
Python: Reading and Manipulating CSV Files for Machine Learning
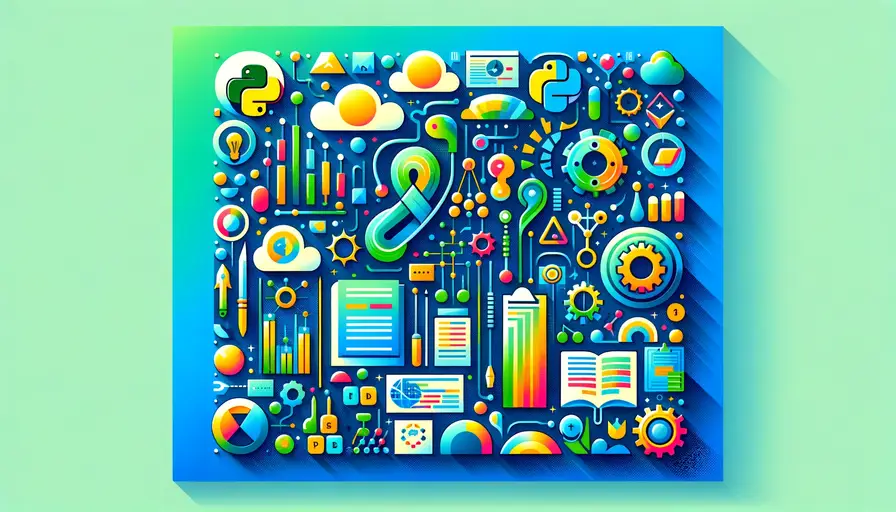
Data manipulation is a critical aspect of machine learning, and CSV files are one of the most common formats for storing datasets. This guide provides a comprehensive approach to reading and manipulating CSV files in Python for machine learning purposes. By the end, you'll have a thorough understanding of how to handle CSV files, perform data preprocessing, and prepare datasets for machine learning models.
Content
Reading CSV Files
Using the Pandas Library
The Pandas library is a powerful tool for data manipulation and analysis in Python. It provides easy-to-use data structures and data analysis tools. Reading CSV files with Pandas is straightforward and efficient, making it an essential skill for data scientists and machine learning practitioners.
Pandas can read CSV files into a DataFrame, a two-dimensional data structure similar to a table. This DataFrame provides various methods for accessing and manipulating data, enabling efficient data preprocessing. You can read a CSV file using the read_csv
function, which supports various options for customizing the reading process.
Here’s an example of reading a CSV file using Pandas:
Data Pipeline and ML Implementation Best Practices in Pythonimport pandas as pd
# Reading the CSV file into a DataFrame
df = pd.read_csv('sample_data.csv')
# Displaying the first few rows of the DataFrame
print(df.head())
Handling Missing Values
Missing values are a common issue in datasets and can significantly affect the performance of machine learning models. Pandas provides several methods for handling missing values, including dropping rows or columns with missing values, filling missing values with a specific value, or using more sophisticated techniques like interpolation.
Handling missing values appropriately is crucial for ensuring the quality and reliability of the dataset. Depending on the context and the amount of missing data, different strategies may be employed.
Here’s an example of handling missing values using Pandas:
# Dropping rows with missing values
df_cleaned = df.dropna()
# Filling missing values with the mean of the column
df_filled = df.fillna(df.mean())
# Displaying the cleaned DataFrame
print(df_cleaned.head())
print(df_filled.head())
Reading Large CSV Files
Working with large CSV files can be challenging due to memory constraints. Pandas provides several techniques for efficiently reading and processing large CSV files, such as reading the file in chunks, specifying data types, and using memory mapping.
Saving and Loading Machine Learning Models in RReading large CSV files in chunks allows you to process the data in smaller, manageable portions. This can be particularly useful when the dataset is too large to fit into memory. Specifying data types can reduce memory usage by using appropriate data types for each column.
Here’s an example of reading a large CSV file in chunks using Pandas:
# Reading the CSV file in chunks
chunk_size = 10000
chunks = pd.read_csv('large_data.csv', chunksize=chunk_size)
# Processing each chunk
for chunk in chunks:
# Performing operations on the chunk
print(chunk.head())
Manipulating CSV Files
Filtering Data
Filtering data is a fundamental operation in data preprocessing. It involves selecting specific rows or columns based on certain conditions. Pandas provides powerful methods for filtering data, enabling you to easily extract relevant subsets of the dataset.
Filtering can be based on various criteria, such as column values, ranges, or conditions. This allows you to focus on specific parts of the dataset that are relevant to your analysis or machine learning tasks.
A Comprehensive Guide on Deploying Machine Learning Models with FlaskHere’s an example of filtering data using Pandas:
# Filtering rows based on a condition
filtered_df = df[df['column_name'] > 50]
# Selecting specific columns
selected_columns_df = df[['column1', 'column2']]
# Displaying the filtered DataFrame
print(filtered_df.head())
print(selected_columns_df.head())
Sorting Data
Sorting data is another essential operation for organizing and analyzing datasets. Pandas provides methods for sorting DataFrames by one or more columns, either in ascending or descending order. Sorting can help you understand the distribution of data and identify patterns.
Sorting can be particularly useful when you need to rank data, identify outliers, or prepare the dataset for further analysis. Pandas makes it easy to sort data based on different criteria, enabling efficient data manipulation.
Here’s an example of sorting data using Pandas:
Exploring the Feasibility of Machine Learning on AMD GPUs# Sorting the DataFrame by a specific column in ascending order
sorted_df = df.sort_values(by='column_name', ascending=True)
# Sorting the DataFrame by multiple columns
sorted_df_multi = df.sort_values(by=['column1', 'column2'], ascending=[True, False])
# Displaying the sorted DataFrame
print(sorted_df.head())
print(sorted_df_multi.head())
Grouping and Aggregating Data
Grouping and aggregating data are powerful techniques for summarizing and analyzing datasets. Pandas provides the groupby
method, which allows you to group data by one or more columns and apply aggregate functions such as sum, mean, count, and more.
Grouping data enables you to analyze the dataset at different levels of granularity, providing insights into the relationships between variables. Aggregation functions can then be applied to these groups to summarize the data.
Here’s an example of grouping and aggregating data using Pandas:
# Grouping the DataFrame by a specific column and calculating the mean
grouped_df = df.groupby('group_column').mean()
# Grouping by multiple columns and calculating the sum
grouped_df_multi = df.groupby(['column1', 'column2']).sum()
# Displaying the grouped DataFrame
print(grouped_df.head())
print(grouped_df_multi.head())
Data Cleaning and Transformation
Removing Duplicates
Duplicate rows in a dataset can lead to biased or incorrect analysis. Pandas provides methods for identifying and removing duplicate rows based on one or more columns. Removing duplicates is an important data cleaning step to ensure the integrity of the dataset.
The Best Tools for Optimizing Airflow in Machine Learning PipelinesIdentifying duplicates involves checking for rows that have identical values in specific columns. Once identified, these duplicates can be removed to create a clean and reliable dataset.
Here’s an example of removing duplicates using Pandas:
# Identifying and removing duplicate rows
df_no_duplicates = df.drop_duplicates()
# Removing duplicates based on specific columns
df_no_duplicates_columns = df.drop_duplicates(subset=['column1', 'column2'])
# Displaying the DataFrame without duplicates
print(df_no_duplicates.head())
print(df_no_duplicates_columns.head())
Data Type Conversion
Converting data types is a common task in data preprocessing. Pandas provides methods for converting the data types of columns to ensure consistency and optimize memory usage. Correct data types are essential for accurate analysis and efficient computation.
Data type conversion may involve changing numerical columns to categorical, converting strings to datetime objects, or optimizing numerical columns to appropriate integer or float types. These conversions help in performing accurate computations and analyses.
Elasticsearch: No Machine Learning Anomaly Detection API YetHere’s an example of data type conversion using Pandas:
# Converting a column to datetime
df['date_column'] = pd.to_datetime(df['date_column'])
# Converting a column to a categorical type
df['category_column'] = df['category_column'].astype('category')
# Converting a column to an integer type
df['integer_column'] = df['integer_column'].astype('int')
# Displaying the DataFrame with converted data types
print(df.dtypes)
Feature Engineering
Feature engineering involves creating new features from existing ones to improve the performance of machine learning models. This can include generating interaction features, creating polynomial features, or extracting meaningful information from datetime columns.
Feature engineering can enhance the predictive power of machine learning models by providing additional relevant information. It is a critical step in the data preprocessing pipeline, enabling more accurate and robust models.
Here’s an example of feature engineering using Pandas:
# Creating interaction features
df['interaction_feature'] = df['feature1'] * df['feature2']
# Creating polynomial features
df['feature_squared'] = df['feature1'] ** 2
# Extracting information from datetime columns
df['year'] = df['date_column'].dt.year
df['month'] = df['date_column'].dt.month
df['day'] = df['date_column'].dt.day
# Displaying the DataFrame with new features
print(df.head())
Preparing Data for Machine Learning
Splitting the Dataset
Splitting the dataset into training and testing sets is a crucial step in preparing data for machine learning. This allows you to evaluate the performance of the model on unseen data. Scikit-learn provides a convenient method for splitting datasets into training and testing sets.
The training set is used to train the machine learning model, while the testing set is used to evaluate its performance. It is important to ensure that the split is representative of the overall dataset to avoid biased evaluation.
Here’s an example of splitting the dataset using Scikit-learn:
from sklearn.model_selection import train_test_split
# Splitting the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(df[['feature1', 'feature2']], df['target'], test_size=0.2, random_state=42)
# Displaying the shapes of the training and testing sets
print(X_train.shape, X_test.shape)
print(y_train.shape, y_test.shape)
Scaling Features
Feature scaling is essential for many machine learning algorithms that are sensitive to the scale of the input features. Scikit-learn provides various methods for scaling features, such as standardization and normalization. Scaling ensures that all features contribute equally to the model.
Standardization involves rescaling the features to have a mean of zero and a standard deviation of one. Normalization rescales the features to a fixed range, typically [0, 1]. Both techniques help improve the performance and convergence of machine learning models.
Here’s an example of scaling features using Scikit-learn:
from sklearn.preprocessing import StandardScaler, MinMaxScaler
# Standardizing the features
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
# Normalizing the features
normalizer = MinMaxScaler()
X_train_normalized = normalizer.fit_transform(X_train)
X_test_normalized = normalizer.transform(X_test)
# Displaying the scaled features
print(X_train_scaled[:5])
print(X_train_normalized[:5])
Encoding Categorical Variables
Encoding categorical variables is necessary for using them in machine learning models. Scikit-learn provides methods for encoding categorical variables, such as one-hot encoding and label encoding. These encodings convert categorical values into numerical representations that can be used by machine learning algorithms.
One-hot encoding creates binary columns for each category, while label encoding assigns a unique integer to each category. The choice of encoding depends on the specific machine learning algorithm and the nature of the categorical variable.
Here’s an example of encoding categorical variables using Scikit-learn:
from sklearn.preprocessing import OneHotEncoder, LabelEncoder
# One-hot encoding categorical variables
onehot_encoder = OneHotEncoder(sparse=False)
X_train_onehot = onehot_encoder.fit_transform(X_train[['category_column']])
X_test_onehot = onehot_encoder.transform(X_test[['category_column']])
# Label encoding categorical variables
label_encoder = LabelEncoder()
y_train_encoded = label_encoder.fit_transform(y_train)
y_test_encoded = label_encoder.transform(y_test)
# Displaying the encoded variables
print(X_train_onehot[:5])
print(y_train_encoded[:5])
Advanced Data Manipulation Techniques
Merging and Joining DataFrames
Merging and joining DataFrames are essential techniques for combining datasets based on common keys or columns. Pandas provides various methods for merging and joining DataFrames, such as merge
, join
, and concat
. These methods enable you to combine data from different sources into a single DataFrame.
Merging is typically used to combine DataFrames based on common columns, similar to SQL joins. Joining is used to combine DataFrames based on their index. Concatenation is used to append DataFrames either vertically or horizontally.
Here’s an example of merging and joining DataFrames using Pandas:
# Merging DataFrames based on a common column
merged_df = pd.merge(df1, df2, on='key_column')
# Joining DataFrames based on their index
joined_df = df1.join(df2, lsuffix='_left', rsuffix='_right')
# Concatenating DataFrames vertically
concatenated_df = pd.concat([df1, df2], axis=0)
# Displaying the combined DataFrame
print(merged_df.head())
print(joined_df.head())
print(concatenated_df.head())
Pivoting and Melting Data
Pivoting and melting are techniques for reshaping DataFrames to facilitate data analysis. Pivoting converts long-format data to wide-format by creating a new table where one column's unique values become the new columns. Melting is the reverse process, converting wide-format data to long-format.
These techniques are useful for transforming data into a more suitable format for analysis or visualization. Pivoting can help aggregate data and summarize information, while melting can help normalize data for machine learning models.
Here’s an example of pivoting and melting data using Pandas:
# Pivoting the DataFrame to wide format
pivoted_df = df.pivot(index='index_column', columns='pivot_column', values='value_column')
# Melting the DataFrame to long format
melted_df = pd.melt(df, id_vars=['id_column'], value_vars=['value1', 'value2'])
# Displaying the reshaped DataFrame
print(pivoted_df.head())
print(melted_df.head())
Applying Custom Functions
Applying custom functions to DataFrames enables you to perform complex transformations and analyses. Pandas provides the apply
method, which allows you to apply a function to each element, row, or column of a DataFrame. This method is highly flexible and can be used for various data manipulation tasks.
Custom functions can be used to create new features, clean data, or perform calculations. The apply
method can be applied to entire DataFrames, specific columns, or rows, providing a powerful tool for data preprocessing.
Here’s an example of applying custom functions using Pandas:
# Defining a custom function to apply to a column
def custom_function(x):
return x * 2
# Applying the custom function to a specific column
df['new_column'] = df['existing_column'].apply(custom_function)
# Applying a custom function to each row
def row_function(row):
return row['column1'] + row['column2']
df['row_sum'] = df.apply(row_function, axis=1)
# Displaying the DataFrame with the applied functions
print(df.head())
Reading and manipulating CSV files are fundamental skills for machine learning practitioners. By leveraging powerful libraries like Pandas and Scikit-learn, you can efficiently handle data preprocessing tasks, ensuring that your datasets are clean, well-organized, and ready for machine learning models. From reading and cleaning data to performing advanced manipulations, this guide provides a comprehensive approach to working with CSV files in Python.
If you want to read more articles similar to Python: Reading and Manipulating CSV Files for Machine Learning, you can visit the Tools category.
You Must Read