
Is Machine Learning Non-parametric: Exploring Model Flexibility
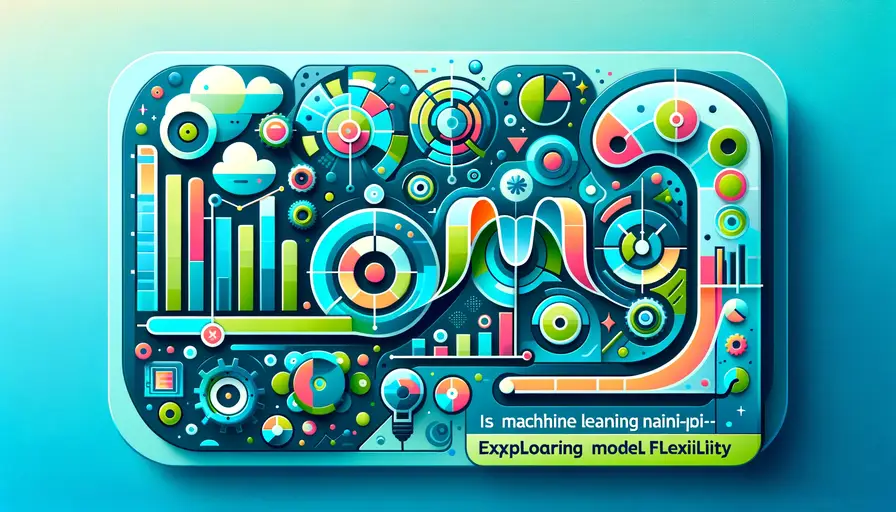
Content
Understanding Non-parametric Models
Non-parametric models in machine learning are flexible and do not assume a fixed form for the underlying function of the data. Unlike parametric models, which have a fixed number of parameters, non-parametric models can grow in complexity with the size of the dataset.
What Are Non-parametric Models?
Non-parametric models are types of models that make fewer assumptions about the data's distribution. They can adapt their complexity based on the dataset, which makes them highly flexible. This flexibility allows them to model complex patterns that parametric models might miss.
Advantages of Non-parametric Models
Non-parametric models offer several advantages, including the ability to capture complex relationships in the data, fewer assumptions about the data distribution, and the ability to model data with high variance and non-linear relationships.
Example: K-Nearest Neighbors (KNN) Algorithm
Here’s an example of using the K-Nearest Neighbors algorithm, a non-parametric model, in Python:
Comparing Machine Learning Techniques: Understanding Differencesfrom sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
# Load dataset
data = load_iris()
X = data.data
y = data.target
# Split dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train KNN model
knn = KNeighborsClassifier(n_neighbors=3)
knn.fit(X_train, y_train)
# Make predictions
predictions = knn.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, predictions)
print(f"Model Accuracy: {accuracy}")
Characteristics of Non-parametric Models
Non-parametric models have unique characteristics that distinguish them from parametric models. Understanding these characteristics helps in selecting the appropriate model for different machine learning tasks.
Flexibility and Adaptability
Non-parametric models are highly flexible and can adapt their complexity to fit the data. This adaptability allows them to model intricate patterns and relationships within the data, which parametric models may not capture.
No Assumption of Data Distribution
Unlike parametric models, non-parametric models do not assume a specific distribution for the data. This makes them suitable for a wide range of datasets, including those with unknown or complex distributions.
Example: Decision Trees
Here’s an example of using Decision Trees, a non-parametric model, in Python:
Analysis of Popular Off-the-Shelf Machine Learning Modelsfrom sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
from sklearn.metrics import accuracy_score
# Load dataset
data = load_iris()
X = data.data
y = data.target
# Split dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train Decision Tree model
tree = DecisionTreeClassifier()
tree.fit(X_train, y_train)
# Make predictions
predictions = tree.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, predictions)
print(f"Model Accuracy: {accuracy}")
Types of Non-parametric Models
Several types of non-parametric models are commonly used in machine learning. These models are versatile and can be applied to various tasks such as classification, regression, and clustering.
K-Nearest Neighbors (KNN)
K-Nearest Neighbors (KNN) is a simple, yet effective non-parametric algorithm used for classification and regression. It classifies a data point based on the majority class among its k-nearest neighbors.
Example: KNN for Regression
Here’s an example of using KNN for regression in Python:
from sklearn.datasets import load_boston
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsRegressor
from sklearn.metrics import mean_squared_error
# Load dataset
data = load_boston()
X = data.data
y = data.target
# Split dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train KNN Regressor model
knn = KNeighborsRegressor(n_neighbors=5)
knn.fit(X_train, y_train)
# Make predictions
predictions = knn.predict(X_test)
# Evaluate model
mse = mean_squared_error(y_test, predictions)
print(f"Mean Squared Error: {mse}")
Decision Trees
Decision Trees are versatile non-parametric models used for classification and regression. They split the data into subsets based on feature values, creating a tree-like structure that represents decisions.
Building Machine Learning AIExample: Decision Trees for Classification
Here’s an example of using Decision Trees for classification in Python:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
from sklearn.metrics import accuracy_score
# Load dataset
data = load_iris()
X = data.data
y = data.target
# Split dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train Decision Tree model
tree = DecisionTreeClassifier()
tree.fit(X_train, y_train)
# Make predictions
predictions = tree.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, predictions)
print(f"Model Accuracy: {accuracy}")
Support Vector Machines (SVM) with RBF Kernel
Support Vector Machines (SVM) with a radial basis function (RBF) kernel is a powerful non-parametric method for classification and regression. The RBF kernel maps data into a higher-dimensional space, allowing SVM to handle non-linear relationships.
Example: SVM with RBF Kernel
Here’s an example of using SVM with an RBF kernel in Python:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# Load dataset
data = load_iris()
X = data.data
y = data.target
# Split dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train SVM model with RBF kernel
svm = SVC(kernel='rbf')
svm.fit(X_train, y_train)
# Make predictions
predictions = svm.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, predictions)
print(f"Model Accuracy: {accuracy}")
Applications of Non-parametric Models
Non-parametric models are widely used in various applications due to their flexibility and ability to handle complex data. They are particularly useful in scenarios where the underlying data distribution is unknown or non-linear.
The Role of Abstract Algebra in Data Analysis for Machine LearningMedical Diagnosis
Non-parametric models are extensively used in medical diagnosis to identify diseases based on patient data. Their ability to handle diverse and complex data makes them suitable for predicting health outcomes.
Example: Medical Diagnosis with KNN
Here’s an example of using KNN for medical diagnosis in Python:
from sklearn.datasets import load_breast_cancer
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
# Load dataset
data = load_breast_cancer()
X = data.data
y = data.target
# Split dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train KNN model
knn = KNeighborsClassifier(n_neighbors=5)
knn.fit(X_train, y_train)
# Make predictions
predictions = knn.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, predictions)
print(f"Model Accuracy: {accuracy}")
Financial Forecasting
In finance, non-parametric models are used for forecasting stock prices, credit scoring, and risk assessment. These models can adapt to the volatile and complex nature of financial data.
Example: Financial Forecasting with Decision Trees
Here’s an example of using Decision Trees for financial forecasting in Python:
KNN Machine Learning in R: A Syntax Guideimport pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeRegressor
from sklearn.metrics import mean_squared_error
# Load dataset
data = pd.read_csv('financial_data.csv') # Example CSV file
X = data.drop(columns=['target'])
y = data['target']
# Split dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train Decision Tree Regressor model
tree = DecisionTreeRegressor()
tree.fit(X_train, y_train)
# Make predictions
predictions = tree.predict(X_test)
# Evaluate model
mse = mean_squared_error(y_test, predictions)
print(f"Mean Squared Error: {mse}")
Image Recognition
Non-parametric models, especially those combined with deep learning techniques, are used in image recognition tasks. Their ability to handle high-dimensional data and capture intricate patterns makes them ideal for this application.
Example: Image Recognition with SVM
Here’s an example of using SVM for image recognition in Python:
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# Load dataset
digits = datasets.load_digits()
X = digits.data
y = digits.target
# Split dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train SVM model
svm = SVC(kernel='rbf')
svm.fit(X_train, y_train)
# Make predictions
predictions = svm.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, predictions)
print(f"Model Accuracy: {accuracy}")
Challenges of Non-parametric Models
Despite their advantages, non-parametric models come with challenges that need to be addressed for effective implementation.
Computational Complexity
Non-parametric models can be computationally intensive, especially with large datasets. Their flexibility and adaptability often come at the cost of increased computational resources and time.
Bayesian Machine Learning for AB Testing with Python TechniquesExample: Handling Computational Complexity
Here’s an example of using dimensionality reduction to mitigate computational complexity:
from sklearn.decomposition import PCA
from sklearn.neighbors import KNeighborsClassifier
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load dataset
data = load_iris()
X = data.data
y = data.target
# Reduce dimensionality
pca = PCA(n_components=2)
X_reduced = pca.fit_transform(X)
# Split dataset
X_train, X_test, y_train, y_test = train_test_split(X_reduced, y, test_size=0.2, random_state=42)
# Train KNN model
knn = KNeighborsClassifier(n_neighbors=3)
knn.fit(X_train, y_train)
# Make predictions
predictions = knn.predict(X_test)
# Evaluate model
accuracy = accuracy_score(y_test, predictions)
print(f"Model Accuracy: {accuracy}")
Overfitting
Non-parametric models are prone to overfitting, especially with small datasets. They can capture noise in the data, leading to poor generalization to new data.
Example: Preventing Overfitting
Here’s an example of using cross-validation to prevent overfitting:
from sklearn.model_selection import cross_val_score
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
# Load dataset
data = load_iris()
X = data.data
y = data.target
# Train Decision Tree model
tree = DecisionTreeClassifier()
# Perform cross-validation
scores = cross_val_score(tree, X, y, cv=5)
print(f"Cross-Validation Scores: {scores}")
print(f"Mean Cross-Validation Score: {scores.mean()}")
Scalability
Scalability can be a concern with non-parametric models when dealing with extremely large datasets. Efficiently handling and processing large volumes of data requires careful consideration and optimization.
Example: Improving Scalability
Here’s an example of using a subset of data to improve scalability:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# Load dataset
data = load_iris()
X = data.data
y = data.target
# Use a subset of data
X_subset, _, y_subset, _ = train_test_split(X, y, test_size=0.8, random_state=42)
# Train SVM model
svm = SVC(kernel='rbf')
svm.fit(X_subset, y_subset)
# Make predictions
predictions = svm.predict(X)
# Evaluate model
accuracy = accuracy_score(y, predictions)
print(f"Model Accuracy: {accuracy}")
Non-parametric models offer significant flexibility and adaptability, making them suitable for a wide range of machine learning tasks. By not assuming a fixed form for the underlying data distribution, these models can capture complex patterns and relationships. However, they also present challenges such as computational complexity, overfitting, and scalability issues. Understanding these challenges and applying appropriate strategies can help in effectively leveraging non-parametric models for various applications. By exploring the different types of non-parametric models, their applications, and the techniques to handle their challenges, you can make informed decisions and build robust machine learning models.
If you want to read more articles similar to Is Machine Learning Non-parametric: Exploring Model Flexibility, you can visit the Artificial Intelligence category.
You Must Read