s learning support extends to its active community, which frequently contributes tutorials, blog posts, and sample projects. This wealth of information is invaluable for both beginners and experienced developers, offering guidance and inspiration for their machine learning projects.
Platforms like edX, Coursera, and Udacity offer specialized courses in machine learning with Python, providing structured learning paths and hands-on experience. These courses often feature projects and assignments that reinforce learning and help developers apply their skills in real-world scenarios.
Here’s an example of accessing Python’s official documentation online:
# Open Python documentation in a web browser
import webbrowser
webbrowser.open('https://docs.python.org/3/')
This code snippet shows how to access Python’s official documentation.
Rapid Prototyping
Simplicity and Readability
Python’s simplicity and readability make it ideal for rapid prototyping. Developers can quickly write and test code, allowing them to experiment with different machine learning models and techniques. This rapid iteration cycle is crucial for refining models and achieving optimal performance.
Python’s clear syntax and extensive library support enable developers to focus on the logic of their machine learning algorithms rather than the intricacies of the language. This focus accelerates development and allows for more efficient problem-solving.
Extensive Community Support
Python’s extensive community support further enhances its suitability for rapid prototyping. The active community continually develops and shares new tools, libraries, and frameworks that simplify and accelerate the development process.
This collaborative environment ensures that developers have access to the latest advancements in machine learning, enabling them to prototype and iterate on their models quickly. The community support also provides solutions to common challenges, helping developers overcome obstacles and improve their models.
Here’s an example of a simple machine learning prototype using Scikit-learn:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train the model
model = LogisticRegression()
model.fit(X_train, y_train)
# Predict and evaluate
y
_pred = model.predict(X_test)
print("Accuracy:", accuracy_score(y_test, y_pred))
This code demonstrates a simple machine learning prototype using Scikit-learn.
Programming Paradigms
Object-Oriented Programming
Python supports object-oriented programming (OOP), allowing developers to create reusable and modular code. OOP principles such as inheritance, encapsulation, and polymorphism enable the development of complex machine learning models in a structured and organized manner.
The use of classes and objects in Python promotes code reuse and maintainability. By encapsulating functionality within classes, developers can create modular components that are easy to manage and extend, making it easier to build and scale machine learning applications.
Functional Programming
Python also supports functional programming, which emphasizes the use of functions and immutable data. Functional programming techniques such as map, filter, and reduce enable developers to write concise and expressive code, facilitating the implementation of complex machine learning algorithms.
The combination of object-oriented and functional programming paradigms provides flexibility in designing machine learning solutions. Developers can choose the most appropriate paradigm for their specific needs, leveraging the strengths of both approaches to create efficient and maintainable code.
Here’s an example of functional programming in Python:
# Using map, filter, and reduce in Python
from functools import reduce
# Sample data
numbers = [1, 2, 3, 4, 5]
# Map: apply a function to all items
squares = list(map(lambda x: x**2, numbers))
# Filter: filter items based on a condition
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
# Reduce: apply a function cumulatively to the items
sum_of_numbers = reduce(lambda x, y: x + y, numbers)
print(squares)
print(even_numbers)
print(sum_of_numbers)
This code demonstrates the use of functional programming techniques in Python.
Data Visualization
Visualization Tools
Python has a rich ecosystem of data visualization tools that are essential for machine learning. Libraries such as Matplotlib, Seaborn, and Plotly provide powerful tools for creating a wide range of visualizations, from simple line plots to interactive dashboards.
These visualization tools help developers explore and understand their data, identify patterns and trends, and communicate their findings effectively. Visualizations are crucial for the data preprocessing stage and for presenting the results of machine learning models to stakeholders.
Importance of Visualization
Data visualization is an integral part of the machine learning workflow. Visualizing data helps in identifying outliers, understanding feature distributions, and uncovering relationships between variables. This insight is critical for feature engineering and model selection.
Effective visualizations also play a vital role in interpreting and explaining machine learning models. Techniques such as feature importance plots, confusion matrices, and ROC curves provide valuable insights into model performance, helping developers make informed decisions and improve their models.
Here’s an example of creating a simple plot using Matplotlib:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [10, 20, 30, 40, 50]
# Create a line plot
plt.plot(x, y, marker='o')
plt.title('Simple Line Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
This code demonstrates how to create a simple line plot using Matplotlib.
Platform Independence
Cross-Platform Compatibility
Python is platform-independent, meaning it can run on various operating systems such as Windows, macOS, and Linux. This cross-platform compatibility ensures that Python code can be executed on different environments without modification, providing flexibility in deployment and development.
The platform independence of Python simplifies the development process, as developers can write code on one operating system and deploy it on another. This feature is particularly beneficial in machine learning, where models may be trained on powerful servers and deployed on different platforms.
Flexibility in Deployment
Python’s flexibility in deployment makes it an ideal choice for machine learning projects. Whether running on local machines, cloud servers, or edge devices, Python provides the tools and libraries needed to deploy machine learning models efficiently.
Cloud platforms such as AWS, Google Cloud, and Azure offer extensive support for Python, providing scalable infrastructure for training and deploying models. This flexibility allows developers to choose the best deployment environment for their specific needs, ensuring optimal performance and cost-effectiveness.
Here’s an example of running Python code on different platforms:
# Sample Python script
print("Hello, Python!")
# This script can be executed on Windows, macOS, and Linux
This simple script demonstrates Python’s cross-platform compatibility.
Python's extensive libraries, simple syntax, active community, and versatility make it the preferred language for machine learning. Its ability to integrate with other languages and tools, comprehensive documentation, and support for rapid prototyping further enhance its appeal. Python’s platform independence and rich ecosystem of data visualization tools ensure that developers can build and deploy robust machine learning models efficiently across various environments.
If you want to read more articles similar to Why Python is the Preferred Language for Machine Learning, you can visit the Education category.
You Must Read