
Flask: Best Practices for Deploying ML Models
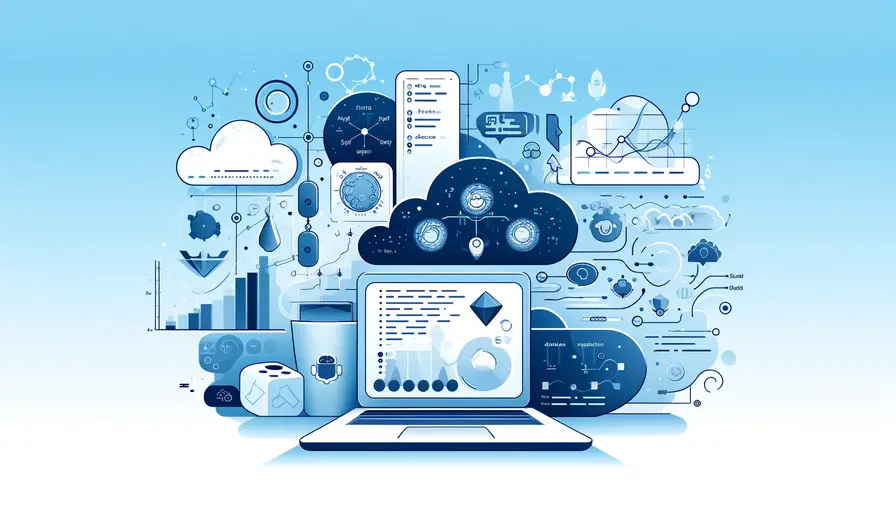
Deploying machine learning (ML) models is a crucial step in transforming them from experimental projects into practical applications that provide real value. Flask, a lightweight web framework for Python, is a popular choice for deploying ML models due to its simplicity and flexibility. This article explores best practices for deploying ML models using Flask, ensuring robust and efficient production environments.
Flask for ML Deployment
Why Use Flask for ML Deployment?
Flask is a micro web framework written in Python that is known for its simplicity and ease of use. It provides the essential tools to build web applications and APIs, making it an excellent choice for deploying machine learning models. Flask’s minimalist design allows developers to create custom deployments without unnecessary overhead, which is particularly useful for lightweight ML applications.
Flask’s flexibility also means that it can be easily integrated with other tools and services, such as Docker for containerization, or cloud platforms like AWS and Google Cloud for scalability. This adaptability makes Flask a versatile choice for both small-scale and enterprise-level ML deployments.
Additionally, Flask’s active community and extensive documentation provide ample resources for troubleshooting and improving deployments. This support network can be invaluable for addressing challenges and ensuring that deployments follow best practices.
SQL Server Machine Learning Services: A Step-by-Step GuidePreparing Your Environment
Before deploying an ML model with Flask, it is essential to prepare your development environment. This includes setting up Python, Flask, and any necessary libraries or dependencies. Using a virtual environment is recommended to manage dependencies and avoid conflicts with other projects.
To set up a virtual environment, you can use venv
or virtualenv
. Once the environment is created, you can install Flask and other dependencies using pip
.
Example of setting up a virtual environment and installing Flask:
# Create a virtual environment
python3 -m venv myenv
# Activate the virtual environment
source myenv/bin/activate # On Windows, use `myenv\Scripts\activate`
# Install Flask
pip install Flask
Structuring Your Flask Application
A well-structured Flask application enhances maintainability and scalability. Organizing your code into separate modules for routes, models, and utilities can help keep the application clean and manageable. This modular approach also facilitates testing and debugging.
Efficient Model Governance with Amazon SageMakerA typical Flask project structure might look like this:
my_flask_app/
├── app/
│ ├── __init__.py
│ ├── routes.py
│ ├── models.py
│ ├── utils.py
│ ├── static/
│ └── templates/
├── tests/
│ ├── __init__.py
│ ├── test_routes.py
│ └── test_models.py
├── venv/
├── config.py
├── run.py
└── requirements.txt
In this structure, routes.py
handles the application routes, models.py
contains the ML models, and utils.py
includes helper functions. The static
and templates
directories store static files and HTML templates, respectively.
Building and Serving ML Models
Loading and Preprocessing Data
The first step in deploying an ML model is to load and preprocess the data. This process involves reading data from various sources, cleaning it, and transforming it into a format suitable for model consumption. Preprocessing steps might include normalization, encoding categorical variables, and feature extraction.
In a Flask application, it is a good practice to separate data loading and preprocessing logic into reusable functions or modules. This modularity allows for easier updates and testing of data processing pipelines.
Pre-configured VM Image: Ideal for Machine LearningExample of a data preprocessing function in utils.py
:
import pandas as pd
from sklearn.preprocessing import StandardScaler
def load_and_preprocess_data(file_path):
# Load data
data = pd.read_csv(file_path)
# Perform data preprocessing
features = data.drop('target', axis=1)
target = data['target']
# Normalize features
scaler = StandardScaler()
features = scaler.fit_transform(features)
return features, target
Training and Saving Models
Training an ML model involves selecting an appropriate algorithm, fitting the model to the training data, and evaluating its performance. Once trained, the model should be saved to disk so it can be loaded and used for predictions in the Flask application.
Using libraries such as scikit-learn, TensorFlow, or PyTorch can simplify the process of training and saving models. These libraries provide built-in functions for model serialization, allowing models to be saved as files and loaded later for inference.
Example of training and saving a model in models.py
:
import joblib
from sklearn.ensemble import RandomForestClassifier
from utils import load_and_preprocess_data
def train_model(data_path):
# Load and preprocess data
X, y = load_and_preprocess_data(data_path)
# Train model
model = RandomForestClassifier(n_estimators=100)
model.fit(X, y)
# Save model to disk
joblib.dump(model, 'model.joblib')
if __name__ == '__main__':
train_model('data/train.csv')
Serving Models with Flask
Serving ML models with Flask involves creating routes that handle requests, load the saved model, and return predictions. Flask’s route decorators make it easy to define endpoints that process incoming data and respond with the model’s output.
It is essential to ensure that the model is loaded only once and reused for subsequent requests to optimize performance. This can be achieved by loading the model when the Flask application starts.
Example of serving a model with Flask in routes.py
:
from flask import Flask, request, jsonify
import joblib
app = Flask(__name__)
# Load the model
model = joblib.load('model.joblib')
@app.route('/predict', methods=['POST'])
def predict():
# Get data from request
data = request.json
features = data['features']
# Make prediction
prediction = model.predict([features])
# Return prediction as JSON
return jsonify({'prediction': prediction.tolist()})
if __name__ == '__main__':
app.run(debug=True)
Enhancing Performance and Scalability
Using WSGI Servers
Flask’s built-in development server is not suitable for production use. For production deployments, it is recommended to use a WSGI (Web Server Gateway Interface) server such as Gunicorn or uWSGI. These servers provide better performance, scalability, and reliability.
Popular R Package for Supervised Learning Tasks: CaretGunicorn is a popular choice for deploying Flask applications due to its simplicity and ease of use. It can handle multiple worker processes, which improves the handling of concurrent requests.
Example of running a Flask app with Gunicorn:
# Install Gunicorn
pip install gunicorn
# Run the Flask app with Gunicorn
gunicorn -w 4 -b 0.0.0.0:8000 app:app
Containerizing with Docker
Docker is a powerful tool for containerizing applications, providing a consistent environment for development, testing, and production. By packaging a Flask application and its dependencies into a Docker container, you can ensure that it runs reliably across different environments.
Creating a Dockerfile for a Flask application involves specifying the base image, installing dependencies, and defining the command to run the application.
Python: Reading and Manipulating CSV Files for Machine LearningExample of a Dockerfile for a Flask application:
# Use the official Python image as a base
FROM python:3.8-slim
# Set the working directory
WORKDIR /app
# Copy the requirements file and install dependencies
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
# Copy the application code
COPY . .
# Expose the port the app runs on
EXPOSE 8000
# Define the command to run the application
CMD ["gunicorn", "-w", "4", "-b", "0.0.0.0:8000", "app:app"]
Implementing Load Balancing
As your application scales, it is essential to implement load balancing to distribute incoming traffic across multiple instances of the Flask application. Load balancers help improve performance, availability, and reliability by ensuring that no single instance is overwhelmed by traffic.
Using services like Nginx or cloud-based load balancers from providers like AWS, Google Cloud, or Azure can simplify the process of setting up and managing load balancing.
Example of configuring Nginx as a load balancer:
http {
upstream flask_app {
server app1.example.com:8000;
server app2.example.com:8000;
server app3.example.com:8000;
}
server {
listen 80;
location / {
proxy_pass http://flask_app;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
}
Ensuring Security and Reliability
Securing your Flask application involves implementing authentication and authorization mechanisms to control access to resources. Using libraries like Flask-Login and Flask-JWT-Extended can help simplify the process of adding user authentication and managing user sessions.
Example of implementing JWT authentication with Flask:
from flask import Flask, request, jsonify
from flask_jwt_extended import JWTManager, create_access_token, jwt_required
app = Flask(__name__)
app.config['JWT_SECRET_KEY'] = 'your_jwt_secret_key'
jwt = JWTManager(app)
users = {'user1': 'password1'}
@app.route('/login', methods=['POST'])
def login():
username = request.json.get('username')
password = request.json.get('password')
if users.get(username) == password:
access_token = create_access_token(identity=username)
return jsonify(access_token=access_token)
return jsonify({"msg": "Bad username or password"}), 401
@app.route('/protected', methods=['GET'])
@jwt_required()
def protected():
return jsonify({"msg": "Access granted"})
if __name__ == '__main__':
app.run(debug=True)
Handling Errors and Logging
Robust error handling and logging are critical for maintaining the reliability of your Flask application. Implementing error handlers ensures that users receive meaningful error messages, and logging helps in diagnosing and resolving issues.
Using Python’s built-in logging module and Flask’s error handling mechanisms, you can create a comprehensive logging and error management system.
Example of setting up error handling and logging in Flask:
import logging
from flask import Flask, jsonify
app = Flask(__name__)
# Set up logging
logging.basicConfig(level=logging.INFO)
@app.errorhandler(404)
def not_found(error):
app.logger.error(f"Not Found: {error}")
return jsonify({"error": "Not Found"}), 404
@app.errorhandler(500)
def internal_error(error):
app.logger.error(f"Server Error: {error}")
return jsonify({"error": "Internal Server Error"}), 500
@app.route('/divide')
def divide():
try:
result = 1 / 0
except ZeroDivisionError as e:
app.logger.error(f"Error: {e}")
return jsonify({"error": "Division by zero is not allowed"}), 400
return jsonify({"result": result})
if __name__ == '__main__':
app.run(debug=True)
Testing and Monitoring
Thorough testing and monitoring are essential for ensuring that your Flask application operates reliably in production. Writing unit tests and integration tests helps identify and fix issues early. Using testing frameworks like pytest can streamline the testing process.
Monitoring tools like Prometheus, Grafana, and New Relic provide insights into application performance, helping you detect and address potential problems before they impact users.
Example of a unit test for a Flask route using pytest:
import pytest
from app import app
@pytest.fixture
def client():
with app.test_client() as client:
yield client
def test_predict(client):
response = client.post('/predict', json={'features': [1, 2, 3, 4]})
json_data = response.get_json()
assert response.status_code == 200
assert 'prediction' in json_data
Deploying machine learning models with Flask requires careful planning and adherence to best practices to ensure performance, scalability, security, and reliability. By following the guidelines outlined in this article, you can create robust Flask applications that effectively serve your ML models, providing valuable insights and predictions to users.
If you want to read more articles similar to Flask: Best Practices for Deploying ML Models, you can visit the Tools category.
You Must Read