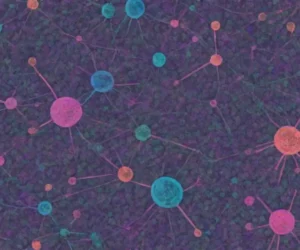
Building Your First ML.NET Pipeline: A Step-by-Step Guide
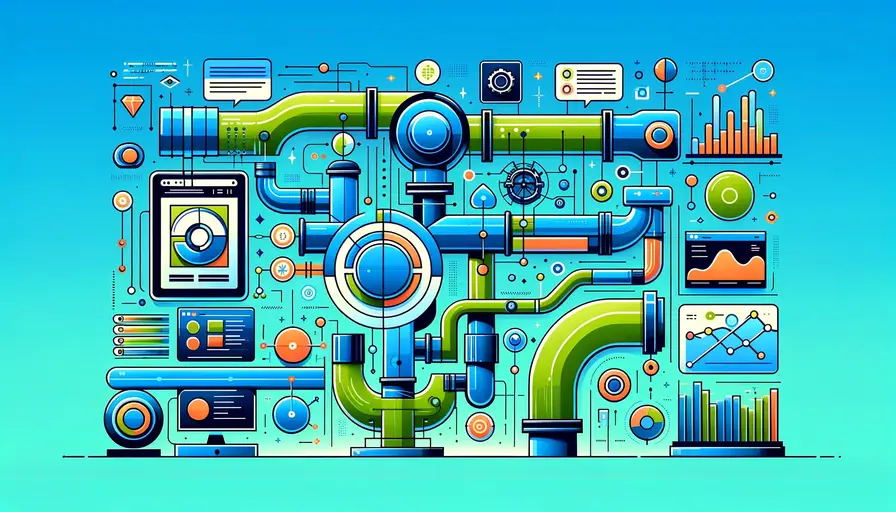
- Understand the Basics of ML.NET and Its Purpose
- Install the Necessary Software and Dependencies
- Collect and Prepare Your Data for Training
- Choose an Appropriate ML Algorithm for Your Problem
- Train Your ML Model Using the Training Data
- Evaluate the Performance of Your Model Using the Testing Data
- Fine-Tune Your Model for Better Accuracy
- Deploy Your ML Model for Production Use
Understand the Basics of ML.NET and Its Purpose
To effectively build an ML.NET pipeline, it's essential to understand what ML.NET is and why it is used. ML.NET is a cross-platform, open-source machine learning framework designed for .NET developers. It allows you to build custom machine learning models using C# or F# without needing extensive knowledge of machine learning or statistics.
Install ML.NET
Step 1: Start by installing ML.NET. Ensure you have .NET Core installed on your system. You can install ML.NET via NuGet Package Manager in Visual Studio or using the .NET CLI.
Gather and Prepare Your Data
Step 2: Collect and prepare your data. This step involves cleaning the data, handling missing values, and transforming it into a format suitable for machine learning.
Define Your ML.NET Pipeline
Step 3: Define your ML.NET pipeline. This involves setting up data loaders, transformers, and specifying the learning algorithm. The pipeline acts as a workflow for data processing and model training.
Machine Learning's Impact on Advanced AI in Medical DevicesTrain and Evaluate Your Model
Step 4: Train your model using the prepared data. After training, evaluate the model's performance using metrics like accuracy, precision, and recall.
Deploy and Use Your Model
Step 5: Deploy the trained model for use in production environments. This may involve integrating it into applications and setting up APIs for inference.
Example of ML.NET Pipeline in C
Here's an example of defining and training a simple ML.NET pipeline in C#:
using System;
using Microsoft.ML;
using Microsoft.ML.Data;
class Program
{
static void Main()
{
var context = new MLContext();
// Load data
var data = context.Data.LoadFromTextFile<ModelInput>("data.csv", hasHeader: true, separatorChar: ',');
// Define pipeline
var pipeline = context.Transforms.Categorical.OneHotEncoding("Category")
.Append(context.Transforms.Concatenate("Features", "Category", "NumericFeature"))
.Append(context.Transforms.NormalizeMinMax("Features"))
.Append(context.Regression.Trainers.Sdca());
// Train model
var model = pipeline.Fit(data);
// Evaluate model
var predictions = model.Transform(data);
var metrics = context.Regression.Evaluate(predictions);
Console.WriteLine($"R^2: {metrics.RSquared}");
}
}
public class ModelInput
{
public string Category { get; set; }
public float NumericFeature { get; set; }
}
Install the Necessary Software and Dependencies
Before you can start building your ML.NET pipeline, you need to install the necessary software and dependencies, such as .NET Core and ML.NET NuGet packages.
Transformative Power of AI and Machine Learning in AMLInstall .NET Core
Ensure that .NET Core is installed on your development machine. You can download it from the official .NET website.
Install ML.NET NuGet Packages
Install ML.NET via NuGet Package Manager in Visual Studio or by using the .NET CLI. This will add the necessary libraries and dependencies to your project.
Example of Installing ML.NET Packages
Using the .NET CLI, you can install ML.NET packages with the following command:
dotnet add package Microsoft.ML
dotnet add package Microsoft.ML.Data
dotnet add package Microsoft.ML.Trainers
Collect and Prepare Your Data for Training
Collecting and preparing data is a crucial step in building an effective machine learning model.
Reinforcement Learning and NLP Integration: Promising for NLPCollecting Data
Gather relevant data from various sources. Ensure the data is comprehensive and representative of the problem you're trying to solve.
Cleaning and Preprocessing Data
Clean the data by handling missing values, removing duplicates, and correcting errors. Preprocessing may also involve normalization, scaling, and encoding categorical variables.
Splitting Data into Features and Labels
Separate your data into features (input variables) and labels (target variable). This distinction is necessary for training supervised learning models.
Example of Data Preprocessing in C
Here's an example of loading and preprocessing data in C# using ML.NET:
Supercharging E-Commerce Strategies with Machine Learningvar context = new MLContext();
// Load data
var data = context.Data.LoadFromTextFile<ModelInput>("data.csv", hasHeader: true, separatorChar: ',');
// Data preprocessing pipeline
var pipeline = context.Transforms.Categorical.OneHotEncoding("Category")
.Append(context.Transforms.Concatenate("Features", "Category", "NumericFeature"))
.Append(context.Transforms.NormalizeMinMax("Features"));
Choose an Appropriate ML Algorithm for Your Problem
Selecting the right machine learning algorithm is critical for the success of your model.
Understand Your Problem
Identify whether your problem is a classification, regression, clustering, or recommendation task. This will guide you in selecting suitable algorithms.
Explore Available ML Algorithms
ML.NET offers a wide range of algorithms, including decision trees, regression algorithms, and neural networks. Explore these options based on your problem type.
Evaluate Algorithm Performance
Test different algorithms to determine which one performs best on your data. Use performance metrics to guide your evaluation.
Leveraging Machine Learning: Unlocking the Benefits for DevelopersExample of Algorithm Selection
Here's an example of using a regression algorithm in C#:
var pipeline = context.Transforms.Categorical.OneHotEncoding("Category")
.Append(context.Transforms.Concatenate("Features", "Category", "NumericFeature"))
.Append(context.Transforms.NormalizeMinMax("Features"))
.Append(context.Regression.Trainers.Sdca());
Train Your ML Model Using the Training Data
Training your ML model involves feeding it the training data and allowing it to learn patterns.
Model Training
Use the training data to fit the model. This process involves optimizing the model's parameters to minimize error on the training set.
Example of Model Training in C
Here's an example of training an ML model using C#:
Top Techniques for Machine Learning-based Size Recognitionvar pipeline = context.Transforms.Categorical.OneHotEncoding("Category")
.Append(context.Transforms.Concatenate("Features", "Category", "NumericFeature"))
.Append(context.Transforms.NormalizeMinMax("Features"))
.Append(context.Regression.Trainers.Sdca());
var model = pipeline.Fit(trainingData);
Evaluate the Performance of Your Model Using the Testing Data
Evaluating your model's performance ensures it generalizes well to new, unseen data.
Accuracy
Measure the accuracy of your model, especially for classification tasks. Accuracy is the ratio of correctly predicted instances to the total instances.
Precision
For classification tasks, precision measures the proportion of true positive predictions among all positive predictions.
Example of Model Evaluation in C
Here's an example of evaluating a model's performance in C#:
var predictions = model.Transform(testingData);
var metrics = context.Regression.Evaluate(predictions);
Console.WriteLine($"R^2: {metrics.RSquared}");
Console.WriteLine($"Mean Absolute Error: {metrics.MeanAbsoluteError}");
Fine-Tune Your Model for Better Accuracy
Fine-tuning your model involves making adjustments to improve its performance.
Evaluate Model Performance
Regularly evaluate your model's performance to identify areas for improvement.
Adjust Hyperparameters
Tuning hyperparameters can significantly impact model performance. Experiment with different settings to find the optimal configuration.
Try Different Algorithms
If necessary, experiment with different algorithms to see if they offer better performance.
Example of Hyperparameter Tuning in C
Here's an example of adjusting hyperparameters in C#:
var pipeline = context.Transforms.Categorical.OneHotEncoding("Category")
.Append(context.Transforms.Concatenate("Features", "Category", "NumericFeature"))
.Append(context.Transforms.NormalizeMinMax("Features"))
.Append(context.Regression.Trainers.Sdca(new SdcaRegressionTrainer.Options
{
L2Regularization = 0.1f,
L1Regularization = 0.01f
}));
var model = pipeline.Fit(trainingData);
Deploy Your ML Model for Production Use
Deploying your ML model involves making it available for use in production environments.
Choosing a Deployment Approach
Decide on the deployment method, such as integrating the model into an application or setting up a web API for inference.
Considerations for Deployment
Consider factors like scalability, latency, and security when deploying your model.
Example of Model Deployment in C
Here's an example of setting up a web API for model inference in C#:
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.ML;
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddSingleton<PredictionEnginePool<ModelInput, ModelOutput>>();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
}
public class ModelInput
{
public string Category { get; set; }
public float NumericFeature { get; set; }
}
public class ModelOutput
{
public float Score { get; set; }
}
[ApiController]
[Route("api/[controller]")]
public class PredictionController : ControllerBase
{
private readonly PredictionEnginePool<ModelInput, ModelOutput> _predictionEnginePool;
public PredictionController(PredictionEnginePool<ModelInput, ModelOutput> predictionEnginePool)
{
_predictionEnginePool = predictionEnginePool;
}
[HttpPost]
public ActionResult<ModelOutput> Predict(ModelInput input)
{
var prediction = _predictionEnginePool.Predict(input);
return Ok(prediction);
}
}
Building your first ML.NET pipeline involves understanding ML.NET, collecting and preparing data, selecting the right algorithm, defining the model structure, training, evaluating, fine-tuning, and deploying the model. Each step requires careful consideration to ensure the model's accuracy and effectiveness. By following this step-by-step guide, you can create robust machine learning solutions using ML.NET, enhancing your applications with predictive capabilities.
If you want to read more articles similar to Building Your First ML.NET Pipeline: A Step-by-Step Guide, you can visit the Applications category.
You Must Read