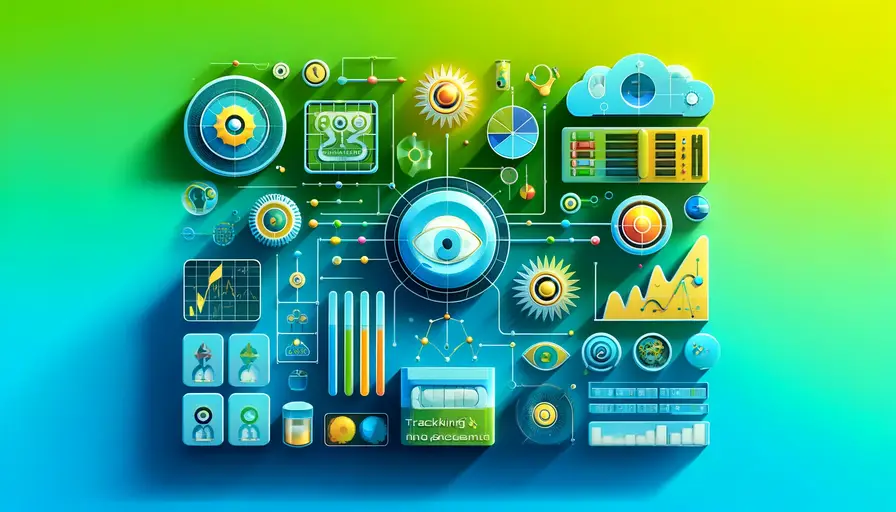
Popular R Package for Supervised Learning Tasks: Caret
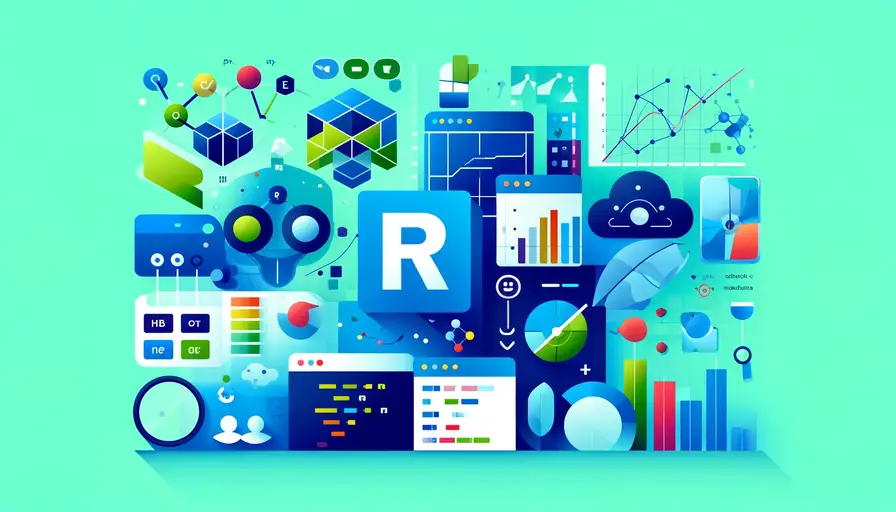
Caret
The caret
package in R is a comprehensive tool designed to streamline the process of creating predictive models. Short for Classification And REgression Training, caret
encompasses numerous functions that facilitate data preparation, model training, and model evaluation, making it a favorite among data scientists and statisticians for supervised learning tasks.
Overview of caret
caret provides a unified interface to a vast array of machine learning algorithms available in R. It supports various stages of the machine learning workflow, from data preprocessing to model tuning and validation. The package aims to simplify the complexity associated with model training, making it accessible to both novice and experienced practitioners.
Importance of caret
The importance of caret lies in its ability to integrate different models and preprocessing steps into a cohesive workflow. By providing a consistent interface, caret allows users to focus on model development and tuning without worrying about the intricacies of individual algorithms.
Example: Installing caret
Here’s an example of installing the caret
package in R:

# Install caret package
install.packages("caret")
# Load caret package
library(caret)
Data Preprocessing with caret
Data preprocessing is a crucial step in the machine learning pipeline. The caret
package offers several functions to handle common preprocessing tasks such as data imputation, normalization, and feature engineering. These functions help prepare the data for model training, ensuring that the models perform optimally.
Imputing Missing Values
Imputing missing values is essential to handle incomplete datasets. The preProcess
function in caret
can be used to impute missing values using various methods such as mean, median, or k-nearest neighbors.
Normalization and Scaling
Normalization and scaling ensure that all features contribute equally to the model. caret
provides options for standardization, range scaling, and other transformations that can be easily applied to the data.
Example: Data Preprocessing in caret
Here’s an example of data preprocessing using the caret
package:

# Load dataset
data(iris)
# Create a preProcess object
preProc <- preProcess(iris[, -5], method = c("center", "scale"))
# Apply preprocessing
iris_transformed <- predict(preProc, iris[, -5])
# Display transformed data
head(iris_transformed)
Training Models with caret
Training machine learning models is a core functionality of caret
. The package supports a wide range of algorithms, including linear regression, decision trees, support vector machines, and more. The train
function in caret
simplifies the process of training models by providing a unified interface.
Supported Algorithms
Supported algorithms in caret
span across various categories, including linear models, tree-based methods, ensemble techniques, and more. This diversity allows users to experiment with different approaches and select the best-performing model for their task.
Training with train Function
The train
function in caret
is a powerful tool that automates the model training process. It handles cross-validation, parameter tuning, and model fitting, providing a streamlined workflow for developing predictive models.
Example: Training a Model with caret
Here’s an example of training a decision tree model using the train
function in caret
:

# Load dataset
data(iris)
# Set seed for reproducibility
set.seed(123)
# Train a decision tree model
model <- train(Species ~ ., data = iris, method = "rpart")
# Print model summary
print(model)
Cross-Validation and Hyperparameter Tuning
Cross-validation and hyperparameter tuning are critical for assessing model performance and optimizing model parameters. The caret
package provides built-in support for these tasks, enabling users to fine-tune their models for better accuracy and robustness.
Importance of Cross-Validation
Cross-validation is essential for evaluating model performance in a reliable manner. By partitioning the data into training and validation sets multiple times, cross-validation provides an unbiased estimate of model performance.
Hyperparameter Tuning with trainControl
The trainControl
function in caret
allows users to specify the cross-validation method and configure hyperparameter tuning. This function is integral to the train
workflow, enabling systematic optimization of model parameters.
Example: Cross-Validation and Tuning with caret
Here’s an example of performing cross-validation and hyperparameter tuning using caret
:

# Load dataset
data(iris)
# Set seed for reproducibility
set.seed(123)
# Define trainControl
train_control <- trainControl(method = "cv", number = 10)
# Train a model with hyperparameter tuning
model <- train(Species ~ ., data = iris, method = "rf", trControl = train_control, tuneLength = 5)
# Print model summary
print(model)
Evaluating Model Performance
Evaluating model performance is a crucial step in the machine learning process. The caret
package offers various metrics and visualization tools to assess the accuracy, precision, recall, and other performance indicators of trained models.
Common Evaluation Metrics
Evaluation metrics such as accuracy, precision, recall, F1 score, and ROC-AUC are supported by caret
. These metrics provide comprehensive insights into model performance, helping users to select the best model for their task.
Visualization Tools
Visualization tools in caret
include functions for plotting confusion matrices, ROC curves, and variable importance. These visualizations help in interpreting model results and identifying areas for improvement.
Example: Evaluating Model Performance with caret
Here’s an example of evaluating model performance using the caret
package:

# Load dataset
data(iris)
# Set seed for reproducibility
set.seed(123)
# Train a decision tree model
model <- train(Species ~ ., data = iris, method = "rpart")
# Predict on training data
predictions <- predict(model, iris)
# Confusion matrix
conf_matrix <- confusionMatrix(predictions, iris$Species)
print(conf_matrix)
# Plot variable importance
var_imp <- varImp(model)
plot(var_imp)
Feature Engineering with caret
Feature engineering involves creating new features or transforming existing ones to improve model performance. The caret
package includes functions for feature selection, extraction, and transformation, facilitating the creation of more powerful predictive models.
Feature Selection
Feature selection involves identifying the most relevant features for the model. caret
provides functions such as rfe
(recursive feature elimination) to systematically select important features based on their contribution to model performance.
Feature Extraction
Feature extraction transforms raw data into informative features. Techniques such as principal component analysis (PCA) are supported by caret
to reduce dimensionality and enhance model interpretability.
Example: Feature Engineering with caret
Here’s an example of performing feature selection using the caret
package:

# Load dataset
data(iris)
# Set seed for reproducibility
set.seed(123)
# Define control using cross-validation
control <- rfeControl(functions = rfFuncs, method = "cv", number = 10)
# Perform recursive feature elimination
results <- rfe(iris[,1:4], iris[,5], sizes = c(1:4), rfeControl = control)
# Print results
print(results)
# List the chosen features
predictors(results)
Advanced Topics in caret
The caret
package also supports advanced topics in machine learning, including ensemble learning, time series forecasting, and custom model development. These advanced features allow users to tackle more complex problems and improve model performance.
Ensemble Learning
Ensemble learning combines multiple models to create a stronger predictor. The caret
package supports various ensemble techniques, such as bagging, boosting, and stacking, to enhance model accuracy and robustness.
Time Series Forecasting
Time series forecasting involves predicting future values based on historical data. caret
provides functions to handle time series data, enabling users to develop forecasting models for various applications.
Example: Ensemble Learning with caret
Here’s an example of implementing ensemble learning using the caret
package:
# Load dataset
data(iris)
# Set seed for reproducibility
set.seed(123)
# Define control using cross-validation
train_control <- trainControl(method = "cv", number = 10)
# Train a random forest model
rf_model <- train(Species ~ ., data = iris, method = "rf", trControl = train_control)
# Train a boosting model
gbm_model <- train(Species ~ ., data = iris, method = "gbm", trControl = train_control, verbose = FALSE)
# Stack models
stack_control <- trainControl(method = "cv", number = 10)
stacked_model <- caretStack(models = list(rf = rf_model, gbm = gbm_model), method = "glm", trControl = stack_control)
# Print model summary
print(stacked_model)
Practical Applications of caret
The versatility of the caret
package makes it suitable for a wide range of practical applications. From predictive maintenance in manufacturing to fraud detection in finance, caret
provides the tools necessary to build robust and accurate models.
Predictive Maintenance
Predictive maintenance involves predicting equipment failures before they occur. Using caret
, data scientists can develop models that analyze sensor data to forecast potential breakdowns and schedule maintenance activities proactively.
Fraud Detection
Fraud detection in finance relies on identifying unusual patterns in transaction data. caret
enables the development of classification models that can distinguish between legitimate and fraudulent transactions, helping financial institutions reduce losses.
Example: Fraud Detection with caret
Here’s an example of developing a fraud detection model using the caret
package:
# Load dataset
data(fraudData)
# Set seed for reproducibility
set.seed(123)
# Train a logistic regression model
model <- train(fraud ~ ., data = fraudData, method = "glm", family = binomial())
# Predict on new data
new_data <- data.frame(...)
predictions <- predict(model, new_data)
# Print predictions
print(predictions)
Customizing caret for Specific Needs
While caret
offers a wide range of functionalities out of the box, it also allows for customization to meet specific needs. Users can define custom models, preprocessing steps, and evaluation metrics to tailor the package to their unique requirements.
Defining Custom Models
Defining custom models in caret
involves specifying the model's training, prediction, and parameter tuning functions. This flexibility allows users to integrate new algorithms and techniques into the caret
framework.
Custom Preprocessing Steps
Custom preprocessing steps can be added to the caret
workflow to handle specific data transformation needs. Users can define their own functions to preprocess data in ways that are not covered by the built-in methods.
Example: Custom Model in caret
Here’s an example of defining a custom model in the caret
package:
# Define custom model
customModel <- list(
type = "Classification",
library = NULL,
loop = NULL,
parameters = data.frame(parameter = c("parameter1", "parameter2"), class = c("numeric", "numeric"), label = c("Parameter 1", "Parameter 2")),
grid = function(x, y, len = NULL, search = "grid") {
expand.grid(parameter1 = seq(0, 1, length = len), parameter2 = seq(0, 1, length = len))
},
fit = function(x, y, wts, param, lev, last, classProbs, ...) {
model <- train(x, y, method = "rf", tuneGrid = data.frame(parameter1 = param$parameter1, parameter2 = param$parameter2), ...)
return(model)
},
predict = function(modelFit, newdata, submodels = NULL) {
predict(modelFit, newdata)
},
prob = function(modelFit, newdata, submodels = NULL) {
predict(modelFit, newdata, type = "prob")
}
)
# Train custom model
model <- train(Species ~ ., data = iris, method = customModel)
# Print model summary
print(model)
The caret
package is an indispensable tool for supervised learning tasks in R. Its comprehensive suite of functionalities, from data preprocessing to model training and evaluation, makes it a versatile and powerful package for data scientists and statisticians. By understanding and leveraging the features of caret
, users can streamline their machine learning workflows and build robust, accurate models for a wide range of applications. Whether you're working on predictive maintenance, fraud detection, or any other predictive modeling task, caret
provides the tools and flexibility needed to achieve your goals.
If you want to read more articles similar to Popular R Package for Supervised Learning Tasks: Caret, you can visit the Tools category.
You Must Read