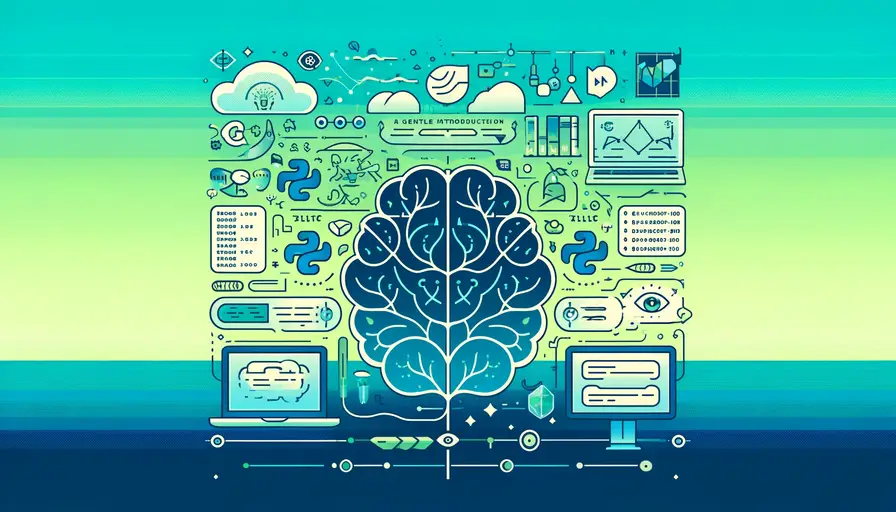
XGBoost: A Powerful ML Model for Classification and Regression

XGBoost (eXtreme Gradient Boosting) has become one of the most popular machine learning algorithms due to its robust performance and flexibility. It is widely used for both classification and regression tasks and has consistently won numerous machine learning competitions. This article delves into the fundamentals of XGBoost, its practical applications, and how to implement it effectively using Python.
Understanding XGBoost
Basics of Gradient Boosting
Gradient boosting is an ensemble learning technique that builds models sequentially. Each new model corrects errors made by the previous models. The primary idea is to combine the predictions of several weak models to form a strong predictive model. Gradient boosting works by minimizing a loss function, typically through gradient descent, which adjusts the model parameters iteratively to reduce the prediction error.
XGBoost extends the gradient boosting framework by optimizing it further for speed and performance. It introduces regularization techniques to prevent overfitting and parallel processing to enhance computational efficiency. These enhancements make XGBoost particularly powerful for large-scale data sets and complex models.
Key Features of XGBoost
XGBoost offers several key features that contribute to its popularity. One of the primary features is regularization, which helps prevent overfitting by adding a penalty to the complexity of the model. This is achieved through L1 (Lasso) and L2 (Ridge) regularization techniques, which control the weights of the model parameters.

Another significant feature is tree pruning. XGBoost uses a technique called Maximum Depth to prune trees, which simplifies the model and prevents overfitting by removing splits that do not provide a significant gain. Additionally, XGBoost includes shrinkage (learning rate) to scale the contribution of each tree, providing a finer control over the training process.
Parallel processing is another key feature of XGBoost. It allows the algorithm to leverage multiple CPU cores during training, significantly speeding up the model-building process. This is particularly beneficial when working with large datasets or complex models, where training time can be a critical factor.
Advantages and Limitations
XGBoost offers several advantages. It is highly efficient, both in terms of speed and memory usage, due to its optimization techniques. XGBoost also provides excellent predictive performance, often outperforming other machine learning algorithms in various competitions and benchmarks. Its flexibility allows it to handle a wide range of data types and problems, including missing values and imbalanced datasets.
However, XGBoost also has some limitations. It can be sensitive to hyperparameter settings, requiring careful tuning to achieve optimal performance. The algorithm's complexity can make it challenging to interpret the model, especially for non-technical stakeholders. Additionally, the computational efficiency of XGBoost may not be as pronounced when dealing with very small datasets, where simpler algorithms could be more appropriate.

Implementing XGBoost for Classification
Preparing the Data
Preparing the data is a crucial step before training an XGBoost model. This involves cleaning the data, handling missing values, encoding categorical variables, and splitting the data into training and testing sets. Ensuring that the data is in the right format and free from errors is essential for building a reliable model.
For this example, let's use the famous Iris dataset available from the UCI Machine Learning Repository. This dataset contains information about three species of iris flowers, including sepal length, sepal width, petal length, and petal width.
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import LabelEncoder
# Load the Iris dataset
url = "https://archive.ics.uci.edu/ml/machine-learning-databases/iris/iris.data"
column_names = ["sepal_length", "sepal_width", "petal_length", "petal_width", "species"]
data = pd.read_csv(url, header=None, names=column_names)
# Encode the target variable
label_encoder = LabelEncoder()
data["species"] = label_encoder.fit_transform(data["species"])
# Split the data into training and testing sets
X = data.drop("species", axis=1)
y = data["species"]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
This code demonstrates how to load the Iris dataset, encode the target variable, and split the data into training and testing sets.
Training the XGBoost Model
After preparing the data, the next step is to train the XGBoost model. This involves creating an instance of the XGBoost classifier, setting the hyperparameters, and fitting the model to the training data. The xgboost
library in Python provides an easy-to-use interface for implementing XGBoost models.

import xgboost as xgb
from sklearn.metrics import accuracy_score
# Create an XGBoost classifier
xgb_clf = xgb.XGBClassifier(objective="multi:softmax", num_class=3, random_state=42)
# Train the model
xgb_clf.fit(X_train, y_train)
# Make predictions
y_pred = xgb_clf.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
This code demonstrates how to create and train an XGBoost classifier on the Iris dataset, make predictions on the test set, and evaluate the model's accuracy.
Evaluating Model Performance
Evaluating the performance of the XGBoost model involves using various metrics to assess how well the model predicts the target variable. Common metrics for classification tasks include accuracy, precision, recall, and F1-score. These metrics provide a comprehensive view of the model's performance, highlighting its strengths and areas for improvement.
Here is an example of generating a classification report and confusion matrix using scikit-learn:
from sklearn.metrics import classification_report, confusion_matrix
# Generate a classification report
report = classification_report(y_test, y_pred, target_names=label_encoder.classes_)
print("Classification Report:\n", report)
# Generate a confusion matrix
conf_matrix = confusion_matrix(y_test, y_pred)
print("Confusion Matrix:\n", conf_matrix)
This code demonstrates how to generate a classification report and confusion matrix, providing detailed insights into the model's performance.

Implementing XGBoost for Regression
Preparing the Data
Preparing the data for a regression task is similar to preparing it for classification. This involves cleaning the data, handling missing values, encoding categorical variables, and splitting the data into training and testing sets. For this example, let's use the California Housing dataset available from scikit-learn. This dataset contains information about housing prices in California.
from sklearn.datasets import fetch_california_housing
# Load the California Housing dataset
housing = fetch_california_housing()
X, y = housing.data, housing.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
This code demonstrates how to load the California Housing dataset and split the data into training and testing sets.
Training the XGBoost Model
Training the XGBoost model for regression involves creating an instance of the XGBoost regressor, setting the hyperparameters, and fitting the model to the training data. The xgboost
library provides an interface for implementing XGBoost regressors.
import xgboost as xgb
from sklearn.metrics import mean_squared_error
# Create an XGBoost regressor
xgb_reg = xgb.XGBRegressor(objective="reg:squarederror", random_state=42)
# Train the model
xgb_reg.fit(X_train, y_train)
# Make predictions
y_pred = xgb_reg.predict(X_test)
# Evaluate the model
mse = mean_squared_error(y_test, y_pred)
print(f"Mean Squared Error: {mse}")
This code demonstrates how to create and train an XGBoost regressor on the California Housing dataset, make predictions on the test set, and evaluate the model's performance using mean squared error (MSE).

Evaluating Model Performance
Evaluating the performance of the XGBoost model for regression involves using various metrics to assess how well the model predicts the target variable. Common metrics for regression tasks include mean squared error (MSE), mean absolute error (MAE), and R-squared (R²). These metrics provide a comprehensive view of the model's performance, highlighting its strengths and areas for improvement.
Here is an example of generating regression metrics using scikit-learn:
from sklearn.metrics import mean_absolute_error, r2_score
# Calculate mean absolute error
mae = mean_absolute_error(y_test, y_pred)
print(f"Mean Absolute Error: {mae}")
# Calculate R-squared
r2 = r2_score(y_test, y_pred)
print(f"R-squared: {r2}")
This code demonstrates how to calculate mean absolute error (MAE) and R-squared (R²), providing detailed insights into the model's performance.
Hyperparameter Tuning in XGBoost
Importance of Hyperparameter Tuning
Hyperparameter tuning is a critical step in optimizing the performance of XGBoost models. Hyperparameters control various aspects of the model, such as the learning rate, the number of trees, and the maximum depth of each tree. Tuning these parameters can significantly improve the model's accuracy and generalization capabilities.

XGBoost provides several hyperparameters that can be tuned to enhance model performance. These include learning_rate
, n_estimators
, max_depth
, min_child_weight
, subsample
, and colsample_bytree
. Each of these hyperparameters plays a crucial role in controlling the complexity and behavior of the model.
Techniques for Hyperparameter Tuning
Several techniques can be used for hyperparameter tuning, including Grid Search, Random Search, and Bayesian Optimization. Grid Search involves specifying a grid of hyperparameter values and evaluating the model performance for each combination. Random Search selects random combinations of hyperparameters from a specified range, providing a more efficient alternative to Grid Search. Bayesian Optimization uses probabilistic models to guide the search for optimal hyperparameters, offering a more advanced and efficient approach.
Here is an example of using Grid Search for hyperparameter tuning with scikit-learn:
from sklearn.model_selection import GridSearchCV
# Define the parameter grid
param_grid = {
'learning_rate': [0.01, 0.1, 0.2],
'n_estimators': [100, 200, 300],
'max_depth': [3, 4, 5],
'min_child_weight': [1, 3, 5],
'subsample': [0.6, 0.8, 1.0],
'colsample_bytree': [0.6, 0.8, 1.0]
}
# Create the XGBoost regressor
xgb_reg = xgb.XGBRegressor(objective="reg:squarederror", random_state=42)
# Create the Grid Search object
grid_search = GridSearchCV(estimator=xgb_reg, param_grid=param_grid, cv=3, scoring='neg_mean_squared_error', verbose=1)
# Fit the Grid Search to the data
grid_search.fit(X_train, y_train)
# Print the best parameters and score
print(f"Best Parameters: {grid_search.best_params_}")
print(f"Best Score: {grid_search.best_score_}")
This code demonstrates how to use Grid Search for hyperparameter tuning, identifying the optimal hyperparameters for the XGBoost model.
Implementing the Tuned Model
After identifying the optimal hyperparameters, the next step is to implement the tuned model and evaluate its performance. This involves creating an instance of the XGBoost model with the best hyperparameters and fitting it to the training data.
# Create the XGBoost regressor with the best parameters
best_params = grid_search.best_params_
xgb_reg_tuned = xgb.XGBRegressor(
objective="reg:squarederror",
learning_rate=best_params['learning_rate'],
n_estimators=best_params['n_estimators'],
max_depth=best_params['max_depth'],
min_child_weight=best_params['min_child_weight'],
subsample=best_params['subsample'],
colsample_bytree=best_params['colsample_bytree'],
random_state=42
)
# Train the tuned model
xgb_reg_tuned.fit(X_train, y_train)
# Make predictions
y_pred_tuned = xgb_reg_tuned.predict(X_test)
# Evaluate the tuned model
mse_tuned = mean_squared_error(y_test, y_pred_tuned)
print(f"Tuned Mean Squared Error: {mse_tuned}")
# Calculate mean absolute error
mae_tuned = mean_absolute_error(y_test, y_pred_tuned)
print(f"Tuned Mean Absolute Error: {mae_tuned}")
# Calculate R-squared
r2_tuned = r2_score(y_test, y_pred_tuned)
print(f"Tuned R-squared: {r2_tuned}")
This code demonstrates how to implement the tuned XGBoost model and evaluate its performance, highlighting the improvements achieved through hyperparameter tuning.
Practical Applications of XGBoost
Finance and Economics
XGBoost is widely used in finance and economics for various predictive modeling tasks. It can be applied to forecast stock prices, predict credit risk, and analyze economic indicators. The algorithm's robustness and accuracy make it suitable for handling the complex and volatile nature of financial data.
For example, XGBoost can be used to predict stock prices by analyzing historical price data, trading volumes, and other financial indicators. The model can identify patterns and trends, providing valuable insights for investors and traders.
Here is an example of using XGBoost for stock price prediction:
import pandas as pd
import yfinance as yf
# Download historical stock price data
data = yf.download('AAPL', start='2020-01-01', end='2021-01-01')
# Prepare the data
data['Return'] = data['Close'].pct_change()
data = data.dropna()
X = data[['Open', 'High', 'Low', 'Volume']]
y = data['Return']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the XGBoost regressor
xgb_reg = xgb.XGBRegressor(objective="reg:squarederror", random_state=42)
xgb_reg.fit(X_train, y_train)
# Make predictions and evaluate the model
y_pred = xgb_reg.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print(f"Mean Squared Error: {mse}")
This code demonstrates how to use XGBoost for stock price prediction, highlighting its application in finance.
Healthcare and Medicine
In healthcare and medicine, XGBoost is used for predicting patient outcomes, diagnosing diseases, and personalizing treatment plans. Its ability to handle large and complex datasets makes it suitable for analyzing medical records, genetic data, and imaging data.
For example, XGBoost can be used to predict patient outcomes based on electronic health records (EHRs). By analyzing patient demographics, medical history, and lab results, the model can identify factors that influence health outcomes and suggest personalized treatment plans.
Here is an example of using XGBoost for predicting patient outcomes:
import pandas as pd
# Load the patient data
data = pd.read_csv('path/to/patient_data.csv')
# Prepare the data
X = data.drop(columns=['outcome'])
y = data['outcome']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the XGBoost classifier
xgb_clf = xgb.XGBClassifier(objective="binary:logistic", random_state=42)
xgb_clf.fit(X_train, y_train)
# Make predictions and evaluate the model
y_pred = xgb_clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
This code demonstrates how to use XGBoost for predicting patient outcomes, showcasing its application in healthcare.
Marketing and Customer Analytics
XGBoost is also widely used in marketing and customer analytics for predicting customer behavior, segmenting markets, and optimizing marketing strategies. By analyzing customer data, such as purchase history, demographics, and online behavior, XGBoost can help businesses understand their customers better and make data-driven decisions.
For example, XGBoost can be used to predict customer churn by analyzing customer interactions, transaction history, and satisfaction scores. The model can identify at-risk customers, allowing businesses to implement targeted retention strategies.
Here is an example of using XGBoost for predicting customer churn:
import pandas as pd
# Load the customer data
data = pd.read_csv('path/to/customer_data.csv')
# Prepare the data
X = data.drop(columns=['churn'])
y = data['churn']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the XGBoost classifier
xgb_clf = xgb.XGBClassifier(objective="binary:logistic", random_state=42)
xgb_clf.fit(X_train, y_train)
# Make predictions and evaluate the model
y_pred = xgb_clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
This code demonstrates how to use XGBoost for predicting customer churn, highlighting its application in marketing and customer analytics.
By understanding the fundamentals of XGBoost, implementing it for various tasks, and tuning its hyperparameters, practitioners can leverage its powerful capabilities to build accurate and efficient models for a wide range of applications. Whether in finance, healthcare, or marketing, XGBoost offers robust solutions for tackling complex predictive modeling challenges.
If you want to read more articles similar to XGBoost: A Powerful ML Model for Classification and Regression, you can visit the Algorithms category.
You Must Read